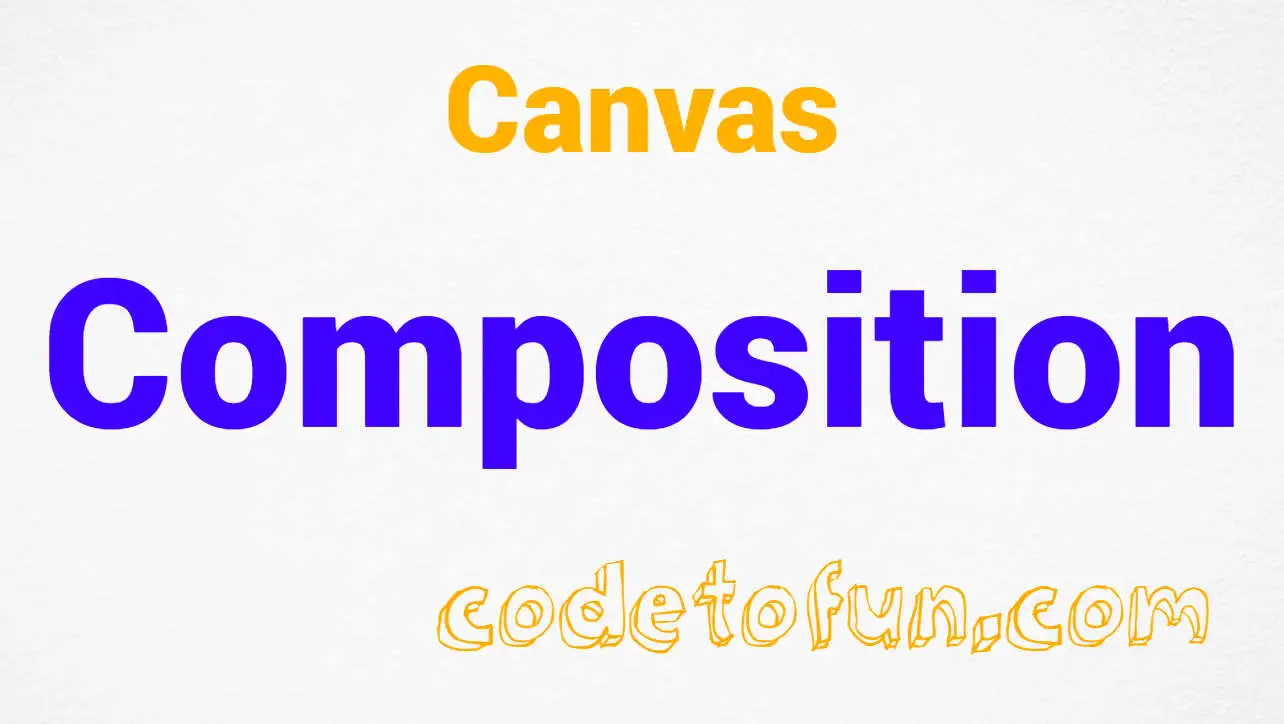
Canvas
- Canvas Introduction
- Canvas Draw Rectangles
- Canvas Draw Lines
- Canvas Draw Bezier
- Canvas Draw Quadratic
- Canvas Draw Arc
- Canvas Draw Paths
- Canvas Manipulating Images
- Canvas Linear Gradients
- Canvas Radial Gradients
- Canvas Text
- Canvas Pattern
- Canvas Shadow
- Canvas States
- Canvas Translate
- Canvas Rotate
- Canvas Scale
- Canvas Transform
- Canvas Composition
Canvas Draw Rectangles
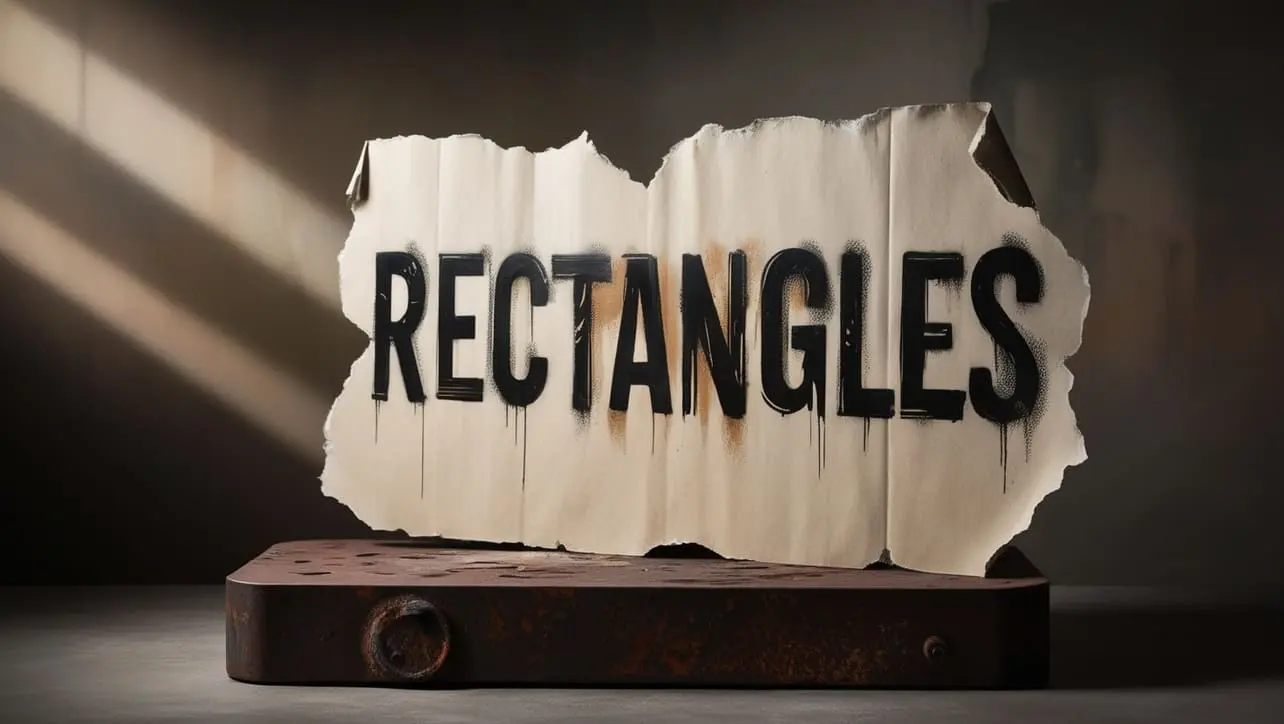
Photo Credit to CodeToFun
🙋 Introduction
Canvas is a powerful HTML element that allows dynamic, scriptable rendering of 2D shapes and bitmap images. Among its many features, the ability to draw rectangles is fundamental for creating complex graphics and visualizations directly within the browser.
In this guide, we'll explore how to draw rectangles on the HTML canvas element using JavaScript.
💡 Syntax
To draw a rectangle on the canvas, you'll use the fillRect() or strokeRect() method. Here's the basic syntax for both:
// Using fillRect() to draw a filled rectangle
context.fillRect(x, y, width, height);
// Using strokeRect() to draw a stroked (outlined) rectangle
context.strokeRect(x, y, width, height);
🧰 Parameters
- x: The x-coordinate of the top-left corner of the rectangle.
