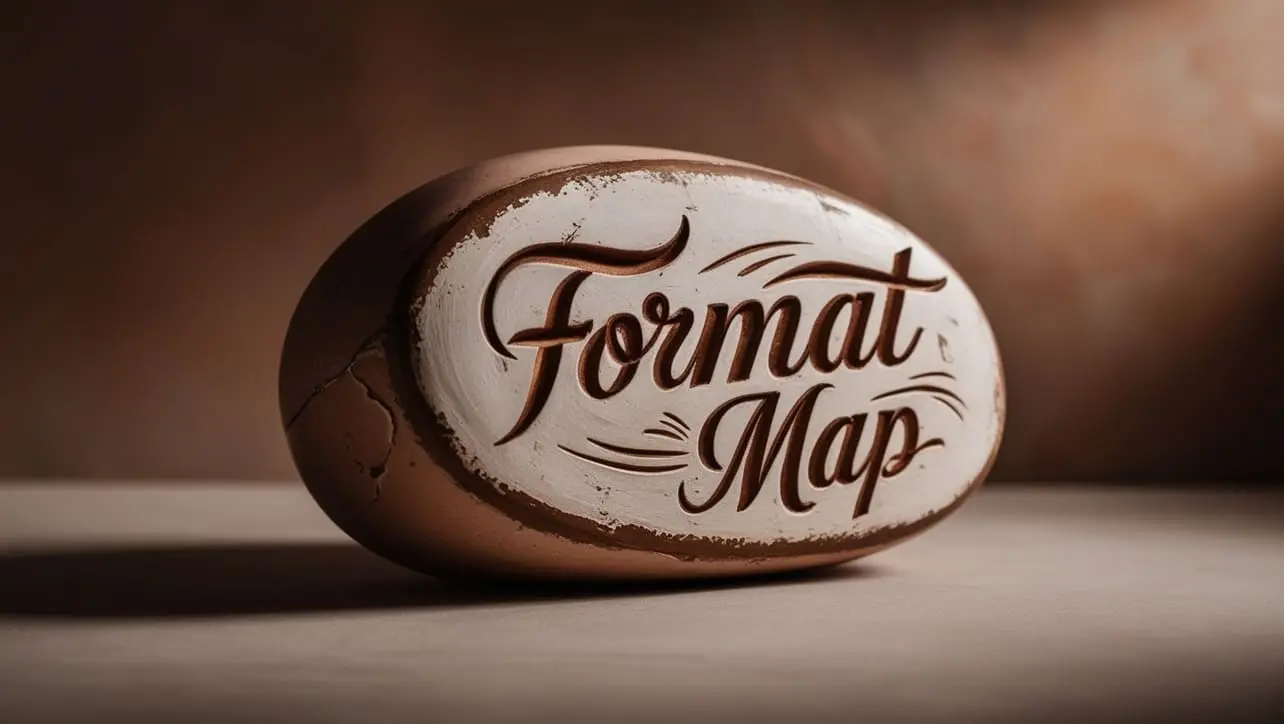
Python Topics
- Python Intro
- Python String Methods
- Python Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Python Star Pattern
- Python Number Pattern
- Python Alphabet Pattern
Python Program to Check Armstrong Number
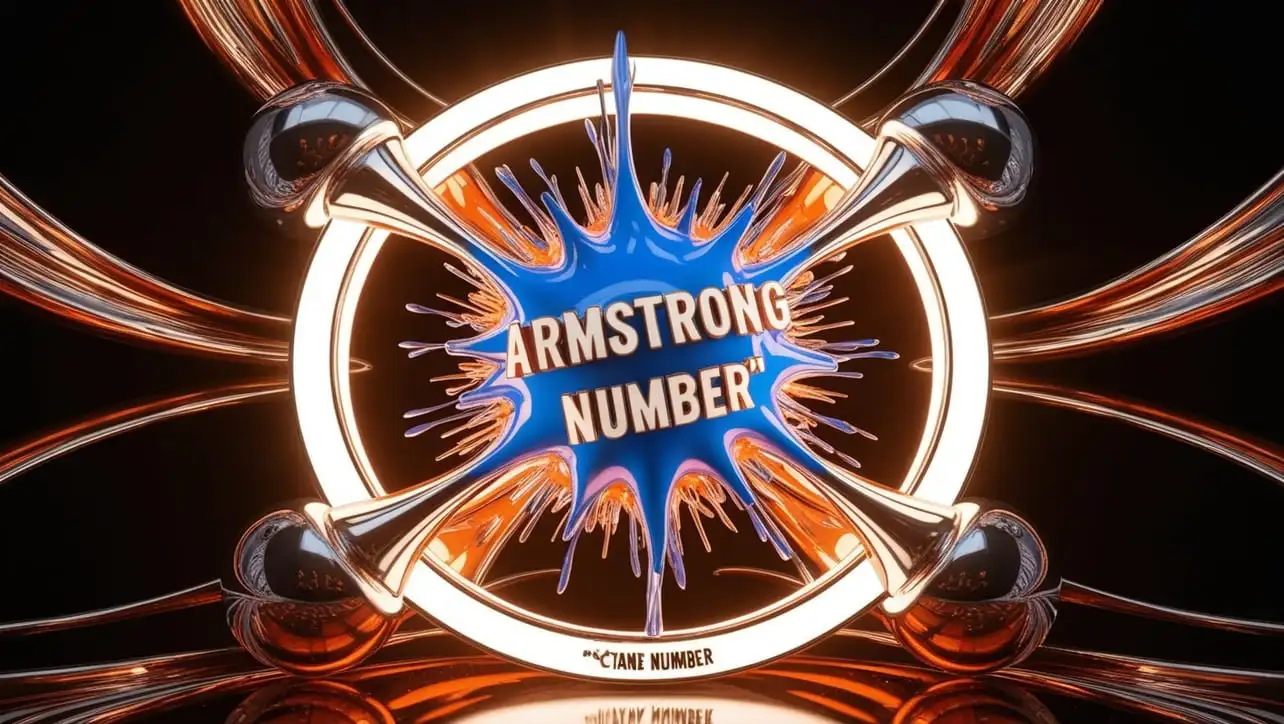
Photo Credit to CodeToFun
Introduction
In the realm of programming, solving mathematical problems is a common task. One such interesting problem is checking whether a given number is an Armstrong number.
Armstrong numbers, also known as narcissistic numbers or pluperfect digital invariants, are numbers that are the sum of their own digits each raised to the power of the number of digits.
In this tutorial, we will explore a Python program designed to check whether a given number is an Armstrong number. The program involves calculating the sum of the digits raised to the power of the number of digits and comparing it with the original number.
Example
Let's delve into the Python code that accomplishes this task.
# Function to check if a number is an Armstrong number
def is_armstrong(number):
original_number, n, result = number, 0, 0
# Count the number of digits
while original_number != 0:
original_number //= 10
n += 1
# Reset original_number to the actual number
original_number = number
# Calculate the sum of nth power of individual digits
while original_number != 0:
remainder = original_number % 10
result += remainder ** n
original_number //= 10
# Check if the result is equal to the original number
return result == number
# Driver program
# Replace this value with the number you want to check
number = 153
# Call the function to check if the number is Armstrong
if is_armstrong(number):
print(f"{number} is an Armstrong number.")
else:
print(f"{number} is not an Armstrong number.")
Testing the Program
To test the program with different numbers, modify the value of number in the code.
153 is an Armstrong number.
How the Program Works
- The program defines a function is_armstrong that takes an integer number as input and checks whether it is an Armstrong number.
- Replace the value of number in the main program with the desired number you want to check.
- The program calls the is_armstrong function and prints the result.
