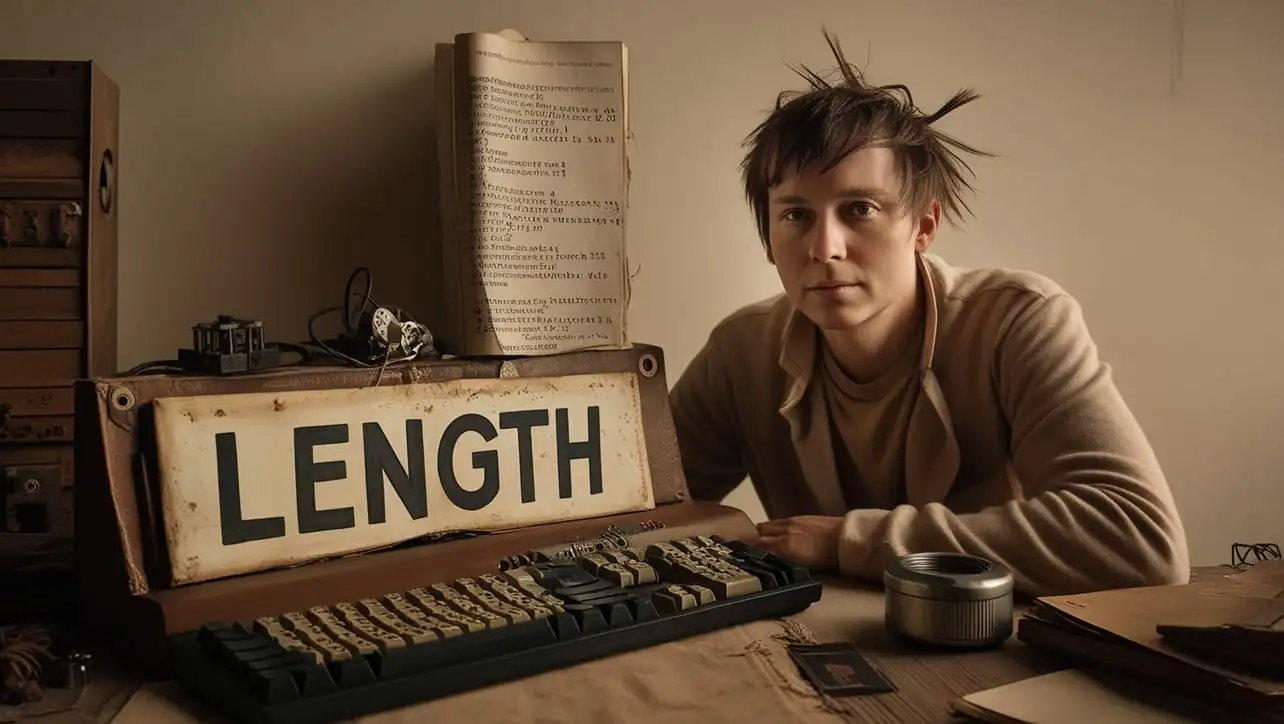
JS Array Methods
JavaScript Array findLastIndex() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a plethora of methods for efficient data manipulation, and the findLastIndex()
method is a valuable addition. This method allows you to find the index of the last element in an array that satisfies a given condition.
In this guide, we'll delve into the findLastIndex()
method, exploring its syntax, usage, best practices, and practical examples.
🧠 Understanding findLastIndex() Method
The findLastIndex()
method searches for an element in the array that satisfies the provided testing function. It executes the function once for each element, starting from the last array element, and returns the index of the last element that passes the test. If no elements pass the test, it returns -1.
💡 Syntax
The syntax for the findLastIndex()
method is straightforward:
array.findLastIndex(callback(element, index, array));
- array: The array to search through.
- callback: A function to execute on each element, taking three arguments:
- element: The current element being processed.
- index: The index of the current element.
- array: The array findLastIndex was called upon.
📝 Example
Let's dive into an example to illustrate the usage of the findLastIndex()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5, 2];
// Using findLastIndex() to find the index of the last even number
const lastIndex = numbers.findLastIndex(element => element % 2 === 0);
console.log(`Index of the last even number: ${lastIndex}`);
In this example, the findLastIndex()
method is employed to find the index of the last even number in the numbers array.
🏆 Best Practices
When working with the findLastIndex()
method, consider the following best practices:
Callback Function:
Ensure that the callback function returns a boolean value, indicating the condition to be met.
example.jsCopiedconst lastIndex = numbers.findLastIndex(element => { // Correct: Return true if the condition is met return element > 3; // Incorrect: Returning the element itself // return element; });
Default Value:
If there's a possibility that the condition might not be met, handle the case where
findLastIndex()
returns -1.example.jsCopiedconst lastIndex = numbers.findLastIndex(element => element > 10); if (lastIndex !== -1) { console.log(`Index of element > 10: ${lastIndex}`); } else { console.log('No element found that satisfies the condition.'); }
Compatibility Checking:
Verify the compatibility of the
findLastIndex()
method in your target environments, especially if supporting older browsers.example.jsCopied// Check if the findLastIndex() method is supported if (Array.prototype.findLastIndex) { // Use the method safely const lastIndex = numbers.findLastIndex(/* callback function */); console.log(`Index found: ${lastIndex}`); } else { console.error('findLastIndex() method not supported in this environment.'); }
📚 Use Cases
Filtering Unique Values:
The
findLastIndex()
method can be utilized to filter out unique values from an array:example.jsCopiedconst uniqueNumbers = numbers.filter((element, index, arr) => { return arr.findLastIndex(el => el === element) === index; }); console.log('Array with unique numbers:', uniqueNumbers);
Locating the Last Occurrence:
Use
findLastIndex()
to locate the index of the last occurrence of a specific element:example.jsCopiedconst targetNumber = 2; const lastOccurrenceIndex = numbers.findLastIndex(element => element === targetNumber); console.log(`Index of the last occurrence of ${targetNumber}: ${lastOccurrenceIndex}`);
🎉 Conclusion
The findLastIndex()
method is a powerful tool for searching arrays and finding the index of the last element that satisfies a given condition.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the findLastIndex()
method in your JavaScript projects.
👨💻 Join our Community:
Author
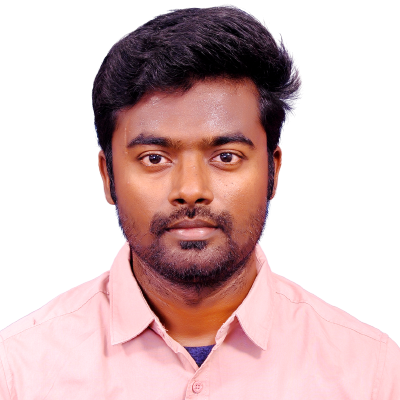
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array findLastIndex() Method), please comment here. I will help you immediately.