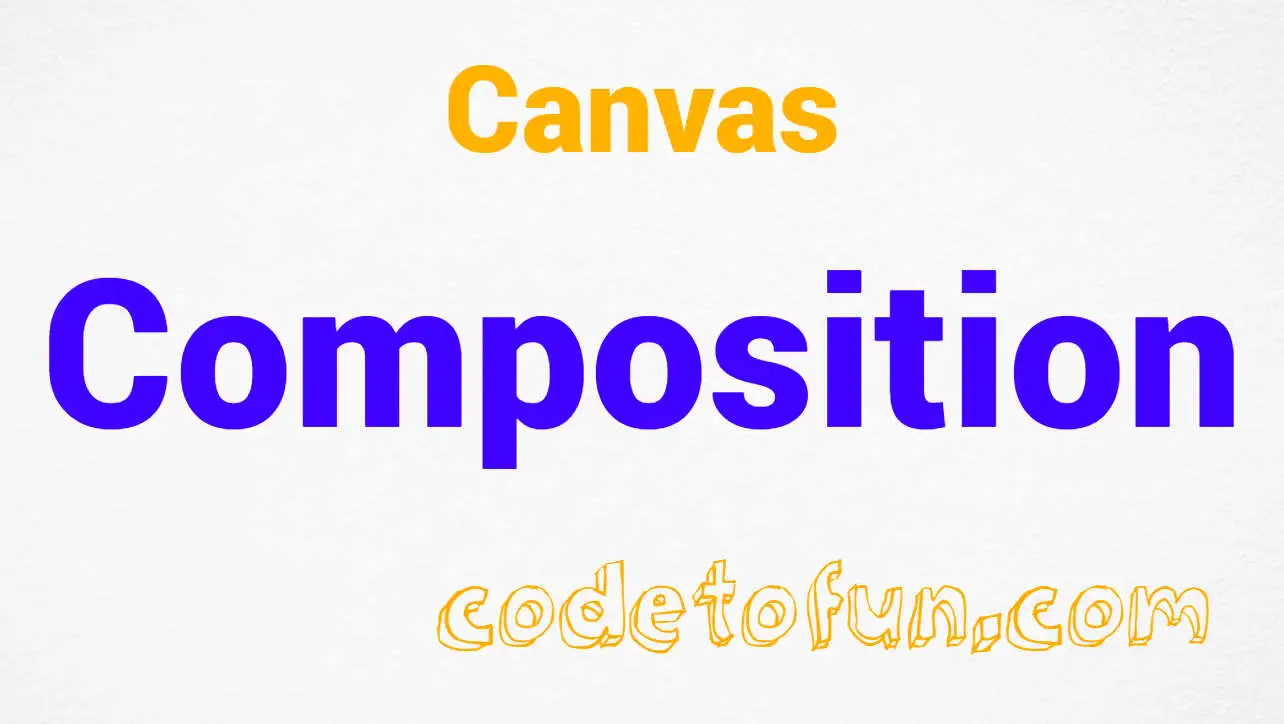
Canvas
- Canvas Introduction
- Canvas Draw Rectangles
- Canvas Draw Lines
- Canvas Draw Bezier
- Canvas Draw Quadratic
- Canvas Draw Arc
- Canvas Draw Paths
- Canvas Manipulating Images
- Canvas Linear Gradients
- Canvas Radial Gradients
- Canvas Text
- Canvas Pattern
- Canvas Shadow
- Canvas States
- Canvas Translate
- Canvas Rotate
- Canvas Scale
- Canvas Transform
- Canvas Composition
Canvas Shadow
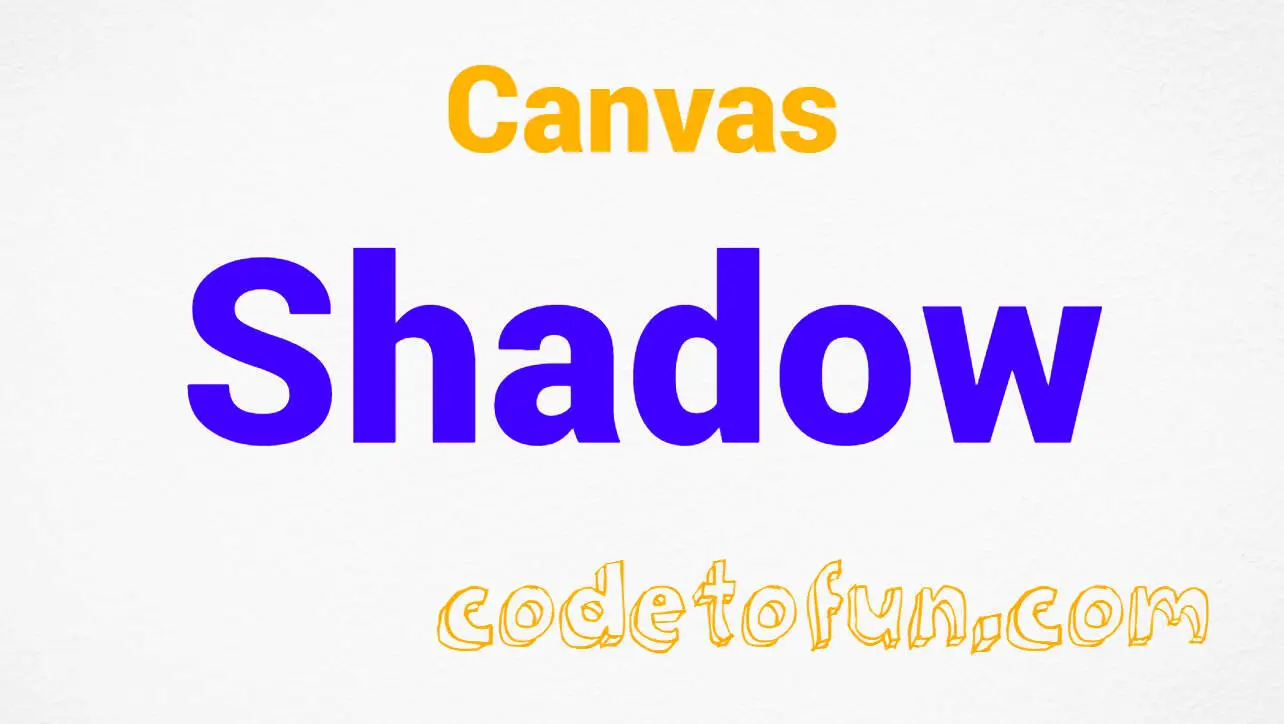
Photo Credit to CodeToFun
🙋 Introduction
The HTML5 <canvas> element allows for dynamic, scriptable rendering of 2D shapes and bitmap images. One of the powerful features of the canvas is the ability to create shadows, which can add depth and realism to your drawings.
In this guide, we will explore how to use canvas shadows, covering the syntax, attributes, and practical examples.
💡 Syntax
To create a shadow on the canvas, you need to use a few specific properties of the canvas's 2D rendering context. These properties are:
- shadowColor
- shadowBlur
- shadowOffsetX
- shadowOffsetY
Here’s how you typically set these properties:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
ctx.shadowColor = 'color';
ctx.shadowBlur = blurLevel;
ctx.shadowOffsetX = offsetX;
ctx.shadowOffsetY = offsetY;
🧰 Attributes
- shadowColor: Specifies the color of the shadow. It accepts any valid CSS color value, such as rgba(0, 0, 0, 0.5) for a semi-transparent black shadow.
- shadowBlur: Specifies the level of blur for the shadow. A higher value means more blur.
