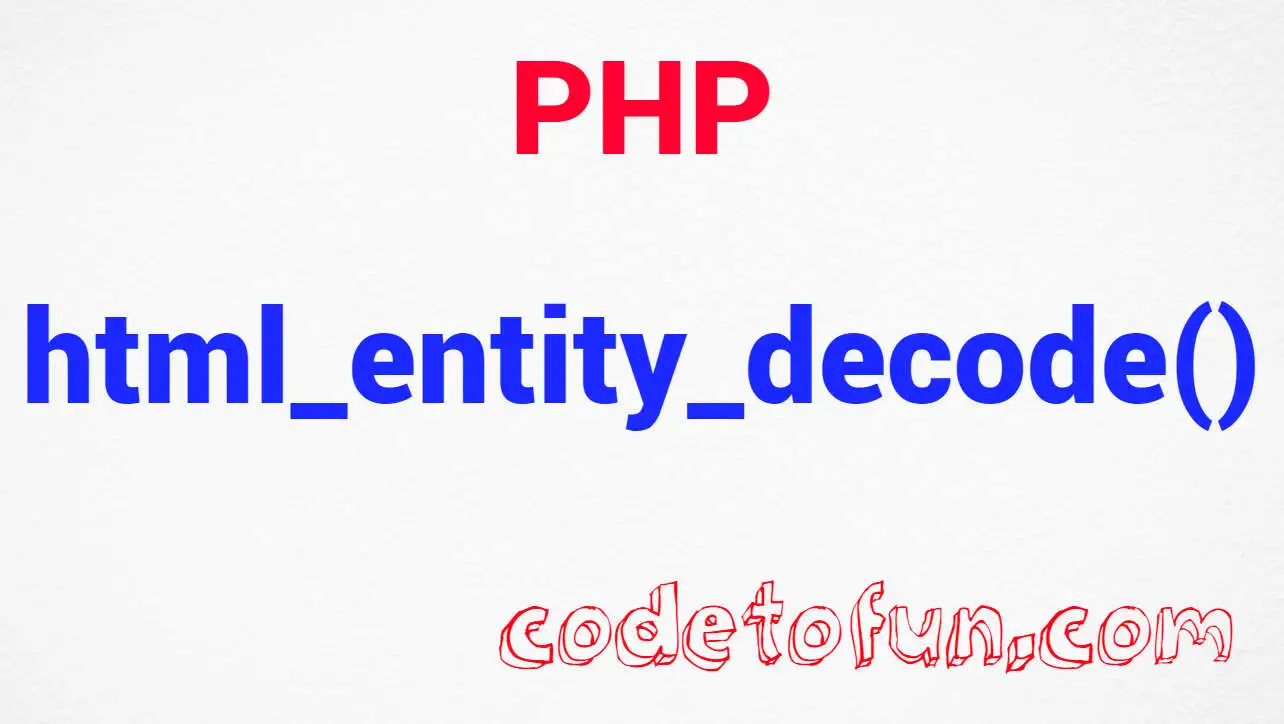
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to find Number Combination
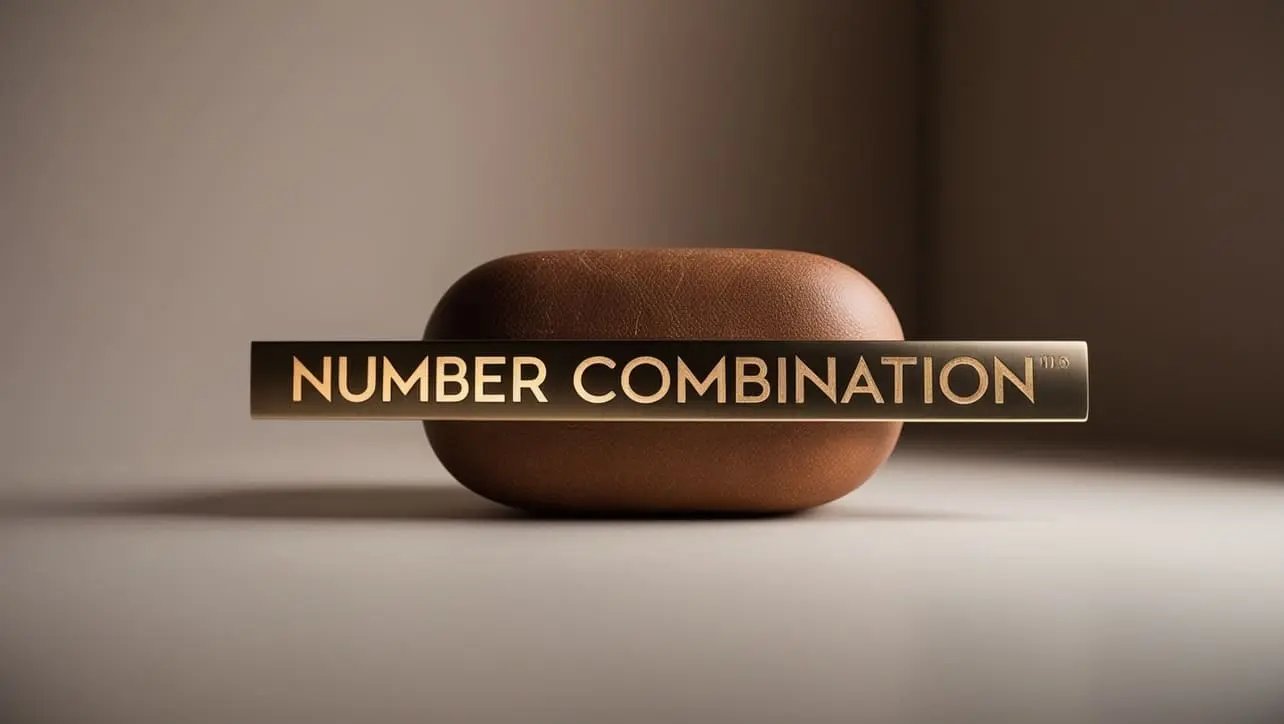
Photo Credit to CodeToFun
π Introduction
In the realm of programming, creating combinations of numbers is a common and useful task. A combination is a selection of items from a collection, where the order of selection does not matter.
In this tutorial, we'll explore a PHP program that generates combinations of two specified numbers within a given range.
π Example
Let's take a look at the PHP code that achieves this functionality.
<?php
// Function to calculate the number of digits in a number
function countDigits($num)
{
$count = 0;
while ($num != 0)
{
$num = (int)($num / 10);
++$count;
}
return $count;
}
// Function to generate combinations of two numbers up to a specified limit
function generateCombinations($number1, $number2, $limit)
{
echo "List of combinations of $number1 and $number2 up to $limit:\n";
for ($i = 1;$i <= $limit;++$i)
{
$currentNumber = $i;
// Check if the current number contains only the specified digits
while ($currentNumber > 0)
{
$digit = $currentNumber % 10;
if ($digit != $number1 && $digit != $number2)
{
break;
}
$currentNumber = (int)($currentNumber / 10);
}
// If the current number contains only the specified digits, print it
if ($currentNumber == 0)
{
echo "$i ";
}
}
echo "\n";
}
// Driver program
// Replace these values with your desired numbers and limit
$targetNumber1 = 4;
$targetNumber2 = 8;
$combinationLimit = 500;
// Call the function to generate combinations
