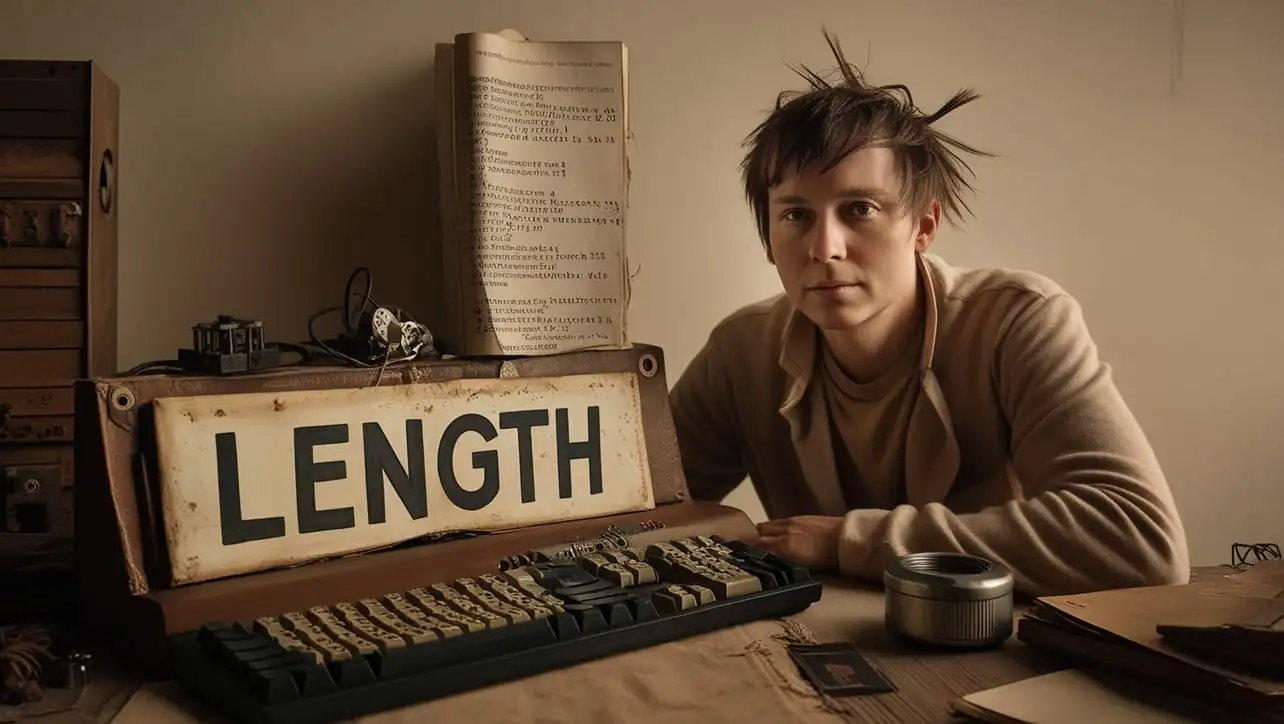
JS String Methods
JavaScript String Methods
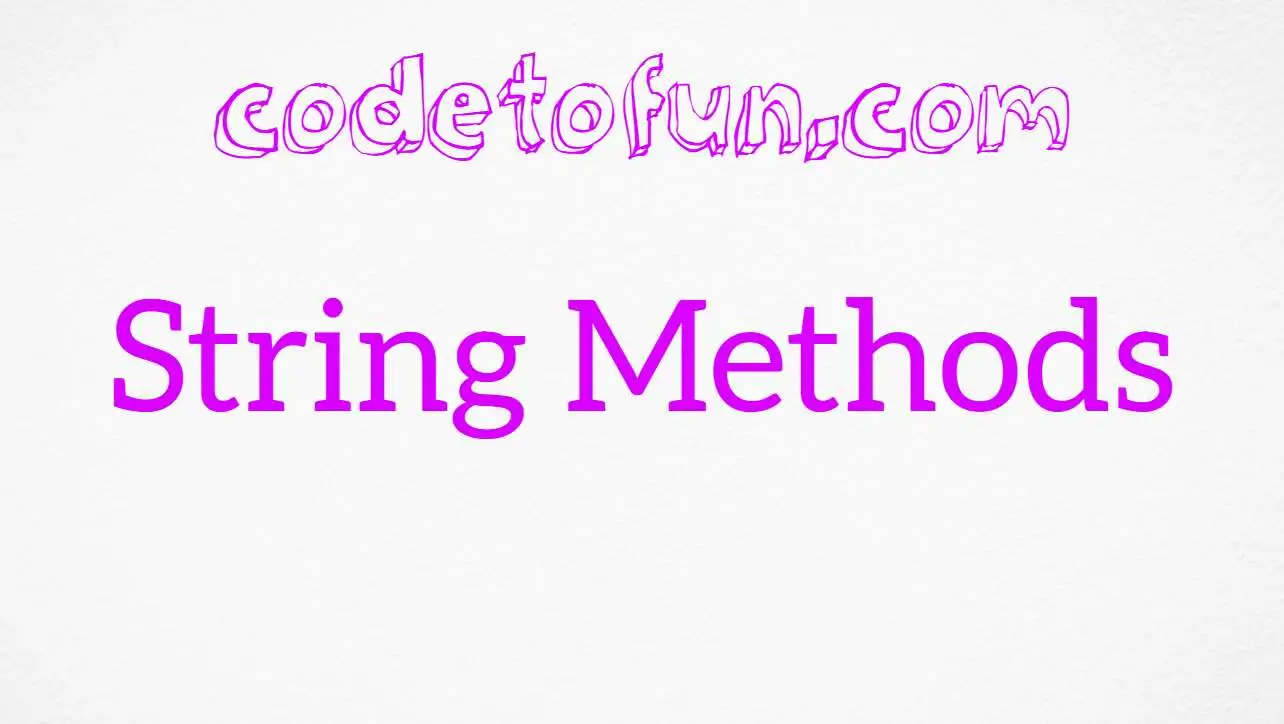
Photo Credit to CodeToFun
🙋 Introduction
JavaScript provides a wide array of built-in methods for working with strings. These methods enable you to manipulate, analyze, and transform strings efficiently.
