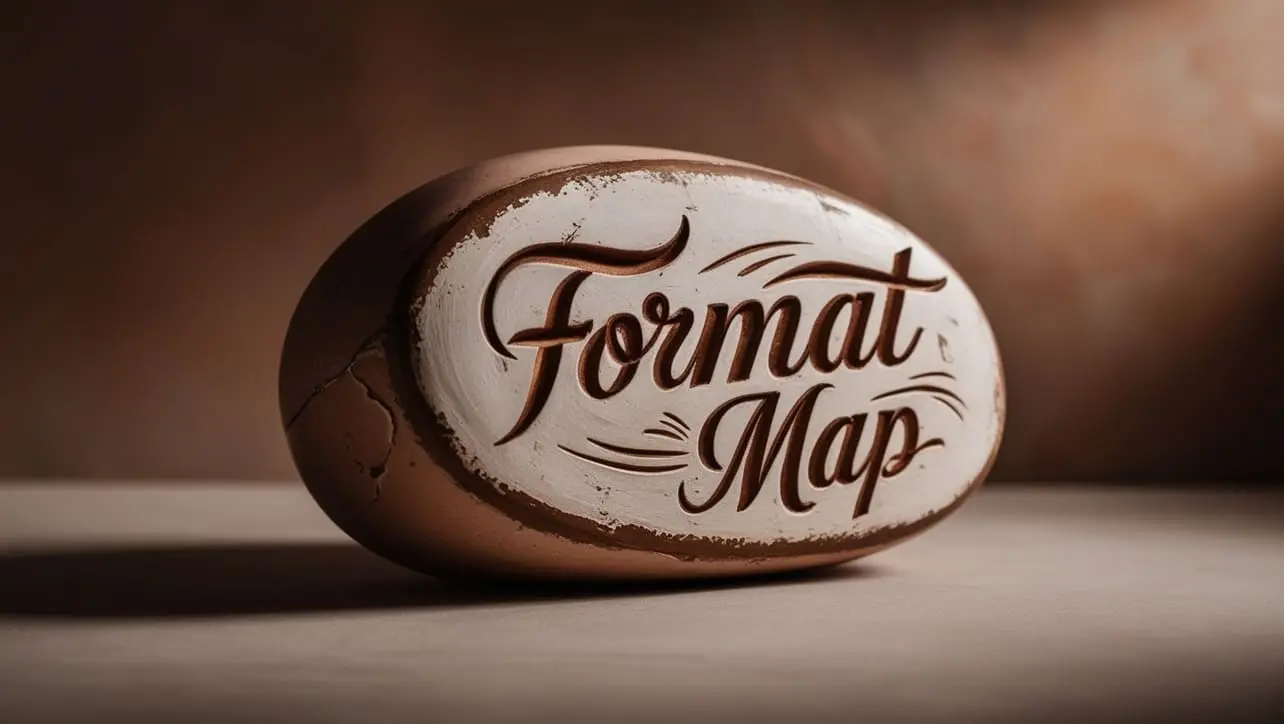
Python Topics
- Python Intro
- Python String Methods
- Python Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Python Star Pattern
- Python Number Pattern
- Python Alphabet Pattern
Python Program to Display Fibonacci Series
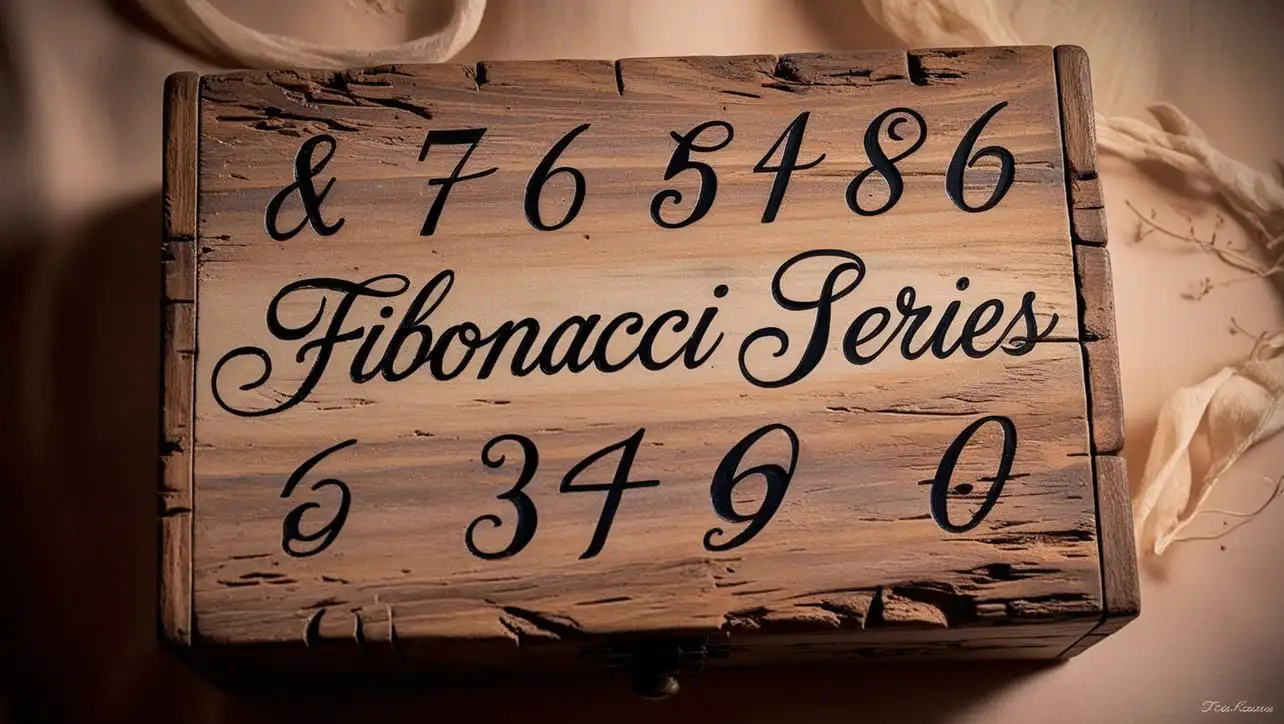
Photo Credit to CodeToFun
Introduction
The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1.
This series has widespread applications in various fields, including mathematics and computer science.
In this tutorial, we'll explore a Python program that generates and displays the Fibonacci series.
Example
Let's delve into the Python code that generates and displays the Fibonacci series.
# Function to display Fibonacci series up to n terms
def display_fibonacci(n):
first, second = 0, 1
print(f"Fibonacci series up to {n} terms: ", end="")
for _ in range(n):
print(first, end=", ")
first, second = second, first + second
print()
# Driver program
if __name__ == "__main__":
# Replace this value with the desired number of terms
terms = 10
# Call the function to display Fibonacci series
display_fibonacci(terms)
Testing the Program
To test the program with a different number of terms, simply replace the value of the terms variable in the if __name__ == "__main__": block.
Fibonacci series up to 10 terms: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Run the script to see the Fibonacci series up to the desired number of terms.
How the Program Works
- The program defines a function display_fibonacci that takes the number of terms (n) as input and prints the Fibonacci series up to n terms.
- Inside the function, it initializes variables first and second with 0 and 1, respectively.
- It then uses a loop to calculate and print the Fibonacci series up to the specified number of terms.
- The loop updates the values of first and second in each iteration to generate the next Fibonacci number.
Understanding the Concept of Fibonacci Series
The Fibonacci series starts with 0 and 1. Each subsequent number in the series is the sum of the two preceding ones. The series begins as follows:
0, 1, 1, 2, 3, 5, 8, 13, 21, ...
