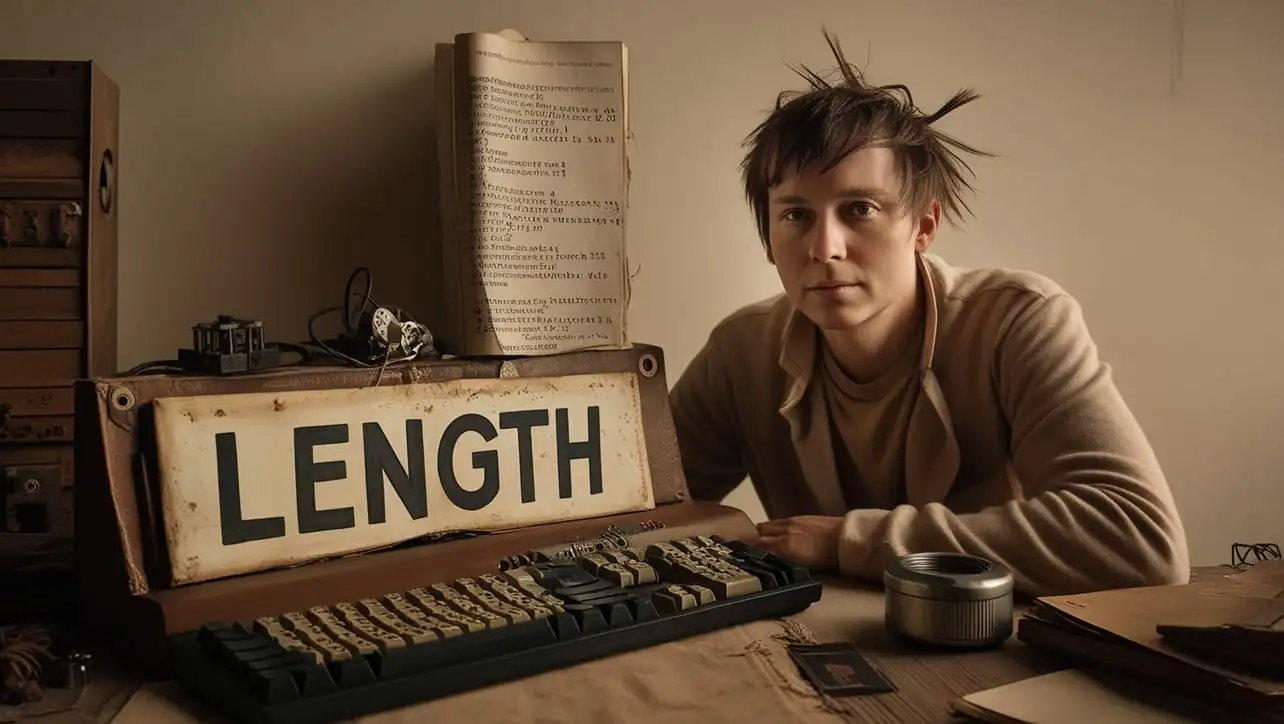
JS Window Methods
JavaScript Window setInterval() Method

Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, the setInterval()
method is a powerful tool that enables you to repeatedly execute a specified function at defined intervals. This functionality is particularly useful for creating animations, managing timed events, and periodically updating content on a webpage.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the setInterval()
method.
🧠 Understanding setInterval() Method
The setInterval()
method is part of the Window object in JavaScript and is used to repeatedly execute a function or a code snippet at specified intervals. It continues to execute until cleared by the clearInterval() method or the webpage is closed.
💡 Syntax
The syntax for the setInterval()
method is straightforward:
setInterval(function, milliseconds, param1, param2, ...);
- function: The function to be executed at each interval.
- milliseconds: The time interval, in milliseconds, at which the function should be executed.
- param1, param2, ... (Optional): Optional parameters to pass to the function.
📝 Example
Let's look at a simple example to demonstrate the usage of the setInterval()
method:
// Function to be executed
function logMessage() {
console.log('Interval elapsed! Executing function...');
}
// Set interval to execute the function every 2000 milliseconds (2 seconds)
const intervalId = setInterval(logMessage, 2000);
In this example, the logMessage function will be executed every 2 seconds.
🏆 Best Practices
When working with the setInterval()
method, consider the following best practices:
Clear Intervals When Not Needed:
Always clear intervals using clearInterval() when they are no longer required to prevent unnecessary execution.
example.jsCopied// Clear the interval after a specific condition is met if (someCondition) { clearInterval(intervalId); }
Avoid Overlapping Intervals:
Ensure that intervals do not overlap, as this can lead to unexpected behavior. Check if the interval is already set before creating a new one.
example.jsCopiedif (!intervalId) { intervalId = setInterval(logMessage, 2000); }
Use Anonymous Functions Judiciously:
While you can use anonymous functions directly as arguments, consider defining named functions for better readability and maintainability.
example.jsCopied// Anonymous function example setInterval(function () { console.log('Anonymous function executed!'); }, 3000); // Named function for better readability function namedFunction() { console.log('Named function executed!'); } setInterval(namedFunction, 3000);
In this example, using a named function (namedFunction) enhances readability and makes the code more maintainable compared to using an anonymous function directly.
📚 Use Cases
Creating Simple Animations:
The
setInterval()
method is commonly used to create simple animations by updating the properties of HTML elements at regular intervals.example.jsCopiedconst movingElement = document.getElementById('animateMe'); let position = 0; setInterval(function () { position += 5; movingElement.style.left = position + 'px'; }, 100);
Real-time Data Updates:
For applications requiring real-time data updates, such as live counters or clocks,
setInterval()
is an excellent choice.example.jsCopiedfunction updateClock() { const currentTime = new Date(); const formattedTime = currentTime.toLocaleTimeString(); document.getElementById('clock').innerText = formattedTime; } setInterval(updateClock, 1000);
🎉 Conclusion
The setInterval()
method is a valuable asset for JavaScript developers, providing a convenient way to execute functions at regular intervals.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setInterval()
method in your JavaScript projects.
👨💻 Join our Community:
Author
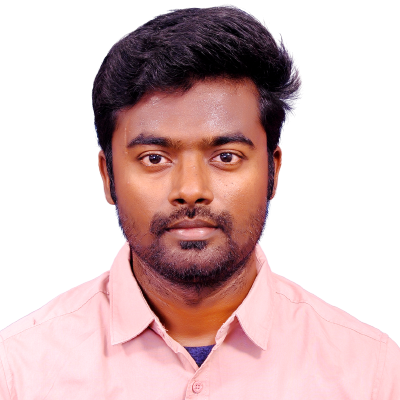
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window setInterval() Method), please comment here. I will help you immediately.