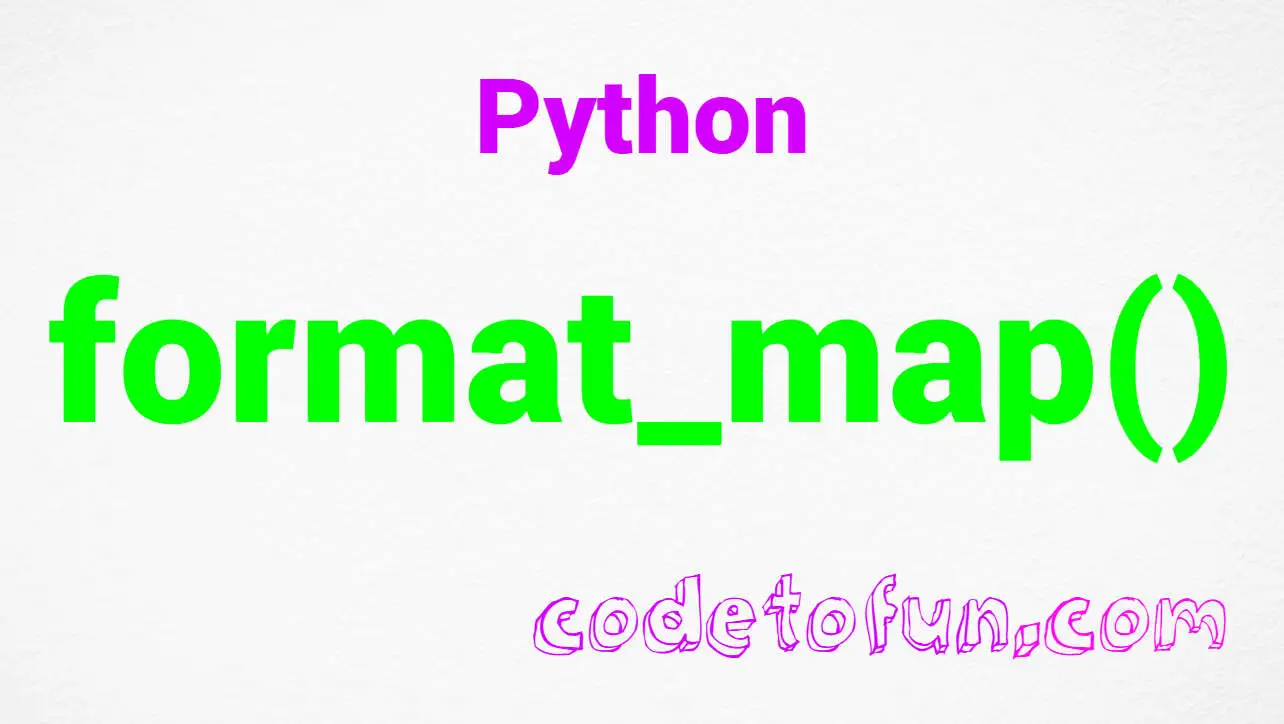
Python Basic
Python Interview Programs
- Python Interview Programs
- Python Abundant Number
- Python Amicable Number
- Python Armstrong Number
- Python Average of N Numbers
- Python Automorphic Number
- Python Biggest of three numbers
- Python Binary to Decimal
- Python Common Divisors
- Python Composite Number
- Python Condense a Number
- Python Cube Number
- Python Decimal to Binary
- Python Decimal to Octal
- Python Disarium Number
- Python Even Number
- Python Evil Number
- Python Factorial of a Number
- Python Fibonacci Series
- Python GCD
- Python Happy Number
- Python Harshad Number
- Python LCM
- Python Leap Year
- Python Magic Number
- Python Matrix Addition
- Python Matrix Division
- Python Matrix Multiplication
- Python Matrix Subtraction
- Python Matrix Transpose
- Python Maximum Value of an Array
- Python Minimum Value of an Array
- Python Multiplication Table
- Python Natural Number
- Python Number Combination
- Python Odd Number
- Python Palindrome Number
- Python Pascalβs Triangle
- Python Perfect Number
- Python Perfect Square
- Python Power of 2
- Python Power of 3
- Python Pronic Number
- Python Prime Factor
- Python Prime Number
- Python Smith Number
- Python Strong Number
- Python Sum of Array
- Python Sum of Digits
- Python Swap Two Numbers
- Python Triangular Number
Python Program to Check for a Cube Number
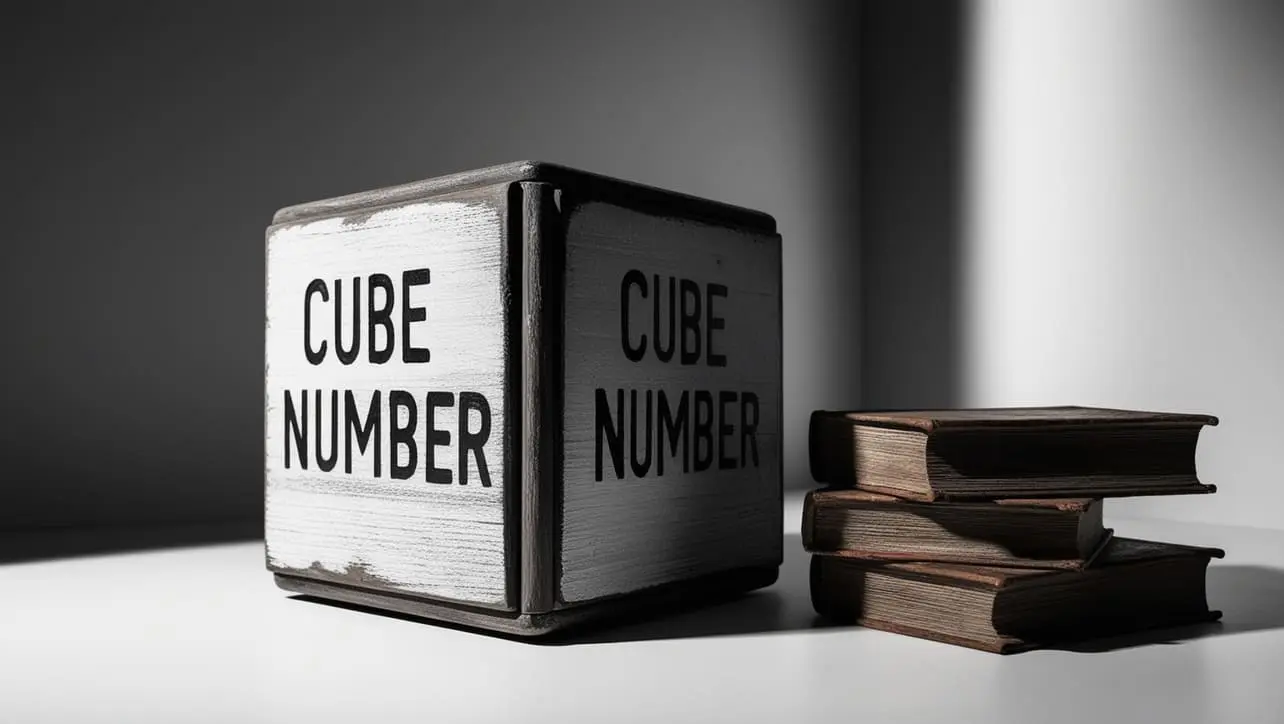
Photo Credit to CodeToFun
π Introduction
In the realm of programming, mathematical properties of numbers often lead to interesting and practical applications. One such property is being a cube number. A cube number is an integer that is the cube of an integer.
In this tutorial, we'll delve into a Python program that efficiently checks whether a given number is a perfect cube.
π Example
Let's take a look at the Python code that accomplishes this functionality.
def is_perfect_cube(number):
# Calculate the cube root of the number
cube_root = number ** (1/3)
# Check if the cube root is close to an integer
return abs(cube_root - round(cube_root)) < 1e-10
# Driver program
if __name__ == "__main__":
# Replace this value with your desired number
number = 27
# Check if the number is a perfect cube
if is_perfect_cube(number):
print(f"{number} is a perfect cube.")
else:
print(f"{number} is not a perfect cube.")
π» Testing the Program
