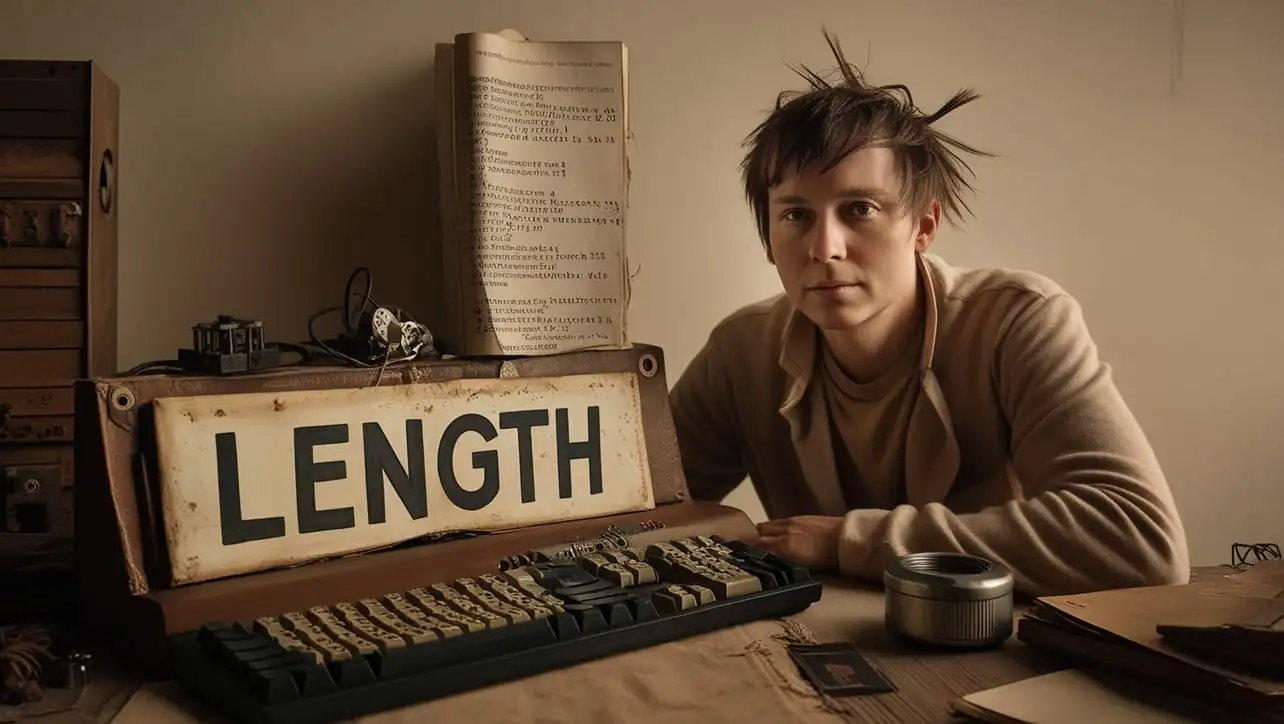
JS Basic
JavaScript File Handling
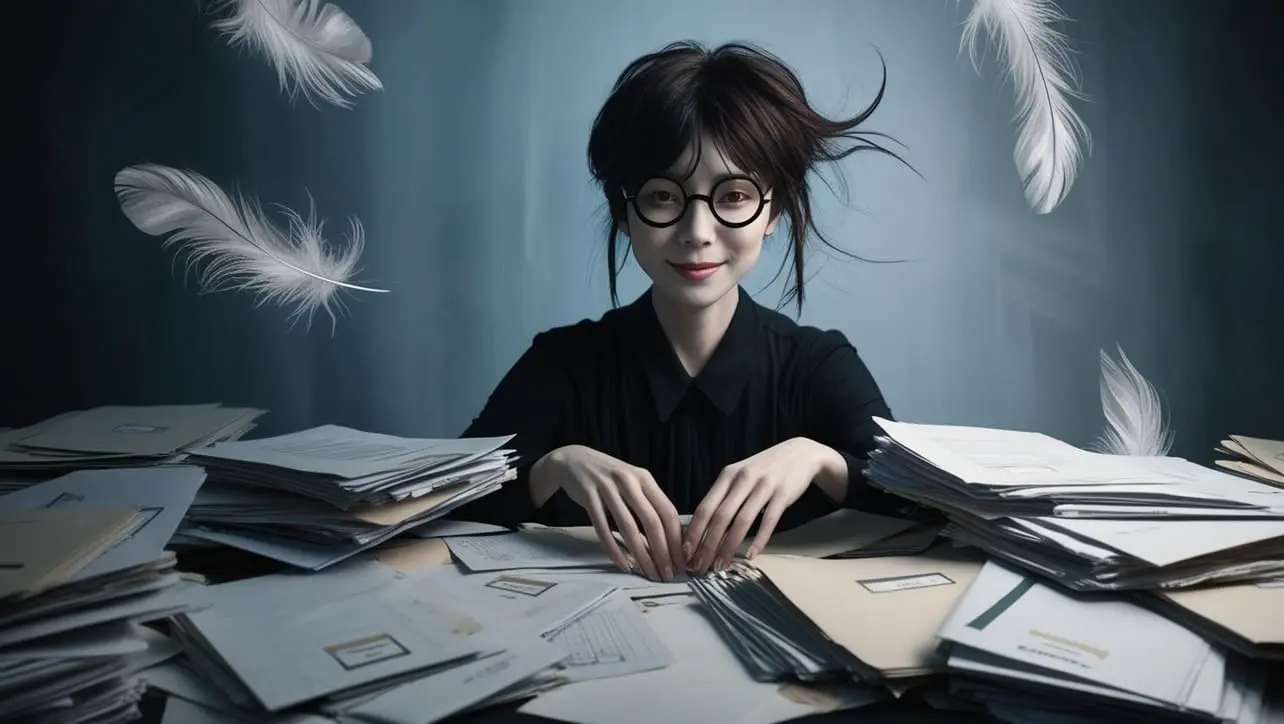
Photo Credit to CodeToFun
Introduction
JavaScript file handling involves working with files like text files, images, and other types of binary data directly in the browser or through server-side platforms like Node.js.
Using APIs like the File API and FileReader, we can read, write, and process files within a web application without needing server interactions.
What is File Handling in JavaScript?
File handling in JavaScript enables users to work with files programmatically, such as reading file contents, modifying data, and even creating new files (server-side). This can be done both on the client side using browser APIs and on the server side using Node.js.
Key Features of File Handling
- File Access: Directly access files selected by the user.
- Asynchronous Operations: File operations in JavaScript are often asynchronous, preventing the UI from freezing.
- Binary and Text Processing: Handle both text and binary files for various use cases.
- Integration with Form Uploads: Easily integrate file input and upload forms with JavaScript for file validation or preview.
Client-Side vs Server-Side File Handling
- Client-Side (Browser): On the client side, JavaScript can handle files using the File API and FileReader, allowing the user to select and read files without uploading them to the server.
- Server-Side (Node.js): On the server side, file handling is done using the fs (File System) module in Node.js. This allows reading and writing files on the server, creating file streams, and more.
File Handling Using the File API
The File API allows web applications to read files locally using an input element. You can capture the selected file and process it without submitting the form.
