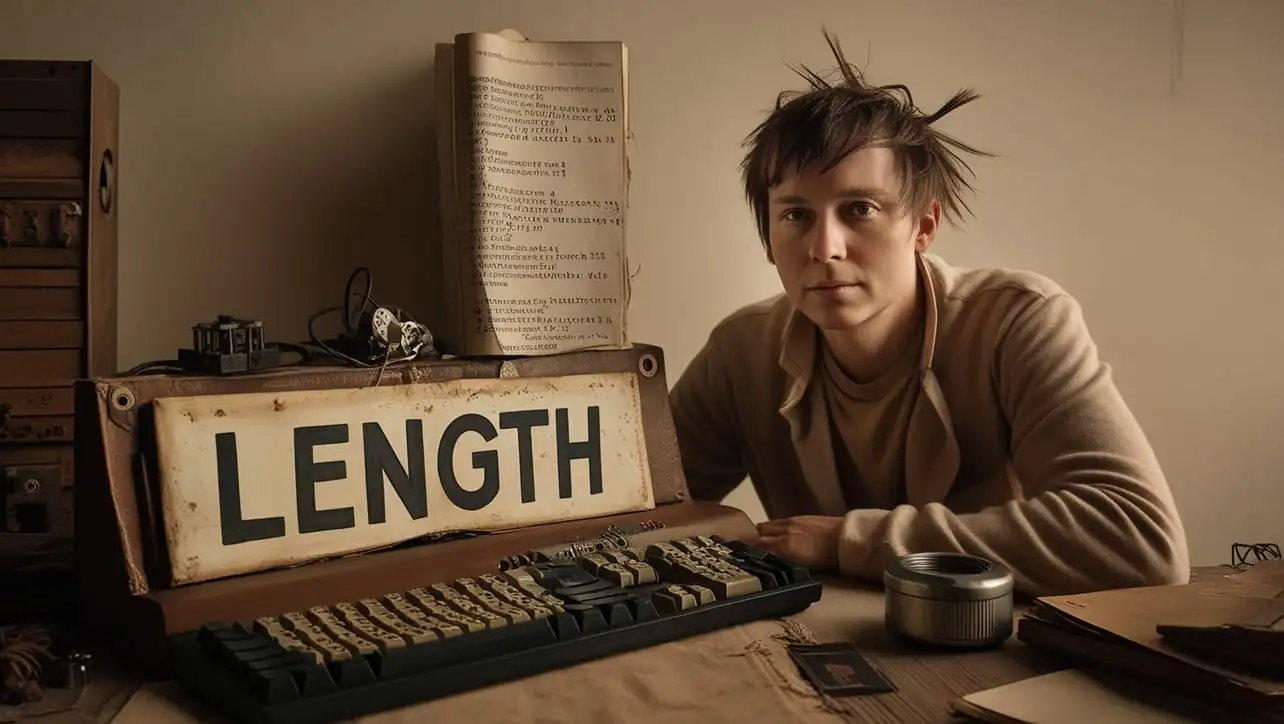
JS Array Methods
JavaScript Array lastIndexOf() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a plethora of methods for efficient data manipulation, and the lastIndexOf()
method is a powerful addition when it comes to searching for elements. This method allows you to find the last occurrence of a specified element within an array.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the lastIndexOf()
method.
🧠 Understanding lastIndexOf() Method
The lastIndexOf()
method returns the last index at which a specified element is found in an array. It searches the array from the end to the beginning, making it a valuable tool for scenarios where you need to locate the last occurrence of a particular value.
💡 Syntax
The syntax for the lastIndexOf()
method is straightforward:
array.lastIndexOf(searchElement, fromIndex);
- array: The array in which to search for the element.
- searchElement: The element to locate within the array.
- fromIndex (optional): The index at which to start searching. The search is performed backward from this index. If not specified, the search starts from the last element.
📝 Example
Let's dive into a simple example to illustrate the usage of the lastIndexOf()
method:
// Sample array
const numbers = [1, 2, 3, 4, 2, 5, 2];
// Using lastIndexOf() to find the last occurrence of the element 2
const lastIndex = numbers.lastIndexOf(2);
console.log(`Last occurrence of 2 is at index ${lastIndex}`);
In this example, the lastIndexOf()
method is employed to find the last occurrence of the element 2 within the numbers array.
🏆 Best Practices
When working with the lastIndexOf()
method, consider the following best practices:
Check for -1:
The
lastIndexOf()
method returns -1 if the specified element is not found. Always check for this value to handle scenarios where the element is not present in the array.example.jsCopiedconst elementToFind = 7; const lastIndex = numbers.lastIndexOf(elementToFind); if (lastIndex !== -1) { console.log(`Last occurrence of ${elementToFind} is at index ${lastIndex}`); } else { console.log(`${elementToFind} not found in the array`); }
Understanding fromIndex:
Be mindful of how the fromIndex parameter affects the search. If provided, the search starts from the specified index backward. If not specified, the search begins from the last element.
📚 Use Cases
Removing the Last Occurrence:
The
lastIndexOf()
method is handy when you need to remove the last occurrence of a specific element from an array:example.jsCopiedconst elementToRemove = 2; const lastIndex = numbers.lastIndexOf(elementToRemove); if (lastIndex !== -1) { numbers.splice(lastIndex, 1); console.log(`Removed last occurrence of ${elementToRemove}. Updated array:`, numbers); } else { console.log(`${elementToRemove} not found in the array`); }
Finding the Last Index of a Subarray:
You can use
lastIndexOf()
to find the last index of a subarray within a larger array:example.jsCopiedconst subArray = [2, 5]; const lastIndexOfSubArray = numbers.lastIndexOf(subArray[0], numbers.length - subArray.length); console.log(`Last index of subarray [2, 5] is ${lastIndexOfSubArray}`);
🎉 Conclusion
The lastIndexOf()
method is a valuable addition to your array manipulation toolkit in JavaScript, especially when you need to pinpoint the last occurrence of a specific element.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the lastIndexOf()
method in your JavaScript projects.
👨💻 Join our Community:
Author
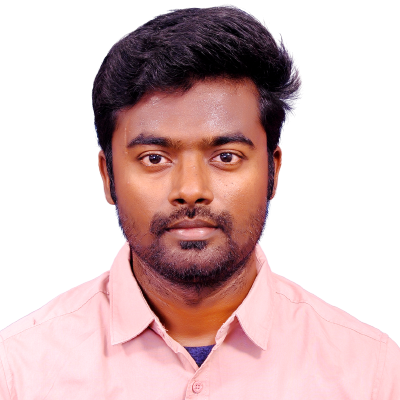
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array lastIndexOf() Method), please comment here. I will help you immediately.