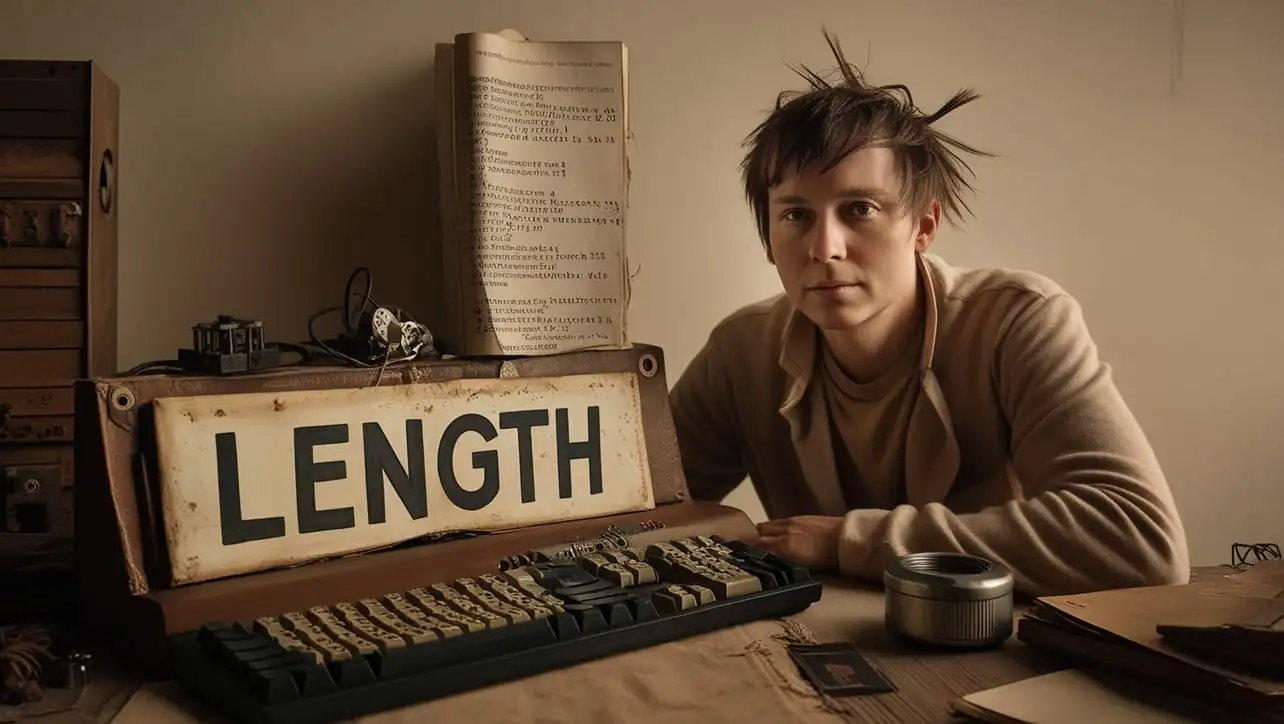
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array findLast() Method
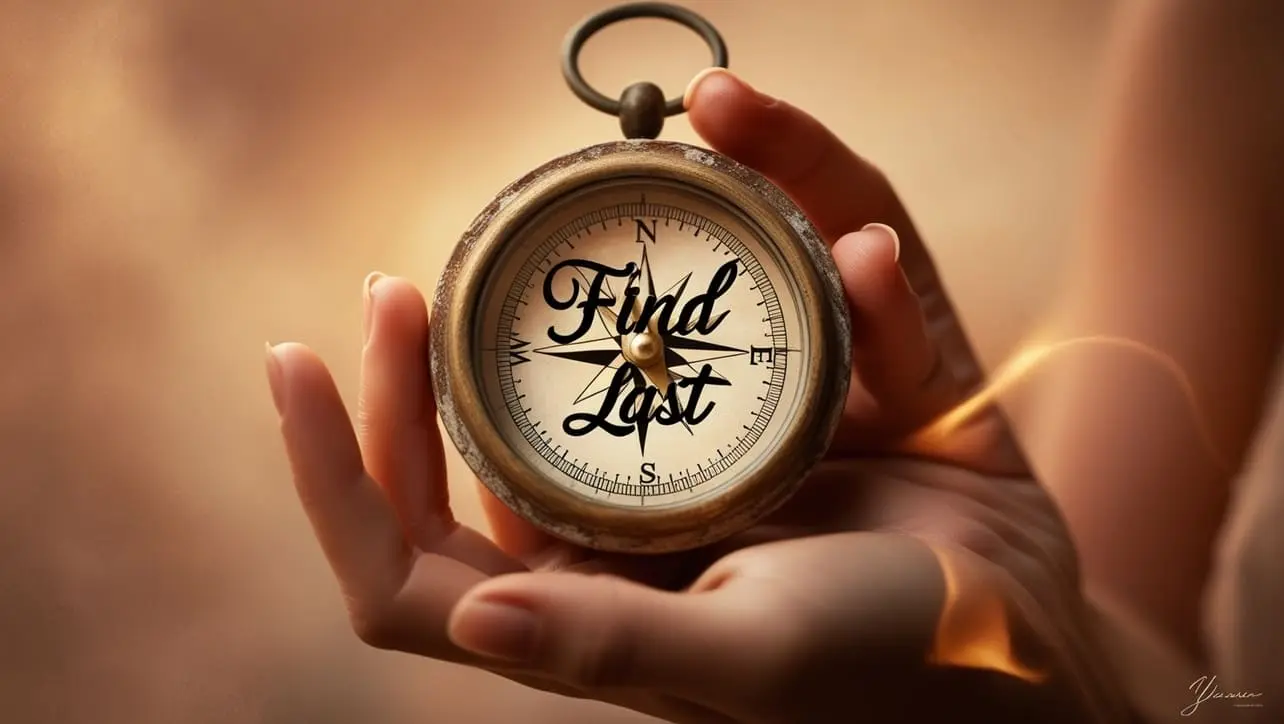
Photo Credit to CodeToFun
Introduction
JavaScript arrays provide a myriad of methods to simplify data manipulation, and the findLast()
method is a valuable addition when it comes to searching for elements. This method allows you to locate the last element in an array that satisfies a given condition.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical use cases of the findLast()
method.
