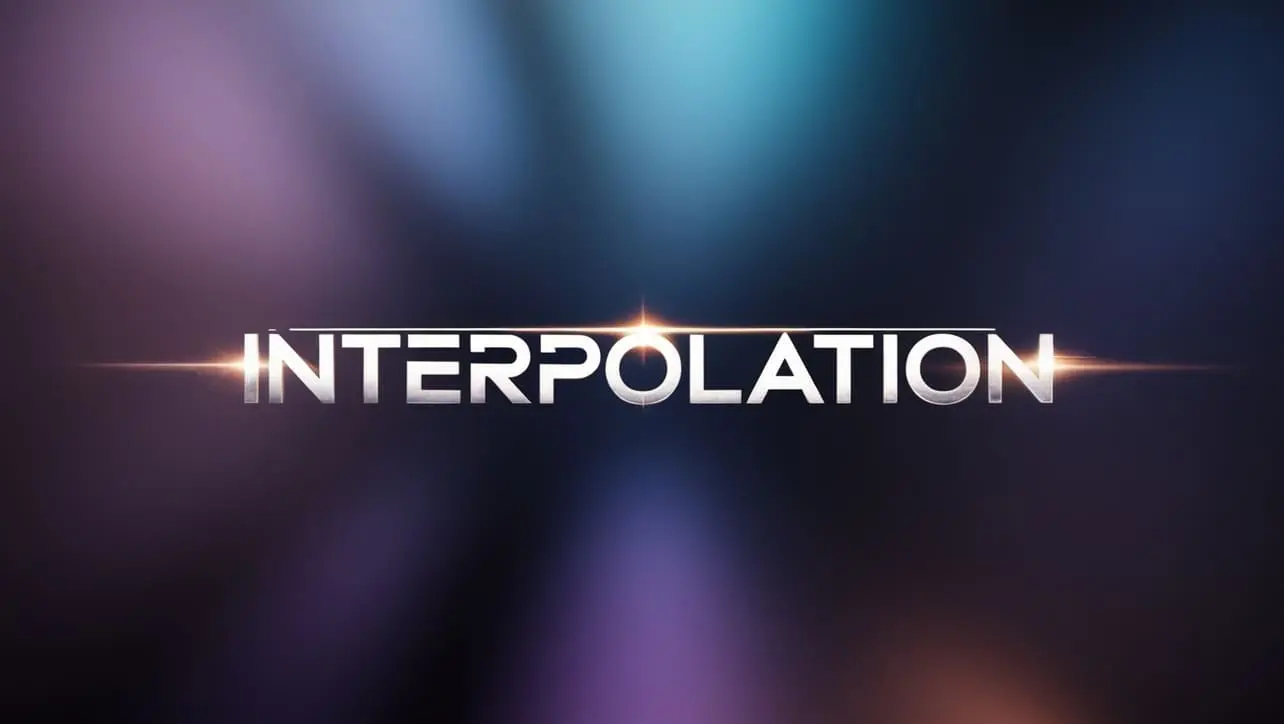
Sass Introspection
Sass get-function() Function
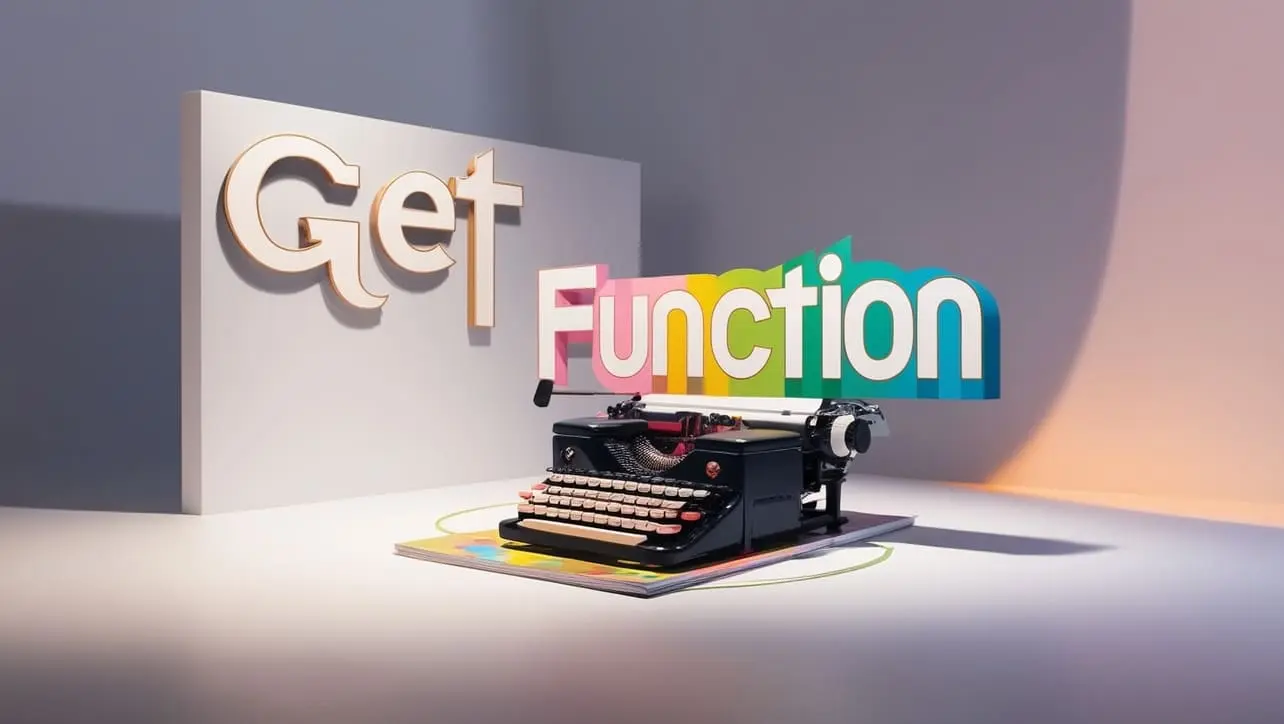
Photo Credit to CodeToFun
π Introduction
The get-function()
function in Sass is used to retrieve a function that has been defined using the @function directive. This allows you to dynamically access and use functions in your Sass code.
It's particularly useful for advanced use cases where you need to call functions based on variable inputs or manage functions in a more modular fashion.
π‘ Syntax
The syntax for get-function()
is simple and requires only one argument:
get-function(name)
π’ Parameters
- name: The name of the function you wish to retrieve. This should be provided as a string.
β©οΈ Return Value
The function returns the reference to the specified function. This reference can then be called like any other function within Sass.
π Example Usage
Letβs look at some practical examples to understand how get-function()
can be used in Sass.
π Example 1: Basic Usage
// Define a function
@function double($value) {
@return $value * 2;
}
// Retrieve the function and use it
$function-name: "double";
$result: call(get-function($function-name), 10);
p {
content: $result;
}
In this example, the double function is defined to multiply a value by 2. We use get-function()
to retrieve the function by name and then use the call() function to invoke it with the argument 10. The result, which is 20, is used in the CSS content.
π Example 2: Dynamic Function Calls
// Define multiple functions
@function add($a, $b) {
@return $a + $b;
}
@function subtract($a, $b) {
@return $a - $b;
}
// Function map
$functions: (
"add": add,
"subtract": subtract
);
// Retrieve and use functions dynamically
$operation: "add";
