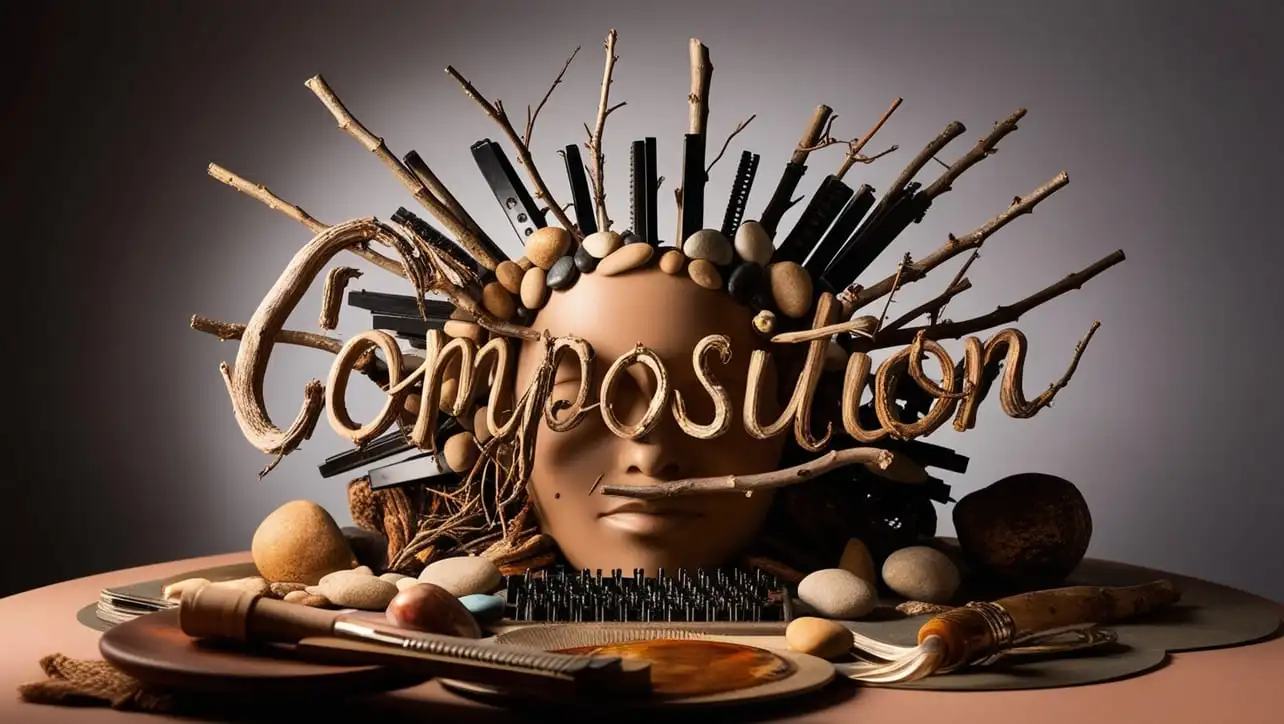
Canvas
- Canvas Introduction
- Canvas Draw Rectangles
- Canvas Draw Lines
- Canvas Draw Bezier
- Canvas Draw Quadratic
- Canvas Draw Arc
- Canvas Draw Paths
- Canvas Manipulating Images
- Canvas Linear Gradients
- Canvas Radial Gradients
- Canvas Text
- Canvas Pattern
- Canvas Shadow
- Canvas States
- Canvas Translate
- Canvas Rotate
- Canvas Scale
- Canvas Transform
- Canvas Composition
Canvas Linear Gradients
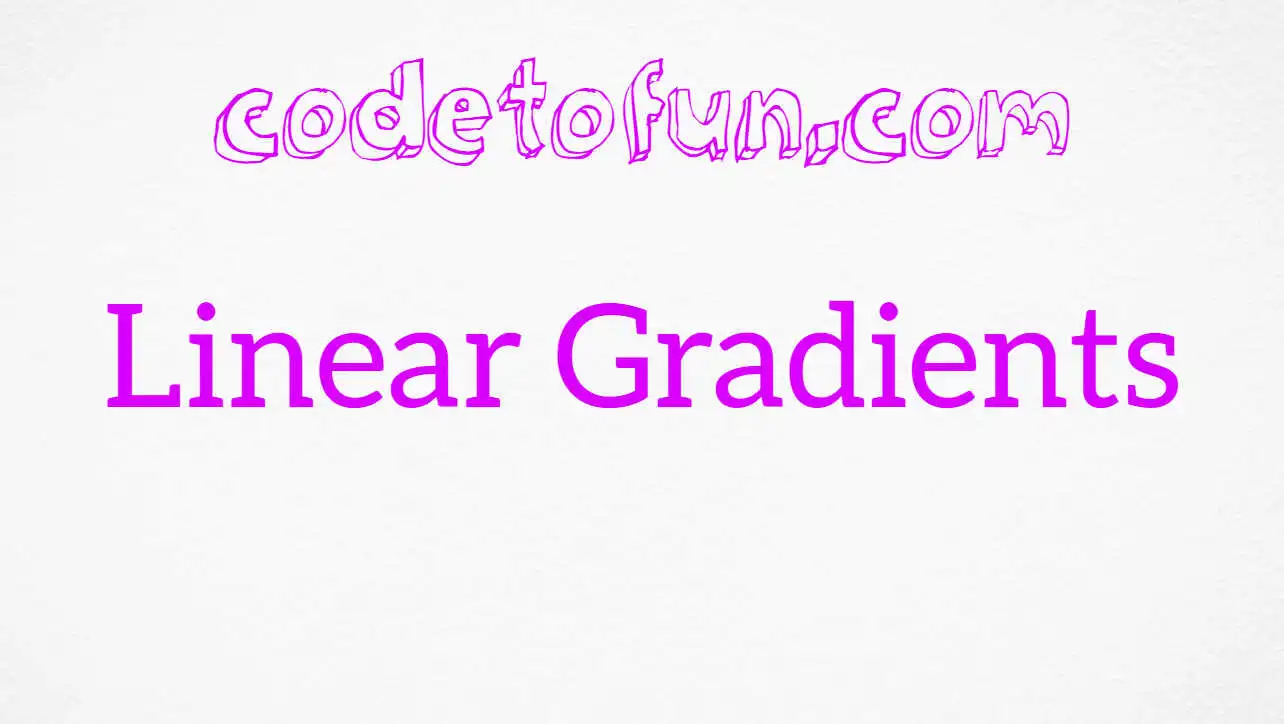
Photo Credit to CodeToFun
🙋 Introduction
Linear gradients are powerful tools in web development for creating smooth transitions between colors. When it comes to HTML5 Canvas, linear gradients offer a flexible way to fill shapes and backgrounds with color transitions.
In this guide, we'll explore how to use linear gradients in the HTML5 Canvas element.
💡 Syntax
To create a linear gradient in Canvas, you'll need to follow these steps:
- Initialize a gradient object using the createLinearGradient() method.
- Define the starting and ending points of the gradient.
- Add color stops to specify the colors and their positions along the gradient.
Here's the basic syntax:
const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
// Create gradient
const gradient = context.createLinearGradient(x0, y0, x1, y1);
// Add colors
gradient.addColorStop(stopPosition1, 'color1');
gradient.addColorStop(stopPosition2, 'color2');