Understanding findLast() Method
The findLast()
method is specifically designed to find the last element in an array that meets the criteria specified by a provided testing function. This function is called for each element, and the first element that satisfies the condition is returned.
Syntax
The syntax for the findLast()
method is straightforward:
array.findLast(callback(element, index, array), thisArg);
- array: The array in which you want to search for the last element.
- callback: A function that is called for each element in the array. It takes three arguments: element (the current element being processed), index (the index of the current element), and array (the array being traversed).
- thisArg (optional): An object to which the this keyword can refer in the callback function. If omitted, this will refer to the global object.
Example
Let's dive into a practical example to illustrate the usage of the findLast()
method:
// Sample array of numbers
const numbers = [10, 20, 30, 40, 50];
// Using findLast() to locate the last element greater than 30
const result = numbers.findLast((element) => element > 30);
console.log(result); // Output: 50
In this example, the findLast()
method is employed to locate the last element in the numbers array that is greater than 30.
Best Practices
When working with the findLast()
method, consider the following best practices:
Callback Function Efficiency:
Ensure that the callback function is efficient, as it is executed for each element until a match is found.
example.jsCopiedconst targetValue = 42; // Inefficient callback function const inefficientFind = numbers.findLast((element) => { console.log(`Checking element: ${element}`); return element === targetValue; }); console.log(inefficientFind); // Output: 50
Default Value or Error Handling:
If there is a possibility that no element satisfies the condition, consider providing a default value or implementing error handling.
example.jsCopiedconst targetValue = 60; // Using a default value if no match is found const findWithDefault = numbers.findLast((element) => element > targetValue) || 'No match found'; console.log(findWithDefault); // Output: 'No match found'
Compatibility Checking:
Verify the compatibility of the
findLast()
method in your target environments, especially if you need to support older browsers.example.jsCopied// Check if the findLast() method is supported if (Array.prototype.findLast) { // Use the method safely const result = numbers.findLast((element) => element > 30); console.log(result); } else { console.error('findLast() method not supported in this environment.'); }
Use Cases
Filtering Unique Values:
The
findLast()
method is useful when filtering an array for unique values based on a specific condition:example.jsCopiedconst uniqueNumbers = [1, 2, 3, 4, 5, 2, 4, 6, 8, 10]; const lastUnique = uniqueNumbers.findLast((value, index, array) => array.lastIndexOf(value) === index); console.log(lastUnique); // Output: 10
Locating the Last Updated Item:
In scenarios where an array represents items with timestamps,
findLast()
can be utilized to locate the most recently updated item:example.jsCopiedconst items = [ { id: 1, name: 'Item A', timestamp: 1635900000000 }, { id: 2, name: 'Item B', timestamp: 1635910000000 }, { id: 3, name: 'Item C', timestamp: 1635920000000 }, ]; const lastUpdatedItem = items.findLast((item) => item.timestamp === Math.max(...items.map((i) => i.timestamp))); console.log(lastUpdatedItem); // Output: { id: 3, name: 'Item C', timestamp: 1635920000000 }
Conclusion
The findLast()
method enhances the array searching capabilities in JavaScript, providing a straightforward way to locate the last element that satisfies a given condition.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the findLast()
method in your JavaScript projects.
Join our Community:
Author
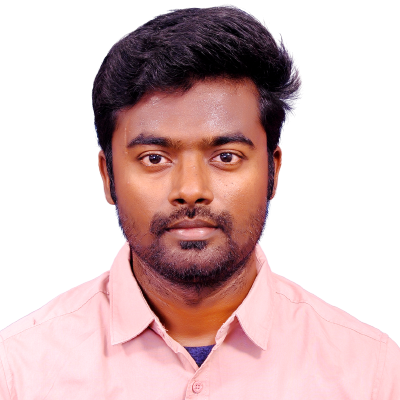
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array findLast() Method), please comment here. I will help you immediately.