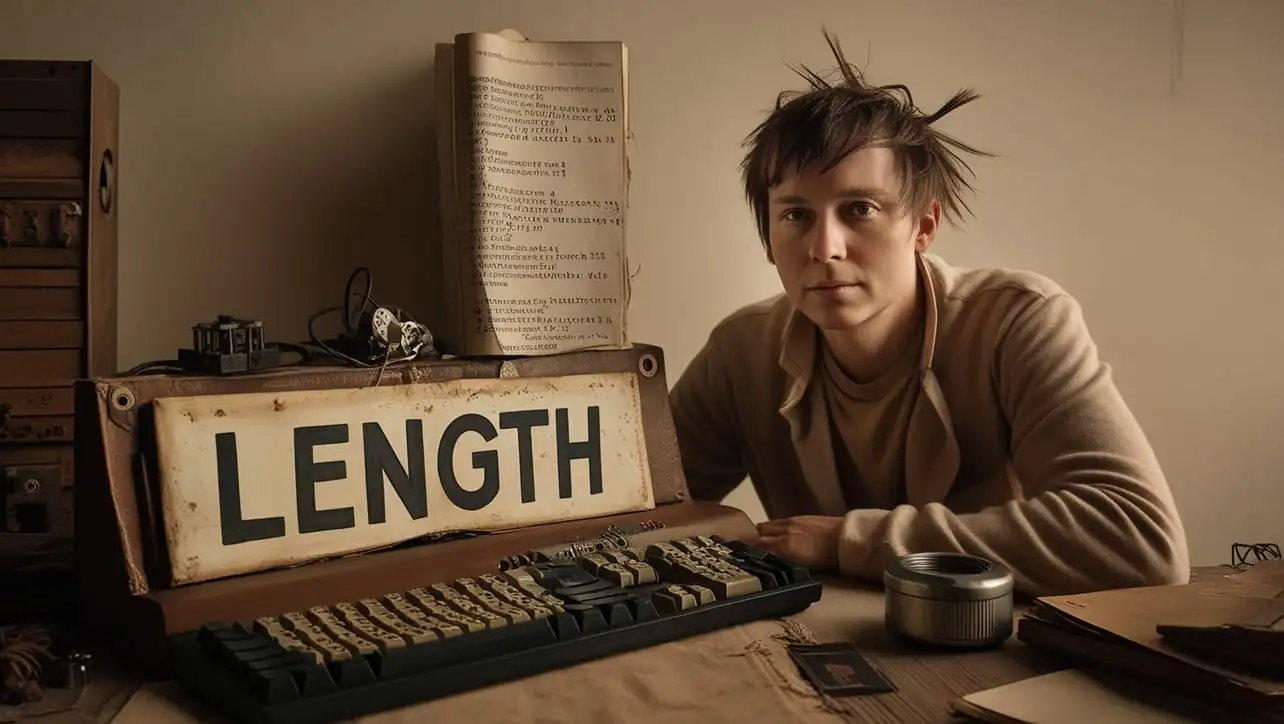
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array copyWithin() Method
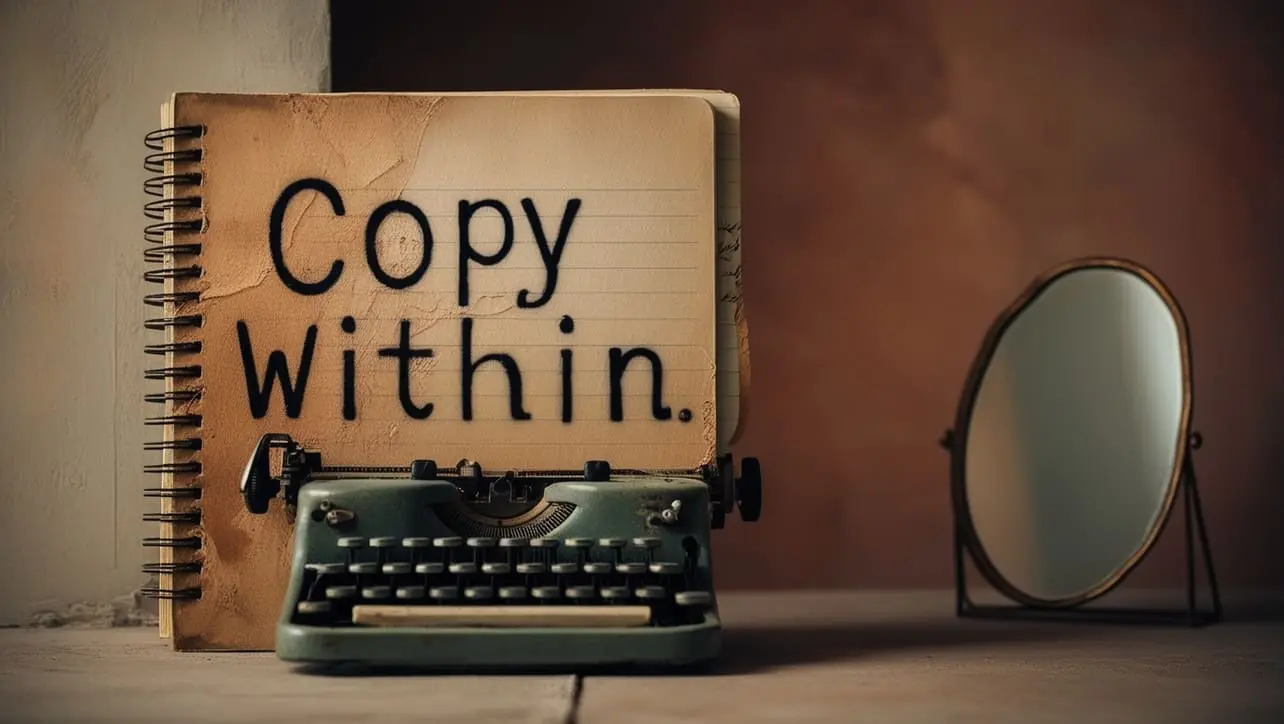
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a variety of methods for manipulating their contents, and one such method is copyWithin()
. This method provides a powerful way to copy a sequence of elements within an array.
