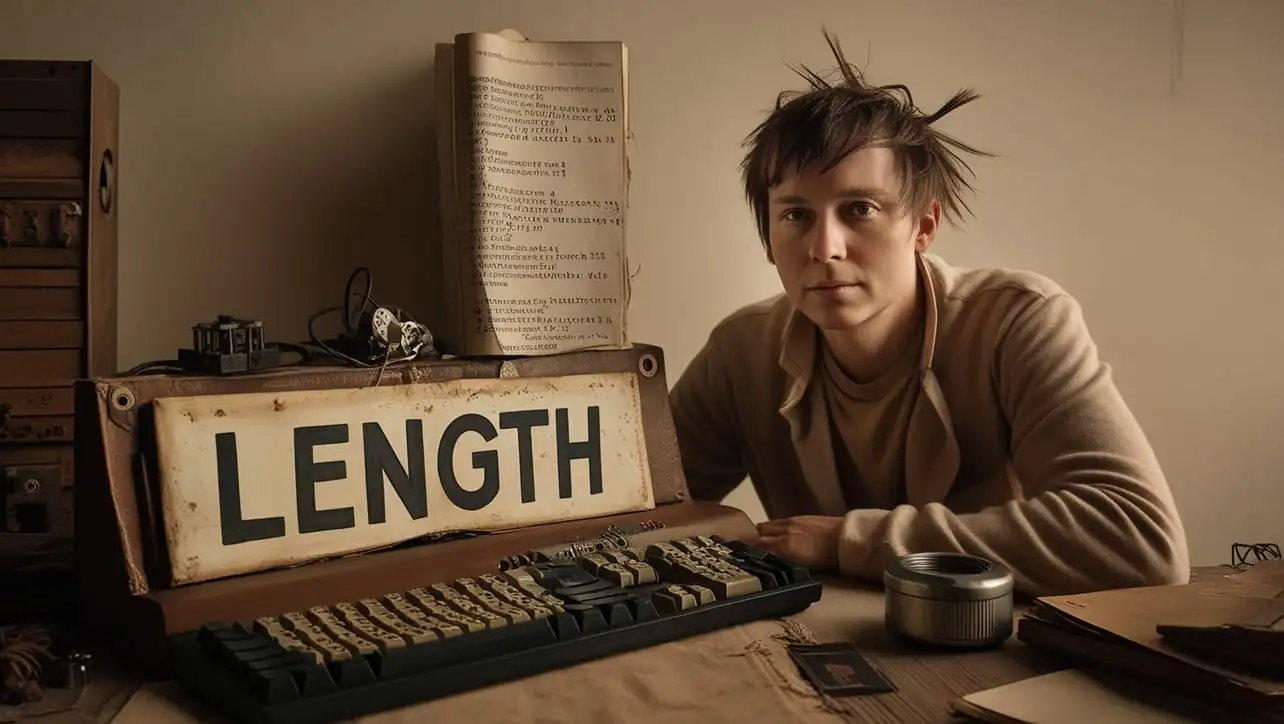
JS Array Methods
JavaScript Array valueOf() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays are fundamental data structures that empower developers to manage collections of elements. The valueOf()
method is a valuable addition to the array toolkit, allowing you to retrieve the primitive value of an array.
In this guide, we'll explore the syntax, usage, best practices, and practical applications of the valueOf()
method.
🧠 Understanding valueOf() Method
The valueOf()
method in JavaScript arrays returns the primitive value of the array. This can be particularly useful when you need to convert an array into a primitive value for comparisons or concatenations.
💡 Syntax
The syntax for the valueOf()
method is straightforward:
array.valueOf();
- array: The array for which you want to retrieve the primitive value.
📝 Example
Let's dive into a simple example to illustrate the usage of the valueOf()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5];
// Using valueOf() to obtain the primitive value
const primitiveValue = numbers.valueOf();
console.log(primitiveValue); // Output: 1,2,3,4,5
In this example, the valueOf()
method is employed to obtain the primitive value of the numbers array.
🏆 Best Practices
When working with the valueOf()
method, consider the following best practices:
Type Checking:
Check the type of the primitive value returned by
valueOf()
to ensure it matches your expectations.example.jsCopiedconst result = numbers.valueOf(); if (typeof result === 'string') { console.log('Primitive value is a string:', result); } else if (typeof result === 'number') { console.log('Primitive value is a number:', result); } else { console.log('Unexpected primitive value type:', result); }
Contextual Usage:
Utilize the
valueOf()
method when you specifically need the primitive value representation of the array.example.jsCopiedconst arrayAsString = 'Array: ' + numbers.valueOf(); console.log(arrayAsString);
📚 Use Cases
Comparing Arrays:
The
valueOf()
method can be useful when comparing arrays for equality, especially in scenarios where primitive value comparison is sufficient:example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [1, 2, 3]; if (array1.valueOf() === array2.valueOf()) { console.log('Arrays are equal.'); } else { console.log('Arrays are not equal.'); }
String Concatenation:
Converting an array to its primitive value using
valueOf()
is handy when you want to concatenate it with a string:example.jsCopiedconst message = 'The array values are: ' + numbers.valueOf(); console.log(message);
🎉 Conclusion
The valueOf()
method is a valuable tool in JavaScript arrays, providing a straightforward way to obtain the primitive value of an array.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the valueOf()
method in your JavaScript projects.
👨💻 Join our Community:
Author
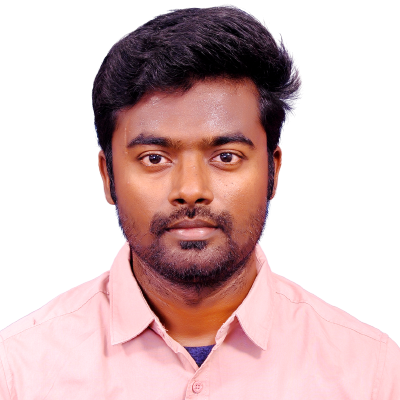
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array valueOf() Method), please comment here. I will help you immediately.