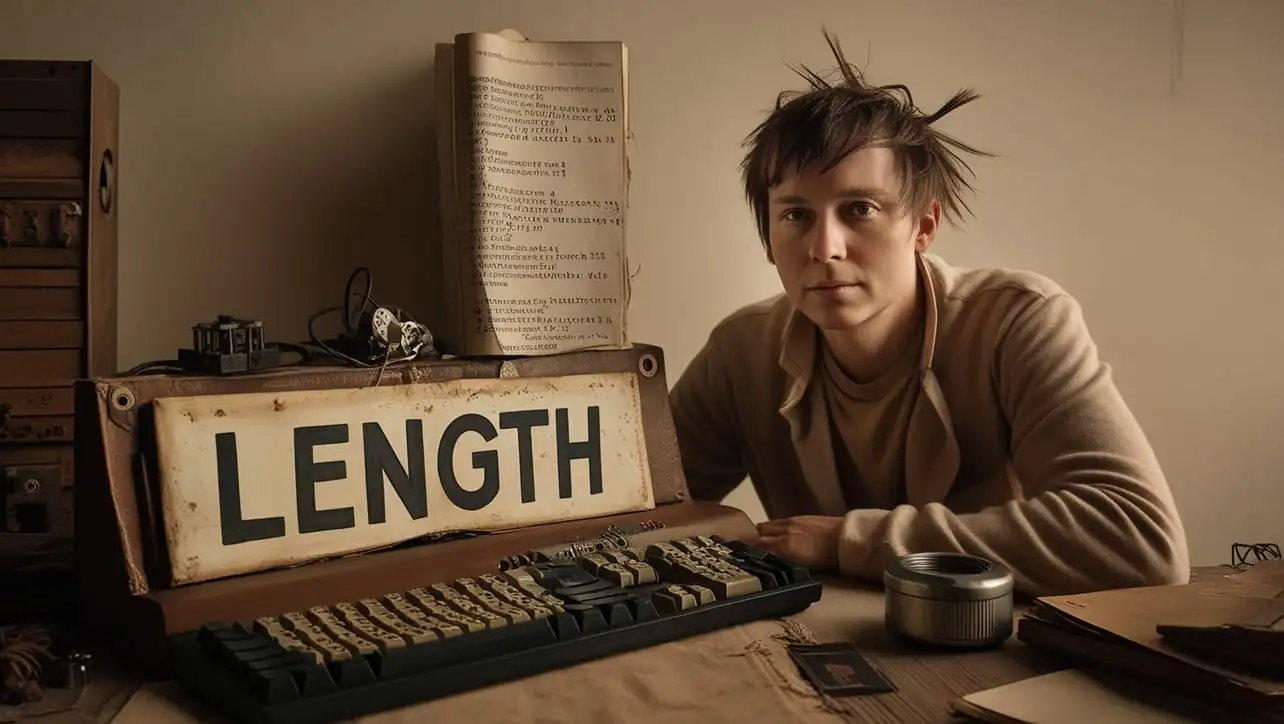
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array with() Method

Photo Credit to CodeToFun
Introduction
In the realm of JavaScript arrays, the with()
method emerges as a powerful tool for efficiently modifying array elements. It serves as a copying mechanism, allowing you to replace the value at a specific index with a new one.
In this guide, we'll explore the syntax, functionality, and best practices associated with the with()
method.
Understanding with() Method
The with()
method for Array instances is akin to using bracket notation to alter the value of a given index. What distinguishes it is its ability to create a new array with the specified modification, leaving the original array untouched. This method proves useful when you need to make changes without directly mutating the existing array.
Syntax
The syntax for the with()
method is straightforward:
const newArray = array.with(index, newValue);
- array: The array you want to operate on.
- index: The index of the element you want to replace.
- newValue: The new value you want to assign to the specified index.
Example
Let's delve into an example to illustrate the application of the with()
method:
// Sample array
const fruits = ['apple', 'orange', 'banana', 'grape', 'kiwi'];
// Using with() to replace an element
const modifiedFruits = fruits.with(2, 'pineapple');
console.log(modifiedFruits);
// Output: ['apple', 'orange', 'pineapple', 'grape', 'kiwi']
In this example, the with()
method creates a new array (modifiedFruits) with the element at index 2 replaced by 'pineapple'.
Best Practices
When working with the with()
method, consider the following best practices:
Immutable Operations:
Leverage the immutability aspect of
with()
by creating new arrays rather than modifying existing ones directly.example.jsCopied// Correct const modifiedArray = originalArray.with(3, 'newElement'); // Avoid mutating the original array // Incorrect: originalArray.with(3, 'newElement');
Error Handling:
Validate the index to prevent potential errors, ensuring it falls within the valid range of the array.
example.jsCopiedconst indexToModify = 4; if (indexToModify >= 0 && indexToModify < fruits.length) { const updatedFruits = fruits.with(indexToModify, 'mango'); console.log(updatedFruits); } else { console.error('Invalid index specified.'); }
Use Cases
Dynamic Array Modification:
The
with()
method shines when you need to dynamically modify array elements based on specific conditions:example.jsCopiedconst dynamicIndex = determineIndex(); const updatedArray = originalArray.with(dynamicIndex, 'newElement'); console.log(updatedArray);
Creating a Copy with Modification:
You can use
with()
to create a modified copy of an array, preserving the original:example.jsCopiedconst originalData = [1, 2, 3, 4, 5]; const modifiedData = originalData.with(2, 999); console.log(originalData); // Original array remains unchanged console.log(modifiedData); // Output: [1, 2, 999, 4, 5]
Conclusion
The with()
method empowers JavaScript developers with a convenient means of modifying array elements while maintaining immutability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the with()
method in your JavaScript projects.
Join our Community:
Author
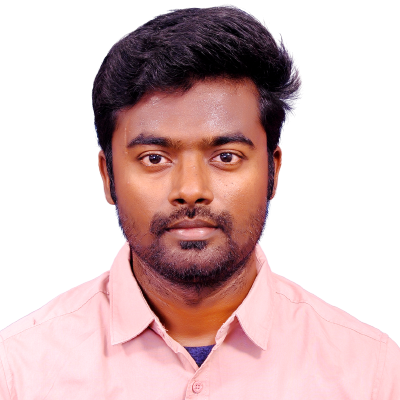
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array with() Method), please comment here. I will help you immediately.