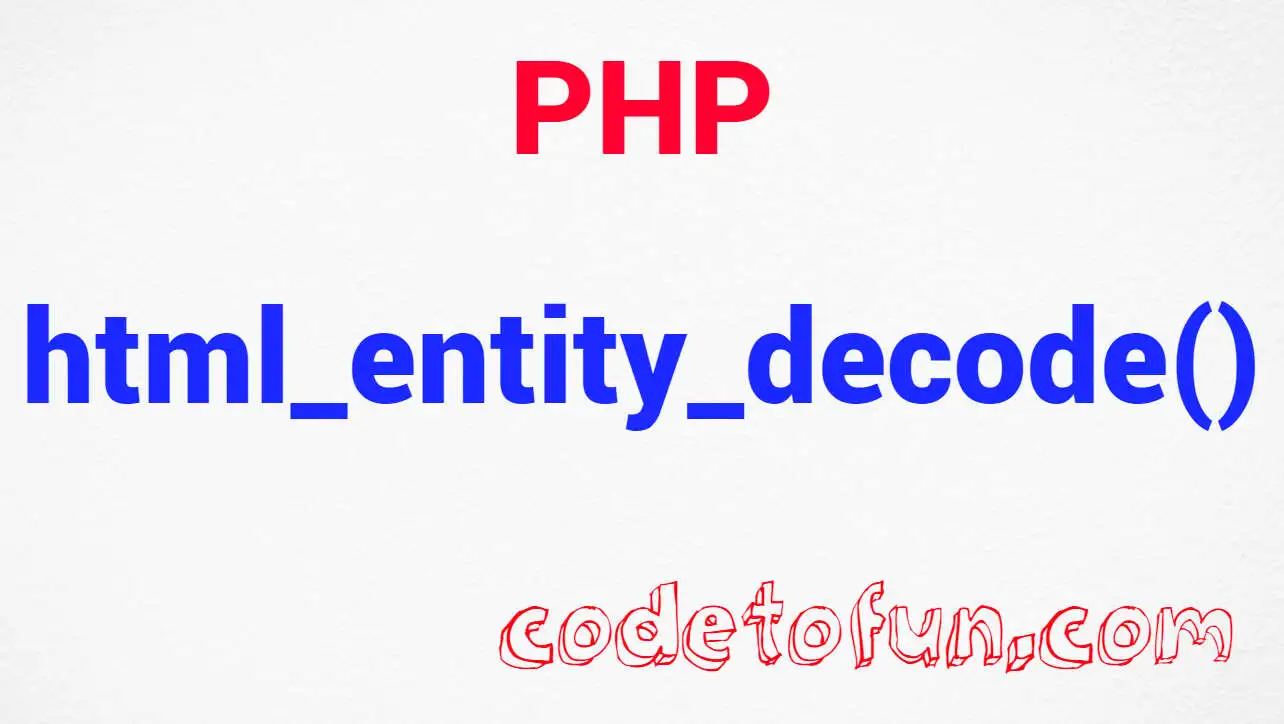
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Check Perfect Number
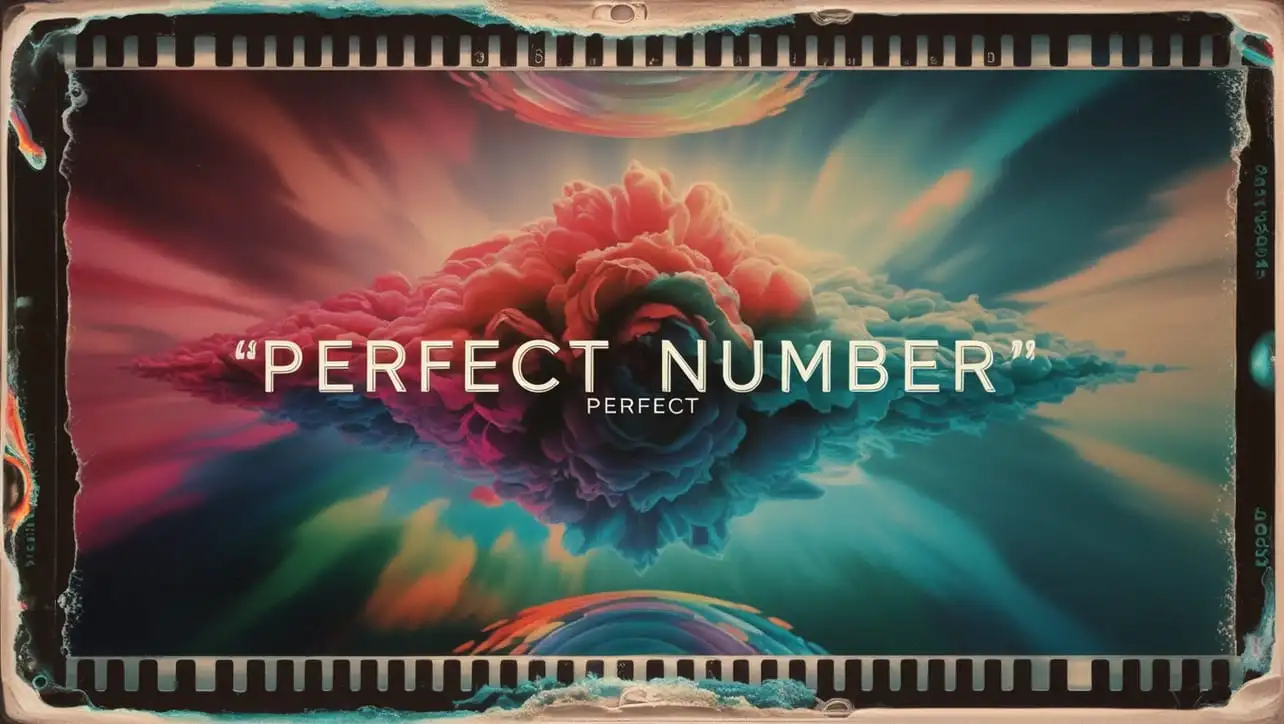
Photo Credit to CodeToFun
π Introduction
In the realm of programming, exploring the properties of numbers is a fascinating task. One such special type of number is a perfect number.
