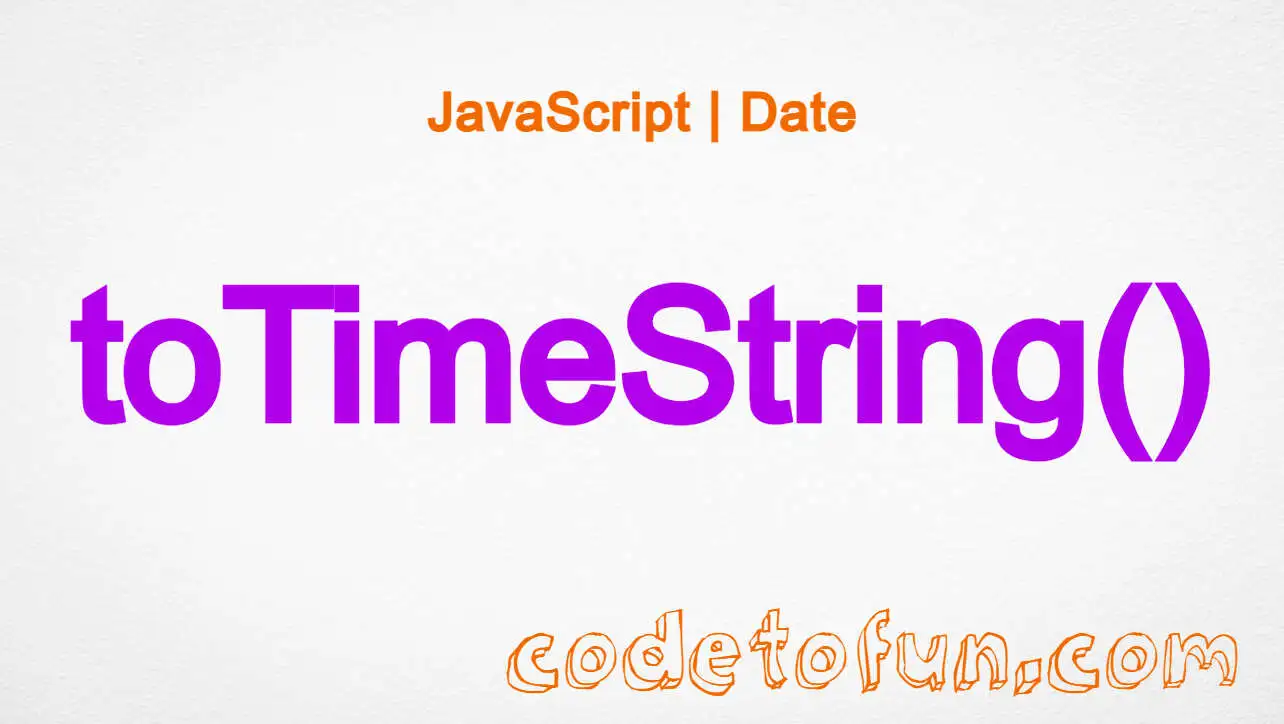
JS Window Methods
JavaScript Window clearInterval() Method
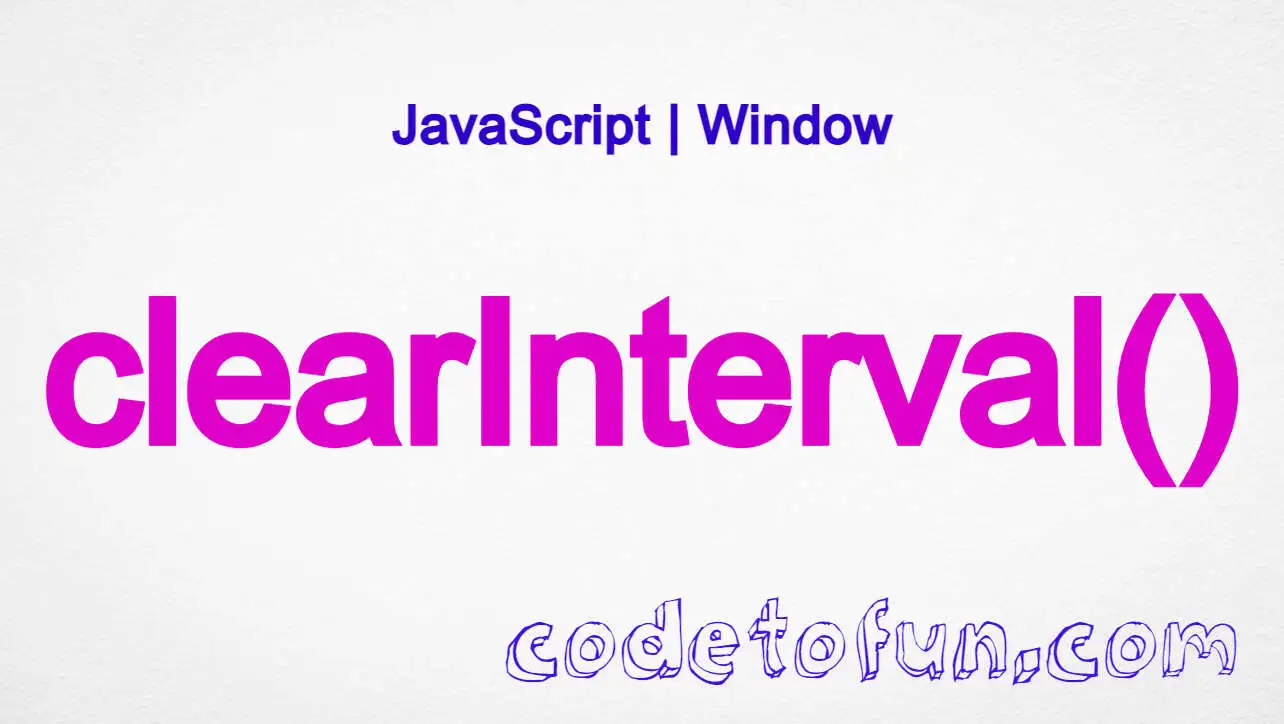
Photo Credit to CodeToFun
🙋 Introduction
Timers in JavaScript are crucial for executing code at specified intervals. The setInterval() function is commonly used to repeatedly invoke a function. However, to control and stop this repetition, the clearInterval()
method comes into play.
In this guide, we'll explore the clearInterval()
method, its syntax, usage, best practices, and practical examples.
🧠 Understanding clearInterval() Method
The clearInterval()
method is utilized to stop the execution of code specified by the setInterval() function. It takes the interval ID returned by setInterval() as its parameter, allowing you to target and halt a specific interval.
💡 Syntax
The syntax for the clearInterval()
method is straightforward:
clearInterval(intervalId);
- intervalId: The ID of the interval to be cleared, as returned by the setInterval() function.
📝 Example
Let's look at a simple example to understand how clearInterval()
works:
// Set up a simple interval function
const intervalId = setInterval(() => {
console.log('Executing repeated code...');
}, 1000);
// After 5 seconds, stop the interval
setTimeout(() => {
clearInterval(intervalId);
console.log('Interval cleared after 5 seconds.');
}, 5000);
In this example, the setInterval() function is used to log a message every second, and after 5 seconds, the clearInterval()
method is employed to stop the repetition.
🏆 Best Practices
When working with the clearInterval()
method, consider the following best practices:
Store Interval IDs:
Keep track of interval IDs in a variable to have a reference for later clearing.
example.jsCopiedconst intervalId = setInterval(() => { // Code to be executed at intervals }, 1000); // Later in the code clearInterval(intervalId);
