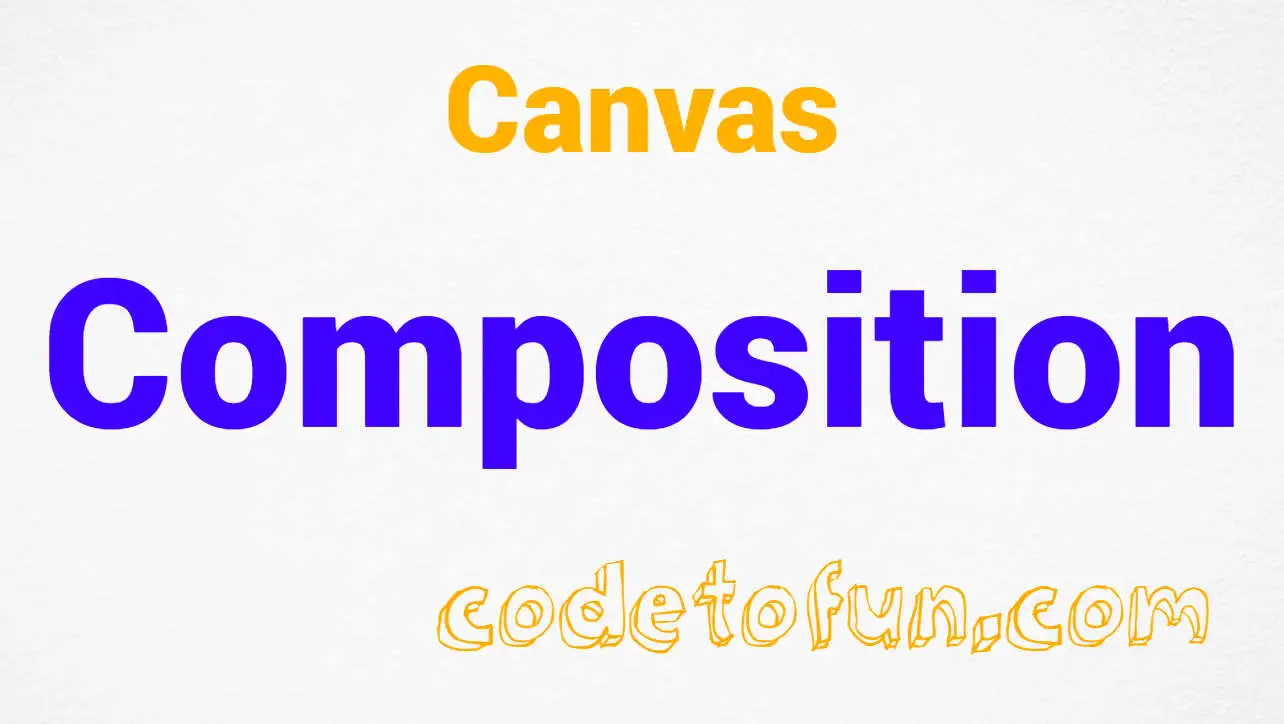
Canvas
- Canvas Introduction
- Canvas Draw Rectangles
- Canvas Draw Lines
- Canvas Draw Bezier
- Canvas Draw Quadratic
- Canvas Draw Arc
- Canvas Draw Paths
- Canvas Manipulating Images
- Canvas Linear Gradients
- Canvas Radial Gradients
- Canvas Text
- Canvas Pattern
- Canvas Shadow
- Canvas States
- Canvas Translate
- Canvas Rotate
- Canvas Scale
- Canvas Transform
- Canvas Composition
Canvas Transform
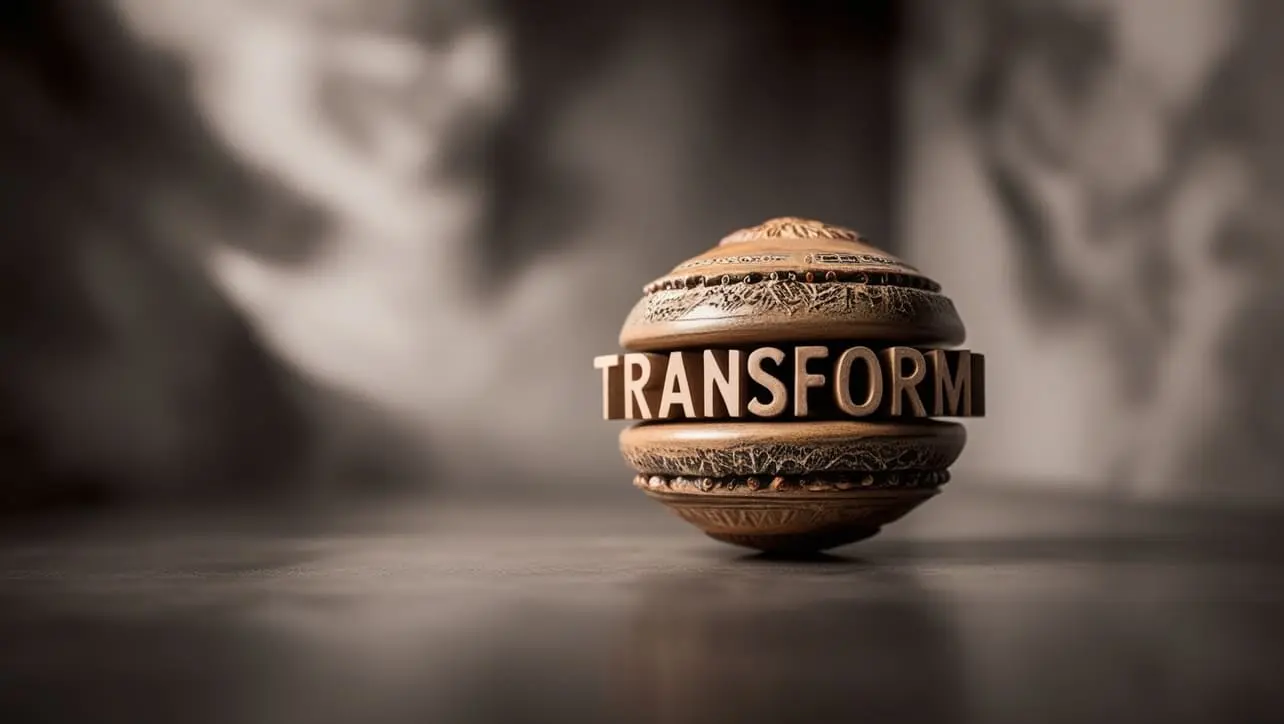
Photo Credit to CodeToFun
🙋 Introduction
Canvas transformation is a powerful feature in HTML5 that allows developers to manipulate the drawing context of the <canvas> element. By applying transformations, such as scaling, rotation, translation, and skewing, developers can create complex and dynamic visual effects.
In this guide, we'll explore the syntax, methods, and examples of canvas transformations.
💡 Syntax
The canvas transformation functions are part of the 2D rendering context, accessed through the getContext('2d') method. Here's a basic syntax overview:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Translate
ctx.translate(x, y);
// Scale
ctx.scale(scaleX, scaleY);
// Rotate
ctx.rotate(angle);
// Skew
ctx.transform(a, b, c, d, e, f);
// Reset transformations
ctx.setTransform(1, 0, 0, 1, 0, 0);
🧰 Methods and Parameters
- translate(x, y): Moves the origin of the canvas to the specified (x, y) coordinates.
