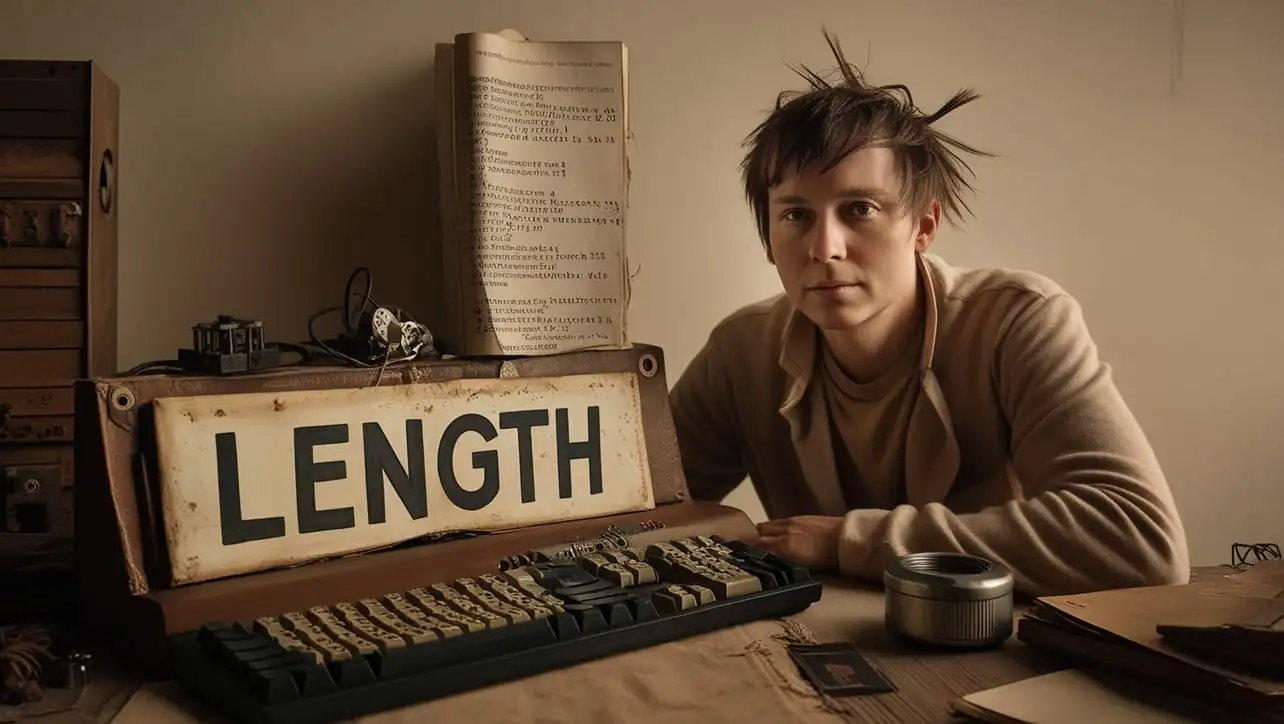
JS Array Methods
JavaScript Array includes() Method
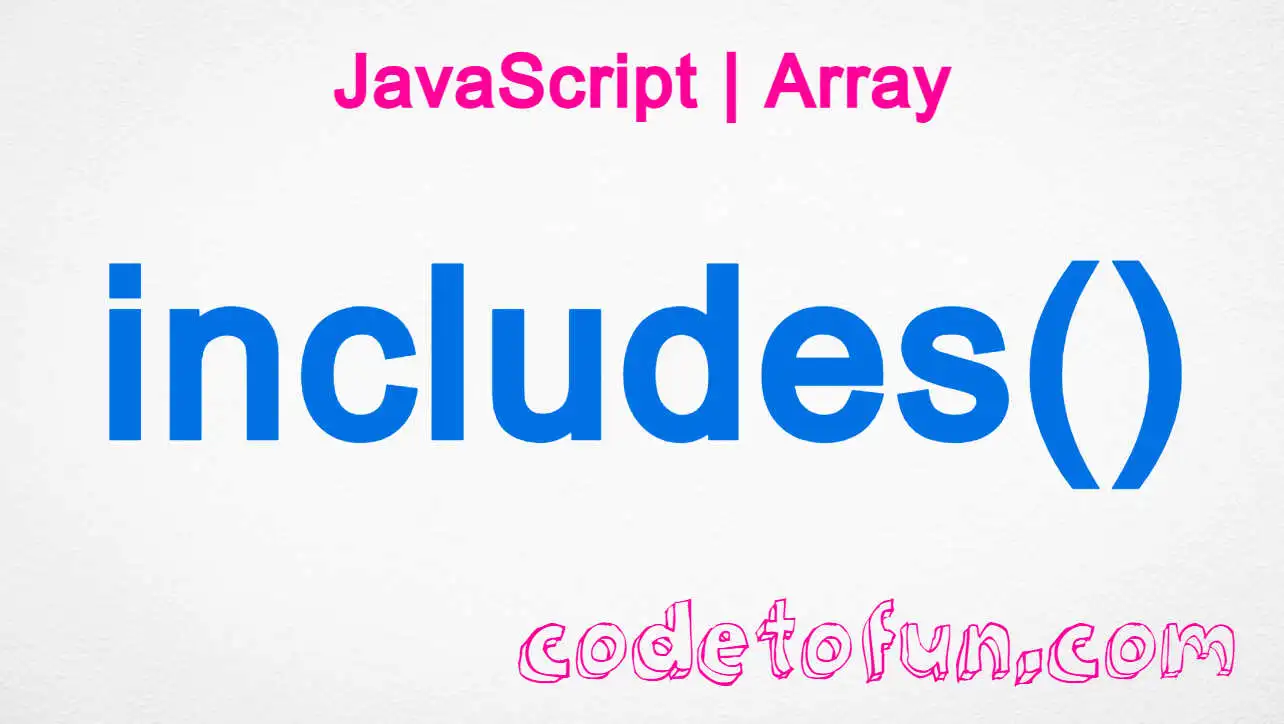
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer powerful features for managing collections of data, and the includes()
method is a handy addition that simplifies the process of checking for the presence of a specific element within an array.
