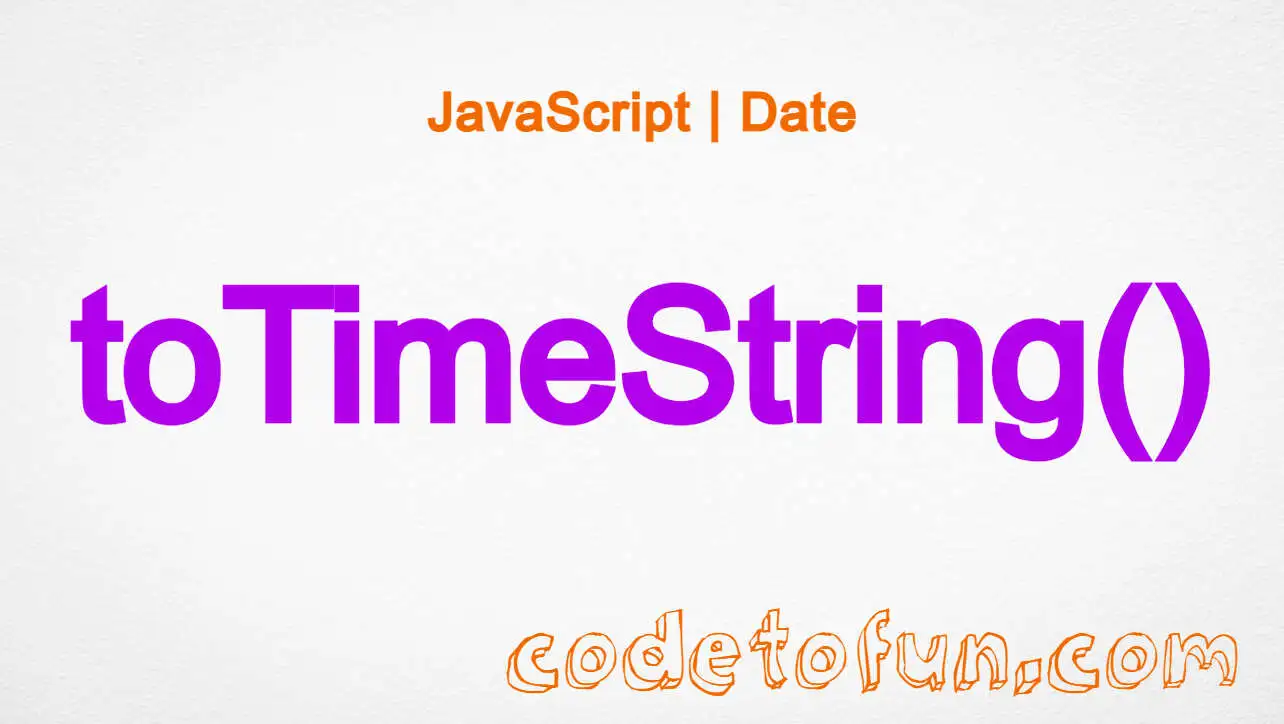
JS Array Methods
JavaScript Array slice() Method
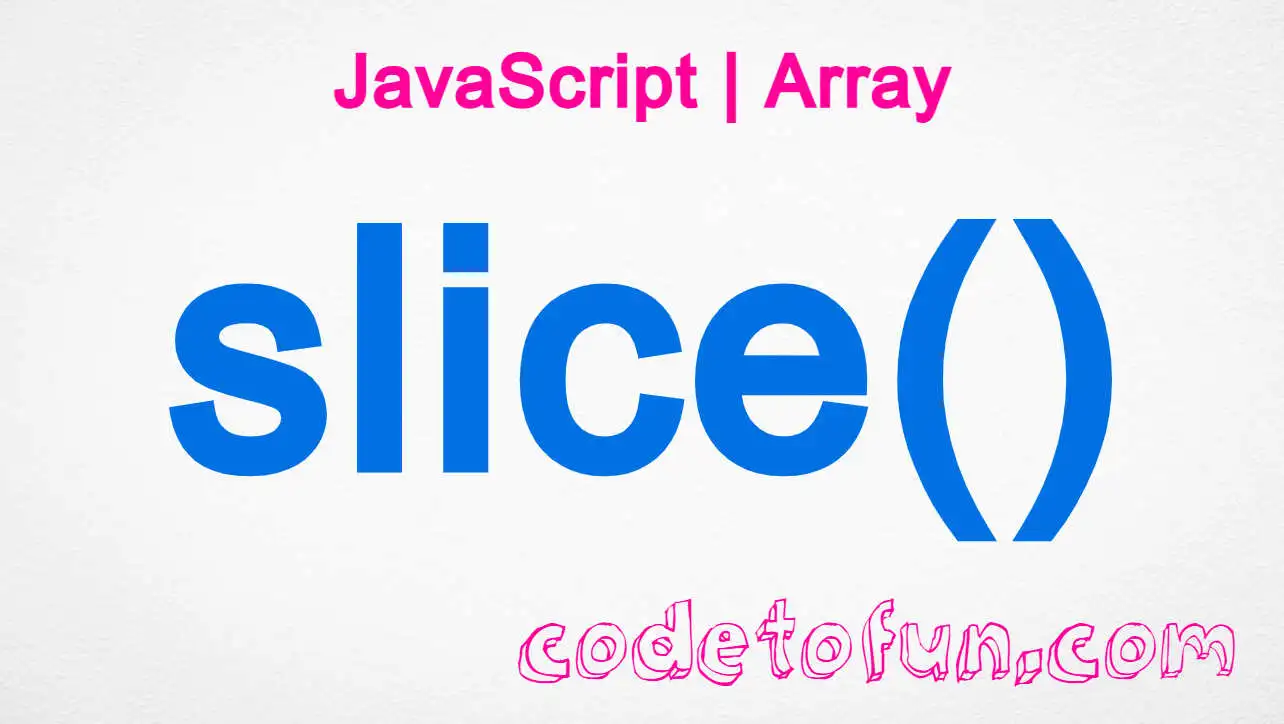
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays provide a multitude of methods for manipulating and extracting data. The slice()
method is a powerful tool that allows you to create a shallow copy of a portion of an array, providing flexibility and control over your data.
In this guide, we'll delve into the slice()
method, understanding its syntax, and exploring its applications in JavaScript programming.
🧠 Understanding slice() Method
The slice()
method is used to extract a section of an array and return a new array with the selected elements. This method does not modify the original array, making it a non-destructive option for array manipulation.
💡 Syntax
The syntax for the slice()
method is straightforward:
array.slice(start, end);
- array: The array from which you want to extract elements.
- start: The zero-based index at which to begin extraction.
- end: The zero-based index before which to stop extraction. The end parameter is optional, and if not specified,
slice()
extracts to the end of the array.
📝 Example
Let's dive into an example to illustrate the usage of the slice()
method:
// Sample array
const fruits = ['apple', 'orange', 'banana', 'grape', 'kiwi'];
// Using slice() to extract a portion
const slicedFruits = fruits.slice(1, 4);
console.log(slicedFruits); // Output: ['orange', 'banana', 'grape']
In this example, the slice()