Clear Unused Intervals:
Ensure to clear intervals that are no longer needed to avoid unnecessary resource consumption.
example.jsCopiedconst intervalId = setInterval(() => { // Code to be executed at intervals }, 1000); // After a certain condition is met if (someCondition) { clearInterval(intervalId); }
Compatibility Checking:
Verify the compatibility of the
clearInterval()
method in your target environments.
📚 Use Cases
Animations and Updates:
setInterval() is commonly used for animations or periodic updates.
clearInterval()
becomes essential when you want to stop these animations or updates at a specific point.example.jsCopiedconst animationInterval = setInterval(() => { // Code for animation or periodic update }, 100); // After a certain event, stop the animation stopButton.addEventListener('click', () => { clearInterval(animationInterval); console.log('Animation stopped.'); });
Real-time Data Polling:
In scenarios where you are polling for real-time data,
clearInterval()
can be employed to stop the polling when the required data is received.example.jsCopiedconst dataPollingInterval = setInterval(() => { // Code to poll for real-time data }, 2000); // After receiving the necessary data if (dataReceived) { clearInterval(dataPollingInterval); console.log('Data polling stopped after receiving required data.'); }
🎉 Conclusion
The clearInterval()
method is a vital tool for managing intervals in JavaScript. Whether you are dealing with animations, periodic updates, or data polling, understanding how to use clearInterval()
effectively is crucial.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the clearInterval()
method in your JavaScript projects.
👨💻 Join our Community:
Author
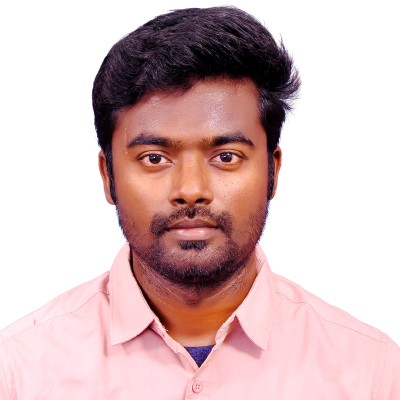
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window clearInterval() Method), please comment here. I will help you immediately.