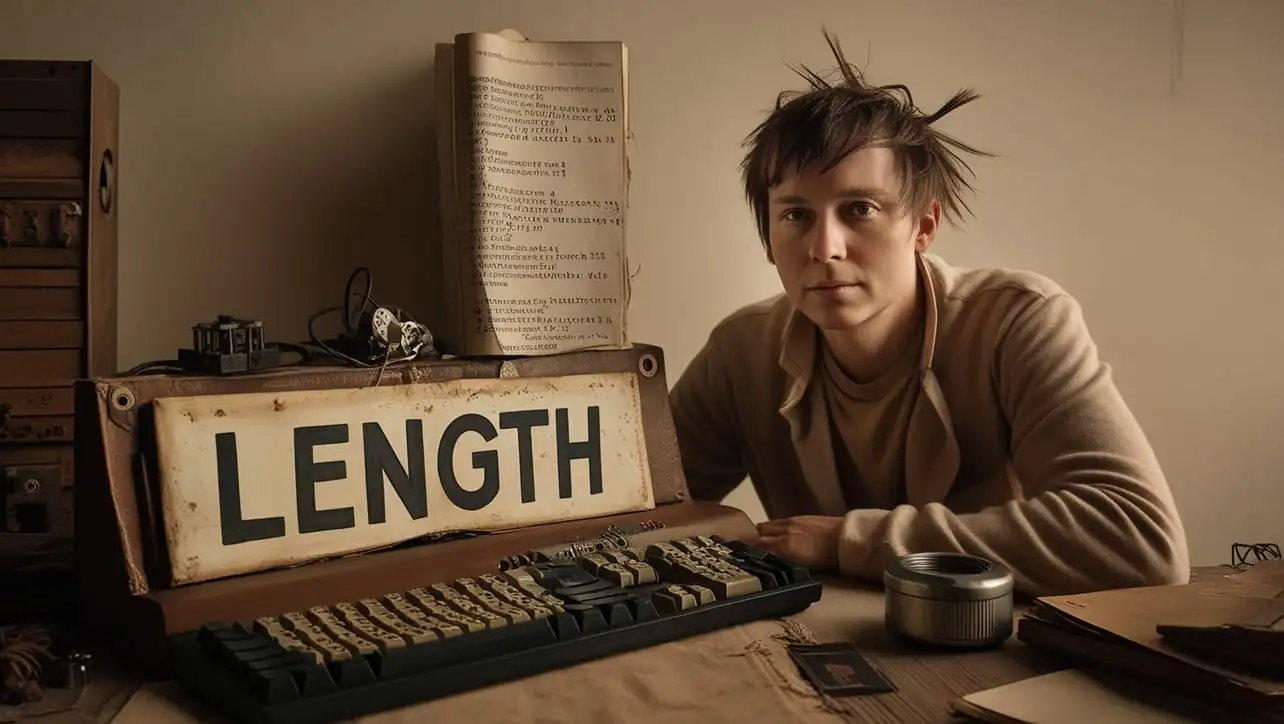
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array at() Method
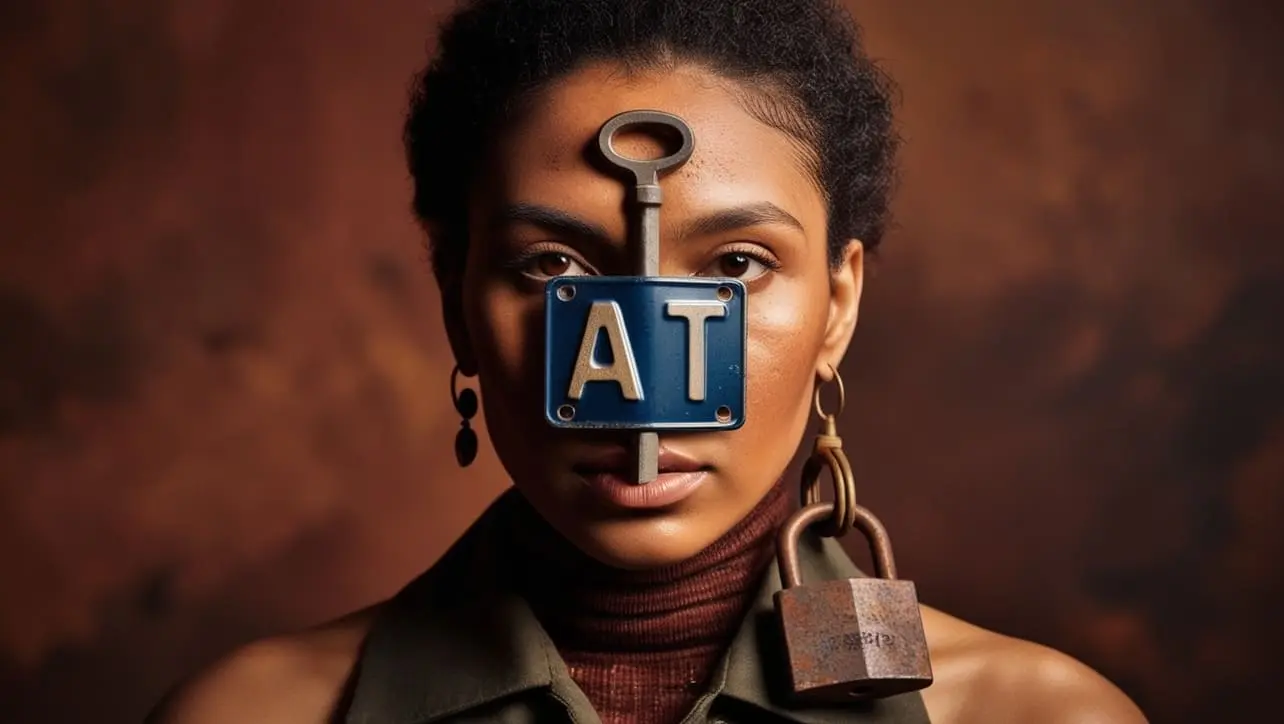
Photo Credit to CodeToFun
🙋 Introduction
Arrays are the backbone of data manipulation in JavaScript, offering a myriad of methods to streamline your coding endeavors. The at()
method is a relatively recent addition to the JavaScript Array prototype, providing an efficient and concise way to access elements at specific indices.
In this comprehensive guide, we will delve into the nuances of the at()
method, exploring its syntax, best practices, and practical use cases.
🧠 Understanding at() Method
The at()
method stands out for its simplicity, allowing developers to access array elements by directly specifying the index. This method offers an alternative to traditional square bracket notation, bringing clarity and brevity to your code.
💡 Syntax
The syntax for the at()
method is straightforward:
array.at(index);
- array: The array from which you want to retrieve an element.
- index: The zero-based index of the element you wish to access.
📝 Example
To grasp the essence of the at()
method, consider the following example:
// Sample array of programming languages
const languages = ['JavaScript', 'Python', 'Java', 'C++', 'Ruby'];
// Using at() to access elements
const firstLanguage = languages.at(0);
const thirdLanguage = languages.at(2);
console.log(firstLanguage); // Output: 'JavaScript'
console.log(thirdLanguage); // Output: 'Java'
In this example, we directly access the elements at indices 0 and 2 of the languages array using the at()
method.
🏆 Best Practices