In this guide, we'll explore the includes()
method, covering its syntax, usage, best practices, and practical applications.
🧠 Understanding includes() Method
The includes()
method is designed to determine whether an array includes a particular value, returning true if the value is found and false if it is not. This method provides a straightforward way to perform inclusion checks without the need for extensive manual iterations.
💡 Syntax
The syntax for the includes()
method is straightforward:
array.includes(searchElement[, fromIndex]);
- array: The array to search for the specified element.
- searchElement: The element to search for within the array.
- fromIndex (Optional): The index to start the search from. If not specified, the search begins from the first element.
📝 Example
Let's delve into a simple example to illustrate the usage of the includes()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5];
// Using includes() to check for the presence of a value
const hasThree = numbers.includes(3);
const hasTen = numbers.includes(10);
console.log(hasThree); // Output: true
console.log(hasTen); // Output: false
In this example, we utilize the includes()
method to check if the array contains the values 3 and 10.
🏆 Best Practices
When working with the includes()
method, consider the following best practices:
Strict Equality:
Keep in mind that
includes()
uses strict equality (===) for comparisons. Be aware of potential type mismatches when searching for elements.example.jsCopiedconst mixedArray = [1, '2', true]; // Using includes() with strict equality const hasStringTwo = mixedArray.includes('2'); console.log(hasStringTwo); // Output: false (type mismatch)
Case-Sensitive Search:
For string arrays, remember that
includes()
performs a case-sensitive search. If case-insensitive matching is needed, consider converting elements to a consistent case before using the method.example.jsCopiedconst fruits = ['apple', 'Orange', 'banana']; // Using includes() for case-sensitive search const hasOrange = fruits.includes('Orange'); console.log(hasOrange); // Output: false (case-sensitive)
Compatibility Checking:
Verify the compatibility of the
includes()
method, especially if supporting older browsers or environments.example.jsCopied// Check if the includes() method is supported if (Array.prototype.includes) { // Use the method safely const hasValue = numbers.includes(3); console.log(hasValue); } else { console.error('includes() method not supported in this environment.'); }
📚 Use Cases
Filtering Arrays:
The
includes()
method is useful for filtering arrays based on the presence of specific elements:example.jsCopiedconst evenNumbers = numbers.filter(number => number % 2 === 0); console.log(evenNumbers); // Output: [2, 4]
Conditional Logic:
The method can be employed in conditional logic to determine whether certain actions should be taken:
example.jsCopiedif (numbers.includes(5)) { console.log('The array contains the value 5.'); } else { console.log('The array does not contain the value 5.'); }
🎉 Conclusion
The includes()
method is a valuable addition to the array toolkit in JavaScript, providing a concise and efficient way to check for the presence of specific elements.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the includes()
method in your JavaScript projects.
👨💻 Join our Community:
Author
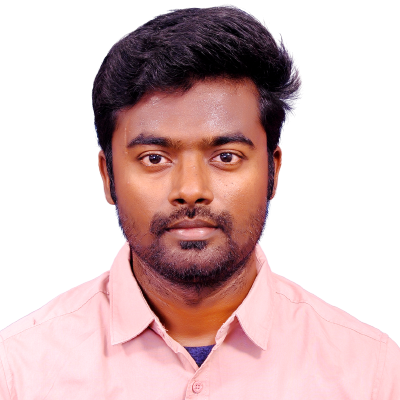
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array includes() Method), please comment here. I will help you immediately.