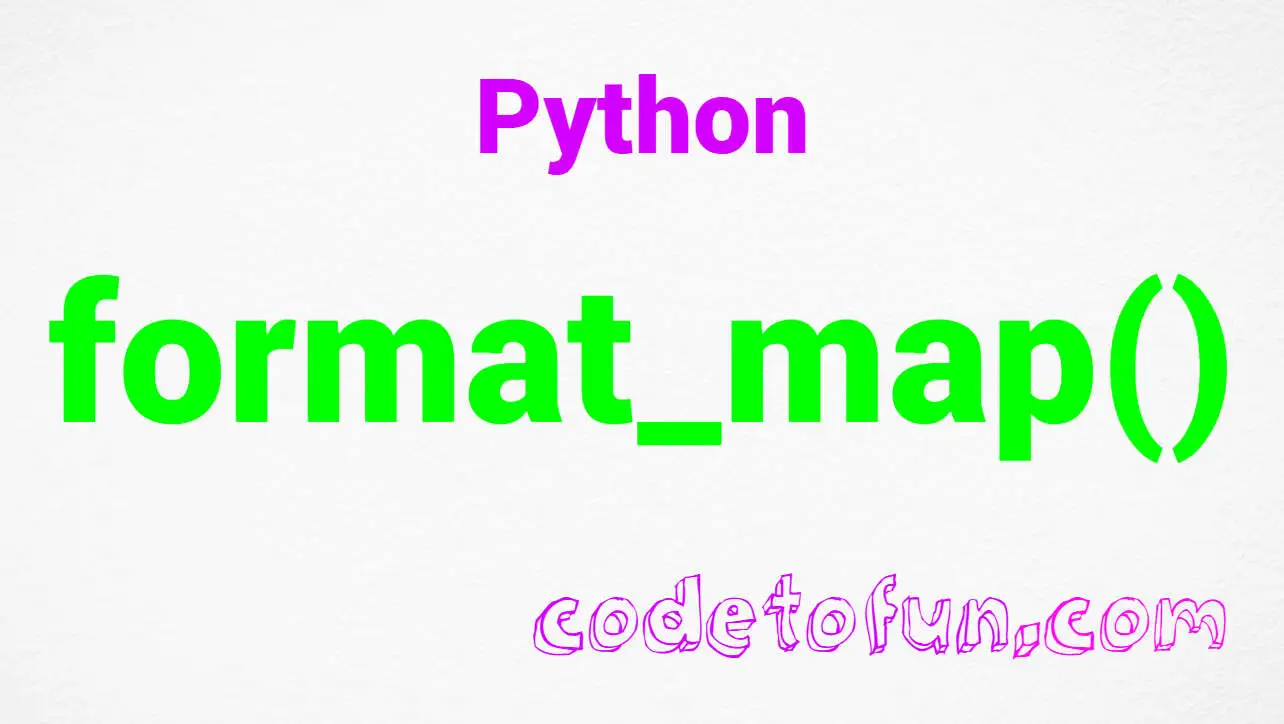
Python Basic
Python Interview Programs
- Python Interview Programs
- Python Abundant Number
- Python Amicable Number
- Python Armstrong Number
- Python Average of N Numbers
- Python Automorphic Number
- Python Biggest of three numbers
- Python Binary to Decimal
- Python Common Divisors
- Python Composite Number
- Python Condense a Number
- Python Cube Number
- Python Decimal to Binary
- Python Decimal to Octal
- Python Disarium Number
- Python Even Number
- Python Evil Number
- Python Factorial of a Number
- Python Fibonacci Series
- Python GCD
- Python Happy Number
- Python Harshad Number
- Python LCM
- Python Leap Year
- Python Magic Number
- Python Matrix Addition
- Python Matrix Division
- Python Matrix Multiplication
- Python Matrix Subtraction
- Python Matrix Transpose
- Python Maximum Value of an Array
- Python Minimum Value of an Array
- Python Multiplication Table
- Python Natural Number
- Python Number Combination
- Python Odd Number
- Python Palindrome Number
- Python Pascalβs Triangle
- Python Perfect Number
- Python Perfect Square
- Python Power of 2
- Python Power of 3
- Python Pronic Number
- Python Prime Factor
- Python Prime Number
- Python Smith Number
- Python Strong Number
- Python Sum of Array
- Python Sum of Digits
- Python Swap Two Numbers
- Python Triangular Number
Python Program to Check Power of 3
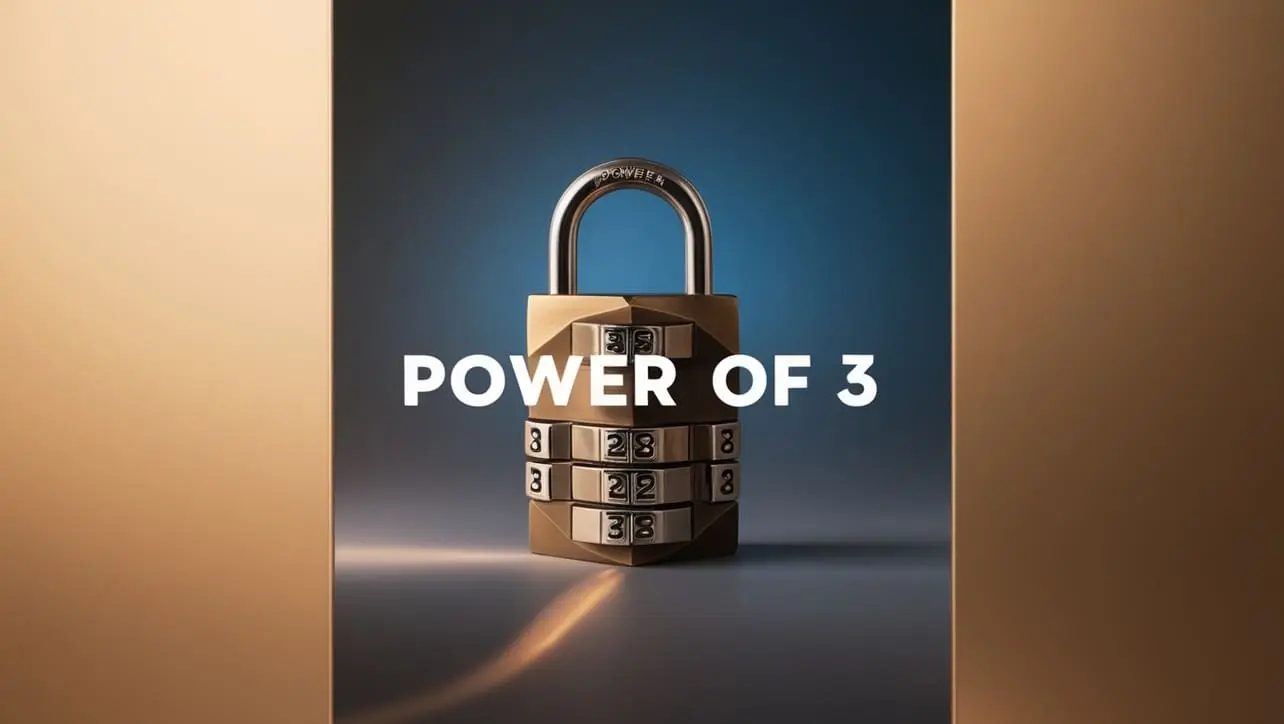
Photo Credit to CodeToFun
π Introduction
In the realm of programming, one often encounters the need to check if a given number is a power of another.
In this context, we'll explore a Python program designed specifically to check if a number is a power of 3.
The logic behind this program involves repeatedly dividing the number by 3 until it becomes 1, indicating that it is a power of 3.
π Example
Let's delve into the Python code that achieves this functionality.
# Function to check if a number is a power of 3
def is_power_of_3(n):
# Keep dividing the number by 3 until it is greater than 1
while n % 3 == 0 and n > 1:
n //= 3
# If the final value is 1, the original number is a power of 3
return n == 1
# Driver program
if __name__ == "__main__":
# Replace this value with your desired number
number = 27
# Check if the number is a power of 3
if is_power_of_3(number):
print(f"{number} is a power of 3")
else:
print(f"{number} is not a power of 3")