// Use gradient
context.fillStyle = gradient;
context.fillRect(x, y, width, height);
🧰 Attribute Values
- x0, y0: Coordinates of the starting point of the gradient.
- x1, y1: Coordinates of the ending point of the gradient.
- stopPosition: Position along the gradient axis where the color stop is placed (0 to 1).
- color: Color value specified in CSS format (e.g., 'red', '#00ff00', 'rgba(255, 0, 0, 0.5)').
📄 Example
Let's create a simple linear gradient in Canvas:
const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
// Create gradient
const gradient = context.createLinearGradient(0, 0, 200, 0);
// Add colors
gradient.addColorStop(0, 'red');
gradient.addColorStop(1, 'blue');
// Draw rectangle with gradient fill
context.fillStyle = gradient;
context.fillRect(20, 20, 150, 100);
🧠 How it works?
In this example, we've created a linear gradient that transitions from red to blue horizontally and filled a rectangle with it.
🎉 Conclusion
Canvas linear gradients offer a versatile way to add depth and visual interest to your web applications and graphics.
By mastering the syntax and attributes involved, you can create stunning color transitions and effects to enhance user experiences.
👨💻 Join our Community:
Author
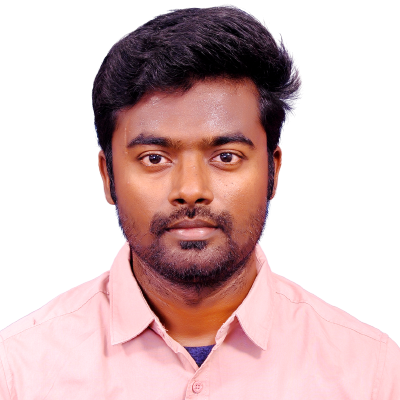
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Canvas Linear Gradients), please comment here. I will help you immediately.