Between the Given Range
Let's take a look at the Python code that checks for Armstrong numbers in the range 1 to 50.
# Function to check if a number is Armstrong
def is_armstrong_number(number):
num_str = str(number)
num_digits = len(num_str)
total = sum(int(digit) ** num_digits for digit in num_str)
return total == number
# Print Armstrong numbers in the range 1 to 50 in the same line
print("Armstrong numbers in the range from 1 to 50:")
armstrong_numbers = [num for num in range(1, 51) if is_armstrong_number(num)]
print(", ".join(map(str, armstrong_numbers)))
Testing the Program
Armstrong numbers in the range from 1 to 50: 1, 2, 3, 4, 5, 6, 7, 8, 9,
The provided program checks for Armstrong numbers in the range from 1 to 50. You can run the script as is to see the Armstrong numbers in that range.
How the Program Works
- The program defines a function is_armstrong_number that checks if a given number is an Armstrong number.
- Inside the function, it calculates the number of digits and computes the sum of each digit raised to the power of the number of digits.
- The main section uses a loop to iterate through numbers in the range 1 to 50 and prints those that are Armstrong numbers.
Understanding the Concept of Armstrong Number
Before delving into the code, let's understand the concept behind Armstrong numbers. An Armstrong number is a number that is the sum of its own digits each raised to the power of the number of digits.
For example, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
