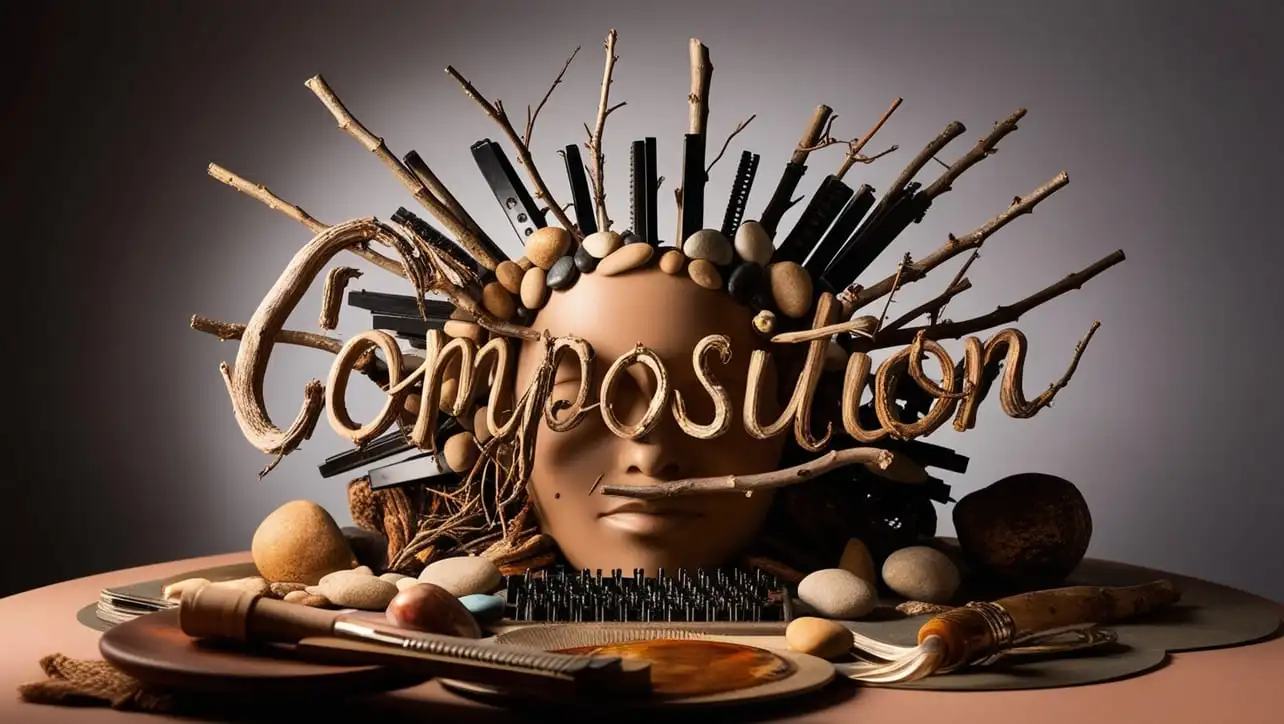
Canvas
- Canvas Introduction
- Canvas Draw Rectangles
- Canvas Draw Lines
- Canvas Draw Bezier
- Canvas Draw Quadratic
- Canvas Draw Arc
- Canvas Draw Paths
- Canvas Manipulating Images
- Canvas Linear Gradients
- Canvas Radial Gradients
- Canvas Text
- Canvas Pattern
- Canvas Shadow
- Canvas States
- Canvas Translate
- Canvas Rotate
- Canvas Scale
- Canvas Transform
- Canvas Composition
Canvas Manipulating Images
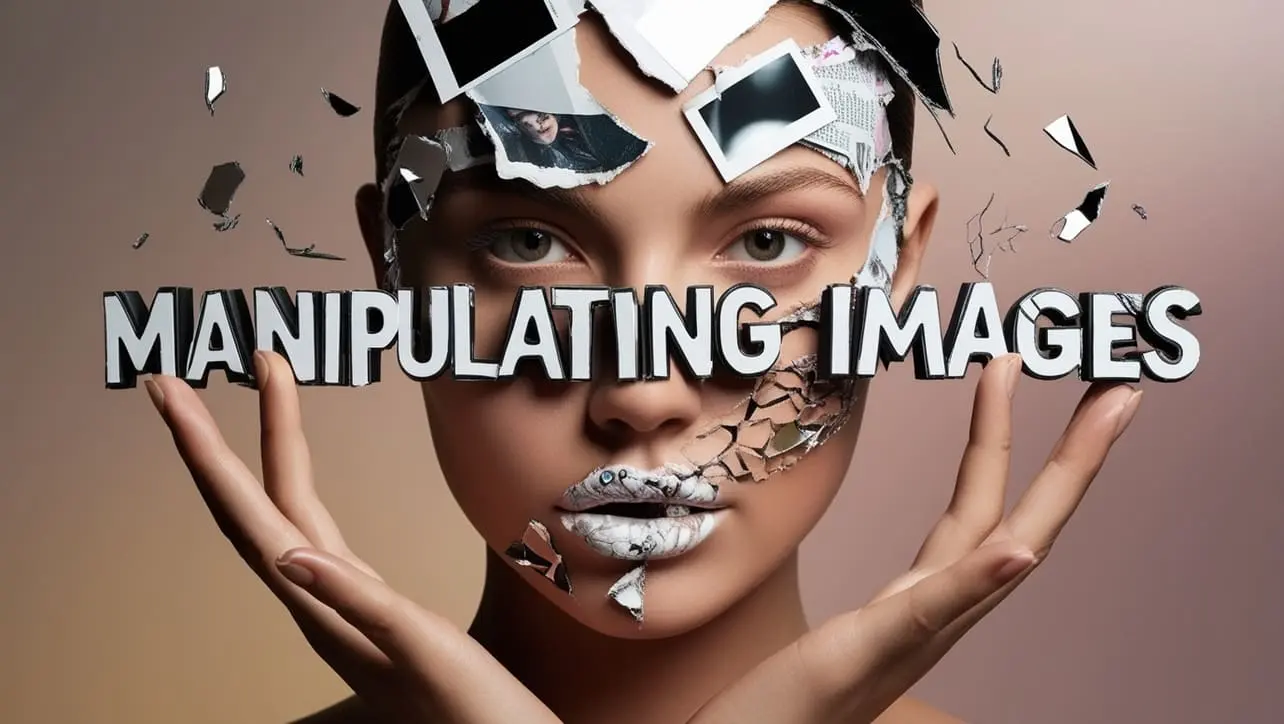
Photo Credit to CodeToFun
Introduction
Canvas, a powerful HTML5 element, provides a platform for dynamic rendering and manipulation of graphics, including images. With Canvas, developers can perform various operations on images, such as scaling, rotating, and applying filters, directly within the browser.
In this guide, we'll explore how to manipulate images on the Canvas element.
Syntax
To work with images on the Canvas, you first need to load the image onto the canvas using JavaScript. Here's a basic outline of the syntax:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
const img = new Image();
img.onload = function() {
ctx.drawImage(img, x, y, width, height);
}
img.src = 'image.jpg';
Operations
Scaling:
You can resize an image on the canvas using the drawImage() method. Adjust the width and height parameters to scale the image:
example.jsCopiedctx.drawImage(img, x, y, width, height);
Rotation:
To rotate an image on the canvas, use the rotate() method along with drawImage():
example.jsCopiedctx.translate(canvas.width / 2, canvas.height / 2); ctx.rotate(Math.PI / 180 * degrees); ctx.drawImage(img, -img.width / 2, -img.height / 2);
