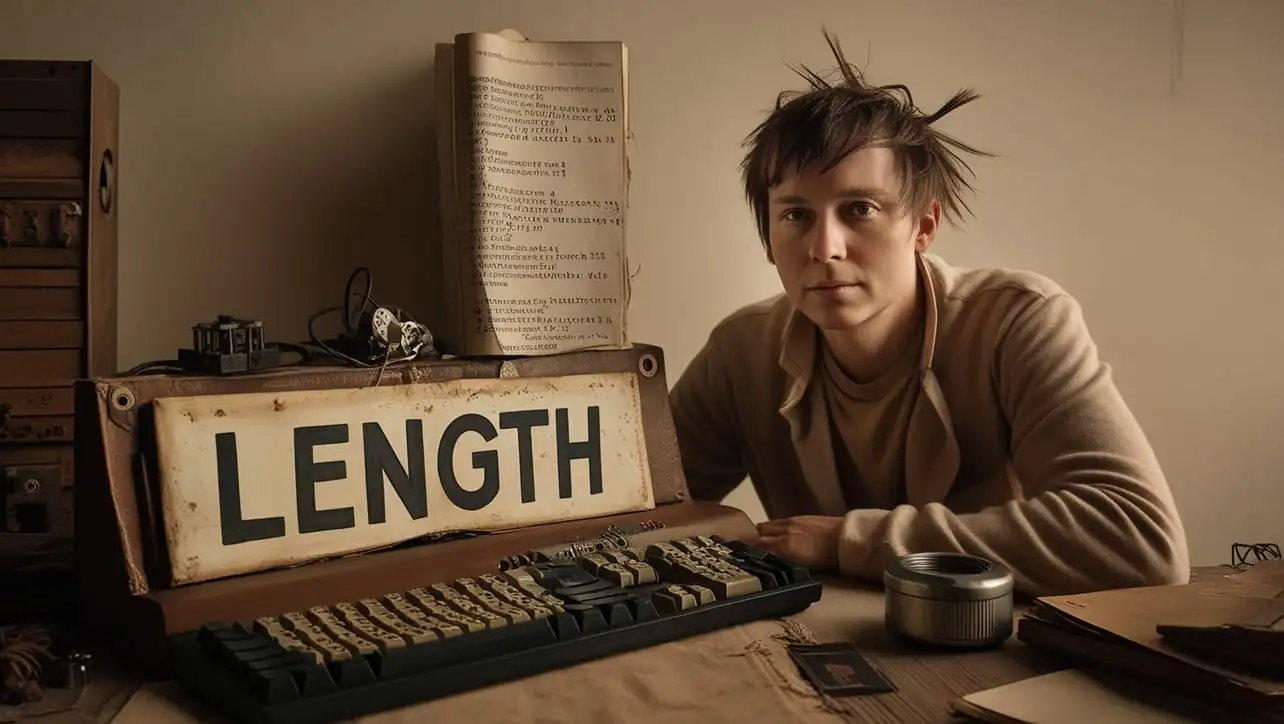
JavaScript Node Methods
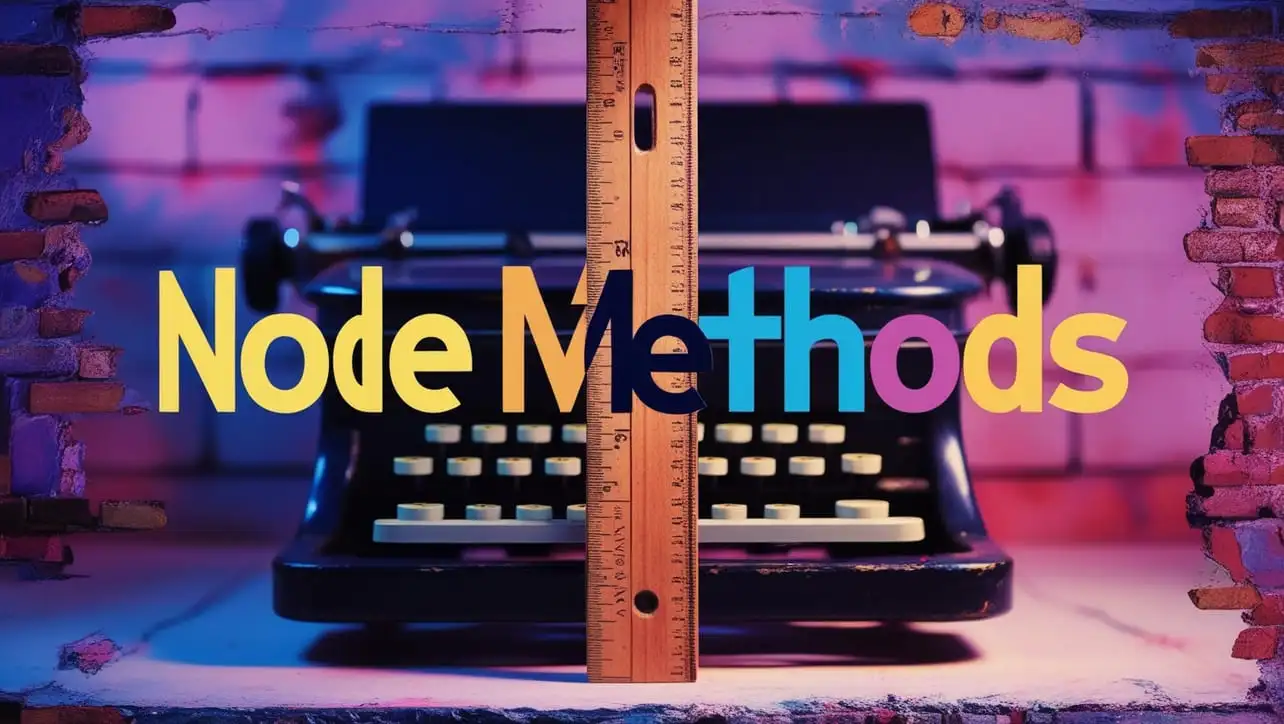
Photo Credit to CodeToFun
Introduction
JavaScript provides a variety of methods for working with nodes in the Document Object Model (DOM). Nodes represent different parts of an HTML or XML document, and these methods enable developers to manipulate, traverse, and interact with nodes efficiently.