Optimizing the Program
While the provided program is straightforward, consider exploring and implementing alternative approaches or optimizations for checking Armstrong numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
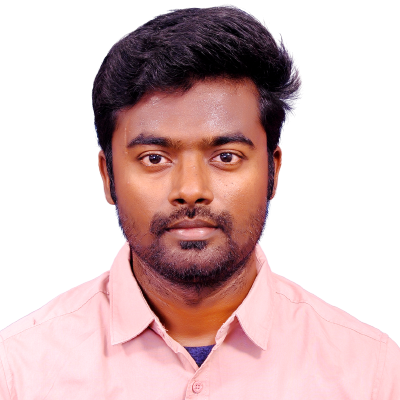
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
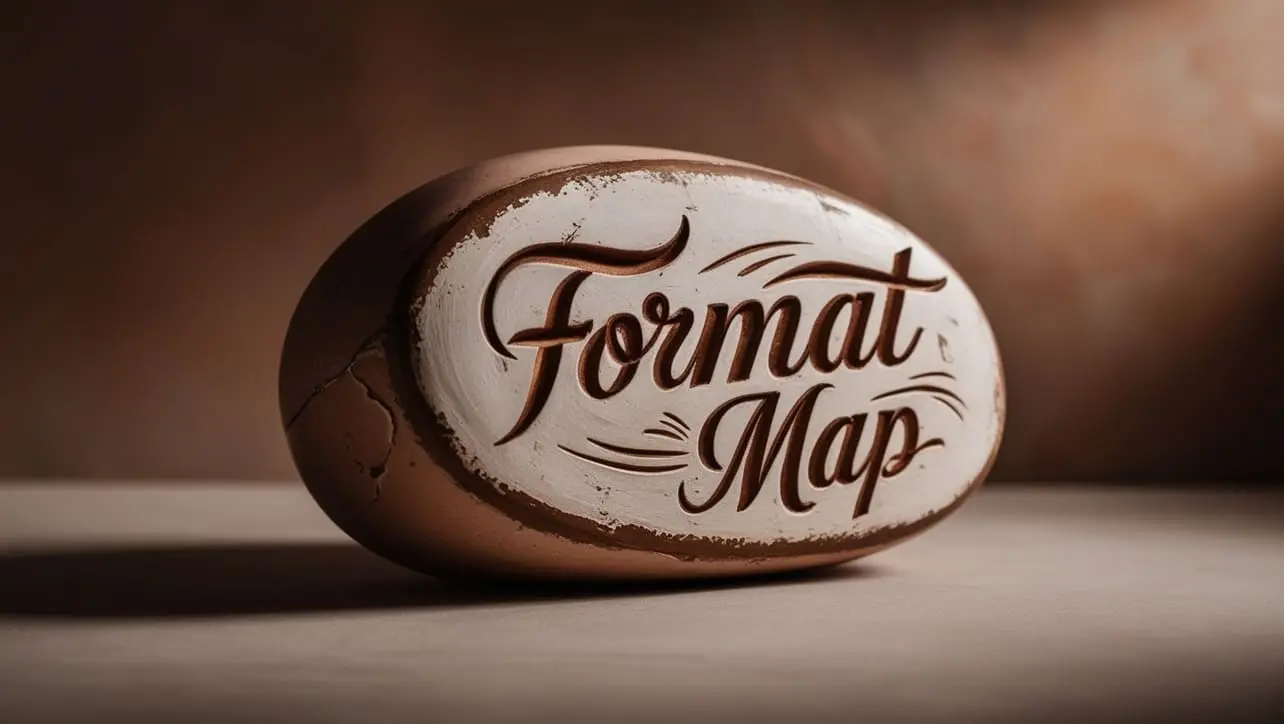
