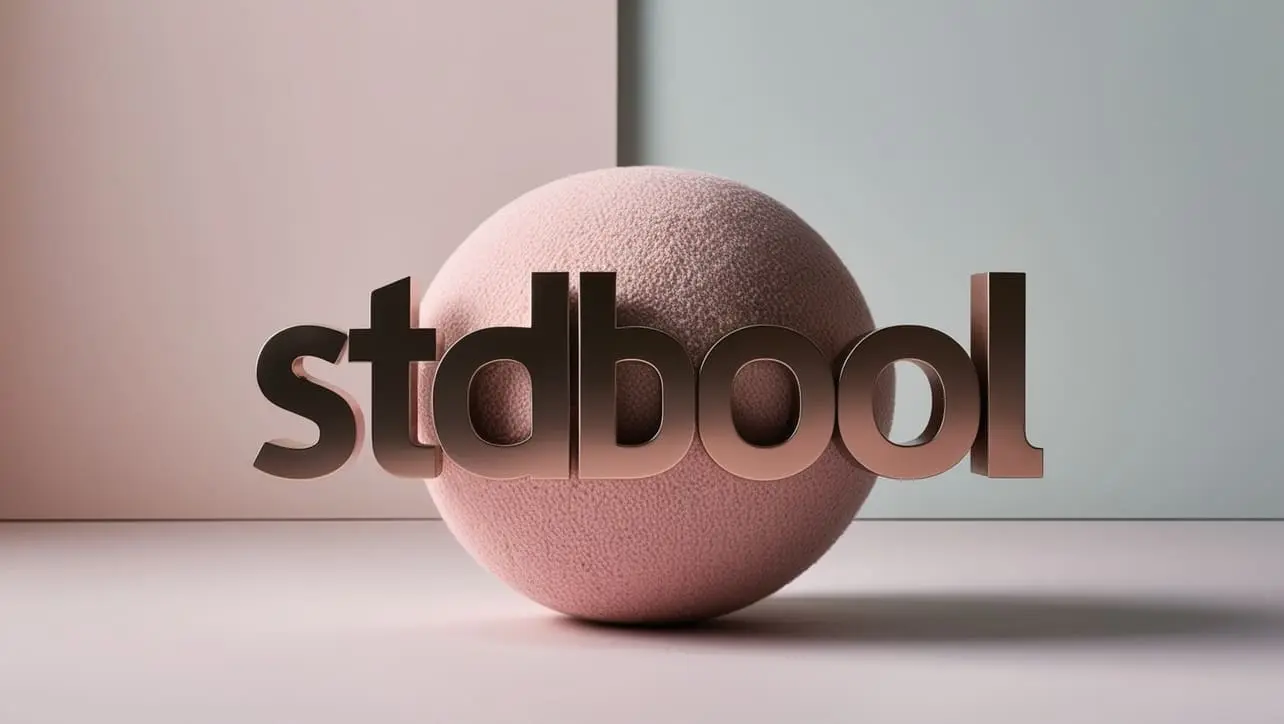
C if Statement
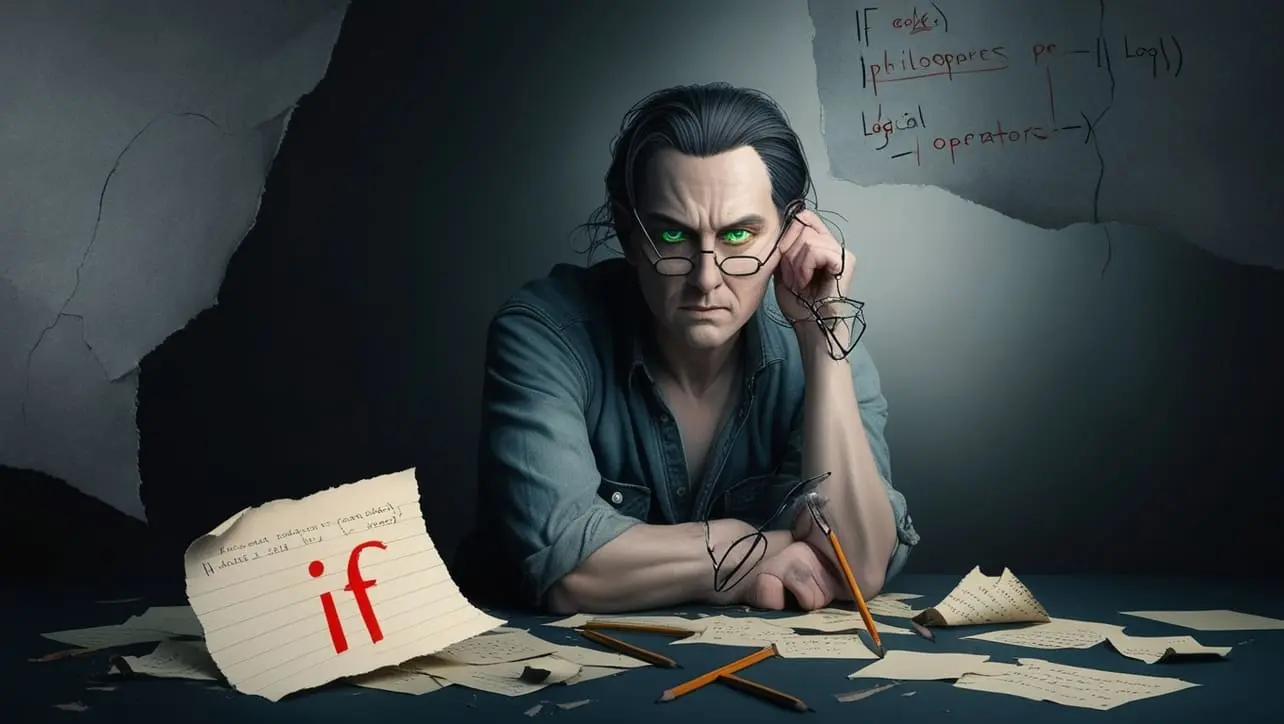
Photo Credit to CodeToFun
🙋 Introduction
The if
statement is one of the most fundamental control structures in C programming. It allows you to execute certain parts of your code based on whether a condition is true or false.
