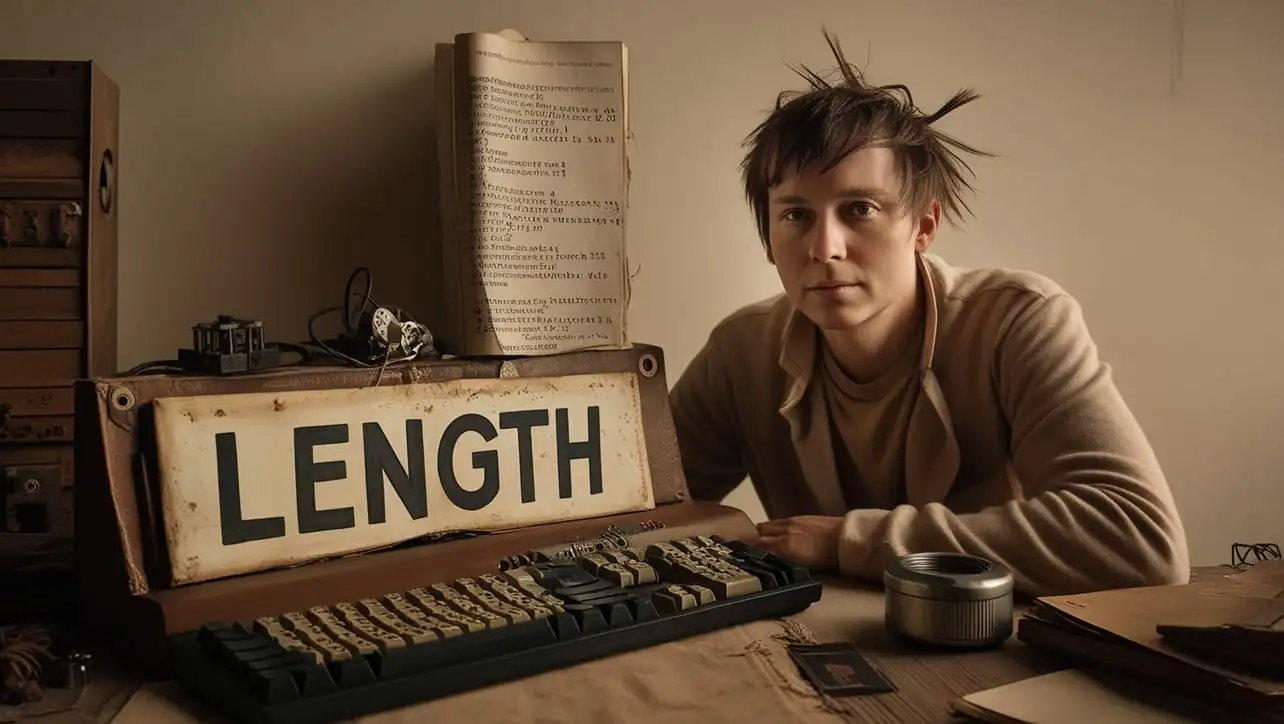
JS Array Methods
JavaScript Array join() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a myriad of methods to manipulate and transform data efficiently. Among these, the join()
method stands out as a powerful tool for creating strings by concatenating array elements.
In this comprehensive guide, we'll delve into the join()
method, exploring its syntax, practical examples, best practices, and various use cases.
🧠 Understanding join() Method
The join()
method in JavaScript arrays is designed to concatenate all the elements of an array into a single string. It allows you to specify a separator that will be inserted between each pair of adjacent elements. Let's dive into the syntax to understand how it works:
💡 Syntax
The syntax for the join()
method is straightforward:
array.join(separator);
- array: The array whose elements you want to join into a string.
- separator (Optional): The string used to separate the array elements. If not specified, a comma is used by default.
📝 Example
Let's explore a simple example to illustrate the basic usage of the join()
method:
// Sample array
const colors = ['red', 'green', 'blue'];
// Using join() to concatenate array elements
const resultString = colors.join('-');
console.log(resultString); // Output: 'red-green-blue'
In this example, the join('-') call concatenates the elements of the colors array using a hyphen as the separator.
🏆 Best Practices
When working with the join()
method, consider the following best practices:
Separator Choice:
Choose a separator that suits your specific use case. It could be a single character, multiple characters, or even an empty string if no separation is desired.
example.jsCopiedconst items = ['apple', 'orange', 'banana']; // Using a space as separator const spaceSeparated = items.join(' '); console.log(spaceSeparated); // Output: 'apple orange banana' // Using an empty string as separator const noSeparator = items.join(''); console.log(noSeparator); // Output: 'appleorangebanana'
Array of Numbers:
When dealing with an array of numbers, convert them to strings before using
join()
to avoid unexpected results.example.jsCopiedconst numbers = [1, 2, 3, 4]; // Incorrect: Results in '1,2,3,4' const incorrectResult = numbers.join(','); // Correct: Results in '1,2,3,4' const correctResult = numbers.map(String).join(',');
📚 Use Cases
Creating URL Parameters:
The
join()
method is handy when constructing URL parameters from an array:example.jsCopiedconst queryParams = ['page=1', 'limit=10', 'sort=desc']; const queryString = queryParams.join('&'); const finalUrl = `https://example.com/data?${queryString}`; console.log(finalUrl); // Output: 'https://example.com/data?page=1&limit=10&sort=desc'
Displaying a List:
Displaying a list from an array becomes straightforward using
join()
:example.jsCopiedconst shoppingList = ['Milk', 'Bread', 'Eggs']; const formattedList = `Shopping List: ${shoppingList.join(', ')}`; console.log(formattedList); // Output: 'Shopping List: Milk, Bread, Eggs'
🎉 Conclusion
The join()
method is a powerful asset in your JavaScript toolkit for efficiently concatenating array elements into a string.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the join()
method in your JavaScript projects.
👨💻 Join our Community:
Author
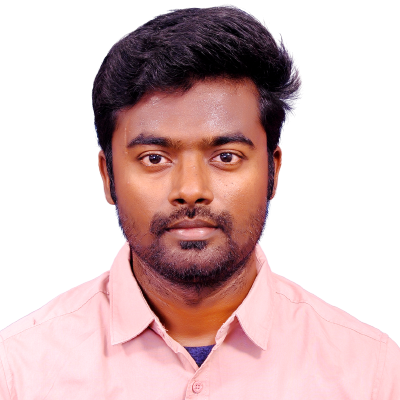
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array join() Method), please comment here. I will help you immediately.