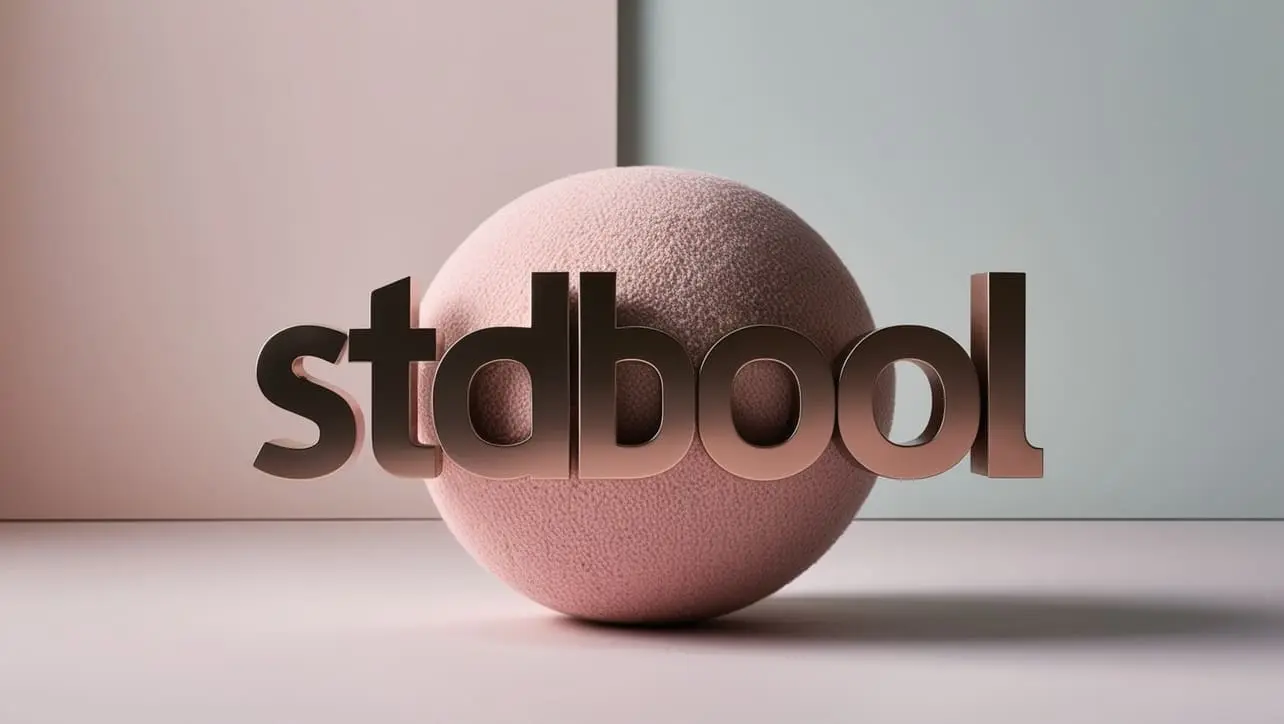
C else-if Statement
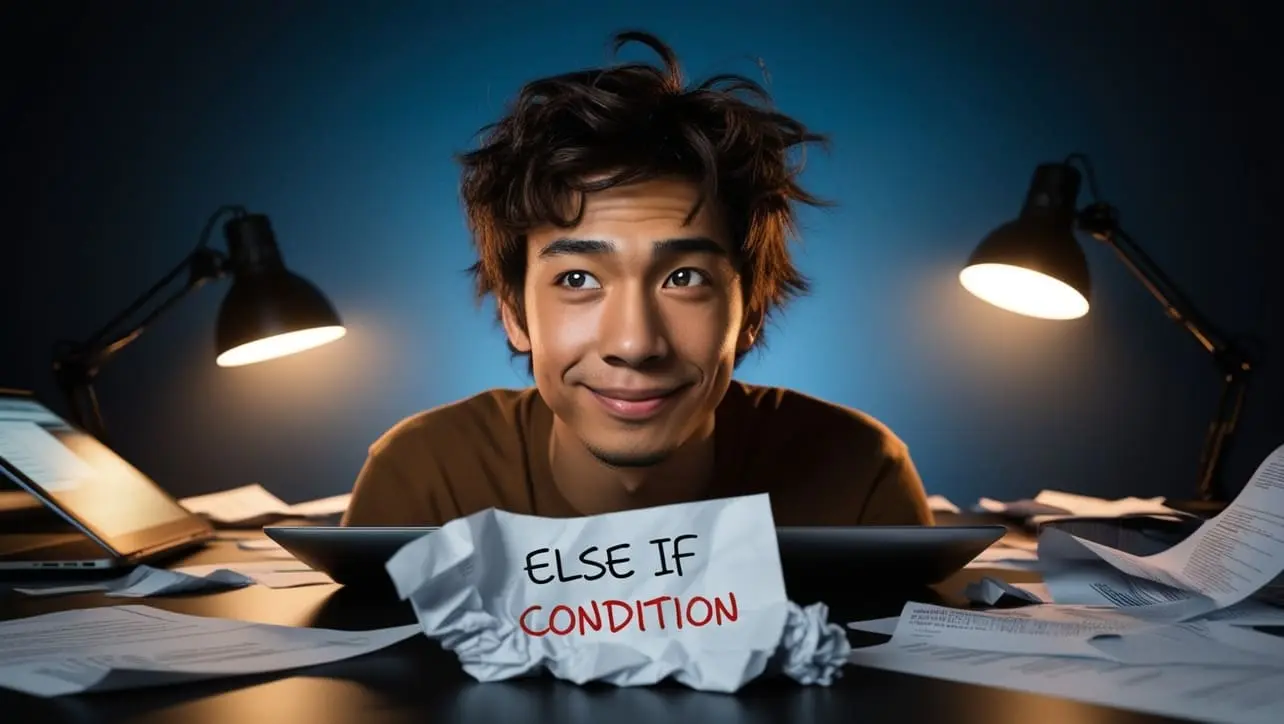
Photo Credit to CodeToFun
🙋 Introduction
The else if
statement in C is a conditional statement used to check multiple conditions in sequence. It allows you to create complex decision-making structures in your programs, providing flexibility in handling different scenarios.
🤔 What is the else if Statement?
In C programming, the else if
statement is used to evaluate multiple conditions. It extends the basic if statement by adding additional conditions to be tested if the previous if or else if condition evaluates to false
.
🔑 Key Features
- Multiple Conditions: Allows checking multiple conditions in a sequential manner.
- Controlled Flow: Directs program flow based on condition evaluations.
- Improved Readability: Makes complex decision structures more readable and manageable.
💡 Syntax
The syntax for the else if
statement follows this structure:
Explanation:
- if Statement: Evaluates the first condition.
- else if Statements: Evaluates subsequent conditions if previous ones are false.
- else Statement: Executes code if all conditions are false.
Example
Let's consider an example where we determine the grade of a student based on their score:
#include <stdio.h>
int main() {
int score = 85;
if (score >= 90) {
printf("Grade: A\n");
} else if (score >= 80) {
printf("Grade: B\n");
} else if (score >= 70) {
printf("Grade: C\n");
} else if (score >= 60) {
printf("Grade: D\n");
} else {
printf("Grade: F\n");
}
return 0;
}
In this example, the program evaluates the score and assigns a grade based on the following conditions:
- score >= 90: Grade A
- score >= 80: Grade B
- score >= 70: Grade C
- score >= 60: Grade D
- Otherwise: Grade F
Since the score is 85, the output will be:
Grade: B
You can nest else if
statements to handle more complex conditions. Here's an example:
#include <stdio.h>
int main() {
int num = 15;
if (num > 0) {
if (num % 2 == 0) {
printf("The number is positive and even.\n");
} else {
printf("The number is positive and odd.\n");
}
} else if (num < 0) {
printf("The number is negative.\n");
} else {
printf("The number is zero.\n");
}
return 0;
}
In this example, the nested else if
statements check if a number is positive, negative, or zero, and further check if a positive number is even or odd. The output for num = 15 will be:
The number is positive and odd
🏆 Best Practices
Keep Conditions Simple:
Ensure each condition in your
else if
statements is simple and easy to understand. Complex conditions can make the code difficult to read and debug.Avoid Redundant Checks:
Avoid checking conditions that are already covered by previous if or
else if
statements. This improves efficiency and readability.Use Braces for Clarity:
Always use braces {} for the code blocks in if, else if, and else statements, even if they contain only one line of code. This prevents errors and enhances readability.
Consider Logical Order:
Arrange else if conditions logically, starting with the most specific and ending with the most general. This ensures that the most critical conditions are checked first.
🎉 Conclusion
The else if
statement in C is an essential tool for handling multiple conditions in your programs.
By understanding its syntax and best practices, you can create efficient and readable decision-making structures that enhance the functionality of your code.
👨💻 Join our Community:
Author
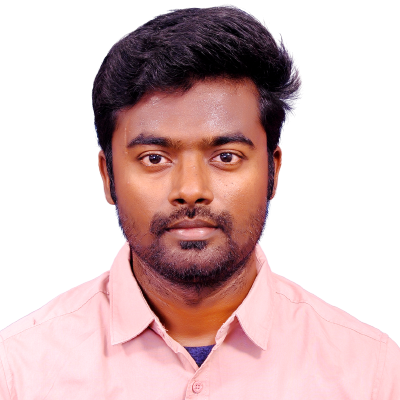
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C else-if Statement), please comment here. I will help you immediately.