Optimizing the Program
While the provided program is straightforward, consider exploring and implementing optimizations for larger Fibonacci series, such as using memoization techniques to avoid redundant calculations.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
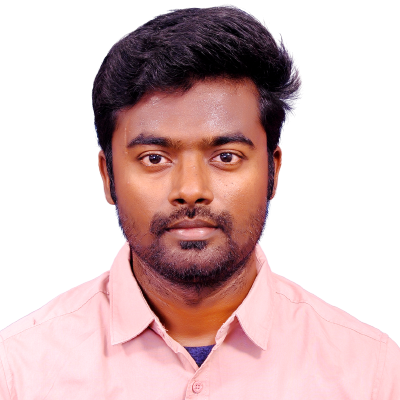
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
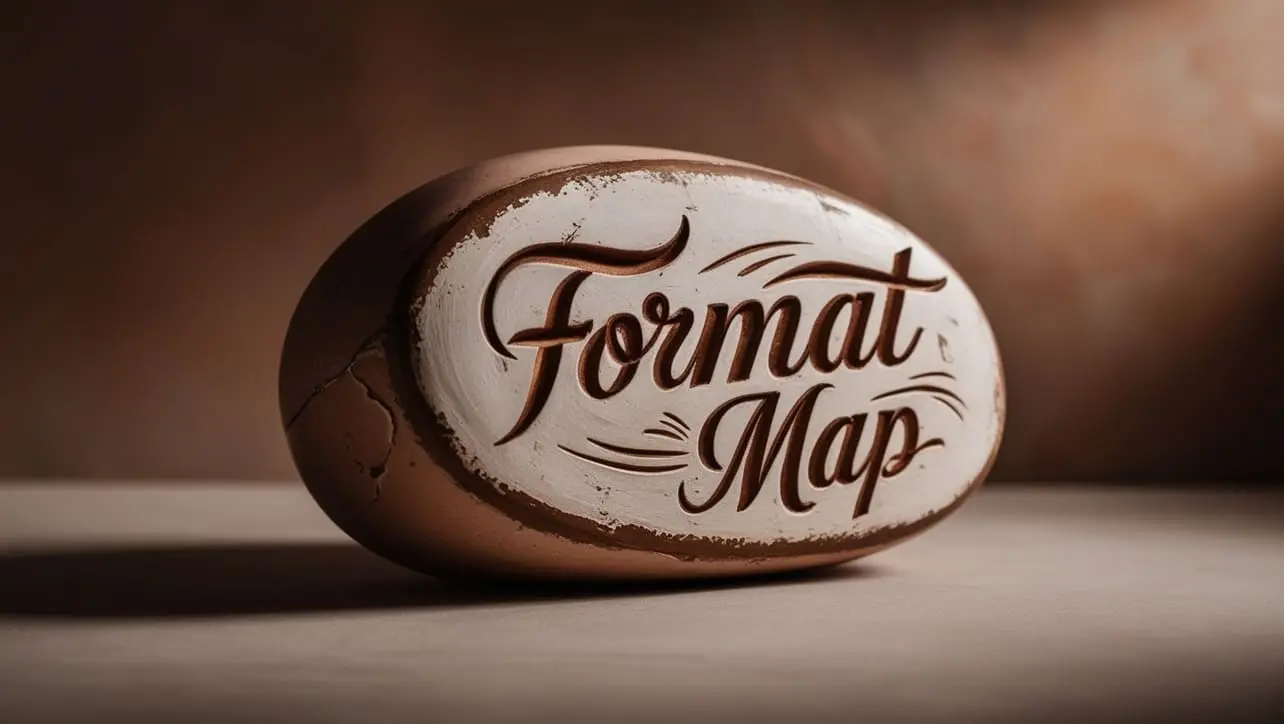
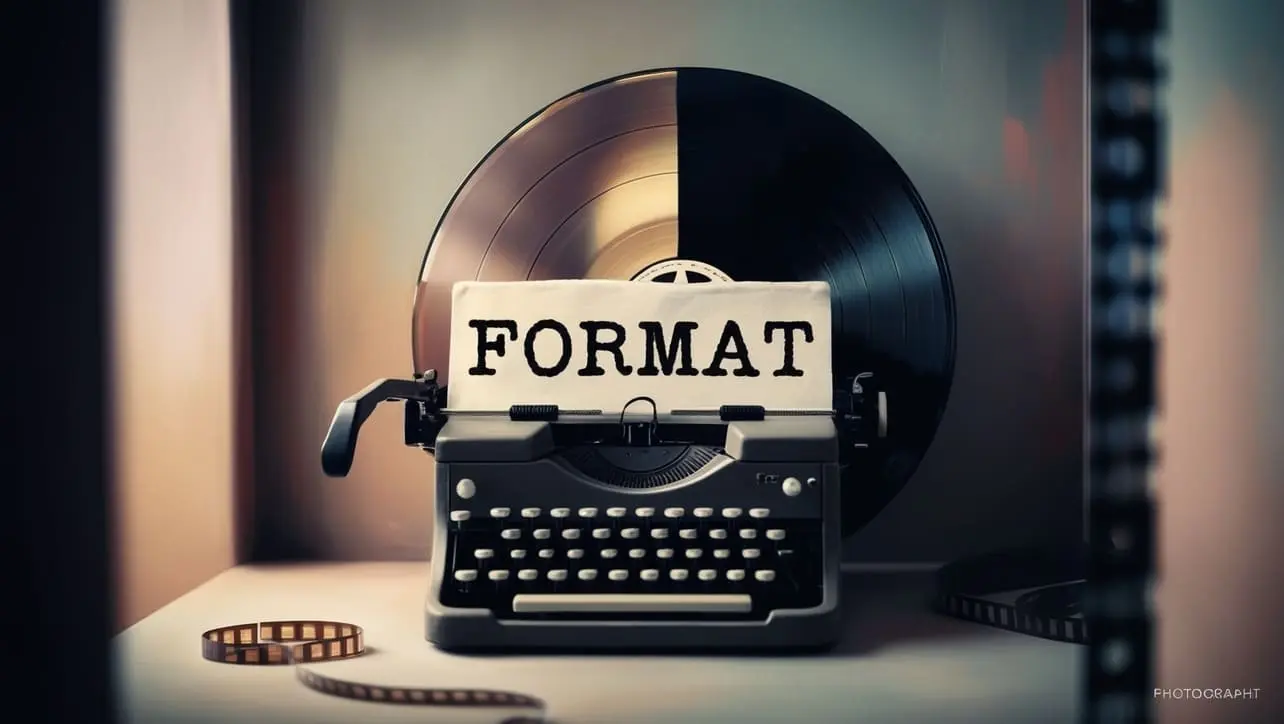
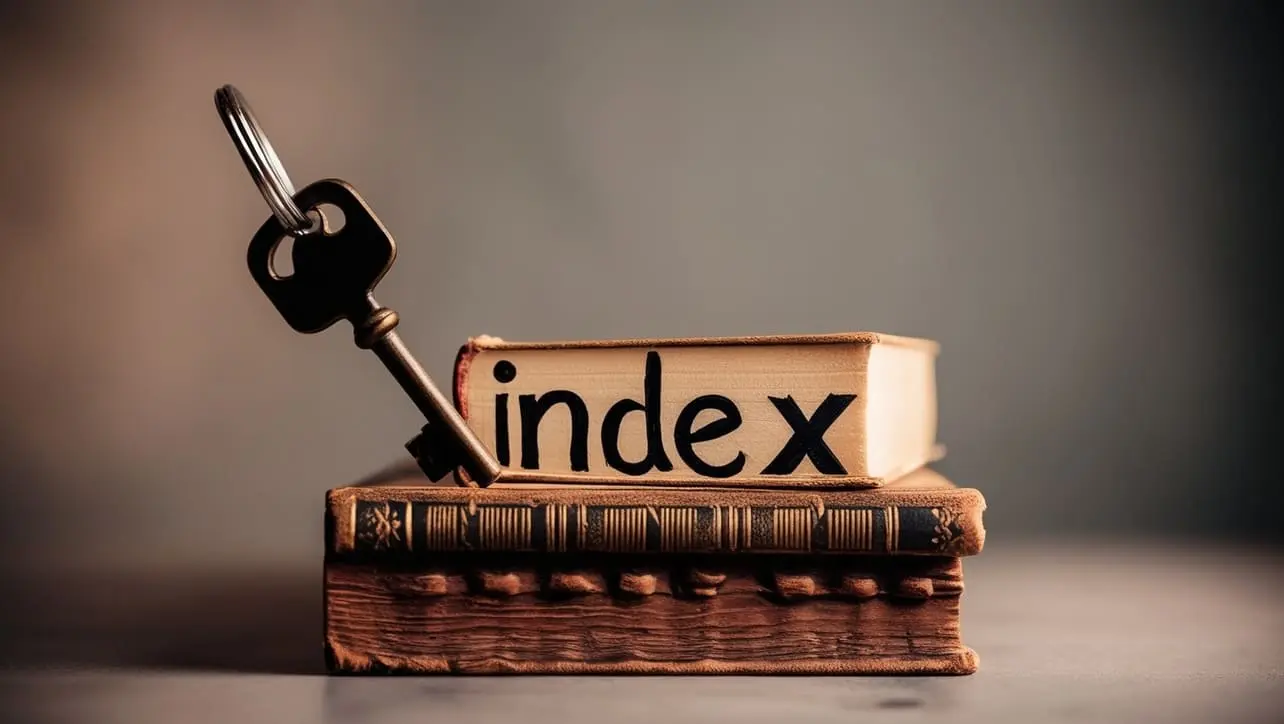
