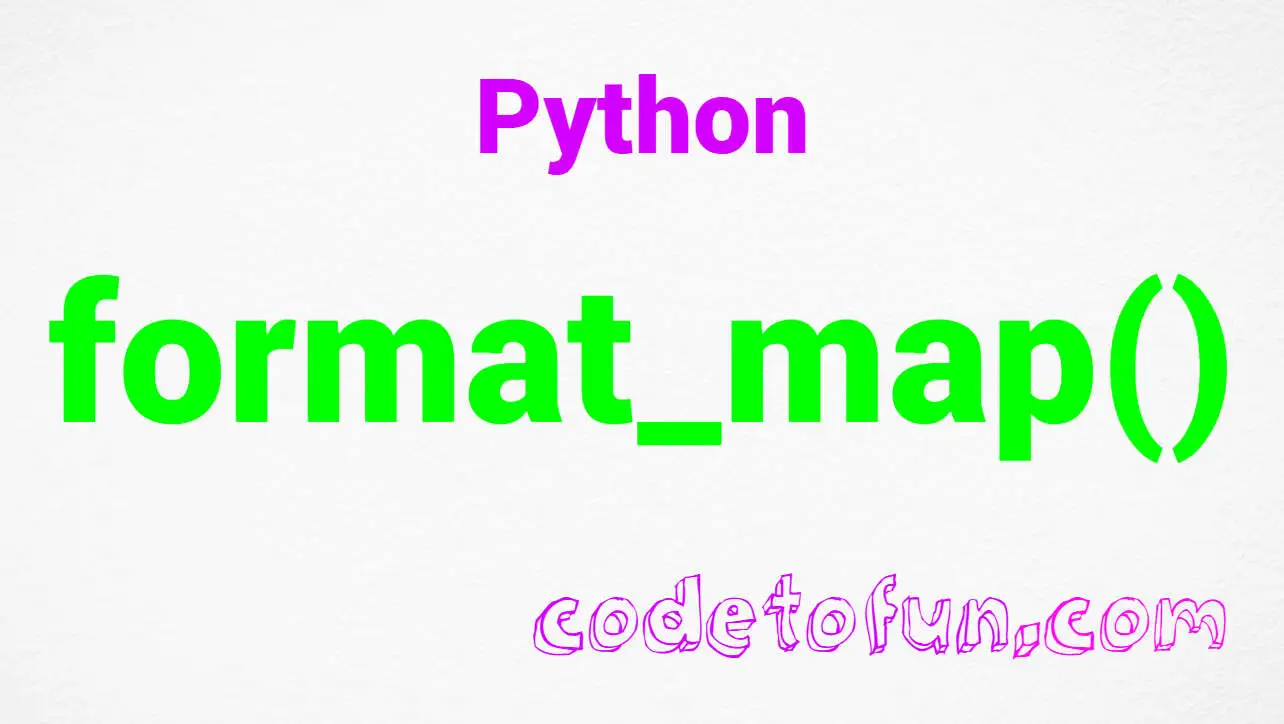
Python Basic
Python String Methods
Python string count() Method
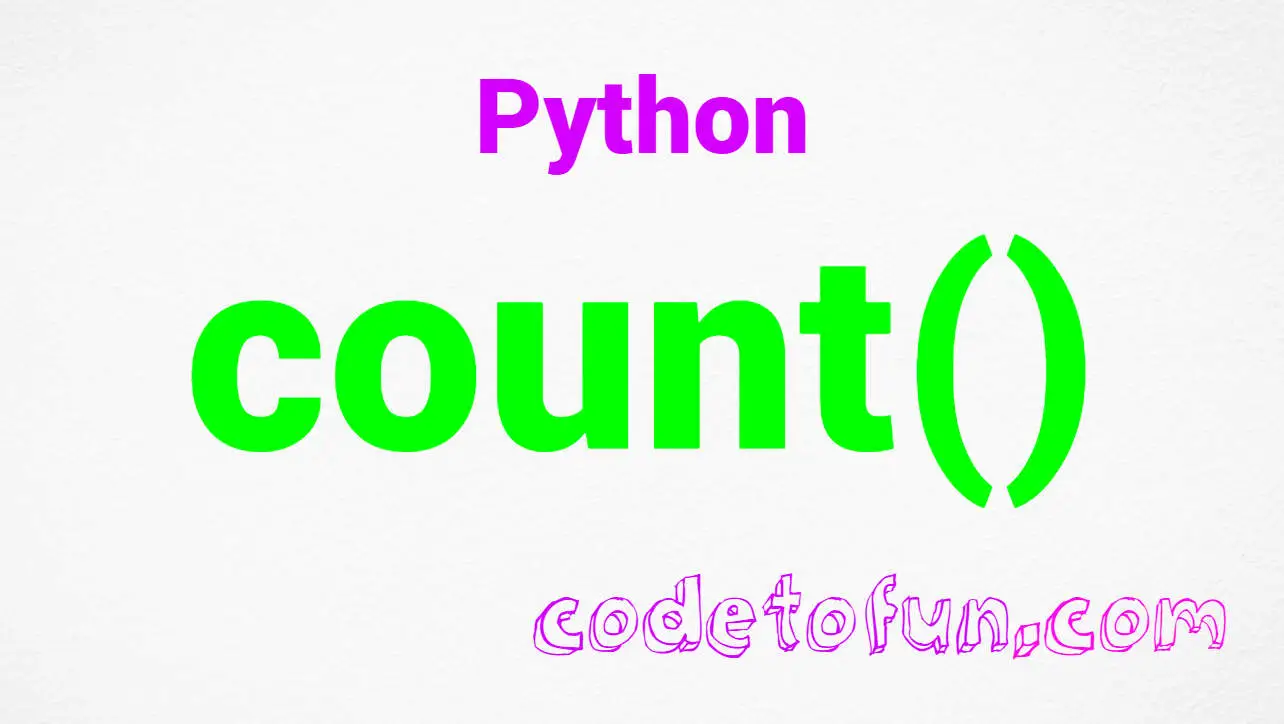
Photo Credit to CodeToFun
🙋 Introduction
In Python, strings are versatile data types, and Python provides a variety of built-in methods for string manipulation.
The count()
method is one such method that allows you to count the occurrences of a specified substring within a string.
This method is particularly useful when you need to analyze or manipulate text data.
🤓 Understanding the count() Method
The count()
method is a built-in string method in Python that returns the number of occurrences of a specified substring in the given string.
It is case-sensitive, meaning that it distinguishes between uppercase and lowercase characters.
💡 Syntax
The syntax for the count()
method is:
string.count(substring, start, end)
- string: The original string in which you want to count occurrences.
- substring: The substring for which you want to count occurrences.
- start (optional): The starting index from where the search begins (default is 0).
- end (optional): The ending index where the search stops (default is the end of the string).
📄 Example
Let's explore a simple example to understand how the count()
method works:
# Using count() method
original_string = "python is powerful, python is easy, python is fun"
substring = "python"
count_occurrences = original_string.count(substring)
# Displaying the result
print(f"The substring '{substring}' appears {count_occurrences} times.")
💻 Testing the Program
The substring 'python' appears 3 times.
