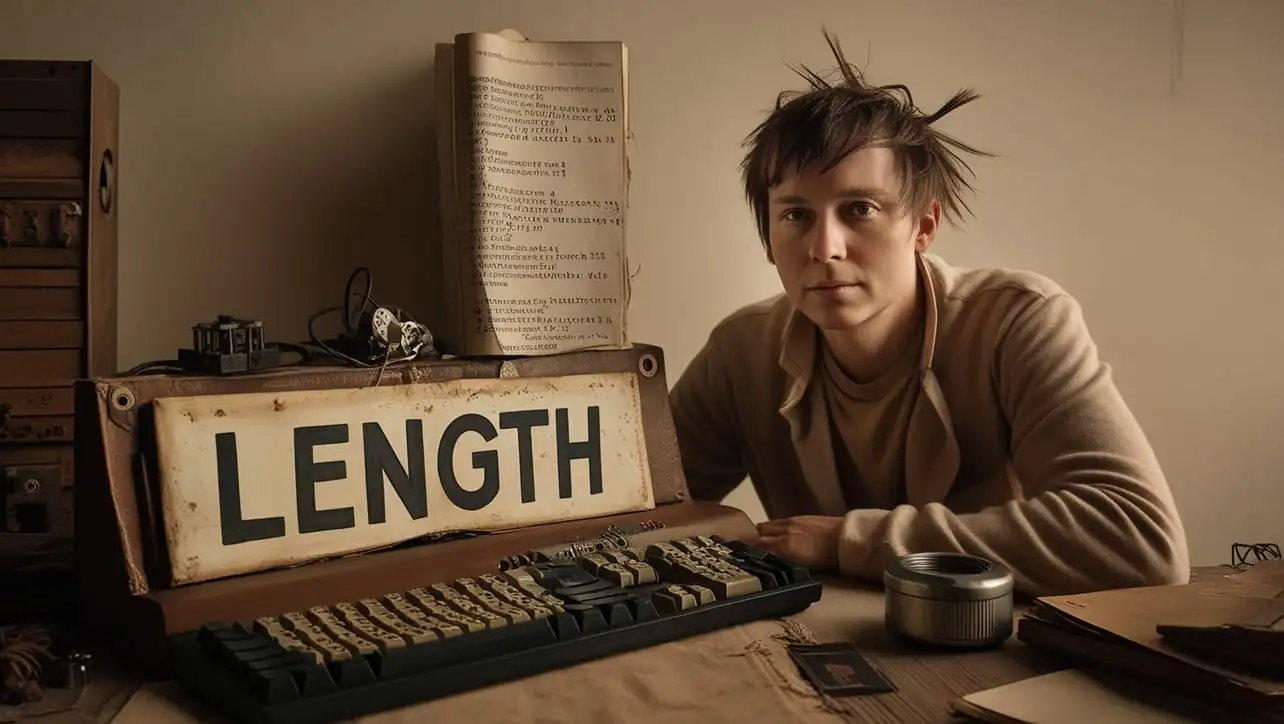
JS Array Methods
JavaScript Array find() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer powerful tools for data manipulation, and the find()
method is a valuable addition to your arsenal. This method empowers you to search for a specific element in an array based on a given condition.
In this guide, we'll explore the find()
method, understand its syntax, delve into best practices, and explore practical use cases.
🧠 Understanding find() Method
The find()
method in JavaScript arrays is designed to locate the first element that satisfies a specified testing function. It stops the search as soon as an element is found, providing a convenient way to retrieve a single item from an array that meets a particular criterion.
💡 Syntax
The syntax for the find()
method is straightforward:
array.find(callback(element, index, array), thisArg);
- array: The array on which the
find()
method is called. - callback: A function that will be called for each element in the array until a match is found. It takes three arguments: element (the current array element), index (the index of the current element), and array (the array being traversed).
- thisArg (Optional): An object to which this will be bound inside the callback function.
📝 Example
Let's illustrate the usage of the find()
method with a simple example:
// Sample array of numbers
const numbers = [10, 25, 5, 40, 15];
// Using find() to locate the first element greater than 20
const result = numbers.find(element => element > 20);
console.log(result); // Output: 25
In this example, the find()
method is employed to locate the first element in the array that is greater than 20.
🏆 Best Practices
When working with the find()
method, consider the following best practices:
Check for undefined:
Since
find()
returns undefined if no element satisfies the condition, ensure to handle this case in your code.example.jsCopiedconst result = numbers.find(element => element > 50); if (result !== undefined) { console.log(result); } else { console.log('No element found.'); }
Use Arrow Functions for Conciseness:
Arrow functions can provide a concise syntax, especially for simple conditions.
example.jsCopiedconst targetNumber = 5; const foundNumber = numbers.find(element => element === targetNumber); console.log(foundNumber);
Ensure Compatibility:
Confirm that the
find()
method is supported in your target environments, especially when dealing with older browsers.example.jsCopied// Check if the find() method is supported if (Array.prototype.find) { // Use the method safely const result = numbers.find(element => element > 20); console.log(result); } else { console.error('find() method not supported in this environment.'); }
📚 Use Cases
Filtering User Data:
Imagine an array of user objects, and you want to find the user with a specific username:
example.jsCopiedconst users = [ { id: 1, username: 'john_doe' }, { id: 2, username: 'jane_smith' }, { id: 3, username: 'bob_jones' } ]; const targetUsername = 'jane_smith'; const foundUser = users.find(user => user.username === targetUsername); console.log(foundUser);
Locating an Object by Property:
You might have an array of objects and need to find an object based on a specific property:
example.jsCopiedconst products = [ { id: 101, name: 'Laptop', price: 1200 }, { id: 102, name: 'Smartphone', price: 800 }, { id: 103, name: 'Tablet', price: 400 } ]; const targetProductID = 102; const foundProduct = products.find(product => product.id === targetProductID); console.log(foundProduct);
🎉 Conclusion
The find()
method is a powerful tool for array manipulation in JavaScript, offering a concise way to locate elements that match specific conditions.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the find()
method in your JavaScript projects.
👨💻 Join our Community:
Author
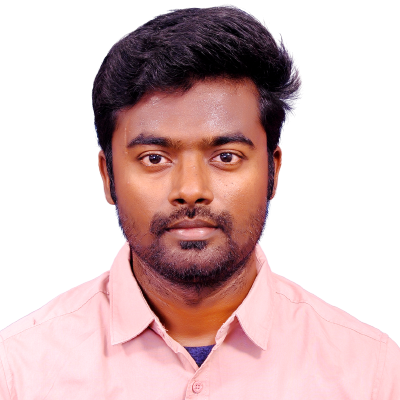
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array find() Method), please comment here. I will help you immediately.