In this guide, we'll delve into the syntax of copyWithin()
, its applications, and examples to illustrate its usage in your JavaScript code.
🧠 Understanding copyWithin() Method
The copyWithin()
method is designed for in-place modification of an array. It copies a sequence of elements within the array, overwriting the existing elements with the copied ones. This operation occurs without changing the array's length.
💡 Syntax
The syntax for the copyWithin()
method is straightforward:
array.copyWithin(target, start, end);
- array: The array to modify.
- target: The index at which to copy the sequence of elements.
- start: The index from which to start copying elements.
- end (optional): The index at which to stop copying elements.
📝 Example
Let's explore a simple example to understand how to use the copyWithin()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
// Copy elements starting from index 3 to index 6, and paste at index 0
numbers.copyWithin(0, 3, 6);
console.log(numbers); // Output: [4, 5, 6, 4, 5, 6, 7, 8, 9]
In this example, the sequence of elements from index 3 to 6 (4, 5, 6) is copied and pasted starting at index 0 of the numbers array.
🏆 Best Practices
When working with the copyWithin()
method, consider the following best practices:
Understanding Indices:
Be mindful of the indices used for the target, start, and end parameters. Ensure they are within the valid range of the array to prevent unexpected results.
example.jsCopiedconst targetIndex = 0; const startIndex = 3; const endIndex = 6; if ( targetIndex >= 0 && targetIndex < numbers.length && startIndex >= 0 && startIndex < numbers.length && endIndex >= 0 && endIndex <= numbers.length ) { numbers.copyWithin(targetIndex, startIndex, endIndex); console.log(numbers); } else { console.log('Invalid indices.'); }
Immutability Consideration:
If immutability is a concern, make a shallow copy of the array before applying
copyWithin()
to preserve the original array.example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const copiedArray = [...originalArray]; // Apply copyWithin() to the copied array copiedArray.copyWithin(0, 2, 4); console.log(originalArray); // Output: [1, 2, 3, 4, 5] console.log(copiedArray); // Output: [3, 4, 3, 4, 5]
In this example, the original array remains unchanged as
copyWithin()
is applied to a shallow copy (copiedArray).
📚 Use Cases
Shifting Elements within an Array:
The
copyWithin()
method is excellent for rearranging elements within an array without resorting to complex manual manipulation. For instance:example.jsCopied// Shift the last three elements to the beginning of the array numbers.copyWithin(0, numbers.length - 3); console.log(numbers); // Output: [7, 8, 9, 4, 5, 6, 7, 8, 9]
Repeating Patterns in an Array:
You can use
copyWithin()
to replicate a pattern within the array:example.jsCopied// Repeat the pattern [1, 2, 3] three times starting from index 3 numbers.copyWithin(3, 0, 3); console.log(numbers); // Output: [1, 2, 3, 1, 2, 3, 1, 2, 3]
🎉 Conclusion
The copyWithin()
method offers a concise and efficient way to manipulate array elements in-place.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the copyWithin()
method in your JavaScript projects.
👨💻 Join our Community:
Author
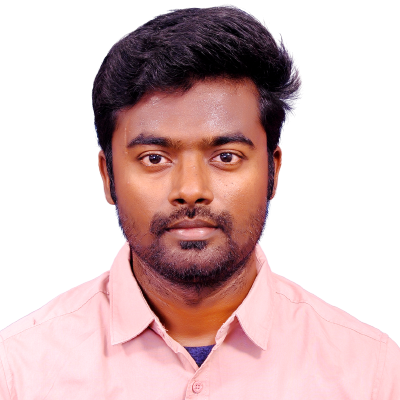
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array copyWithin() Method), please comment here. I will help you immediately.