This guide will cover the basics of the if
statement, its syntax, and practical examples.
🤔 What is the if Statement?
In C programming, the if
statement is used to make decisions in your code. It evaluates a condition and executes a block of code if the condition is true. If the condition is false, the block of code is skipped.
🔑 Key Features
- Conditional Execution: Executes a block of code only if a specified condition is true.
- Flexible Control Flow: Can be combined with else and else if statements to create complex decision-making structures.
- Logical Operations: Supports the use of logical operators to form complex conditions.
💡 Syntax
The syntax for the if
statement is straightforward:
if (condition) {
// Code to execute if the condition is true
}
Simple if Statement
The simplest form of the if
statement evaluates a single condition:
#include <stdio.h>
int main() {
int num = 10;
if (num > 0) {
printf("The number is positive.\n");
}
return 0;
}
In this example, the message "The number is positive." will be printed because the condition num > 0 is true.
else and else if Clauses
The if
statement can be extended with else and else if clauses to handle additional conditions and alternatives.
Using else
The else clause executes a block of code if the if condition is false:
#include <stdio.h>
int main() {
int num = -5;
if (num > 0) {
printf("The number is positive.\n");
} else {
printf("The number is not positive.\n");
}
return 0;
}
In this example, "The number is not positive." will be printed because the condition num > 0 is false.
Using else if
The else if clause allows you to check multiple conditions:
#include <stdio.h>
int main() {
int num = 0;
if (num > 0) {
printf("The number is positive.\n");
} else if (num < 0) {
printf("The number is negative.\n");
} else {
printf("The number is zero.\n");
}
return 0;
}
In this example, "The number is zero." will be printed because both num > 0 and num < 0 are false.
Nested if Statements
You can nest if
statements within each other to handle more complex decision-making:
#include <stdio.h>
int main() {
int num = 15;
if (num > 0) {
if (num % 2 == 0) {
printf("The number is positive and even.\n");
} else {
printf("The number is positive and odd.\n");
}
} else {
printf("The number is not positive.\n");
}
return 0;
}
In this example, "The number is positive and odd." will be printed because num is greater than 0 and not divisible by 2.
Logical Operators in if Statements
You can use logical operators to combine multiple conditions in an if
statement:
- && (logical AND): True if both operands are true.
- || (logical OR): True if at least one operand is true.
- ! (logical NOT): True if the operand is false.
Example with Logical Operators
#include <stdio.h>
int main() {
int num = 20;
if (num > 0 && num % 2 == 0) {
printf("The number is positive and even.\n");
}
return 0;
}
In this example, the message will be printed because both conditions num > 0 and num % 2 == 0 are true.
📚 Common Pitfalls and Best Practices
Braces {} Usage:
Always use braces {} to define the scope of if, else if, and else blocks, even if there is only one statement. This improves readability and prevents errors when modifying code.
Avoid Deep Nesting:
Try to avoid deeply nested
if
statements as they can make your code harder to read and maintain. Consider using logical operators or refactoring your code to simplify complex conditions.Consistent Indentation:
Maintain consistent indentation to enhance the readability of your code. Proper indentation helps in understanding the structure and flow of your
if
statements.
🎉 Conclusion
The if
statement is a crucial part of C programming that enables you to control the flow of your program based on conditions.
By understanding its syntax and best practices, you can write more efficient and readable code.
👨💻 Join our Community:
Author
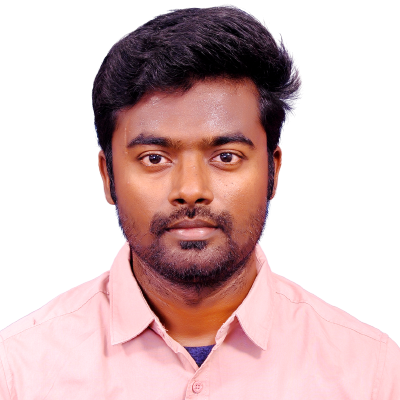
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C if Statement), please comment here. I will help you immediately.