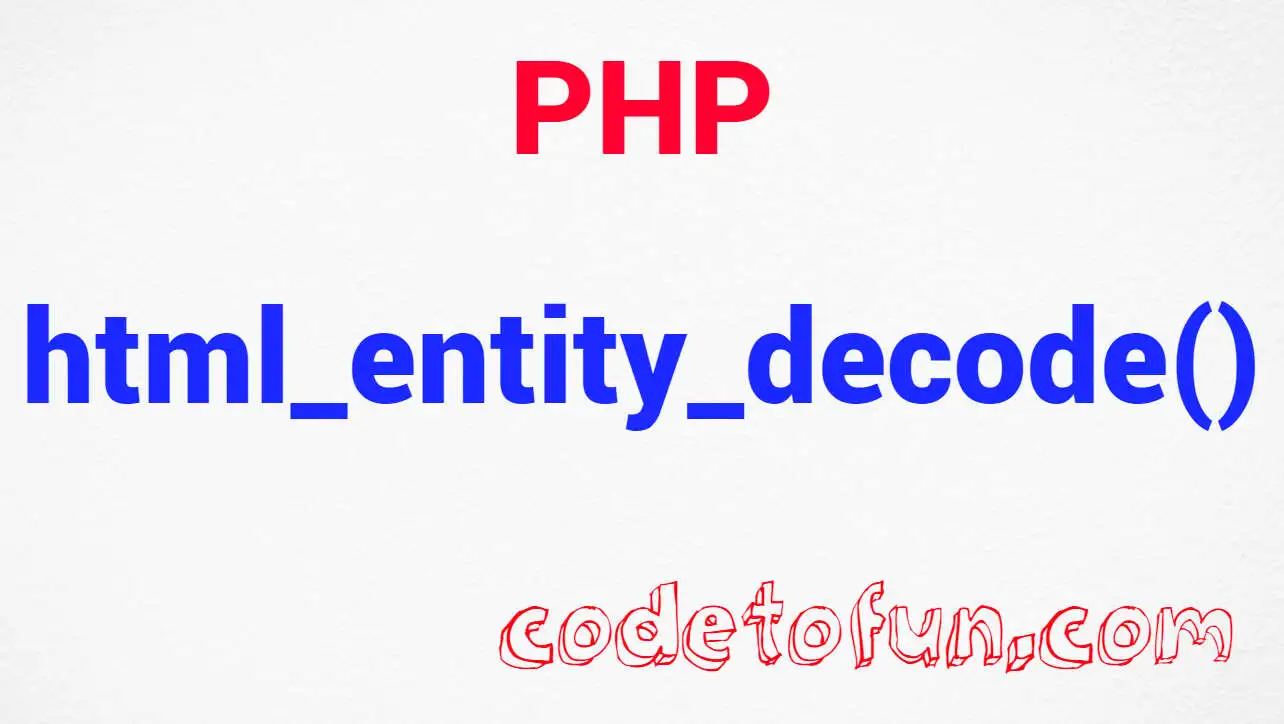
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Check Pronic Number
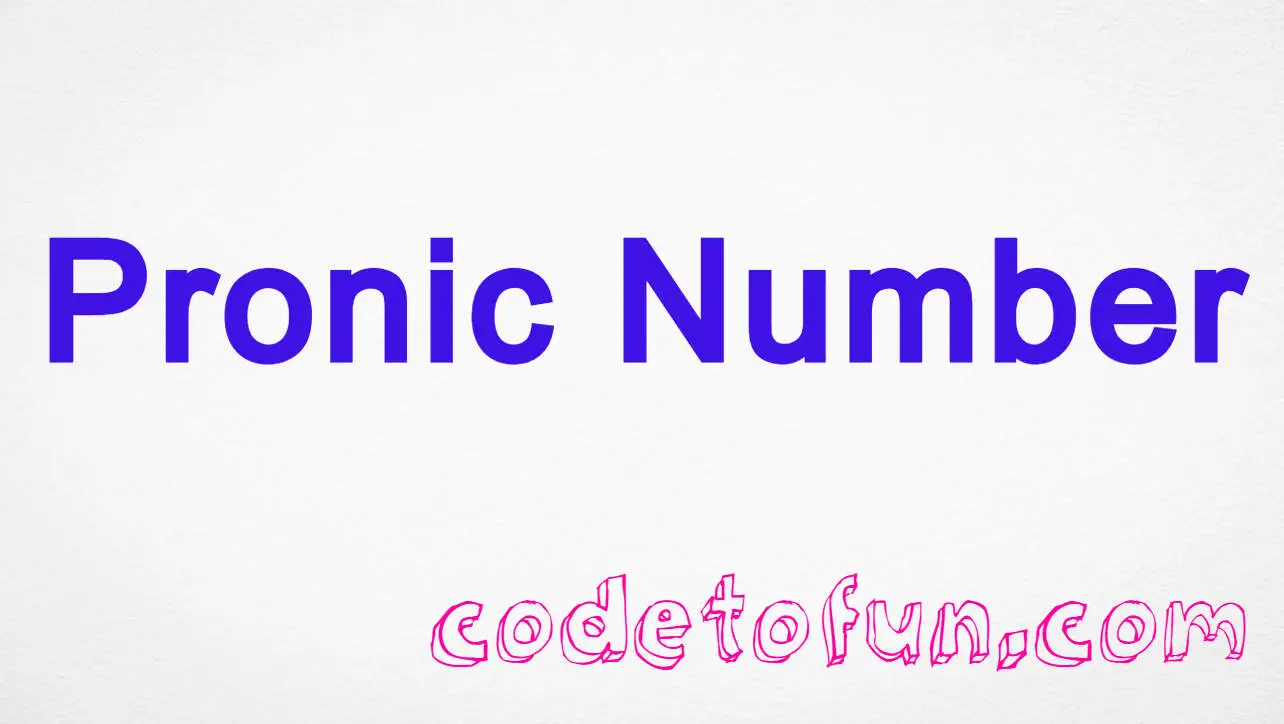
Photo Credit to CodeToFun
π Introduction
In the realm of programming, mathematical patterns and properties often become interesting challenges. One such intriguing concept is the "Pronic Number."