$result: call(get-function(map-get($functions, $operation)), 5, 3);
p {
content: $result;
}
Here, we define two functions, add and subtract, and store them in a map. We use get-function()
to retrieve the desired function based on the value of $operation and call it with the arguments 5 and 3. The result, 8, is used in the CSS content.
π Example 3: Error Handling
@function multiply($value) {
@return $value * 3;
}
// Attempt to retrieve a non-existent function
$function-name: "nonexistent";
$function: get-function($function-name);
@if $function == null {
@warn "Function #{$function-name} does not exist.";
} @else {
$result: call($function, 5);
.result {
content: $result;
}
}
In this example, we attempt to retrieve a function that does not exist. The get-function()
function will return null if the function is not found, and we use conditional logic to handle the error and provide a warning.
π Conclusion
The get-function()
function in Sass is a powerful feature for advanced Sass programming. It enables dynamic function retrieval and invocation, making your Sass code more flexible and modular. By understanding and utilizing get-function()
, you can manage and utilize functions in more sophisticated ways, enhancing your ability to write clean, reusable, and maintainable code.
Experiment with get-function()
in various scenarios to explore its full potential and see how it can streamline your Sass workflows. Whether you're handling multiple functions dynamically or organizing your code more efficiently, get-function()
offers a valuable tool for your Sass toolkit.
π¨βπ» Join our Community:
Author
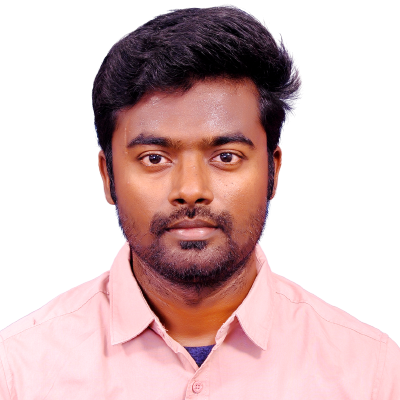
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Sass get-function() Function), please comment here. I will help you immediately.