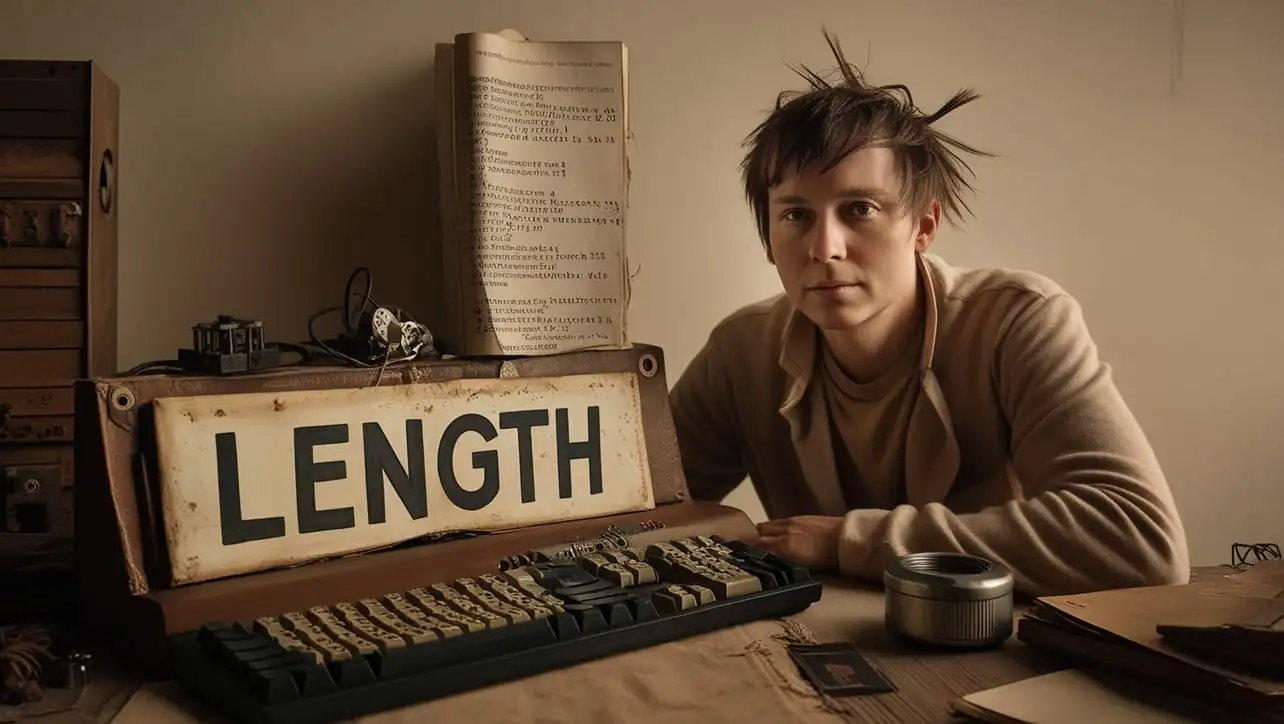
JS Array Methods
JavaScript Array entries() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays provide a robust mechanism for handling collections of data, and the entries()
method adds another layer of versatility. This method allows you to iterate through the key-value pairs of an array effortlessly.
In this guide, we'll delve into the entries()
method, examining its syntax, usage, best practices, and practical use cases.
🧠 Understanding entries() Method
The entries()
method returns a new Array Iterator object that contains key-value pairs for each index in the array. This Iterator can be used to loop through array elements, obtaining both the index and the corresponding value.
💡 Syntax
The syntax for the entries()
method is straightforward:
array.entries();
- array: The array for which you want to retrieve the key-value pairs.
📝 Example
Let's dive into a simple example to illustrate the usage of the entries()
method:
// Sample array
const colors = ['red', 'green', 'blue'];
// Using entries() to iterate through key-value pairs
const iterator = colors.entries();
for (const entry of iterator) {
console.log(entry);
}
In this example, the entries()
method is used to obtain an iterator, and a for...of loop is employed to iterate through the key-value pairs.
🏆 Best Practices
When working with the entries()
method, consider the following best practices:
Destructuring:
Utilize array destructuring to conveniently separate the index and value during iteration.
example.jsCopiedfor (const [index, value] of colors.entries()) { console.log(`Index: ${index}, Value: ${value}`); }
Compatibility Checking:
Verify the compatibility of the
entries()
method in your target environments, especially if you need to support older browsers.example.jsCopied// Check if the entries() method is supported if (Array.prototype.entries) { // Use the method safely const iterator = colors.entries(); for (const entry of iterator) { console.log(entry); } } else { console.error('entries() method not supported in this environment.'); }
📚 Use Cases
Enumerating Array Elements:
The primary use case for the
entries()
method is to enumerate through the key-value pairs of an array:example.jsCopiedfor (const [index, value] of colors.entries()) { console.log(`Color at index ${index}: ${value}`); }
Finding the First Occurrence:
The
entries()
method can be handy when searching for the index of the first occurrence of a specific value:example.jsCopiedconst targetColor = 'green'; for (const [index, value] of colors.entries()) { if (value === targetColor) { console.log(`Found ${targetColor} at index ${index}`); break; } }
🎉 Conclusion
The entries()
method enriches the array manipulation capabilities in JavaScript, providing an efficient way to iterate through key-value pairs.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the entries()
method in your JavaScript projects.
👨💻 Join our Community:
Author
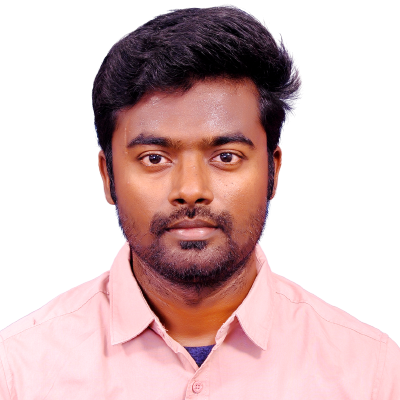
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array entries() Method), please comment here. I will help you immediately.