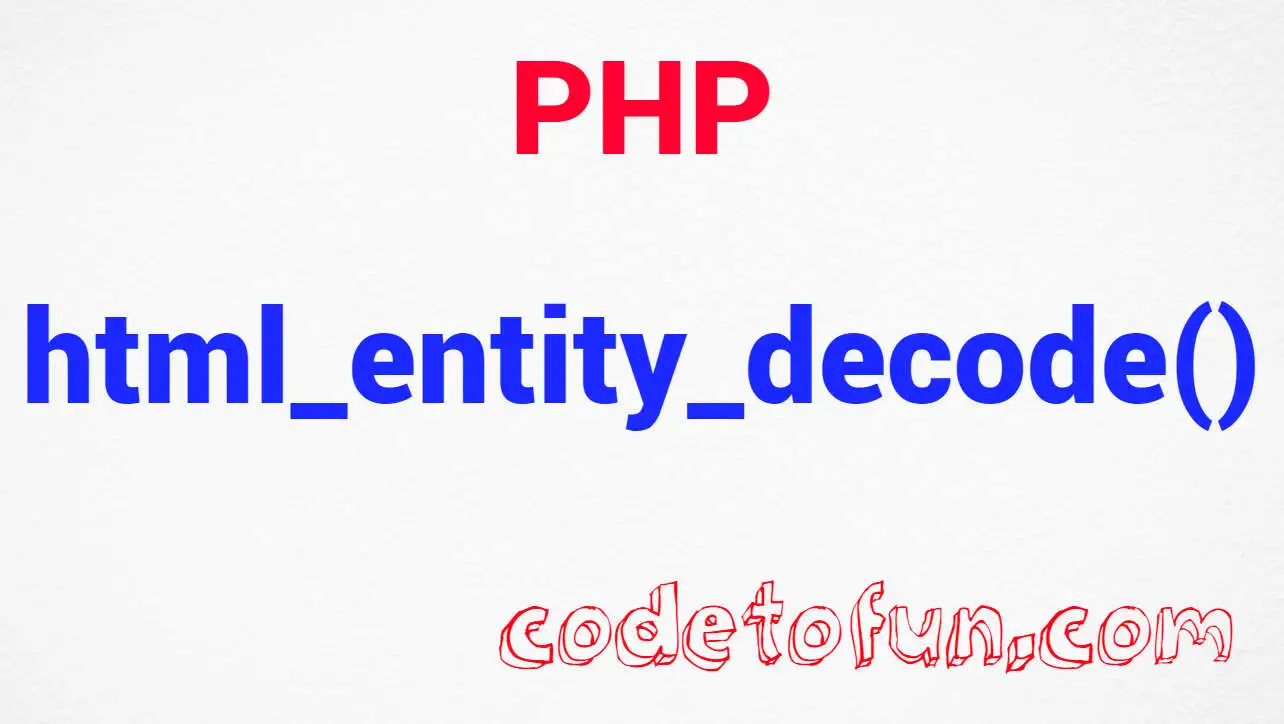
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Perform Matrix Multiplication
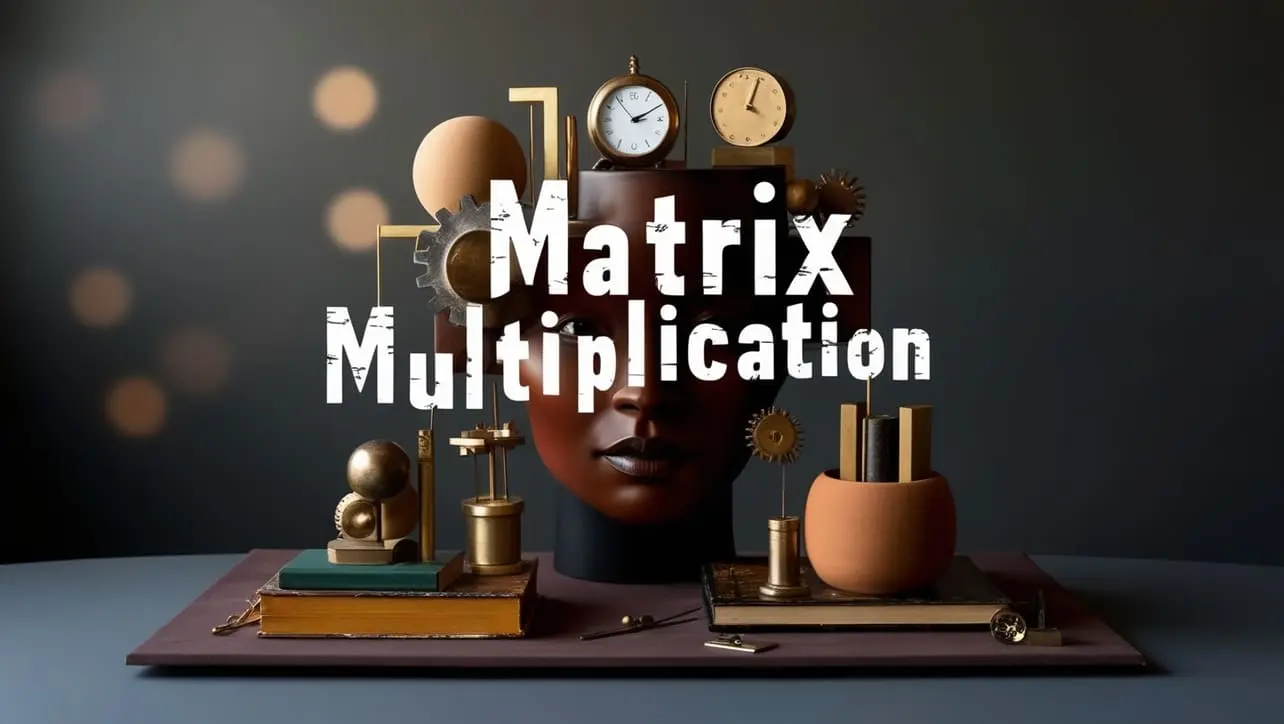
Photo Credit to CodeToFun
π Introduction
Matrix multiplication is a fundamental operation in linear algebra and computer science. It involves multiplying two matrices to produce a third matrix.
