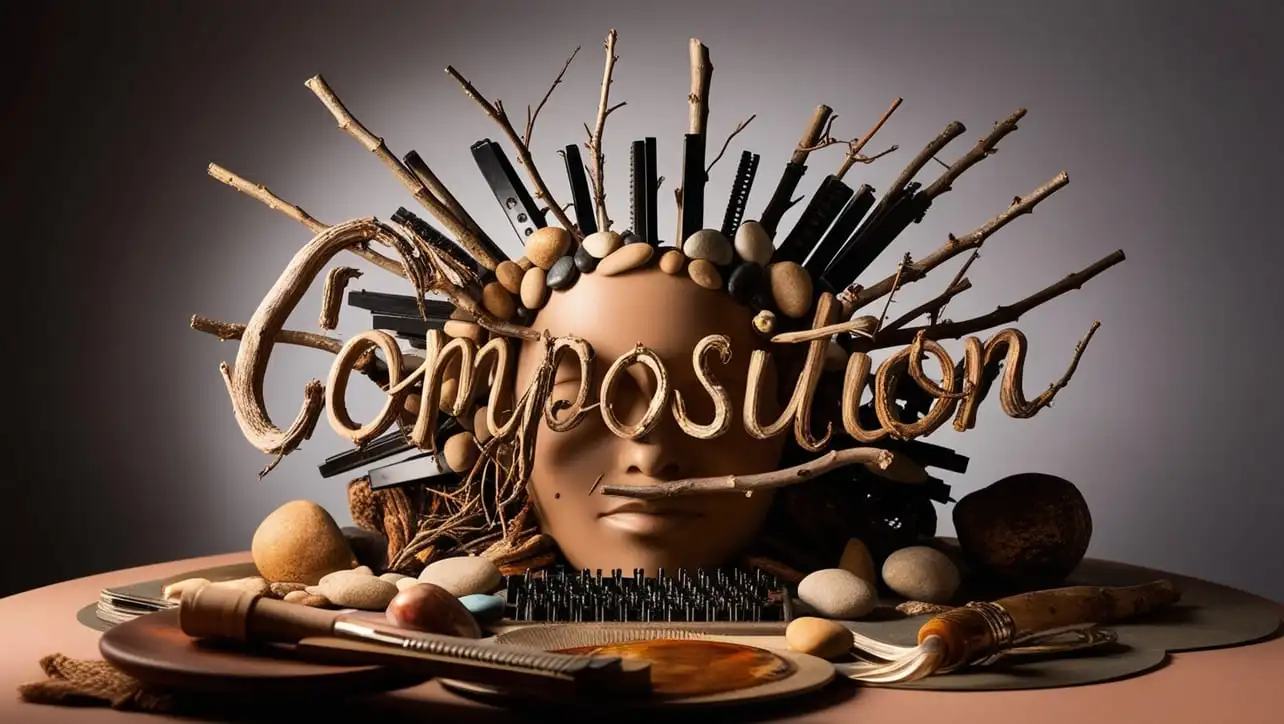
Canvas
- Canvas Introduction
- Canvas Draw Rectangles
- Canvas Draw Lines
- Canvas Draw Bezier
- Canvas Draw Quadratic
- Canvas Draw Arc
- Canvas Draw Paths
- Canvas Manipulating Images
- Canvas Linear Gradients
- Canvas Radial Gradients
- Canvas Text
- Canvas Pattern
- Canvas Shadow
- Canvas States
- Canvas Translate
- Canvas Rotate
- Canvas Scale
- Canvas Transform
- Canvas Composition
Canvas Text
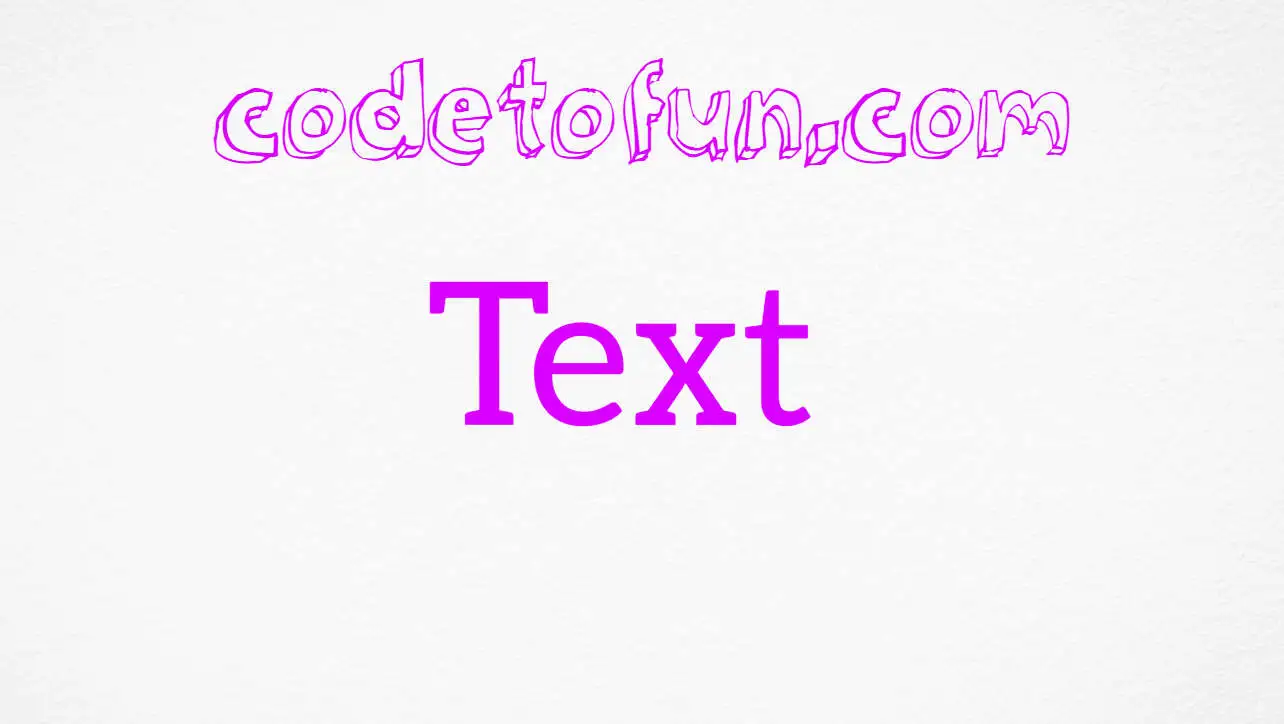
Photo Credit to CodeToFun
π Introduction
The HTML5 <canvas> element provides an exciting way to draw graphics using JavaScript. Among its many capabilities, rendering text is a crucial feature for creating dynamic and interactive web graphics. This guide will cover how to use the canvas API to draw and style text.
π‘ Syntax
To draw text on a canvas, you use the fillText() or strokeText() methods of the canvas 2D context. The basic syntax for these methods is as follows:
context.fillText(text, x, y);
context.strokeText(text, x, y);
π§° Attributes
- text: The string of text you want to render.
- x: The x-coordinate where the text will start.
- y: The y-coordinate where the text will start.
- font (optional): Specifies the font style for the text (e.g., context.font = "20px Arial";).
- textAlign (optional): Specifies the text alignment (e.g., context.textAlign = "center";).
- textBaseline (optional): Specifies the baseline alignment (e.g., context.textBaseline = "top";).