In this reference guide, we'll explore some commonly used JavaScript string methods to enhance your string manipulation capabilities in JavaScript.
📑 Table of Contents
Some of the commonly used string methods in JavaScript includes:
Methods | Explanation |
---|---|
at() | Retrieves the character at a specified index within a string. |
charAt() | Returns the character at a specified index in a string, providing a simple way to access individual characters. |
charCodeAt() | Returns the Unicode value of the character at a specified index within a string. |
codePointAt() | Returns the Unicode code point of the character at a specified index in a string, providing a way to handle non-BMP (Basic Multilingual Plane) characters. |
concat() | Concatenate two or more strings and returns a new string without modifying the existing ones. |
endsWith() | Checks whether a string ends with a specified sequence of characters, returning true or false. |
fromCharCode() | Converts Unicode values to characters, creating a string from the specified sequence of Unicode values. |
includes() | Checks if a string contains another specified string, returning true if found, and false otherwise. |
indexOf() | Returns the index of the first occurrence of a specified substring within a string, or -1 if not found. |
lastIndexOf() | Returns the position of the last occurrence of a specified value within a string. |
localeCompare() | Used to compare strings based on the current locale, providing a mechanism for language-sensitive string comparison. |
match() | Searches a string for a specified pattern and returns an array of matches.. |
padEnd() | Used to pad a string with a specified character or characters until it reaches a specified length. |
padStart() | Used to pad a string with a specified character to reach a desired length from the start. |
repeat() | Used to generate a new string by repeating the original string a specified number of times. |
replace() | Used to replace specified text in a string with another string. |
replaceAll() | Globally replaces all occurrences of a specified substring with another substring in a string. |
search() | Returns the index of the first occurrence of a specified value within a string, or -1 if not found. |
slice() | Extracts a section of a string and returns it as a new string without modifying the original string. |
split() | Separates a string into an array of substrings based on a specified delimiter. |
startsWith() | Checks if a string starts with a specified sequence of characters, returning true or false. |
substr() | Extracts a portion of a string based on specified starting and optional ending indices. |
substring() | Extracts a portion of a string based on specified indices, providing a concise way to manipulate string content. |
toLocaleLowerCase() | Converts a string to lowercase while considering the locale-specific case mappings. |
toLocaleUpperCase() | Converts a string to uppercase letters, considering the locale-specific case mappings. |
toLowerCase() | Converts all the characters in a string to lowercase, providing a convenient way to standardize letter case. |
toString() | Converts a string object to a string and returns the result. |
toUpperCase() | Converts all characters in a string to uppercase, providing a simple way to manipulate text case. |
trim() | Removes leading and trailing whitespace from a string, providing a clean version of the original string. |
trimEnd() | Removes trailing whitespace from the end of a string. |
trimStart() | Removes whitespace characters from the beginning of a string. |
valueOf() | Returns the primitive value of a string object. |
🚀 Usage Tips
Explore the following tips to effectively use JavaScript string methods:
Immutability:
Remember that most string methods do not modify the original string but return a new one. Assign the result to a variable or use it directly.
Regular Expressions:
Utilize regular expressions for powerful and flexible string matching and manipulation.
Parameter Awareness:
Understand optional parameters and their default values to use methods effectively.
Chaining Methods:
Combine multiple string methods in a chain to achieve complex transformations.
📝 Example
Let's illustrate the usage of JavaScript string methods in a practical example:
// Example: Using JavaScript string methods for string manipulation
const originalString = "hello world";
const modifiedString = originalString
.toUpperCase()
.replace("WORLD", "universe")
.slice(0, 6);
console.log(`Original String: ${originalString}`);
console.log(`Modified String: ${modifiedString}`);
In this example, we chain together toUpperCase, replace, and slice methods to transform the original string. Experiment with different methods and parameters to unleash the full potential of JavaScript string methods.
🎉 Conclusion
This reference guide offers insights into essential JavaScript string methods. By incorporating these methods into your code, you can efficiently manipulate and analyze strings in various ways. Experiment with these methods to discover their versatility and enhance your JavaScript programming skills.
👨💻 Join our Community:
Author
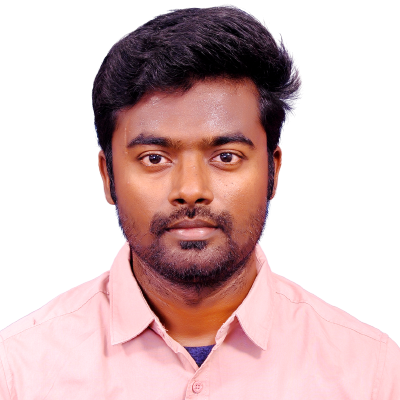
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript String Methods), please comment here. I will help you immediately.