<input type="file" id="fileInput">
JavaScript code to capture the file:
const fileInput = document.getElementById('fileInput');
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0]; // Get the first file
console.log('Selected file:', file.name);
});
Reading Files Using FileReader
The FileReader object allows you to read the content of files asynchronously. It can read files as text, data URLs (for images), and binary strings.
const fileReader = new FileReader();
fileReader.onload = function(event) {
console.log('File content:', event.target.result);
};
fileReader.readAsText(file); // Read file as text
You can also read files as binary or base64 data for images:
fileReader.readAsDataURL(file); // Read file as a base64 data URL (useful for images)
Working with Binary Data
For handling binary files (e.g., images, videos, etc.), you can use ArrayBuffer and Blob objects in combination with FileReader to process the data efficiently.
fileReader.onload = function(event) {
const arrayBuffer = event.target.result;
console.log('Binary data:', new Uint8Array(arrayBuffer));
};
fileReader.readAsArrayBuffer(file); // Read binary data
Handling Errors
When dealing with file operations, errors can occur. Itβs important to implement error handling for a smoother user experience:
fileReader.onerror = function(event) {
console.error('File reading error:', event.target.error);
};
You can also check for unsupported file types or file size limits during file selection.
if (file.size > 1000000) { // Limit file size to 1MB
console.error('File too large');
}
Security
File handling in JavaScript comes with several security concerns, especially on the client side. Here are some critical security aspects to keep in mind:
File Access Limitations:
JavaScript cannot access files directly on the user's file system without explicit user permission. It only works with files selected through an <input> element or a drag-and-drop interface.
File Type Validation:
Always validate the file type on both the client and server sides to prevent malicious files from being uploaded. This helps avoid potential security vulnerabilities like executing harmful scripts (cross-site scripting or XSS).
JavaScriptCopiedif (!file.type.startsWith('text/')) { console.error('Invalid file type'); }
File Size Validation:
Large files can cause performance issues or crashes. Make sure to enforce file size limits for both client-side file selection and server uploads.
Data Handling:
Sensitive data should never be read from or written to files in an unsecured environment. Always encrypt sensitive information and follow data protection guidelines.
Avoid File Path Disclosure:
Ensure that file path information is not disclosed to users, as it can provide insight into your server's structure and increase security risks.
Use of Blob URLs:
If you're creating object URLs with Blob objects, always revoke them once they're no longer needed to prevent memory leaks:
JavaScriptCopiedconst objectURL = URL.createObjectURL(file); // Do something with objectURL URL.revokeObjectURL(objectURL); // Revoke when done
Common Pitfalls
- File Size: Be cautious of large files that may slow down file reading and processing, especially in browsers.
- File Access: JavaScript cannot access the file system directly on the client-side (due to security concerns). File handling on the browser is limited to files selected by the user.
- Browser Compatibility: Not all browsers fully support the File API. Check for compatibility and provide polyfills if necessary.
Example
Hereβs a complete example showing how to select a file, read its contents, and display it:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript File Handling Example</title>
</head>
<body>
<h1>File Handling Example</h1>
<input type="file" id="fileInput">
<pre id="fileContent"></pre>
<script>
const fileInput = document.getElementById('fileInput');
const fileContent = document.getElementById('fileContent');
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0]; // Get the selected file
const fileReader = new FileReader();
fileReader.onload = function(event) {
fileContent.textContent = event.target.result; // Display file content
};
fileReader.onerror = function(event) {
console.error('Error reading file:', event.target.error);
};
fileReader.readAsText(file); // Read the file as text
});
</script>
</body>
</html>
Conclusion
JavaScript file handling enables powerful file manipulation capabilities both in the browser and on the server. By leveraging the File API and FileReader, you can create dynamic, user-friendly file handling features in your web applications. Understanding how to manage files safely and efficiently can enhance user experience, especially when dealing with large or complex files. Always prioritize security, especially when dealing with sensitive data or large file uploads.
π¨βπ» Join our Community:
Author
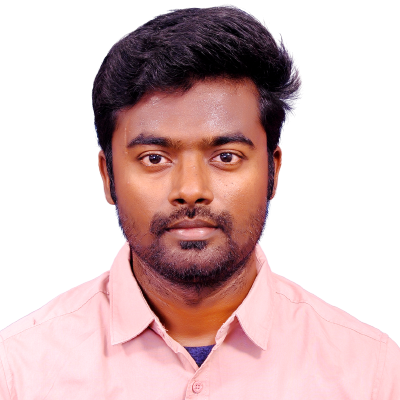
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee