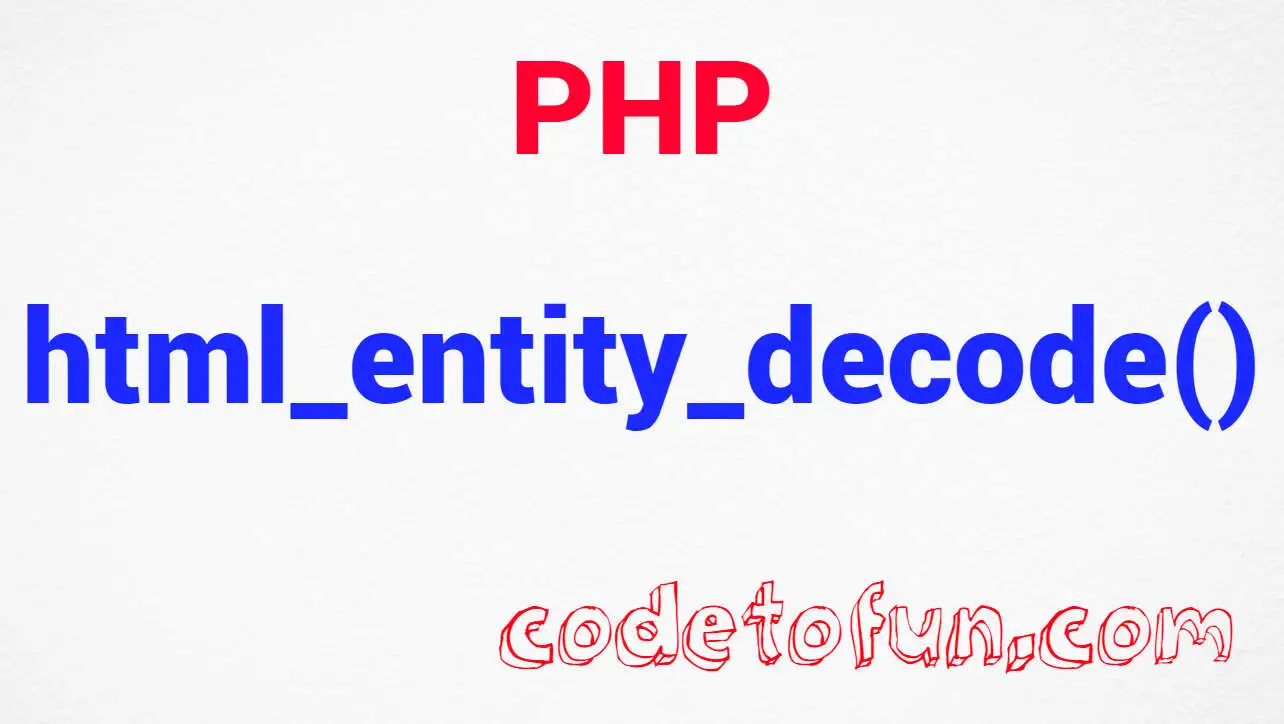
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to find Sum of Array
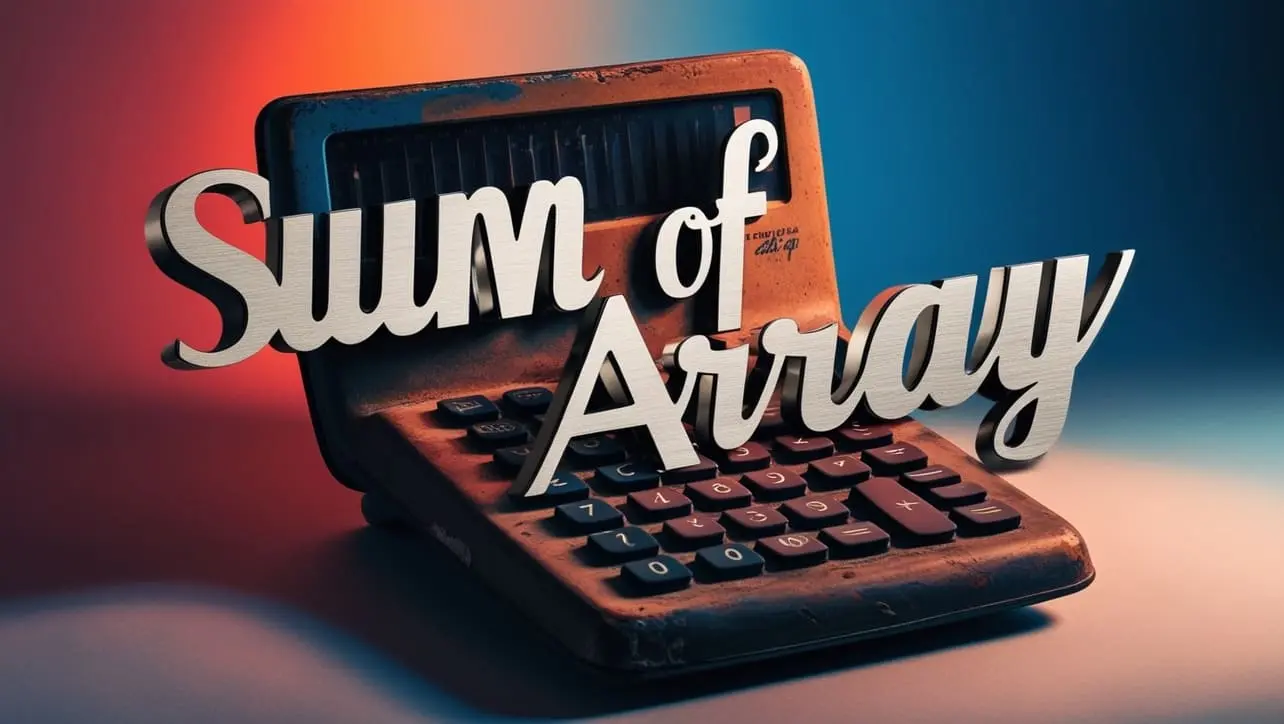
Photo Credit to CodeToFun
π Introduction
In the world of programming, array manipulation is a common and essential task. One frequent operation is finding the sum of elements in an array.
In this tutorial, we will explore a simple yet effective php program that calculates the sum of elements in an array.
π Example
Let's dive into the php code that accomplishes this task.
<?php
// Function to find the sum of array elements
function findSumOfArray($arr)
{
$sum = 0;
foreach ($arr as $element)
{
$sum += $element;
}
return $sum;
}
// Driver program
// Replace these values with your array elements
$array = [1, 2, 3, 4, 5];
// Call the function to find the sum
$sum = findSumOfArray($array);
// Print the result
echo "Sum of the array elements: $sum\n";
?>
π» Testing the Program
To test the program with different array elements, simply replace the values in the array initialization.
Sum of the array elements: 15
Compile and run the program to see the sum of the array elements.
π§ How the Program Works
- The program defines a function findSumOfArray that takes an array as input and returns the sum of its elements.
- Inside the function, it iterates through the array using a foreach loop and accumulates the sum.
- The main part of the script initializes an array with sample values.
- It then calls the findSumOfArray function and prints the result.
π§ Understanding the Concept of Sum of Array
Before diving into the code, it's essential to understand the concept of finding the sum of array elements. The sum of an array is obtained by adding up all the elements it contains.
For example, in the array {1, 2, 3, 4, 5}, the sum is 1 + 2 + 3 + 4 + 5 = 15.
π’ Optimizing the Program
While the provided program is straightforward, you can explore optimizations such as handling large arrays, incorporating error checking, or parallelizing the sum calculation for improved performance.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
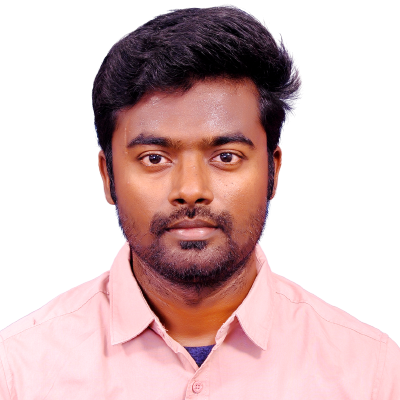
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to find Sum of Array), please comment here. I will help you immediately.