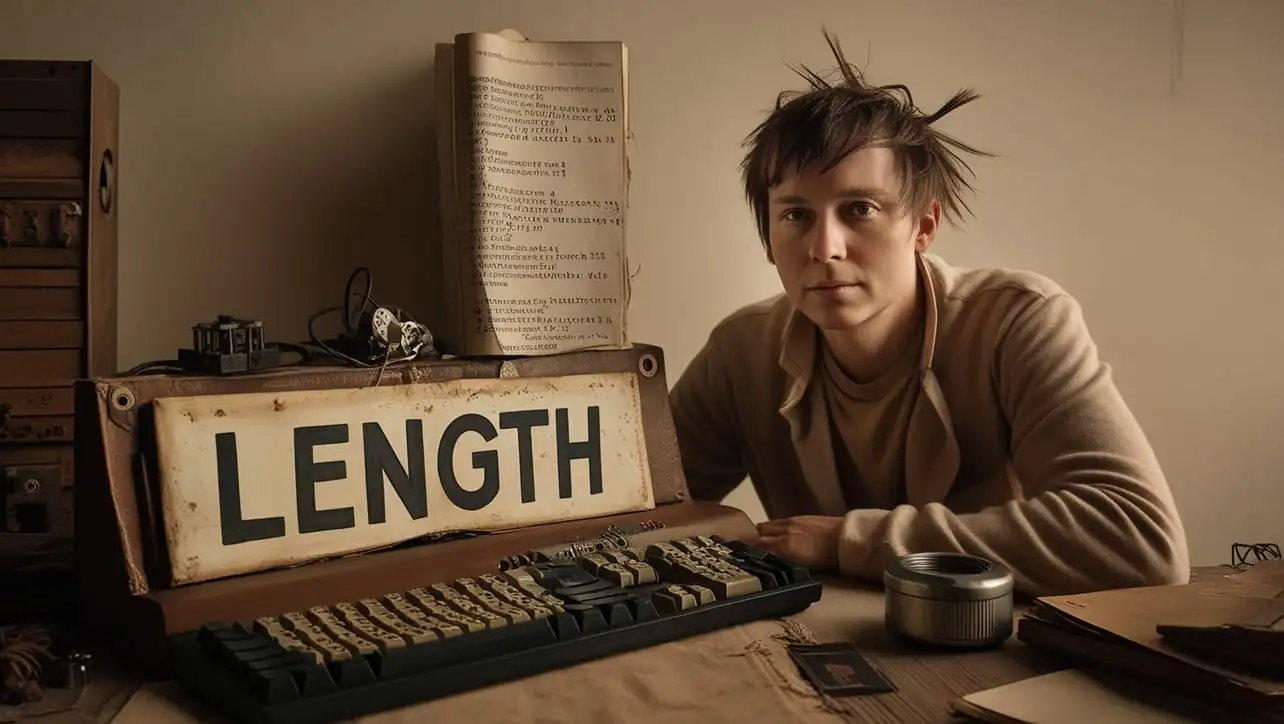
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array fill() Method
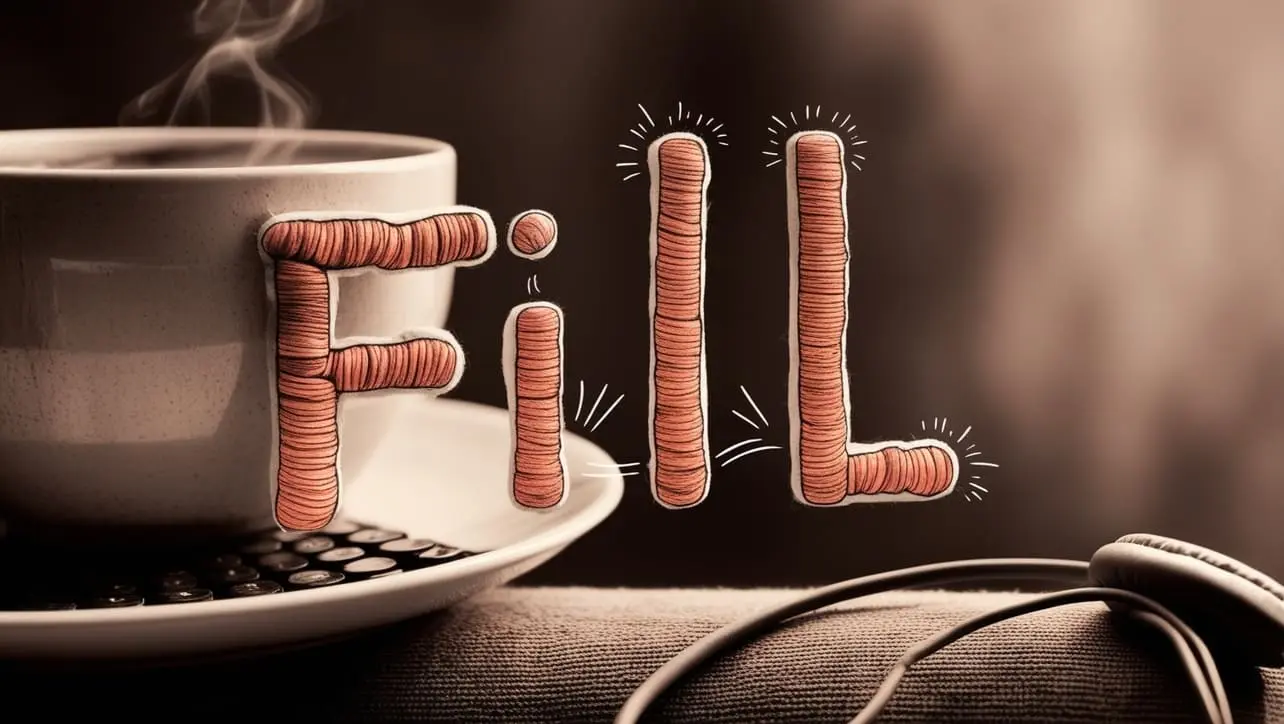
Photo Credit to CodeToFun
Introduction
JavaScript arrays are a cornerstone of data manipulation, and the fill()
method provides a convenient way to initialize or update array elements with a specified value.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the fill()
method.
Understanding fill() Method
The fill()
method changes all elements in an array to a specified value, from a start index (defaulting to 0) to an end index (defaulting to the array length). This method is particularly useful for quickly populating an array with a default value or updating specific portions of an array with a new value.
Syntax
The syntax for the fill()
method is straightforward:
array.fill(value, start, end);
- array: The array to be filled.
- value: The value to fill the array with.
- start (Optional): The index to start filling the array. Defaults to 0.
- end (Optional): The index to stop filling the array. Defaults to array length.
Example
Let's dive into a practical example to illustrate the usage of the fill()
method:
// Create an array with five elements
let numbers = [1, 2, 3, 4, 5];
// Use fill() to replace elements from index 1 to 3 with 10
numbers.fill(10, 1, 4);
console.log(numbers); // Output: [1, 10, 10, 10, 5]
In this example, the fill()
method is employed to replace elements from index 1 to 3 with the value 10.
Best Practices
When working with the fill()
method, consider the following best practices:
Immutable Approach:
If you want to create a new array with the filled values without modifying the original array, use the spread operator or Array.from().
example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const newArray = [...originalArray].fill(10, 1, 4); console.log(newArray); // Output: [1, 10, 10, 10, 5]
Understanding the End Index:
Be mindful of the end index when using
fill()
. The end index is not inclusive, so the value at the end index itself will not be replaced.example.jsCopiedconst arrayToFill = [1, 2, 3, 4, 5]; arrayToFill.fill(0, 2, 4); console.log(arrayToFill); // Output: [1, 2, 0, 0, 5]
Performance Considerations:
For very large arrays, the
fill()
method can be more efficient than manual looping for initialization.
Use Cases
Initializing an Array with Default Values:
The
fill()
method is excellent for initializing an array with default values, especially when dealing with large arrays.example.jsCopiedconst largeArray = new Array(1000).fill(0);
Resetting Elements to a Default Value:
Resetting elements to a default value is a common use case, and the
fill()
method simplifies this task.example.jsCopiedlet flags = new Array(8).fill(false); // Update specific flags flags.fill(true, 2, 5);
Conclusion
The fill()
method is a powerful tool in the JavaScript array arsenal, enabling efficient initialization and modification of array elements.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the fill()
method in your JavaScript projects.
Join our Community:
Author
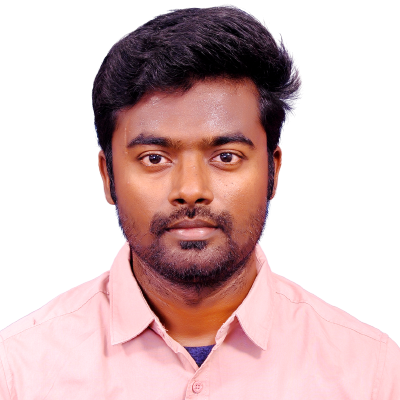
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array fill() Method), please comment here. I will help you immediately.