A perfect number is a positive integer that is equal to the sum of its proper divisors (excluding itself).
In this tutorial, we will explore a PHP program designed to check whether a given number is a perfect number.
π Example
Let's delve into the PHP code that accomplishes this task.
<?php
// Function to check if a number is a perfect number
function isPerfectNumber($number)
{
$sumOfDivisors = 0;
// Iterate through potential divisors up to half of the number
for ($i = 1;$i <= $number / 2;++$i)
{
// If $i is a divisor, add it to the sumOfDivisors
if ($number % $i === 0)
{
$sumOfDivisors += $i;
}
}
// If the sum of divisors equals the original number, it is a perfect number
return $sumOfDivisors === $number;
}
// Driver program
// Replace this value with the number you want to check
$number = 28;
// Call the function to check if the number is a perfect number
if (isPerfectNumber($number))
{
echo "$number is a perfect number.\n";
}
else
{
echo "$number is not a perfect number.\n";
}
?>
π» Testing the Program
To test the program with different numbers, modify the value of $number in the code.
28 is a perfect number.
Run the script to check if the specified number is a perfect number.
π§ How the Program Works
- The program defines a function isPerfectNumber that takes an integer $number as input and returns true if the number is a perfect number, and false otherwise.
- The function iterates through potential divisors up to half of the number and checks if each divisor divides evenly. If so, it adds the divisor to the sum of divisors.
- If the sum of divisors equals the original number, the function returns true, indicating a perfect number.
- Inside the main program, replace the value of $number with the desired number you want to check.
- The program calls the isPerfectNumber function and prints the result.
π Between the Given Range
Let's delve into the php code that identifies perfect numbers in the specified range.
<?php
// Function to check if a number is perfect
function isPerfect($number)
{
$sum = 0;
for ($i = 1;$i <= $number / 2;++$i)
{
if ($number % $i == 0)
{
$sum += $i;
}
}
return $sum == $number;
}
// Display perfect numbers in the range 1 to 50
echo "Perfect Numbers in the range 1 to 50:\n";
for ($i = 2;$i <= 50;++$i)
{
if (isPerfect($i))
{
echo $i . " ";
}
}
?>
π» Testing the Program
Perfect Numbers in the range 1 to 50: 6 28
Run the script to see the perfect numbers in the range from 1 to 50.
π§ How the Program Works
- The program defines a function isPerfect that checks if a given number is perfect.
- Inside the function, it iterates through the numbers from 1 to half of the given number and accumulates the sum of proper divisors.
- The main section of the program displays perfect numbers in the range from 1 to 50 by calling the isPerfect function for each number.
π§ Understanding the Concept of Perfect Number
Before delving into the code, let's understand the concept of perfect numbers. A perfect number is a positive integer that is equal to the sum of its proper divisors (excluding itself).
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing alternative approaches or optimizations for checking perfect numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
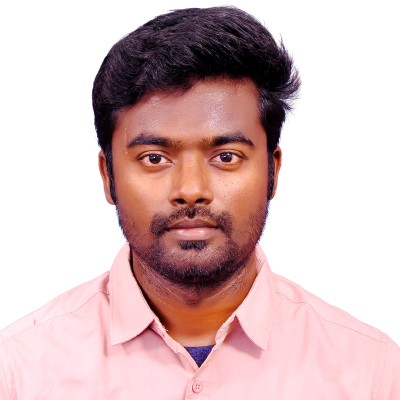
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Check Perfect Number), please comment here. I will help you immediately.