generateCombinations($targetNumber1, $targetNumber2, $combinationLimit);
?>
π» Testing the Program
To test the program with different values, replace the values of $targetNumber1, $targetNumber2, and $combinationLimit in the code.
List of combinations of 4 and 8 up to 500: 4 8 44 48 84 88 444 448 484 488
Run the script to see the list of combinations.
π§ How the Program Works
- The program defines a function countDigits to calculate the number of digits in a given number.
- The function generateCombinations generates combinations of two specified numbers up to a given limit.
- Inside the function, it iterates through numbers from 1 to the specified limit.
- For each iteration, it checks if the current number contains only the specified digits.
- If the condition is met, the current number is a combination, and it is printed.
π§ Understanding the Concept of Number Combination
Before delving into the code, let's take a moment to understand the concept of combinations. In mathematics, a combination is a selection of items from a collection, where the order of selection does not matter.
For example, consider the specified numbers as 4 and 8. The combinations of 4 and 8 up to 500 include 4, 8, 44, 48, 84, 88, 444, 448, 484, and 488.
π’ Optimizing the Program
While the provided program is effective, you can explore and implement optimizations based on specific requirements or constraints.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
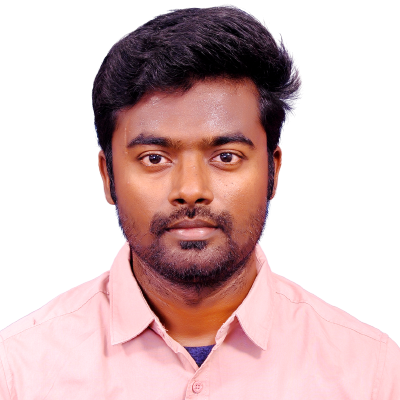
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to find Number Combination), please comment here. I will help you immediately.