A pronic number, also known as a rectangular number, oblong number, or heteromecic number, is the product of two consecutive integers.
In this tutorial, we will delve into a PHP program designed to check whether a given number is a pronic number or not.
π Example
Now, let's explore the PHP code that checks whether a given number is a pronic number or not.
<?php
// Function to check if a number is a pronic number
function isPronic($num)
{
$sqrtNum = sqrt($num);
return ($sqrtNum * ($sqrtNum + 1) == $num);
}
// Driver program
// Replace this value with your desired number
$number = 12;
// Check if the number is a pronic number
if (isPronic($number))
{
echo "$number is a pronic number.\n";
}
else
{
echo "$number is not a pronic number.\n";
}
?>
π» Testing the Program
To test the program with different numbers, simply replace the value of $number in the code.
12 is a pronic number.
Run the script to check if the given number is a pronic number.
π§ How the Program Works
- The program defines a function isPronic that takes a number as input and returns true if the number is a pronic number and false otherwise.
- Inside the function, it calculates the square root of the input number.
- It checks whether the product of the square root and the square root incremented by 1 equals the input number. If yes, the number is a pronic number.
- The driver program tests the function with a sample number and prints the result.
π Between the Given Range
Let's take a look at the php code that checks for pronic numbers in the range from 1 to 20.
<?php
// Function to check if a number is pronic
function isPronic($num)
{
$root = (int)sqrt($num);
return ($root * ($root + 1) == $num);
}
// Display pronic numbers in the range 1 to 20
echo "Pronic Numbers in the range 1 to 20:\n";
for ($i = 1;$i <= 20;++$i)
{
if (isPronic($i))
{
echo $i . " ";
}
}
?>
π» Testing the Program
Pronic Numbers in the range 1 to 20: 2 6 12 20
The provided script checks and displays pronic numbers in the range from 1 to 20. No additional input is required. Simply run the script to see the pronic numbers.
π§ How the Program Works
- The program defines a function isPronic that checks if a given number is a pronic number.
- Inside the function, it calculates the square root of the number and checks if the product of the square root and the square root plus one equals the original number.
- The program then iterates through the range from 1 to 20, calling the isPronic function for each number.
- If a number is pronic, it is displayed.
π§ Understanding the Concept of Pronic Numbers
Before delving into the code, let's take a moment to understand the concept of pronic numbers.
A pronic number is a product of two consecutive integers, and it has applications in various mathematical and geometric contexts.
A pronic number, denoted by Pn, is a number of the form Pn=n Γ (n+1) where n is a non-negative integer. The sequence of pronic numbers starts as 0, 2, 6, 12, 20, and so on.
For example, let's take n = 3. The corresponding pronic number would be P3 = 3x(3+1) = 12.
π’ Optimizing the Program
While the provided program is effective, consider exploring ways to optimize the computation of pronic numbers for larger inputs.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
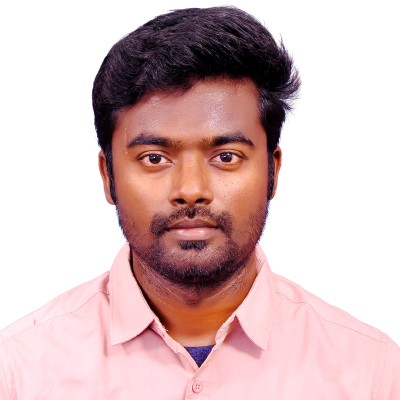
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Check Pronic Number), please comment here. I will help you immediately.