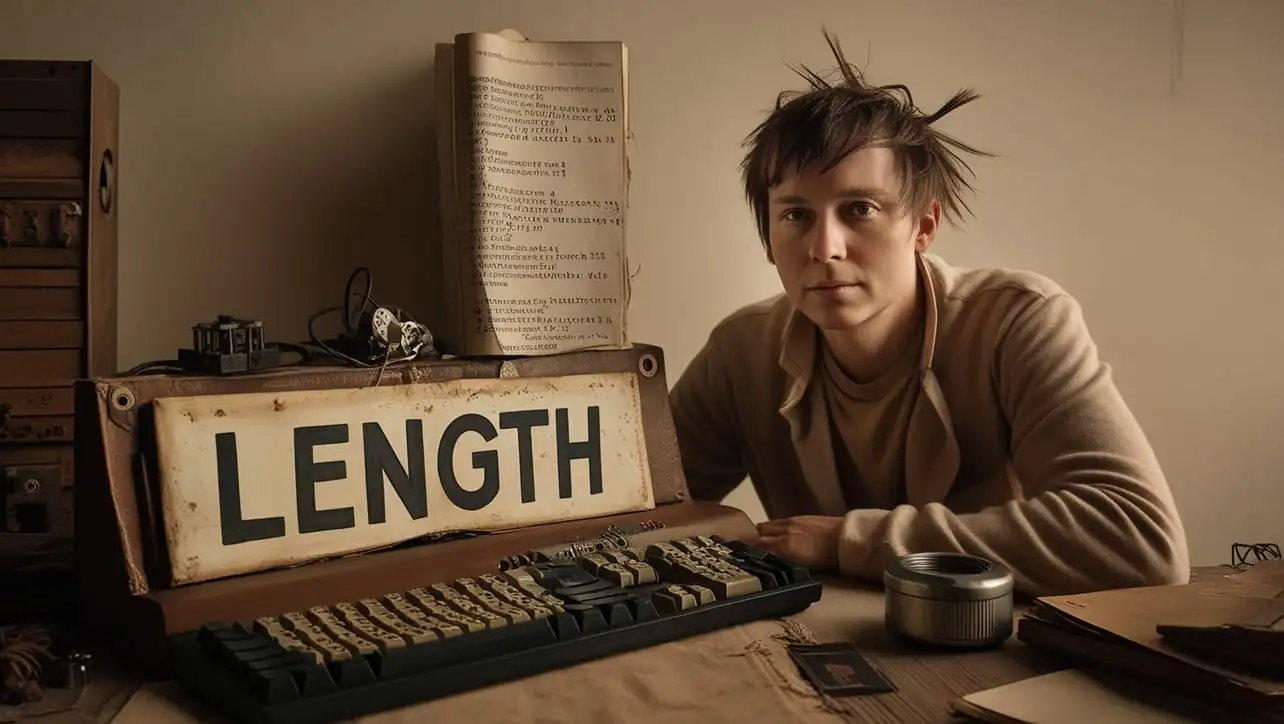
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array every() Method
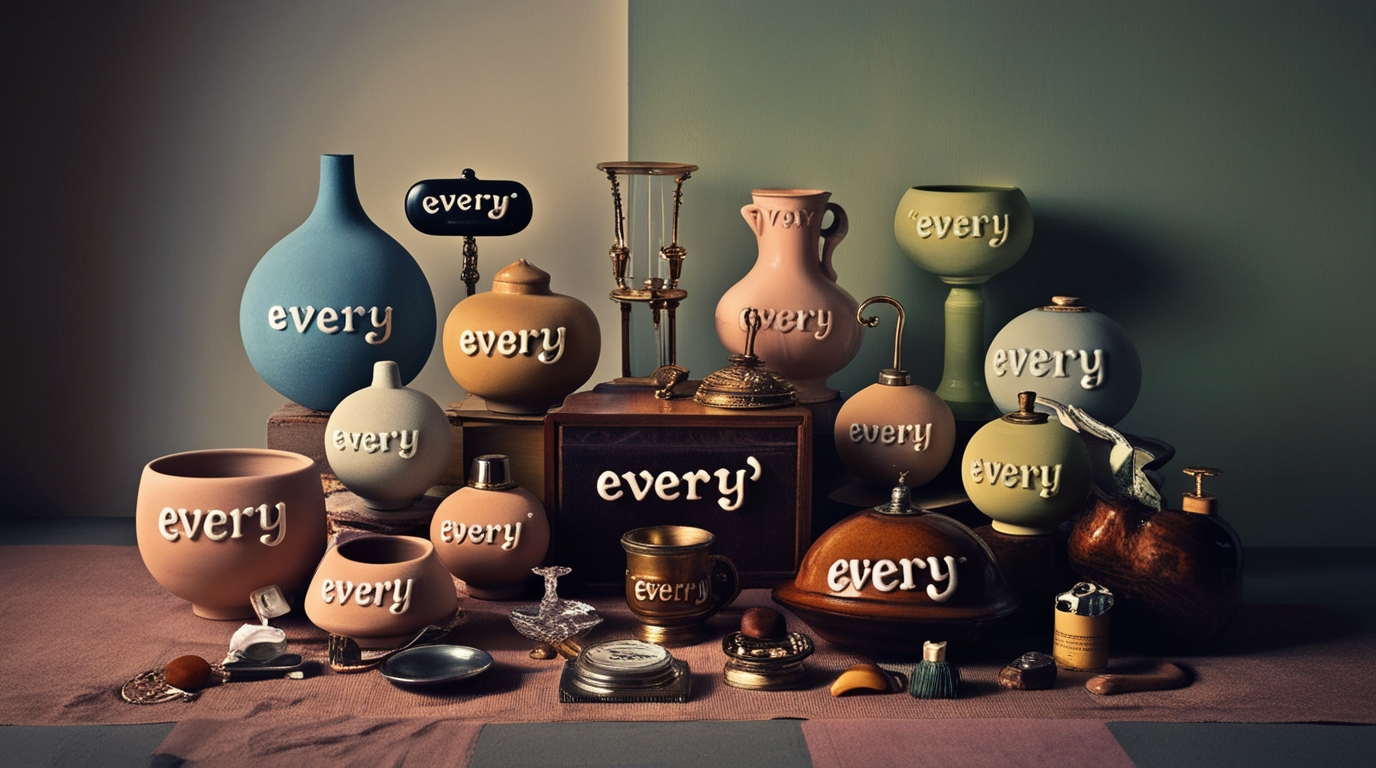
Photo Credit to CodeToFun
Introduction
Arrays in JavaScript offer a myriad of powerful methods for efficient data manipulation, and the every()
method is no exception. This method provides a succinct way to check whether all elements in an array satisfy a given condition.
In this guide, we'll explore the every()
method, examining its syntax, example usage, best practices, and practical applications.
Understanding every() Method
The every()
method tests whether all elements in an array pass the provided function. It returns a boolean valueβtrue if all elements meet the condition, and false otherwise.
Syntax
The syntax for the every()
method is straightforward:
array.every(callback(element, index, array), thisArg);
- array: The array to test against.
- callback: A function to test each element. It takes three arguments: element, index, and array.
- thisArg (optional): An object to use as this when executing the callback.
Example
Let's dive into a straightforward example to illustrate the usage of the every()
method:
// Sample array
const numbers = [2, 4, 6, 8, 10];
// Using every() to check if all elements are even
const allEven = numbers.every((num) => num % 2 === 0);
console.log(allEven); // Output: true
In this example, the every()
method is employed to check if all elements in the numbers array are even.
Best Practices
When working with the every()
method, consider the following best practices:
Callback Function:
Design a clear and concise callback function that accurately represents the condition you are checking.
