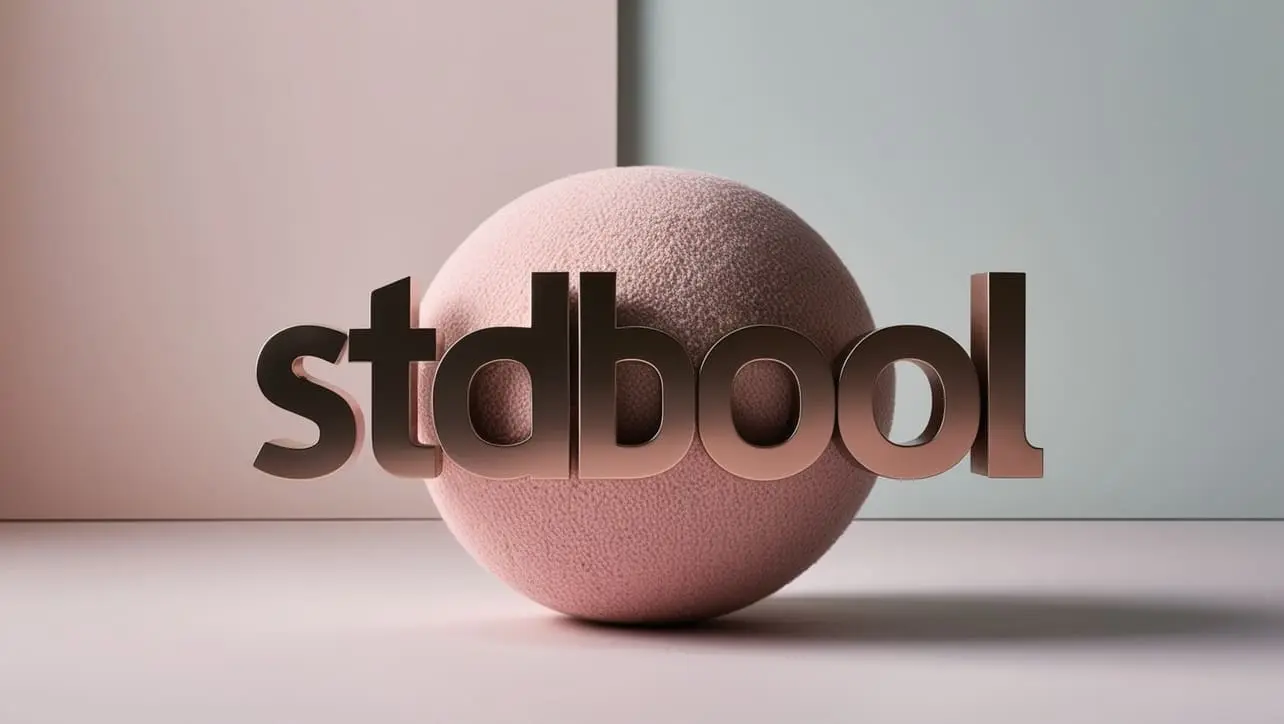
C continue Statement
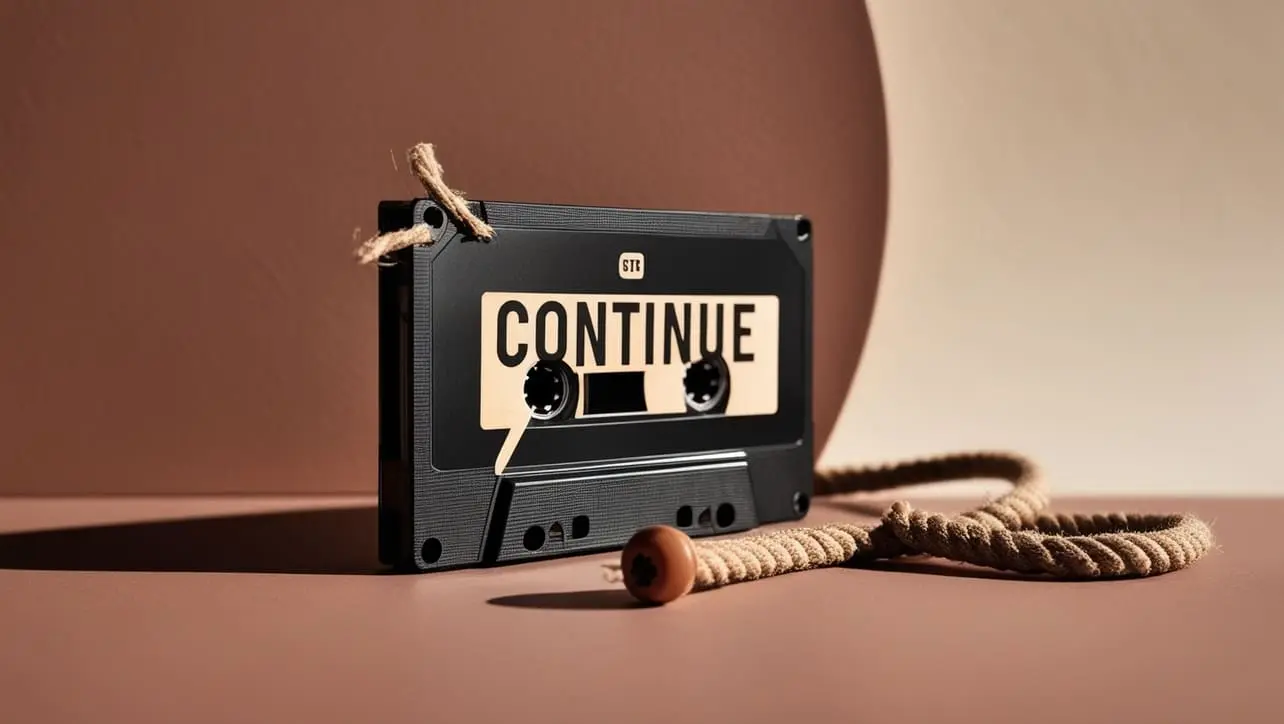
Photo Credit to CodeToFun
🙋 Introduction
In C programming, the continue
statement is a control statement that skips the current iteration of a loop and proceeds to the next iteration.
This guide explores the syntax and usage of the continue
statement, along with practical examples.
🤔 What is the continue Statement?
The continue
statement allows you to bypass the remaining code within a loop's body for the current iteration and proceed to the next iteration of the loop. It is particularly useful for skipping specific conditions or processing certain elements within a loop.
🔑 Key Features of the continue Statement
- Loop Control: Skips the current iteration of a loop.
- Conditional Skipping: Allows conditional skipping based on specific criteria.
- Control Flow: Alters the normal sequence of loop execution.
💡 Syntax
The syntax for the continue
statement is simple:
continue;
Usage in Loops
The continue
statement is typically used within for, while, and do-while loops to skip iterations based on certain conditions.
Example: Using continue in a for Loop
#include <stdio.h>
int main() {
for (int i = 0; i < 5; i++) {
if (i == 2) {
continue; // Skip iteration when i equals 2
}
printf("%d\n", i);
