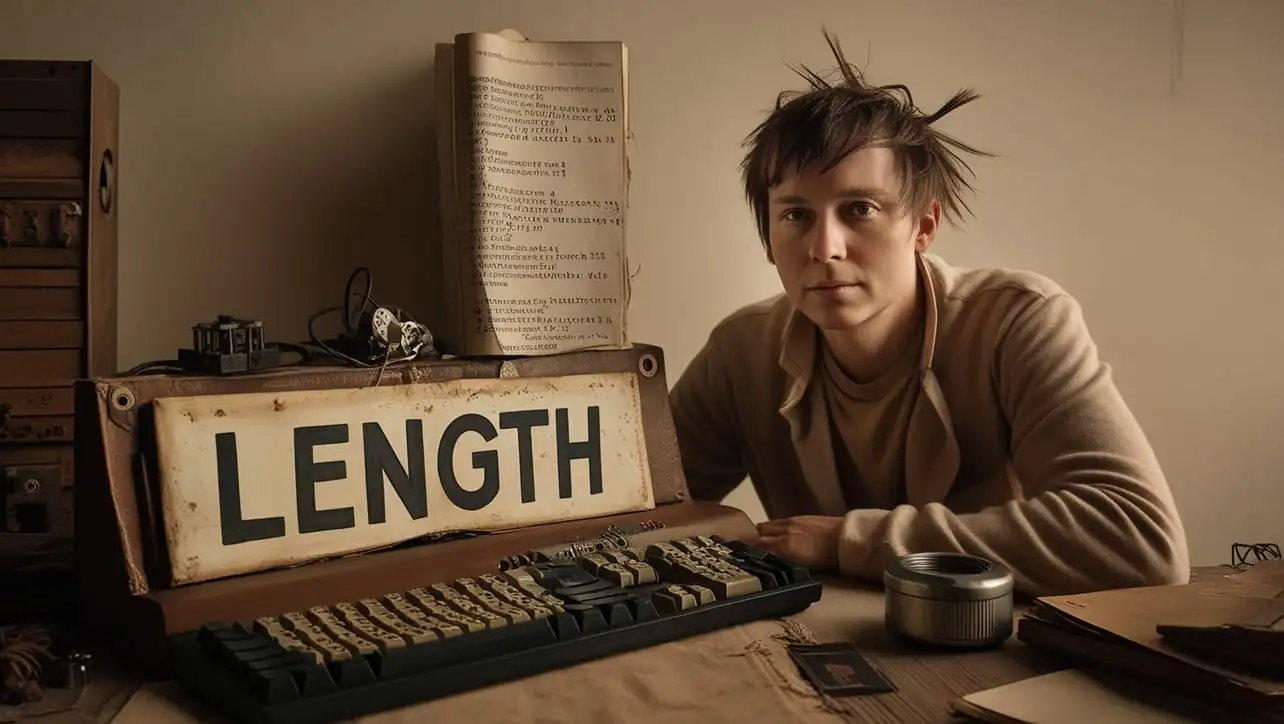
JS Array Methods
JavaScript Array shift() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a plethora of methods for efficient manipulation, and the shift()
method is a powerful addition that allows you to modify the contents of an array by removing its first element.
In this guide, we'll delve into the shift()
method, explore its syntax, and uncover its practical applications in your JavaScript endeavors.
🧠 Understanding shift() Method
The shift()
method is designed to alter the array it is called on by removing the first element and shifting all subsequent elements down by one position. This method is particularly useful when dealing with a queue-like structure, where elements are processed in a first-in, first-out (FIFO) fashion.
💡 Syntax
The syntax for the shift()
method is straightforward:
array.shift();
- array: The array from which you want to remove the first element.
📝 Example
Let's explore a simple example to illustrate the application of the shift()
method:
// Sample array
const colors = ['red', 'blue', 'green', 'yellow'];
// Using shift() to remove the first element
const removedColor = colors.shift();
console.log(removedColor); // Output: 'red'
console.log(colors); // Output: ['blue', 'green', 'yellow']
In this example, shift()
is employed to remove the first element ('red') from the colors array.
🏆 Best Practices
When working with the shift()
method, consider the following best practices:
Array Length Check:
Before calling
shift()
, check if the array is not empty to avoid unexpected behavior.example.jsCopiedif (colors.length > 0) { const removedColor = colors.shift(); console.log(removedColor); } else { console.log('Array is empty.'); }
Performance Considerations:
Keep in mind that removing the first element using
shift()
involves shifting all remaining elements, which may have performance implications for large arrays. If you need to frequently remove elements from the beginning of the array and performance is crucial, consider using a different data structure.
📚 Use Cases
Queue Operations:
The
shift()
method is particularly useful in simulating queue operations, where elements are processed in a first-in, first-out manner. For instance:example.jsCopied// Enqueue const newElement = 'purple'; colors.push(newElement); // Dequeue using shift() const dequeuedColor = colors.shift(); console.log(dequeuedColor); // Output: 'blue' console.log(colors); // Output: ['green', 'yellow', 'purple']
In this example,
shift()
is used to dequeue the first element from the array.Iterating through a Queue:
You can efficiently iterate through a queue-like array using
shift()
until it becomes empty:example.jsCopiedwhile (colors.length > 0) { const currentColor = colors.shift(); console.log(`Processing color: ${currentColor}`); }
This loop will process each element in the array until it is empty.
🎉 Conclusion
The shift()
method is a valuable tool in array manipulation, providing a simple and efficient way to remove the first element and adjust the array accordingly.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the shift()
method in your JavaScript projects.
👨💻 Join our Community:
Author
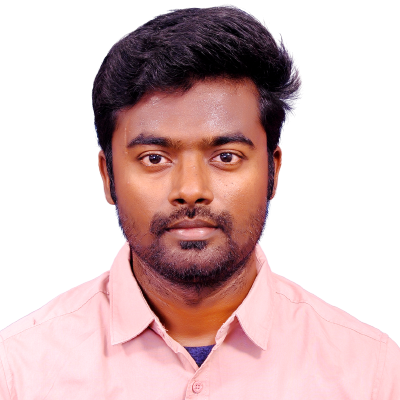
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array shift() Method), please comment here. I will help you immediately.