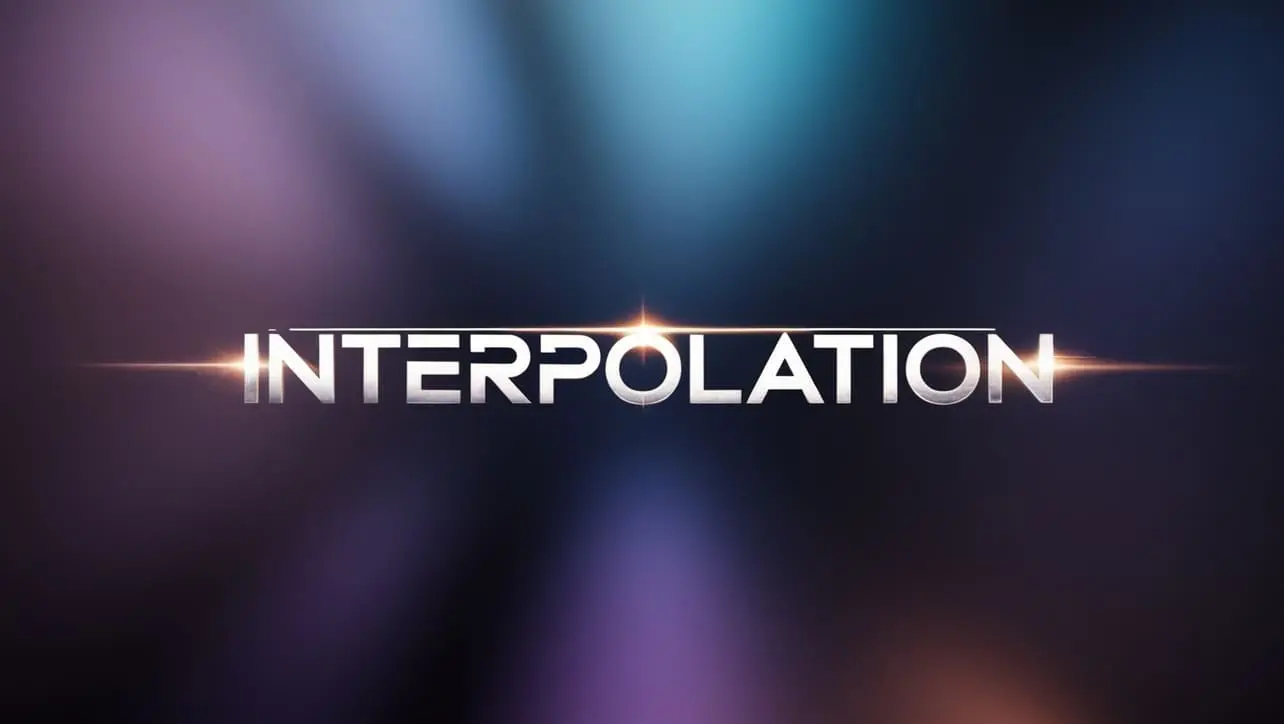
Sass Introspection
Sass call() Function
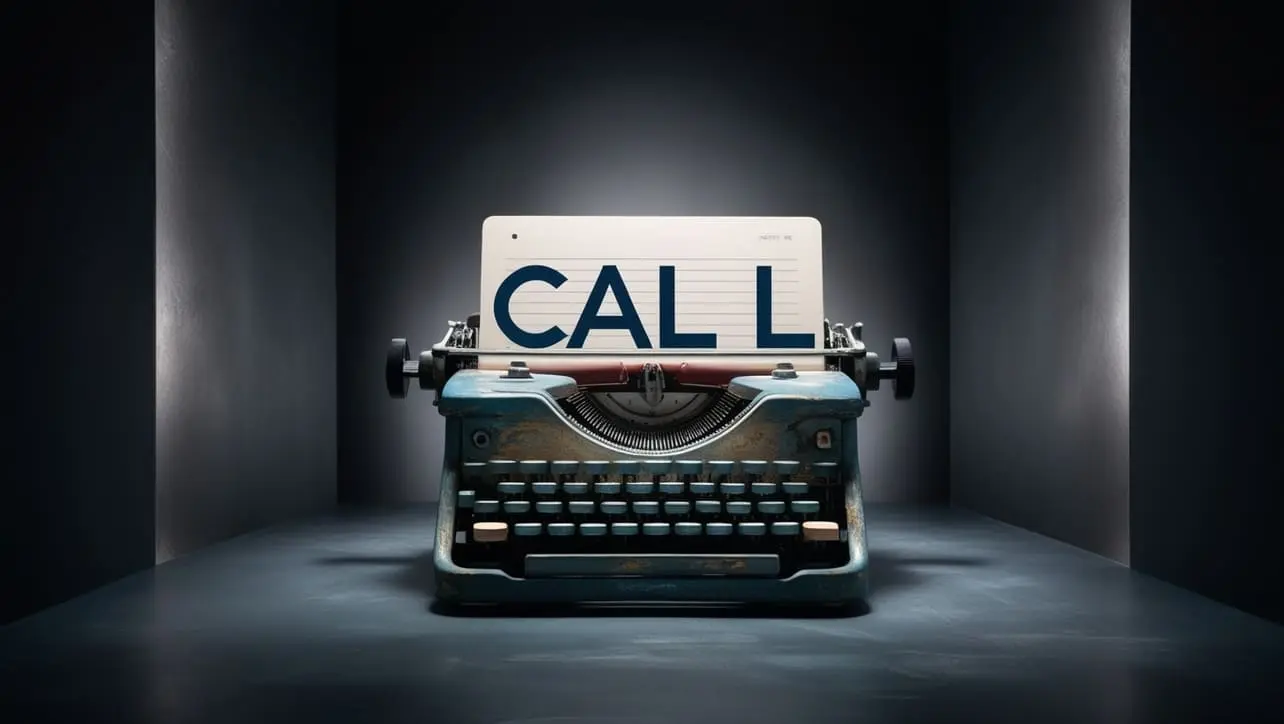
Photo Credit to CodeToFun
π Introduction
The call()
function in Sass is a lesser-known but powerful feature that allows you to dynamically invoke functions.
This is particularly useful when working with higher-order functions, where you want to pass a function as an argument or choose which function to call based on a condition. With call()
, you can make your Sass code more flexible and reusable.
π‘ Syntax
The syntax of the call()
function is simple. It takes a function name as its first argument, followed by any arguments you want to pass to that function.
call(function-name, arguments...)
π’ Parameters
- function-name: A string or identifier representing the name of the function to be called.
- arguments: A list of arguments that the function will accept.
β©οΈ Return Value
The call()
function returns the result of the function it invokes. The return type will depend on the function being called.
π Example Usage
Letβs explore how the call()
function can be used in various scenarios.
π Example 1: Basic Usage
@function add-two($number) {
@return $number + 2;
}
$result: call(add-two, 5); // Result: 7
body {
margin-top: $result * 1px;
}
In this example, the add-two() function is defined to add 2 to a given number. Using call()
, we invoke add-two with 5 as an argument, resulting in 7.
π Example 2: Dynamic Function Calls
@function multiply-by-two($number) {
@return $number * 2;
}
@function divide-by-two($number) {
@return $number / 2;
}
$operation: 'multiply-by-two';
$result: call($operation, 10); // Result: 20
h1 {