- y: The y-coordinate of the top-left corner of the rectangle.
- width: The width of the rectangle.
- height: The height of the rectangle.
📄 Example
Let's create a simple HTML canvas with JavaScript to draw a rectangle:
<canvas id="myCanvas" width="200" height="200"></canvas>
<script>
var canvas = document.getElementById('myCanvas');
var context = canvas.getContext('2d');
// Drawing a filled rectangle
context.fillStyle = 'blue';
context.fillRect(50, 50, 100, 80);
// Drawing a stroked rectangle
context.strokeStyle = 'black';
context.strokeRect(150, 100, 80, 50);
</script>
🧠 How it works?
In this example, we've drawn a filled blue rectangle and a stroked black rectangle on the canvas.
🎉 Conclusion
Using the canvas element and JavaScript, you can dynamically draw rectangles and other shapes to create interactive and visually engaging web content.
Understanding the syntax and parameters for drawing rectangles on the canvas is a foundational skill for web developers and designers looking to leverage the full potential of HTML5 graphics.
👨💻 Join our Community:
Author
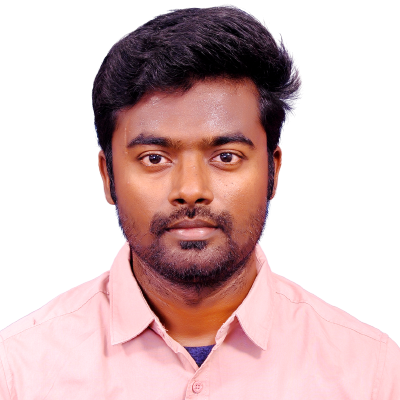
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Canvas Draw Rectangles), please comment here. I will help you immediately.