In this reference guide, we'll explore some commonly used JavaScript Node Methods to enhance your understanding and usage of nodes in the DOM.
Table of Contents
Some of the commonly used node methods in JavaScript includes:
Methods | Explanation |
---|---|
appendChild() | Used to add a new child node to the end of a list of child nodes for a specified parent node. |
cloneNode() | Allows the creation of a duplicate of a node, including all of its attributes and child nodes. |
compareDocumentPosition() | Compares the position of two nodes in a document, providing information about their relative placement. |
getFeature() | Used to obtain a specific interface from a Node in the Document Object Model (DOM), facilitating advanced manipulation of XML documents. |
getUserData() | Used to retrieve user-specific data or information. |
hasAttributes() | Checks if the node has any attributes, returning true if it does and false otherwise. |
hasChildNodes() | Returns a boolean indicating whether a node has child nodes or not. |
insertBefore() | Used to insert a node before a specified child node within a parent element, providing flexibility in dynamically manipulating the DOM. |
isDefaultNamespace() | Used to check if a given namespace URI is the default namespace for the specified node. |
isEqualNode() | Used to check if two nodes are equal, comparing both their properties and children. |
isSameNode() | Determines if two nodes are the same, checking if they reference the exact same node in the DOM tree. |
lookupNamespaceURI() | Used to retrieve the namespace URI associated with a given prefix in a node. |
lookupPrefix() | Used to retrieve the namespace prefix associated with a specified namespace URI in a given Node. |
normalize() | Used to ensure that the text content within a node is in a normalized form, resolving any whitespace or structural inconsistencies. |
removeChild() | Used to remove a specified child node from the DOM (Document Object Model). |
replaceChild() | Replaces a child node with a new node within the parent node. |
setUserData() | Used to associate arbitrary data with a Node, providing a way to store additional information related to the node. |
Usage Tips
Explore the following tips to make the most out of JavaScript Node Methods:
Understanding Node Relationships:
Familiarize yourself with parent-child relationships between nodes to use traversal methods effectively.
Error Handling:
Check for null or undefined values when dealing with methods that return nodes.
Deep Cloning:
Understand the difference between shallow and deep cloning when using cloneNode method.
Feature Detection:
Use feature detection methods to ensure compatibility when working with certain features.
Node Replacement:
When using replaceChild, consider using the returned node to retain a reference to the removed node.
Normalization:
Apply normalize to merge adjacent text nodes and enhance document cleanliness.
Example
Let's look at a practical example of using JavaScript Node Methods to manipulate and traverse the DOM:
// Example: Using JavaScript Node Methods
// Creating a new element
const newElement = document.createElement('div');
newElement.textContent = 'New Node';
// Appending the new element to an existing element
const parentElement = document.getElementById('parent');
parentElement.appendChild(newElement);
// Cloning a node
const clonedNode = newElement.cloneNode(true);
// Inserting the cloned node before another node
const referenceNode = document.getElementById('reference');
parentElement.insertBefore(clonedNode, referenceNode);
// Removing a child node
const removedNode = parentElement.removeChild(newElement);
console.log('Successfully manipulated and traversed the DOM.');
This example demonstrates the use of appendChild, cloneNode, insertBefore, and removeChild methods to modify the DOM structure. Experiment with different methods and parameters to enhance your interaction with the DOM.
Conclusion
This reference guide provides an overview of essential JavaScript Node Methods. By incorporating these methods into your code, you can efficiently manipulate and traverse the DOM to create dynamic and interactive web pages. Experiment with these methods to discover their versatility and enhance your JavaScript programming skills.
Join our Community:
Author
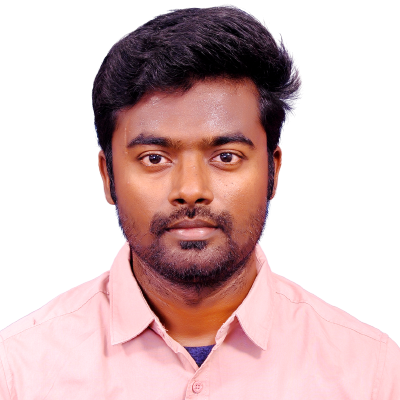
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Node Methods), please comment here. I will help you immediately.