🧠 How the Program Works
In this example, the count()
method is used to count the occurrences of the substring "python" in the original string.
📚 Common Use Cases
Character Occurrence Count:
character-occurrence-count.pyCopiedtext = "programming is interesting" vowel_count = text.count("a") + text.count("e") + text.count("i") + text.count("o") + text.count("u")
Word Frequency Analysis:
word-frequency-analysis.pyCopiedsentence = "This is a simple sentence. Is it simple?" word_count = sentence.count("simple")
Checking Substring Presence:
checking-substring-presence.pyCopieddocument = "Python programming is versatile." if document.count("Java") > 0: print("Java is mentioned in the document.")
📝 Notes
- The
count()
method returns 0 if the substring is not found in the original string. - If
start and
end are provided, the counting is performed within that specified substring.
🎢 Optimization
While the count()
method is efficient for simple substring counting, for more complex patterns and text analysis tasks, consider exploring regular expressions or custom functions.
🎉 Conclusion
The count()
method is a valuable tool when you need to quickly determine the number of occurrences of a specific substring within a string. It is a handy method for various text processing and analysis tasks in Python.
Feel free to incorporate and modify the code examples for your specific use case. Happy coding!
👨💻 Join our Community:
Author
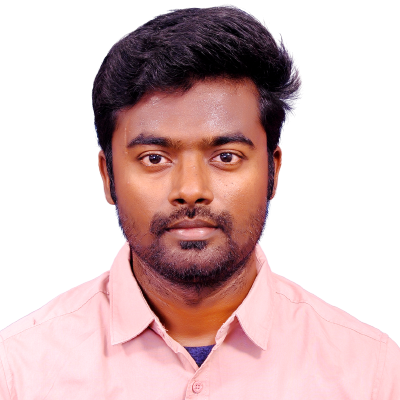
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string count() Method), please comment here. I will help you immediately.