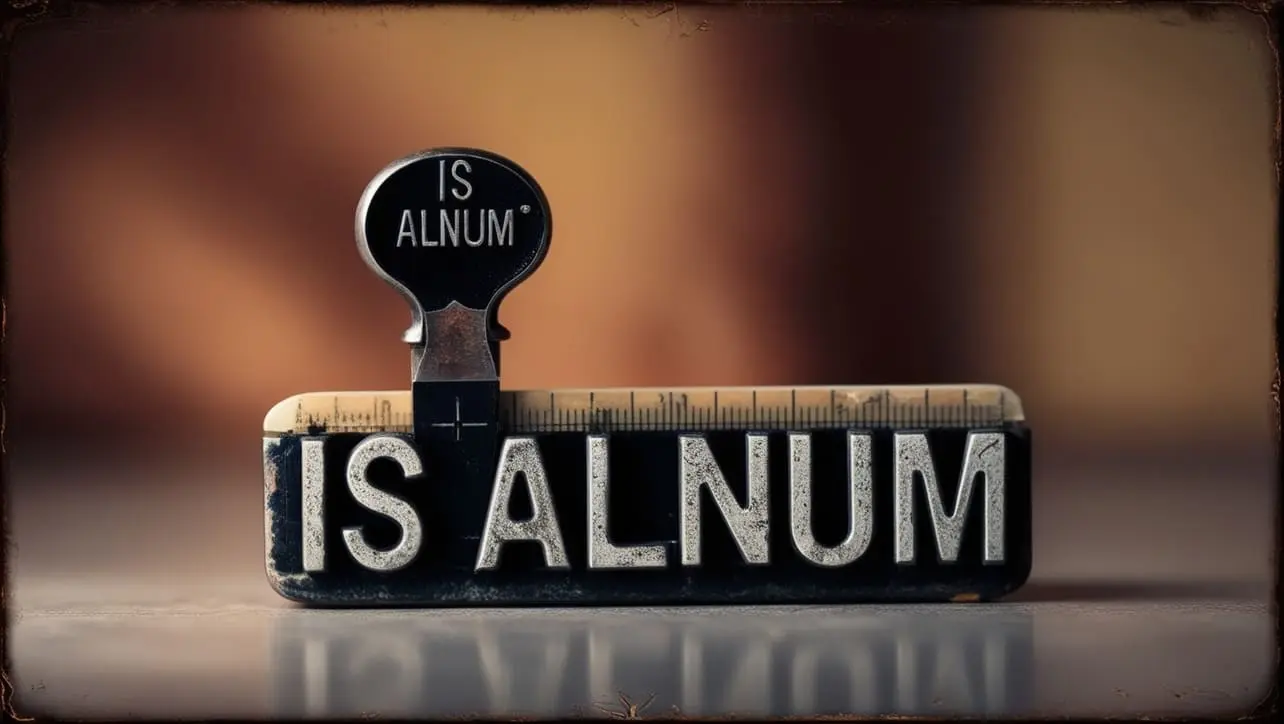
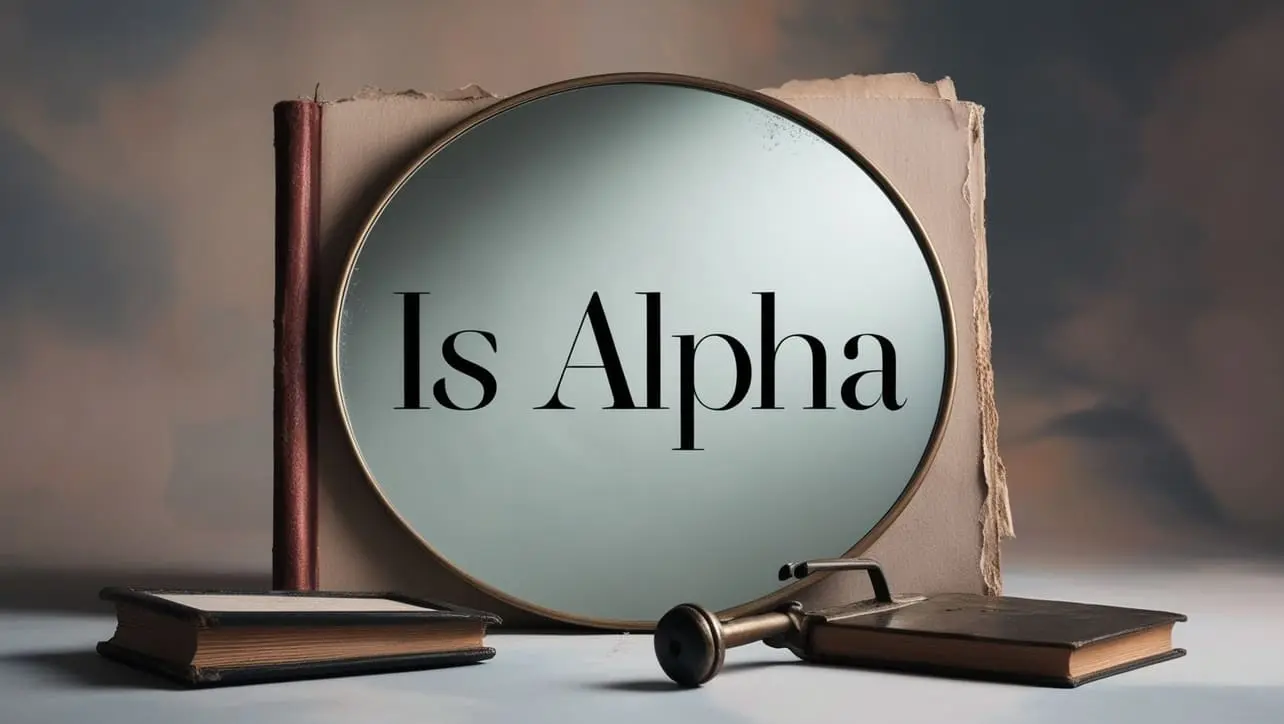
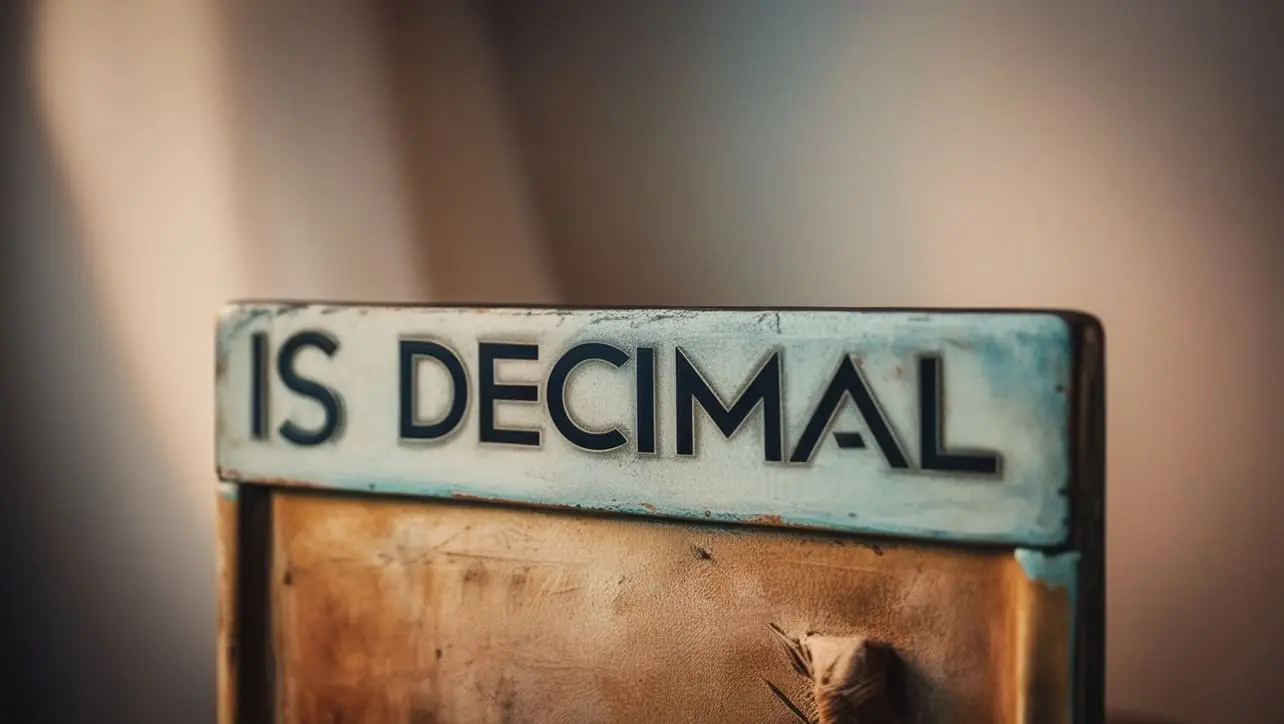
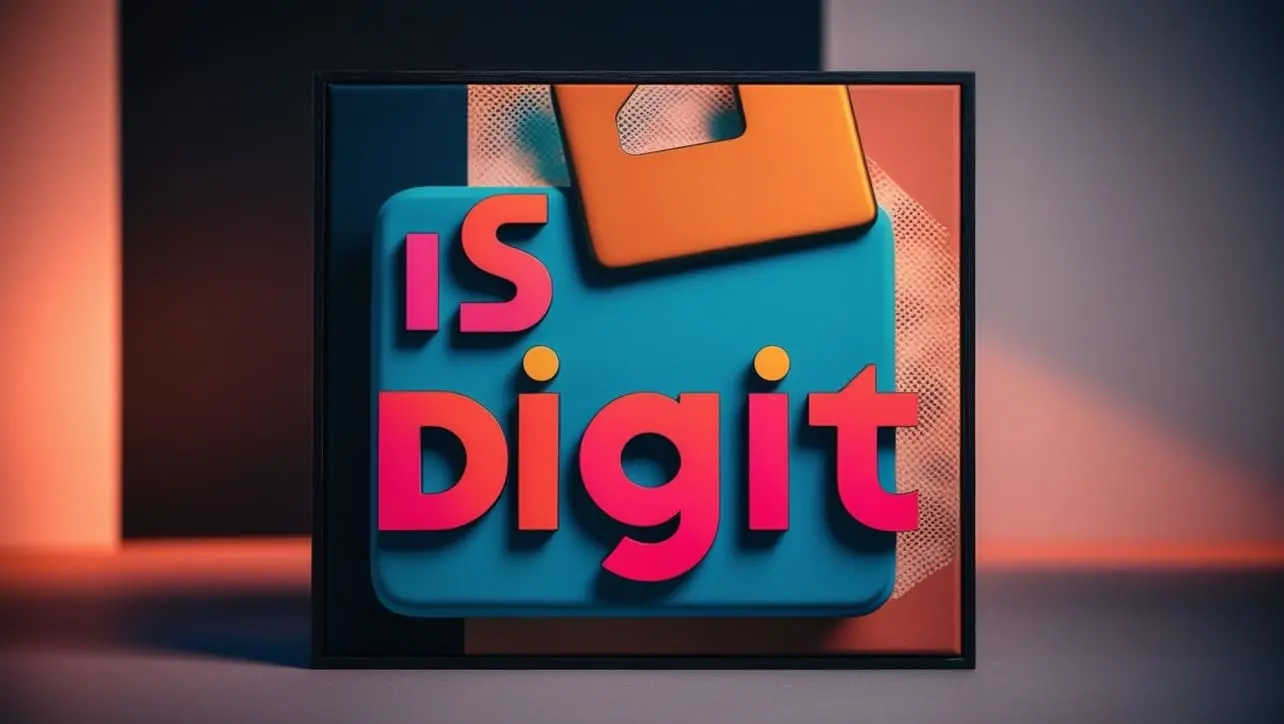
If you have any doubts regarding this article (Python Program to Display Fibonacci Series), please comment here. I will help you immediately.