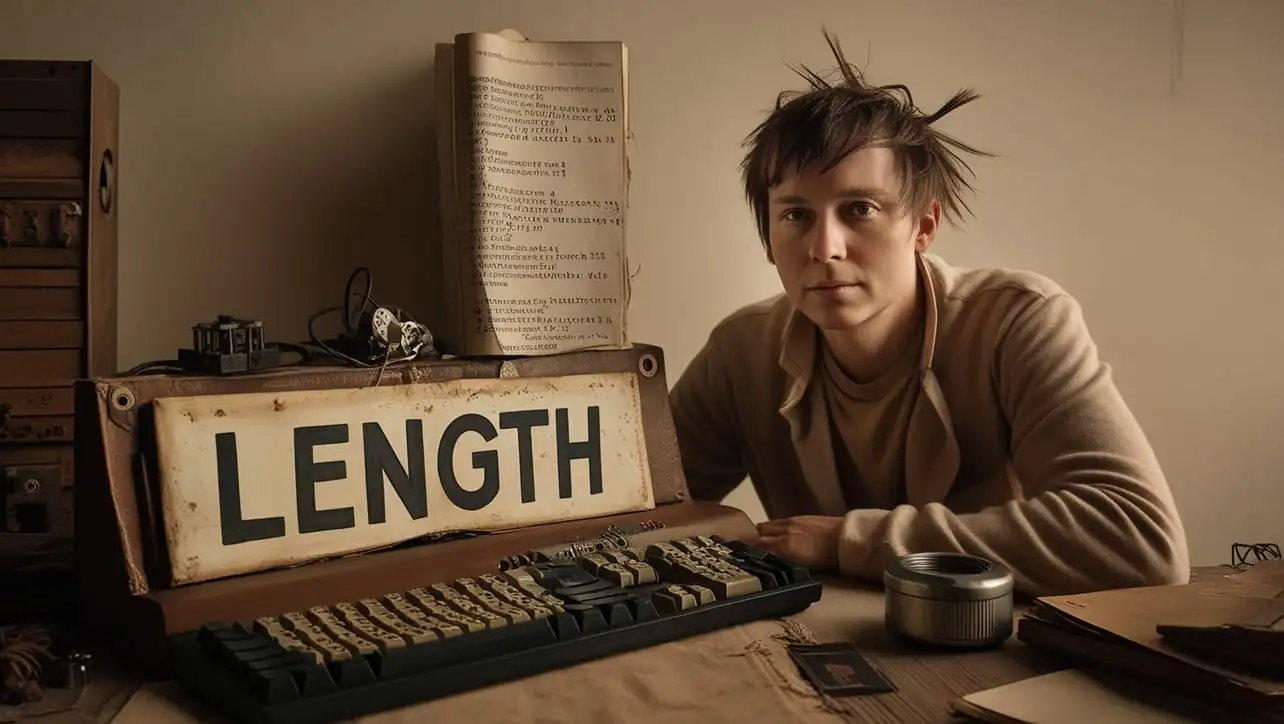
JS Array Methods
JavaScript Array reduce() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays come equipped with powerful methods, and the reduce()
method stands out for its versatility in performing array transformations. This method allows you to iterate over an array, accumulating values and reducing them to a single result.
In this comprehensive guide, we'll explore the reduce()
method, covering its syntax, usage, best practices, and practical applications.
🧠 Understanding reduce() Method
The reduce()
method is used to reduce the elements of an array to a single value. It takes a callback function as its argument, which is executed for each element in the array, resulting in a cumulative value. This cumulative value is then returned as the final result.
💡 Syntax
The syntax for the reduce()
method is straightforward:
array.reduce(callback, initialValue);
- array: The array to be reduced.
- callback: A function that is called once for each element in the array.
- initialValue: An optional initial value for the accumulator.
📝 Example
Let's explore a simple example to illustrate the basic usage of the reduce()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5];
// Using reduce() to calculate the sum of elements
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(sum); // Output: 15
In this example, the reduce()
method is employed to calculate the sum of all elements in the numbers array.
🏆 Best Practices
When working with the reduce()
method, consider the following best practices:
Provide an Initial Value:
While the initialValue parameter is optional, it's often a good practice to provide one. This ensures predictable behavior, especially when dealing with empty arrays.
example.jsCopiedconst result = numbers.reduce((accumulator, currentValue) => { return accumulator + currentValue; }, 0);
Error Handling in Callback:
Include error handling in the callback function to prevent unexpected behavior. For instance, check if the values are of the expected types before performing operations.
example.jsCopiedconst result = numbers.reduce((accumulator, currentValue) => { if (typeof currentValue === 'number') { return accumulator + currentValue; } else { throw new Error('Invalid array element type'); } }, 0);
Compatibility Checking:
Verify the compatibility of the
reduce()
method in your target environments, particularly if you need to support older browsers.example.jsCopied// Check if the reduce() method is supported if (Array.prototype.reduce) { // Use the method safely const result = numbers.reduce((accumulator, currentValue) => { return accumulator + currentValue; }, 0); console.log(result); } else { console.error('reduce() method not supported in this environment.'); }
📚 Use Cases
Calculating Average:
The
reduce()
method can be utilized to calculate the average of an array of numbers:example.jsCopiedconst average = numbers.reduce((accumulator, currentValue, index, array) => { accumulator += currentValue; if (index === array.length - 1) { return accumulator / array.length; } else { return accumulator; } }, 0); console.log(average);
Flattening an Array:
To flatten an array of arrays using
reduce()
:example.jsCopiedconst nestedArray = [[1, 2], [3, 4], [5, 6]]; const flattenedArray = nestedArray.reduce((accumulator, currentValue) => { return accumulator.concat(currentValue); }, []); console.log(flattenedArray);
🎉 Conclusion
The reduce()
method is a powerful tool in the JavaScript array toolkit, enabling concise and efficient transformations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the reduce()
method in your JavaScript projects.
👨💻 Join our Community:
Author
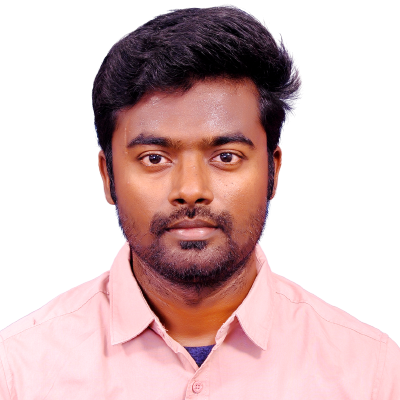
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array reduce() Method), please comment here. I will help you immediately.