font-size: $result * 1px;
}
Here, the operation is dynamically chosen based on the value of the $operation variable. This allows for flexible function calls depending on the context.
π Example 3: Using call() with Built-in Functions
$color: #336699;
$result: call('lighten', $color, 20%); // Result: a lighter shade of the color
nav {
background-color: $result;
}
In this example, we use call()
to invoke the built-in lighten() function, passing the color and the percentage as arguments. This lightens the color dynamically.
π Example 4: Passing Functions as Arguments
@function operate($fn, $value) {
@return call($fn, $value);
}
$result: operate('sqrt', 16); // Result: 4
footer {
height: $result * 1rem;
}
This example shows how you can pass a function name as an argument to another function, making call()
an essential part of writing higher-order functions in Sass.
π Conclusion
The call()
function in Sass opens up new possibilities for dynamic and reusable code. By allowing you to invoke functions programmatically, it brings a level of flexibility that can significantly enhance your workflow, especially when dealing with complex stylesheets. Whether you're working with custom functions or built-in ones, call()
empowers you to write more modular and maintainable Sass code.
Understanding and using call()
effectively can lead to cleaner, more efficient stylesheets, making your design process smoother and more adaptable to changing requirements.
π¨βπ» Join our Community:
Author
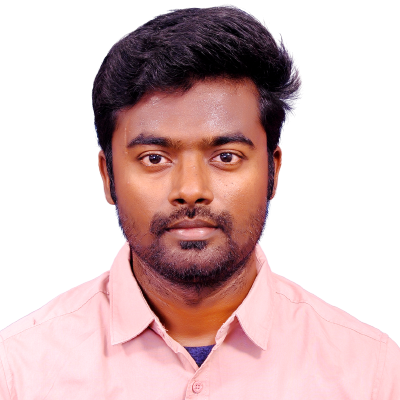
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Sass call() Function), please comment here. I will help you immediately.