Filters:
Applying filters to images on the canvas involves manipulating pixel data. Here's an example of applying a grayscale filter:
example.jsCopiedconst imageData = ctx.getImageData(0, 0, canvas.width, canvas.height); const data = imageData.data; for (let i = 0; i < data.length; i += 4) { const avg = (data[i] + data[i + 1] + data[i + 2]) / 3; data[i] = avg; // Red data[i + 1] = avg; // Green data[i + 2] = avg; // Blue } ctx.putImageData(imageData, 0, 0);
Example
Let's put it all together with a simple example:
<canvas id="myCanvas" width="400" height="300"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
const img = new Image();
img.onload = function() {
ctx.drawImage(img, 0, 0, canvas.width, canvas.height);
// Apply grayscale filter
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);
const data = imageData.data;
for (let i = 0; i < data.length; i += 4) {
const avg = (data[i] + data[i + 1] + data[i + 2]) / 3;
data[i] = avg; // Red
data[i + 1] = avg; // Green
data[i + 2] = avg; // Blue
}
ctx.putImageData(imageData, 0, 0);
}
img.src = 'image.jpg';
</script>
Conclusion
Canvas provides a versatile platform for manipulating images directly within the browser, offering developers the ability to scale, rotate, and apply various effects to images dynamically
By understanding the syntax and operations available, you can create engaging visual experiences for your web applications.
Join our Community:
Author
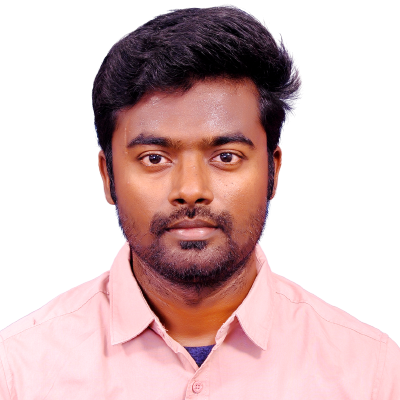
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Canvas Manipulating Images), please comment here. I will help you immediately.