method is employed to create a new array (slicedFruits) containing elements from index 1 to 3 (excluding the element at index 4).
🏆 Best Practices
When working with the slice()
method, consider the following best practices:
Non-Destructive Operation:
Remember that
slice()
does not modify the original array. If you need to alter the original array, assign the result ofslice()
to a variable or use it directly in your code.example.jsCopied// Modifying the original array const modifiedFruits = fruits.slice(1, 4); console.log(fruits); // Original array remains unchanged
📚 Use Cases
Creating a Copy of an Array:
One common use of
slice()
is to create a shallow copy of an entire array:example.jsCopiedconst copiedArray = fruits.slice(); console.log(copiedArray); // Output: ['apple', 'orange', 'banana', 'grape', 'kiwi']
Selective Extraction for Display:
The
slice()
method is handy when you want to display a subset of array elements, preserving the original array intact:example.jsCopied// Display only the first three fruits const displayFruits = fruits.slice(0, 3); console.log(displayFruits); // Output: ['apple', 'orange', 'banana']
🎉 Conclusion
The slice()
method is a versatile tool in JavaScript for selectively extracting elements from arrays without modifying the original data.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the slice()
method in your JavaScript projects.
👨💻 Join our Community:
Author
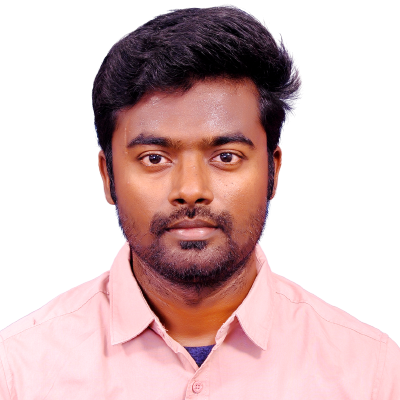
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array slice() Method), please comment here. I will help you immediately.