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the if __name__ == "__main__": block.
27 is a power of 3
Run the script to see if the given number is a power of 3.
π§ How the Program Works
- The program defines a function is_power_of_3 that takes an integer as input and checks if it is a power of 3.
- Inside the function, it repeatedly divides the number by 3 until it becomes greater than 1.
- If the final value is 1, the original number is a power of 3, and the function returns True; otherwise, it returns False.
- The driver program in the if __name__ == "__main__": block tests a specific number and prints whether it is a power of 3 or not.
π Between the Given Range
Let's take a look at the python code that checks for powers of 3 in the given range.
# Function to check if a number is a power of 3
def is_power_of_three(num):
return num > 0 and (3 ** 19) % num == 0
# Display powers of 3 in the range 1 to 20
def display_powers_of_three():
print("Power of 3 in the range 1 to 20:")
for i in range(1, 21):
if is_power_of_three(i):
print(i, end=" ")
# Driver program
if __name__ == "__main__":
# Call the function to display powers of 3
display_powers_of_three()
π» Testing the Program
Power of 3 in the range 1 to 20: 1 3 9
Run the script to see the powers of 3 in the range from 1 to 20.
π§ How the Program Works
- The program defines a function is_power_of_three that checks if a number is a power of 3 using the property that 319 is the largest power of 3 that fits within the 32-bit signed integer range.
- The display_powers_of_three function iterates through the range from 1 to 20 and prints the numbers that are powers of 3.
- The main block calls the function to display the powers of 3 in the specified range.
π§ Understanding the Concept of Power of 3
Before diving into the code, let's understand the concept of a power of 3.
A number is considered a power of 3 if it can be expressed as 3^n, where n is an integer.
For example, 1, 3, 9, 27, and so on are powers of 3.
π’ Optimizing the Program
While the provided program is straightforward, there are alternative approaches to check if a number is a power of 3. Consider exploring other mathematical techniques for optimization.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
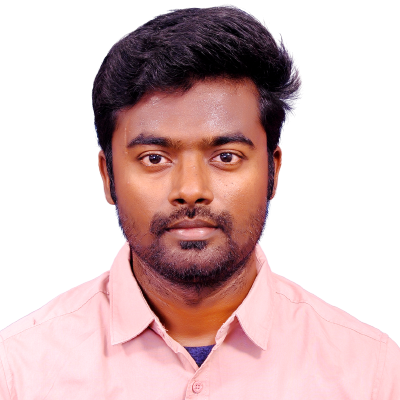
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python Program to Check Power of 3), please comment here. I will help you immediately.