- fillStyle and strokeStyle (optional): Specifies the color of the filled or stroked text.
π Example
Hereβs an example demonstrating how to draw and style text on a canvas:
<canvas id="myCanvas" width="500" height="200"></canvas>
<script>
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
// Set font properties
context.font = "30px Arial";
context.textAlign = "center";
context.textBaseline = "middle";
context.fillStyle = "blue";
context.strokeStyle = "black";
// Draw filled text
context.fillText("Hello, Canvas!", canvas.width / 2, canvas.height / 2);
// Draw stroked text
context.strokeText("Hello, Canvas!", canvas.width / 2, canvas.height / 2 + 50);
</script>
π§ How it works?
In this example, we create a canvas element and use JavaScript to draw text with specific styling. The text "Hello, Canvas!" is drawn twice, once filled in blue and once outlined in black, both centered in the canvas.
π Conclusion
Using the canvas API to render text opens up a wide range of possibilities for web developers. Whether you're creating simple labels or complex graphical text effects, the canvas provides the tools you need.
Understanding the syntax and attributes for drawing text will help you make the most of this powerful feature in your web applications.
π¨βπ» Join our Community:
Author
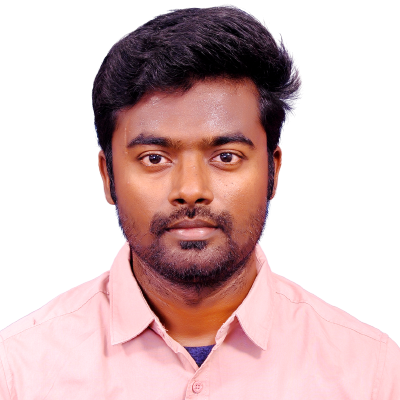
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Canvas Text), please comment here. I will help you immediately.