- shadowOffsetX: Specifies the horizontal distance of the shadow from the shape. Positive values move the shadow to the right, and negative values move it to the left.
- shadowOffsetY: Specifies the vertical distance of the shadow from the shape. Positive values move the shadow down, and negative values move it up.
📄 Example
Here’s an example of drawing a rectangle with a shadow:
<!DOCTYPE html>
<html>
<head>
<title>Canvas Shadow Example</title>
</head>
<body>
<canvas id="myCanvas" width="200" height="200"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Set shadow properties
ctx.shadowColor = 'rgba(0, 0, 0, 0.5)';
ctx.shadowBlur = 10;
ctx.shadowOffsetX = 5;
ctx.shadowOffsetY = 5;
// Draw a rectangle
ctx.fillStyle = 'blue';
ctx.fillRect(50, 50, 100, 100);
</script>
</body>
</html>
🧠 How it works?
In this example, we create a blue rectangle at (50, 50) with a width and height of 100 units. The shadow is semi-transparent black (rgba(0, 0, 0, 0.5)) with a blur level of 10, and it is offset by 5 units to the right and 5 units down.
🎉 Conclusion
Adding shadows to your canvas drawings can significantly enhance the visual appeal by introducing depth and dimension.
By mastering the use of shadowColor, shadowBlur, shadowOffsetX, and shadowOffsetY, you can create sophisticated and realistic graphics on your web pages. Experiment with these properties to see how they can transform your canvas projects!
👨💻 Join our Community:
Author
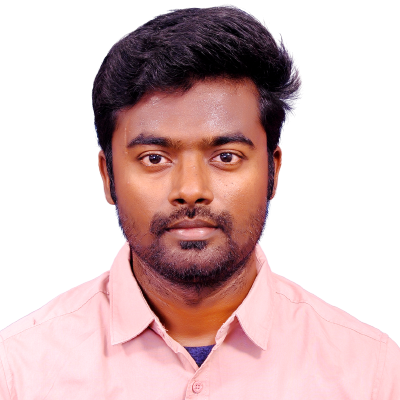
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Canvas Shadow), please comment here. I will help you immediately.