}
return 0;
}
💻 Output
In this example, the loop will print all values of i except 2:
0 1 3 4
Example: Using continue in a while Loop
#include <stdio.h>
int main() {
int i = 0;
while (i < 5) {
i++;
if (i == 2 || i == 4) {
continue; // Skip iterations when i equals 2 or 4
}
printf("%d\n", i);
}
return 0;
}
💻 Output
This example skips printing 2 and 4:
1 3 5
Common Pitfalls and Best Practices
Proper Incrementing:
Ensure that loop control variables are properly incremented within loops to prevent infinite loops when using
continue
.example.cCopied#include <stdio.h> int main() { int i = 0; while (i < 5) { if (i == 2) { i++; // Increment i to prevent infinite loop continue; } printf("%d\n", i); i++; } return 0; }
Clear Logic:
Maintain clear and understandable logic when using
continue
statements to avoid confusion and improve code readability.
🎉 Conclusion
The continue
statement is a valuable tool in C programming for controlling loop execution flow. By utilizing continue
, you can efficiently skip specific iterations of loops based on conditions, streamlining your code and improving its readability.
👨💻 Join our Community:
Author
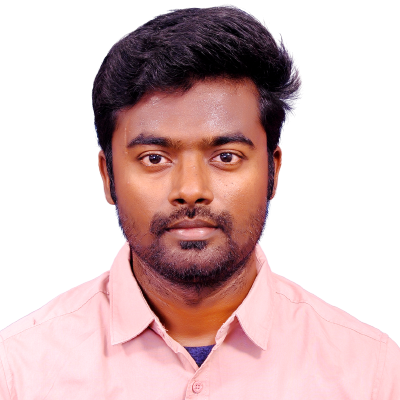
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C continue Statement), please comment here. I will help you immediately.