In this tutorial, we will explore a php program that performs matrix multiplication.
We'll break down the logic step by step and provide a sample implementation.
π Example
Let's dive into the php code that performs matrix multiplication.
<?php
// Function to perform matrix multiplication
function multiplyMatrices($firstMatrix, $secondMatrix)
{
$result = array_fill(0, count($firstMatrix) , array_fill(0, count($secondMatrix[0]) , 0));
// Perform matrix multiplication
for ($i = 0;$i < count($firstMatrix);++$i)
{
for ($j = 0;$j < count($secondMatrix[0]);++$j)
{
for ($k = 0;$k < count($secondMatrix);++$k)
{
$result[$i][$j] += $firstMatrix[$i][$k] * $secondMatrix[$k][$j];
}
}
}
return $result;
}
// Function to display a matrix
function displayMatrix($matrix)
{
foreach ($matrix as $row)
{
echo implode("\t", $row) . PHP_EOL;
}
}
// Sample Input (3x3 Matrices)
$firstMatrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
$secondMatrix = [[9, 8, 7], [6, 5, 4], [3, 2, 1]];
// Call the function to perform matrix multiplication
$resultMatrix = multiplyMatrices($firstMatrix, $secondMatrix);
// Display the matrices
echo "First Matrix:" . PHP_EOL;
displayMatrix($firstMatrix);
echo PHP_EOL . "Second Matrix:" . PHP_EOL;
displayMatrix($secondMatrix);
echo PHP_EOL . "Result Matrix:" . PHP_EOL;
displayMatrix($resultMatrix);
?>
π» Testing the Program
Feel free to replace the sample matrices with your own 3x3 matrices in the main function to test the program with different input.
First Matrix: 1 2 3 4 5 6 7 8 9 Second Matrix: 9 8 7 6 5 4 3 2 1 Result Matrix: 30 24 18 84 69 54 138 114 90
Run the program to see the result of the matrix multiplication.
π§ How the Program Works
- The program defines a function multiplyMatrices that takes two matrices ($firstMatrix and $secondMatrix) and computes their product, storing the result in the $result matrix.
- It uses three nested loops to perform the matrix multiplication.
- The displayMatrix function is used to display the contents of a matrix.
- Sample input matrices ($firstMatrix and $secondMatrix) are provided, and the program calls the multiplication function.
- The program then displays the original matrices and the result matrix.
π§ Understanding the Concept of Matrix Multiplication
Matrix multiplication is defined as follows: given two matrices A (of dimensions m x n) and B (of dimensions n x p), the product matrix C (of dimensions m x p) is obtained by multiplying each element of a row in matrix A by the corresponding element of a column in matrix B and summing up the results.
π Conclusion
Matrix multiplication is a powerful operation used in various fields, including graphics, physics simulations, and machine learning.
Understanding the logic behind matrix multiplication and implementing it in php provides a foundational understanding of linear algebra in computer science.
Feel free to experiment with different matrix sizes and values to further explore the capabilities of this program. Happy coding!
π¨βπ» Join our Community:
Author
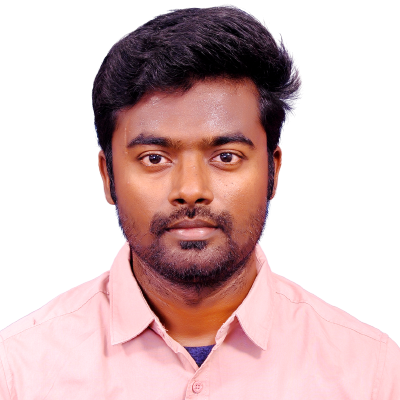
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Perform Matrix Multiplication), please comment here. I will help you immediately.