When working with the at()
method, consider the following best practices:
Error Handling:
Always validate that the specified index is within the array bounds to prevent potential errors.
example.jsCopiedconst indexToCheck = 5; if (indexToCheck >= 0 && indexToCheck < languages.length) { const language = languages.at(indexToCheck); console.log(language); } else { console.log('Index out of bounds.'); }
Compatibility Check:
Verify the compatibility of the
at()
method in your target environments, especially if supporting older browsers.
📚 Use Cases
Navigating Arrays in Reverse:
Exploit the
at()
method's ability to handle negative indices to easily retrieve elements from the end of an array:example.jsCopiedconst lastLanguage = languages.at(-1); console.log(lastLanguage); // Output: 'Ruby'
Selective Element Access in a Loop:
Harness the
at()
method when iterating through an array and selectively accessing elements based on conditions:example.jsCopiedfor (let i = 0; i < languages.length; i++) { if (i % 2 === 0) { const currentLanguage = languages.at(i); console.log(`Language at even index ${i}: ${currentLanguage}`); } }
In this example, we use
at()
to access elements only at even indices during the loop.
🎉 Conclusion
The at()
method introduces a streamlined approach to array element access in JavaScript, offering a concise syntax and versatility.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the at()
method in your JavaScript projects.
👨💻 Join our Community:
Author
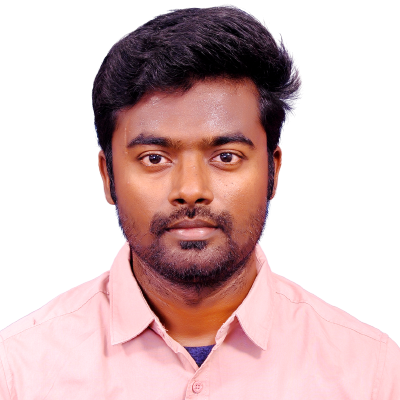
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array at() Method), please comment here. I will help you immediately.