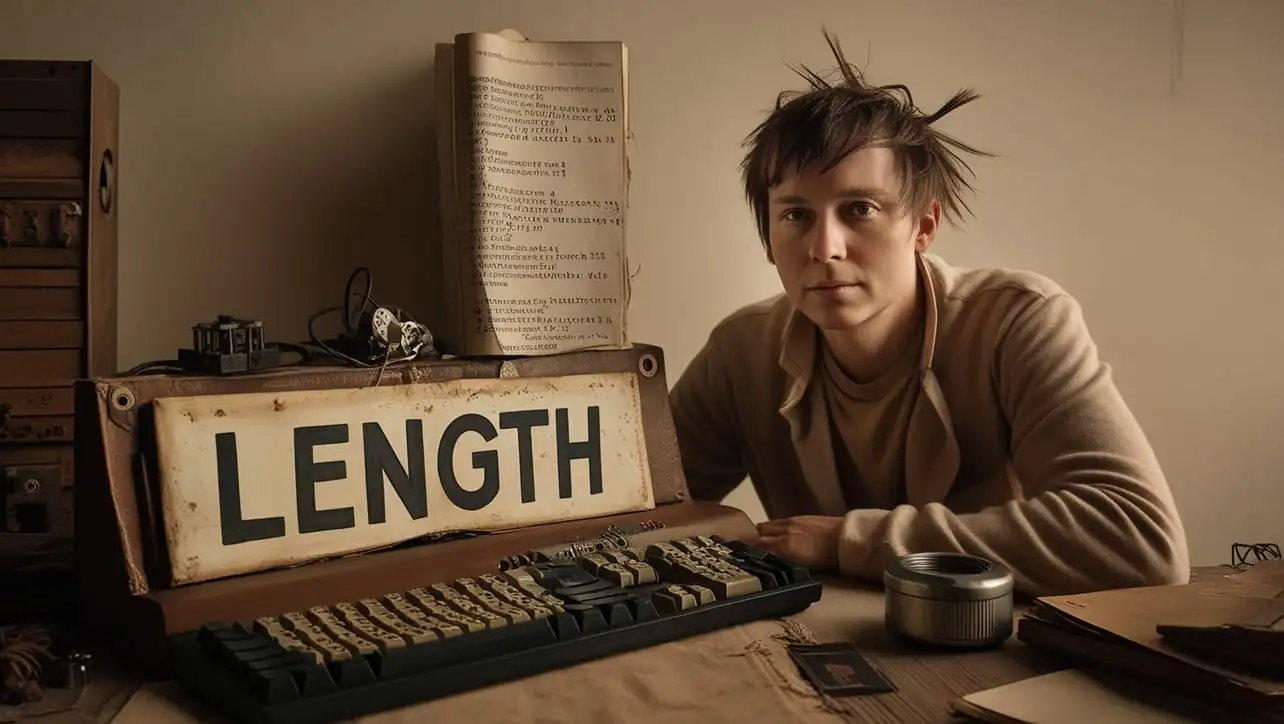
JS Array Methods
JavaScript Array filter() Method

Photo Credit to CodeToFun
🙋 Introduction
Arrays are fundamental in JavaScript, and the filter()
method stands out as a powerful tool for selectively extracting elements based on certain criteria.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and real-world examples of the filter()
method, providing you with the knowledge to wield it effectively in your JavaScript projects.
🧠 Understanding filter() Method
The filter()
method creates a new array with all elements that pass a provided function's test. It's a versatile tool for extracting a subset of elements from an array that meet specific conditions.
💡 Syntax
The syntax for the filter()
method is straightforward:
array.filter(callback(element, index, array), thisArg);
- array: The array to filter.
- callback: Function is a predicate, to test each element of the array. Return true to keep the element, false otherwise.
- thisArg (Optional): Object to use as this when executing the callback.
📝 Example
Let's dive into an example to illustrate the basic usage of the filter()
method:
// Sample array of numbers
const numbers = [1, 5, 10, 15, 20];
// Using filter() to extract even numbers
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // Output: [10, 20]
In this example, the filter()
method is used to create a new array (evenNumbers) containing only the even numbers from the original array.
🏆 Best Practices
When working with the filter()
method, consider the following best practices:
Use Clear and Descriptive Callbacks:
Write clear and descriptive callback functions to make your code more readable.
example.jsCopiedconst students = [ { name: 'Alice', grade: 85 }, { name: 'Bob', grade: 92 }, { name: 'Charlie', grade: 78 }, ]; // Filtering students with grades above 80 const highPerformers = students.filter((student) => student.grade > 80);
Avoid Modifying the Original Array:
Remember that the
filter()
method does not modify the original array but creates a new one. If you need to alter the existing array, consider using other methods like map().example.jsCopied// Incorrect: Modifying the original array const modifiedArray = numbers.filter((num) => { // ... some modification logic return true; });
example.jsCopied// Correct: Using map() for modification const modifiedArray = numbers.map((num) => { // ... some modification logic return modifiedValue; });
Compatibility Checking:
Verify the compatibility of the
filter()
method in your target environments, especially if you need to support older browsers.example.jsCopied// Check if the filter() method is supported if (Array.prototype.filter) { // Use the method safely const filteredArray = numbers.filter((num) => num > 5); console.log(filteredArray); } else { console.error('filter() method not supported in this environment.'); }
📚 Use Cases
Filtering Objects in an Array:
The
filter()
method is particularly useful when dealing with arrays of objects. In the following example, it filters students based on their grades:example.jsCopiedconst students = [ { name: 'Alice', grade: 85 }, { name: 'Bob', grade: 92 }, { name: 'Charlie', grade: 78 }, ]; const highPerformers = students.filter((student) => student.grade > 80);
Removing Falsy Values:
Filtering out falsy values from an array is a common use case. Here, the
filter()
method removes null and undefined values:example.jsCopiedconst values = [10, null, 20, undefined, 30]; const filteredValues = values.filter((value) => value !== null && value !== undefined);
🎉 Conclusion
The filter()
method is a versatile and powerful tool for selectively extracting elements from arrays based on specific criteria.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the filter()
method in your JavaScript projects.
👨💻 Join our Community:
Author
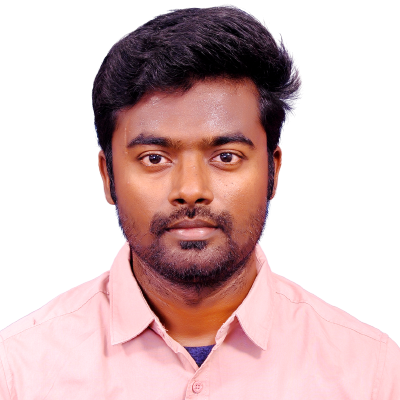
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array filter() Method), please comment here. I will help you immediately.