- scale(scaleX, scaleY): Scales the canvas by the given factors along the x and y axes.
- rotate(angle): Rotates the canvas around the origin by the specified angle (in radians).
- transform(a, b, c, d, e, f): Applies a custom 2D transformation matrix to the canvas.
- setTransform(a, b, c, d, e, f): Resets the current transformation matrix to the identity matrix.
📄 Example
Let's demonstrate a simple canvas transformation:
<canvas id="myCanvas" width="200" height="200"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Translate the origin to (100, 100)
ctx.translate(100, 100);
// Rotate by 45 degrees
ctx.rotate(Math.PI / 4);
// Draw a square
ctx.fillRect(-50, -50, 100, 100);
</script>
🧠 How it works?
In this example, we translate the origin to the center of the canvas, rotate it by 45 degrees, and then draw a square centered at the new origin.
🎉 Conclusion
Canvas transformations open up a world of possibilities for creating dynamic and interactive graphics in web applications.
By understanding the syntax and methods for applying transformations, developers can unleash their creativity and build engaging visual experiences.
👨💻 Join our Community:
Author
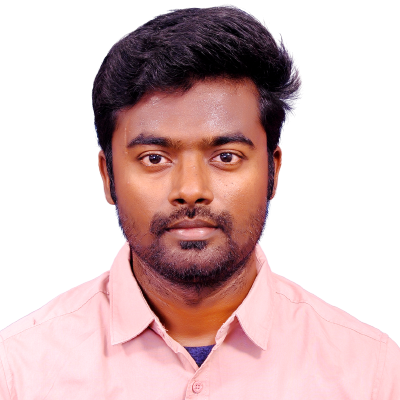
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Canvas Transform), please comment here. I will help you immediately.