example.jsCopied// Check if all elements are greater than 10 const allGreaterThanTen = numbers.every((num) => num > 10); console.log(allGreaterThanTen); // Output: false
Empty Arrays:
Be aware that an empty array will always return true when using
every()
. If the array is empty, the condition is trivially satisfied.example.jsCopiedconst emptyArray = []; const result = emptyArray.every((elem) => elem > 0); console.log(result); // Output: true
Use Cases
Validating User Input:
The
every()
method can be useful when validating user input against a set of conditions:example.jsCopiedconst userInput = ['John', 'Doe', 25, 'john.doe@example.com']; const isValidInput = userInput.every((input) => typeof input === 'string'); console.log(isValidInput); // Output: false
In this example, we use every() to check if all elements in the userInput array are of type string.
Checking Array of Objects:
When dealing with an array of objects,
every()
is handy for verifying a property across all objects:example.jsCopiedconst students = [ { name: 'Alice', age: 22 }, { name: 'Bob', age: 25 }, { name: 'Charlie', age: 20 }, ]; const allAdults = students.every((student) => student.age >= 18); console.log(allAdults); // Output: true
Here,
every()
checks if all students are adults based on the age property.
Conclusion
The every()
method is a powerful tool for array manipulation in JavaScript, offering a concise way to verify if all elements meet a specified condition.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the every()
method in your JavaScript projects.
Join our Community:
Author
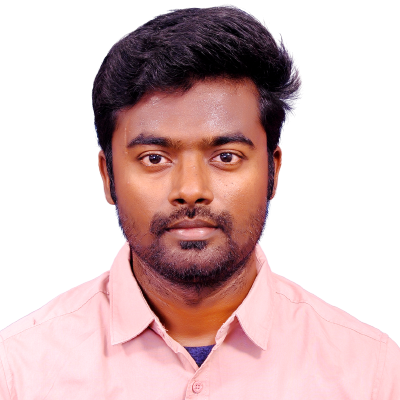
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array every() Method), please comment here. I will help you immediately.