To test the program with different numbers, simply replace the value of the number variable in the if __name__ == "__main__": block.
27 is a perfect cube.
Run the script to check if the number is a perfect cube.
π§ How the Program Works
- The program dexfines a function is_perfect_cube that takes an integer as input and returns True if it is a perfect cube, and False otherwise.
- Inside the function, it calculates the cube root of the number using the exponentiation operator.
- It then checks if the cube root is close to an integer using a tolerance value (1e-10 in this case).
- The if __name__ == "__main__": block initializes a variable with a number and calls the is_perfect_cube function to determine if it's a perfect cube.
π Between the Given Range
Let's dive into the Python code that checks for cube numbers in the range from 1 to 50.
# Function to check if a number is a cube number
def is_cube_number(num):
cube_root = round(num ** (1 / 3))
return cube_root ** 3 == num
# Main program to find cube numbers in the range 1 to 50
def find_cube_numbers():
print("Cube numbers in the range 1 to 50:")
for i in range(1, 51):
if is_cube_number(i):
print(i, end=" ")
# Call the function to find cube numbers
find_cube_numbers()
π» Testing the Program
Cube numbers in the range 1 to 50: 1 8 27
Run the script to see the cube numbers in the specified range.
π§ How the Program Works
- The program defines a function is_cube_number that checks if a given number is a cube number.
- Inside the function, it calculates the cube root of the number and checks if raising it to the power of 3 results in the original number.
- The main program, find_cube_numbers, iterates through the range from 1 to 50 and prints the cube numbers.
π§ Understanding the Concept of Cube Number
A cube number is the result of raising an integer to the power of 3. For example, 2 * 2 * 2 = 8, so 8 is a cube number.
π’ Optimizing the Program
The provided program is straightforward, but you might consider optimizing it further. For instance, you can explore alternative methods for checking if a number is a cube number without using the cbrt function.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
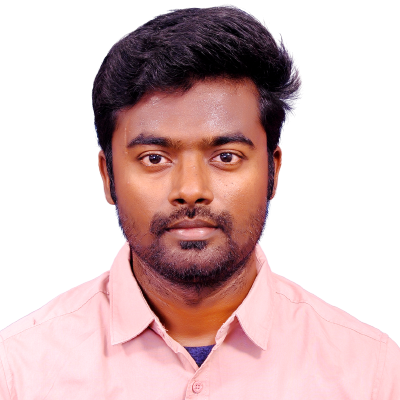
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python Program to Check for a Cube Number), please comment here. I will help you immediately.