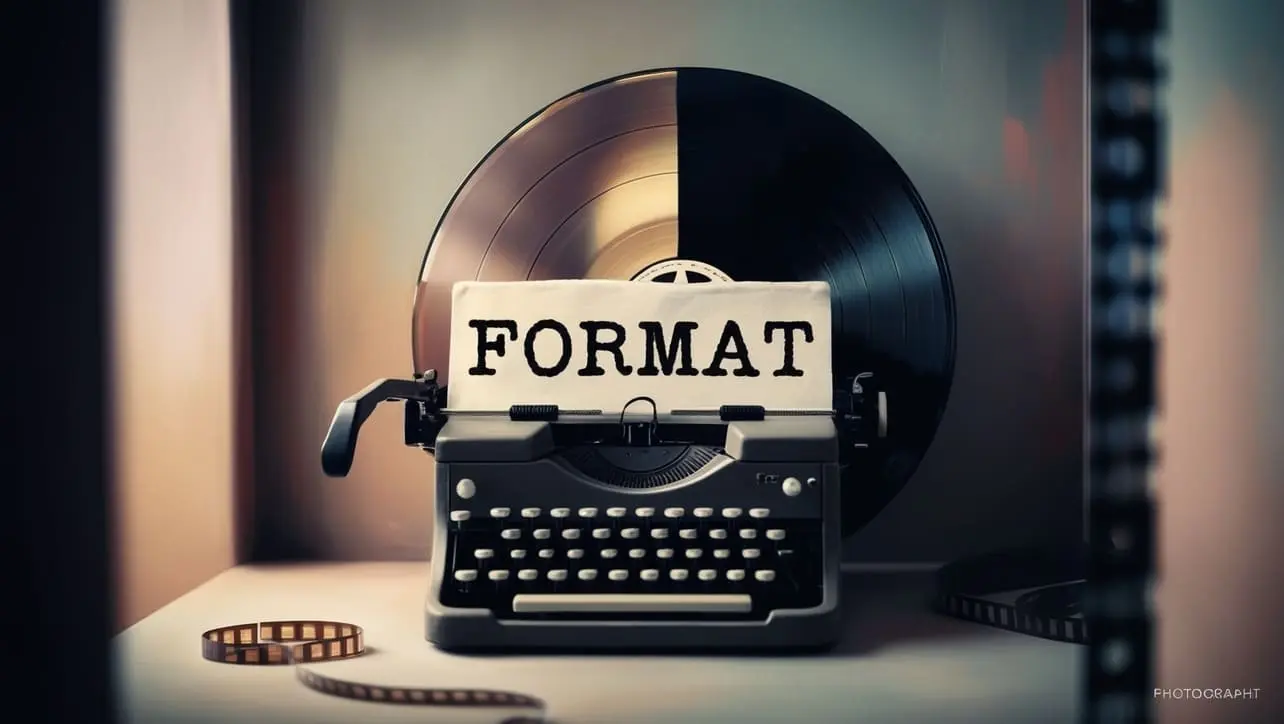
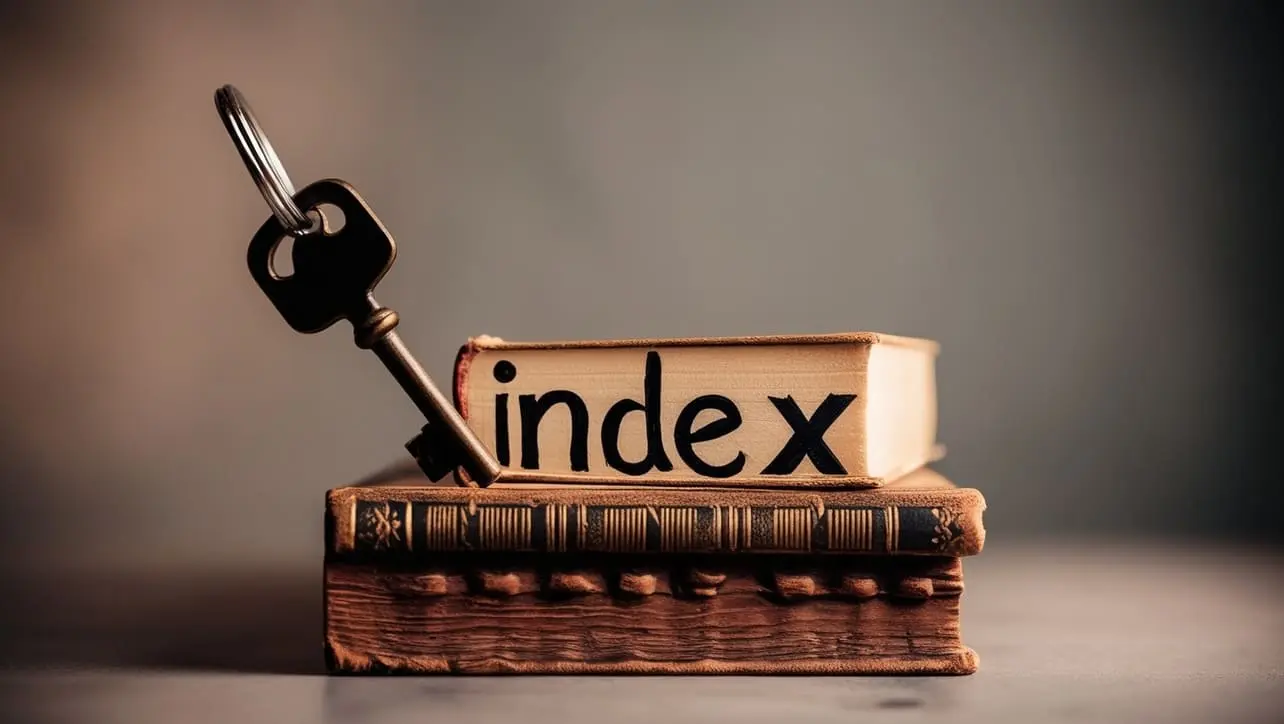
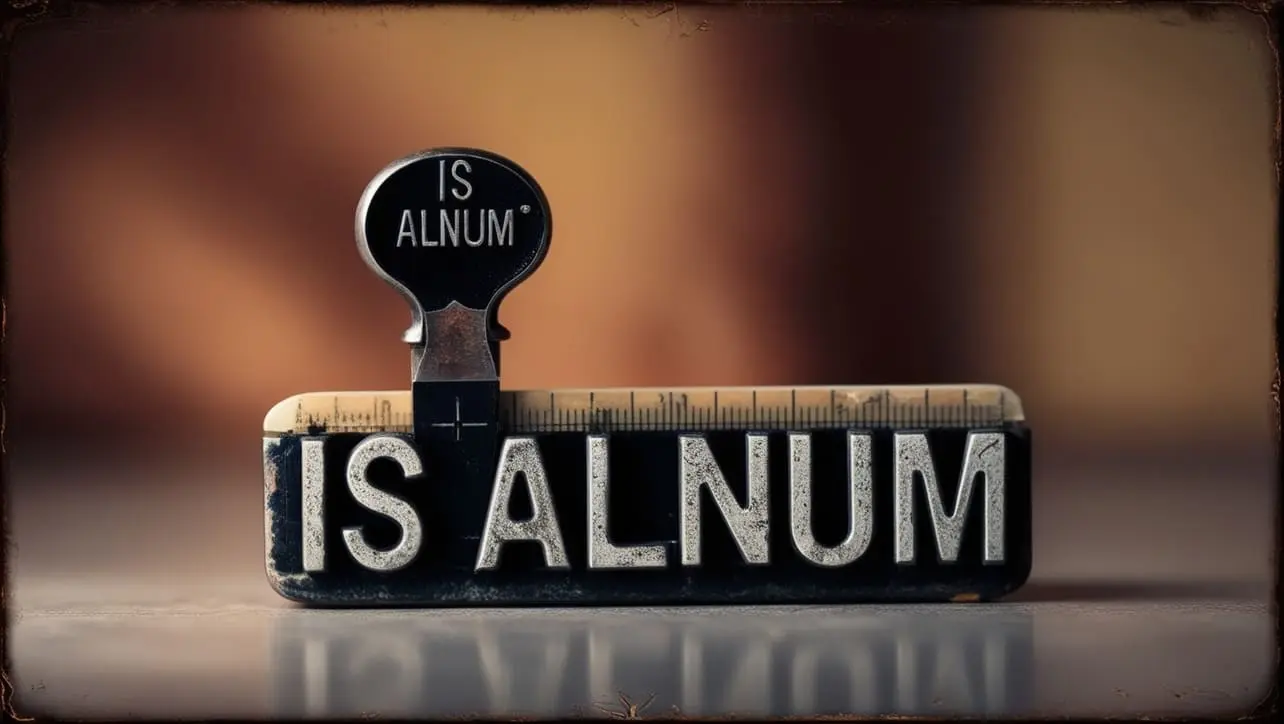
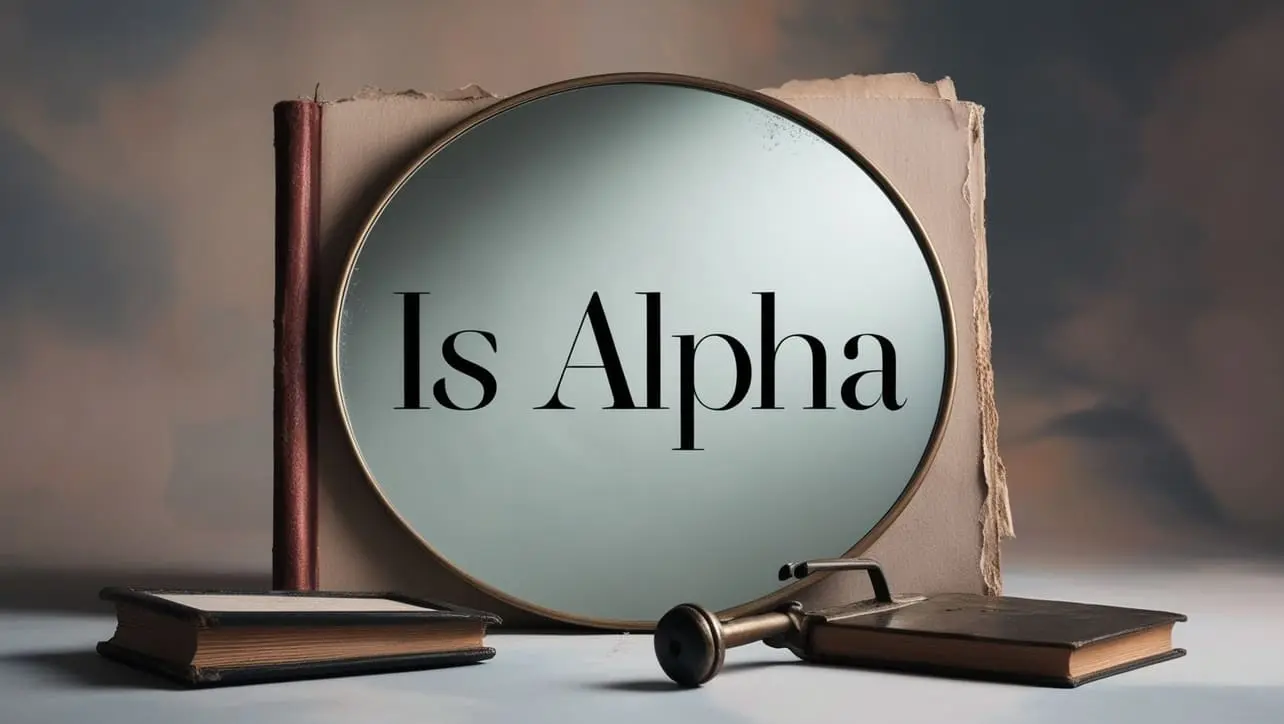
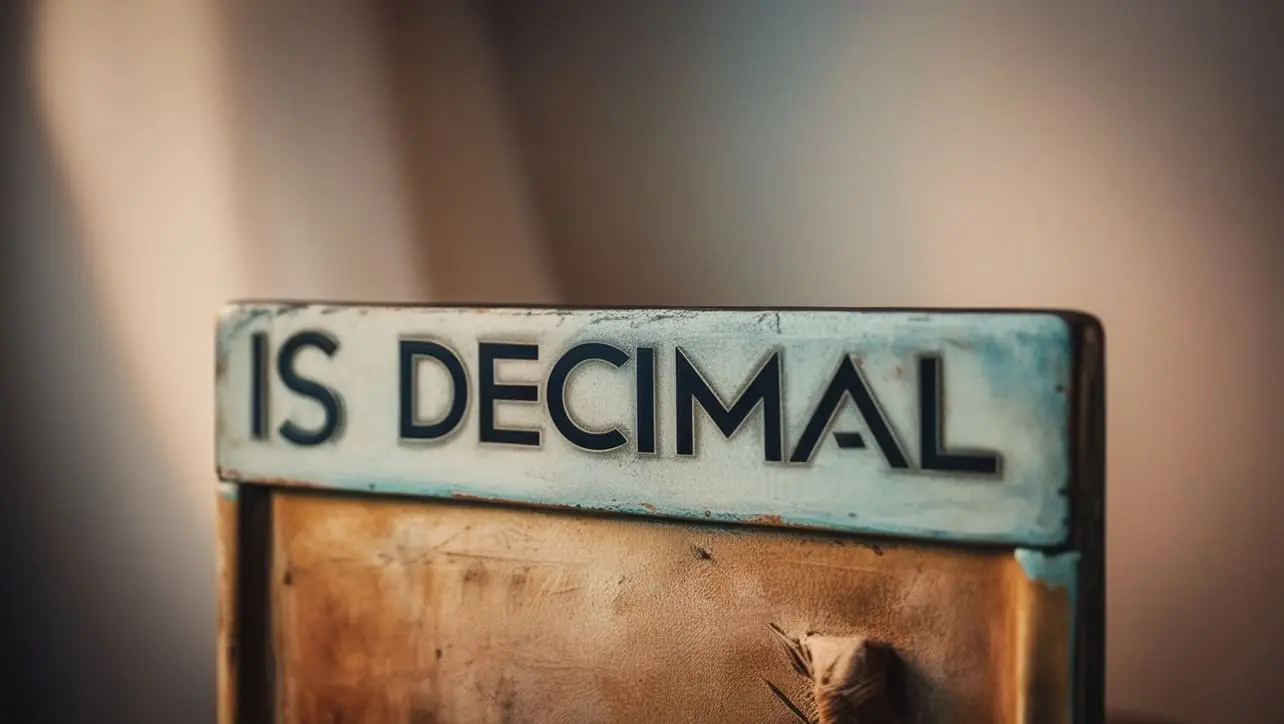
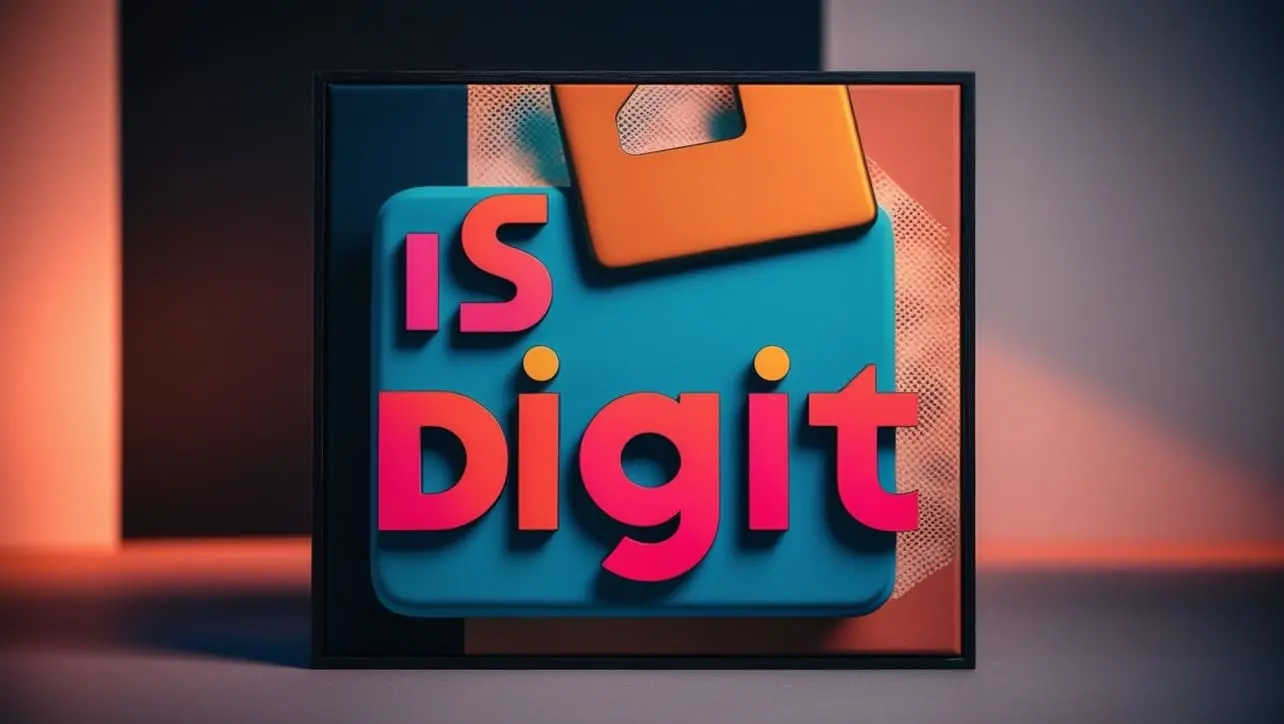
If you have any doubts regarding this article (Python Program to Check Armstrong Number), please comment here. I will help you immediately.