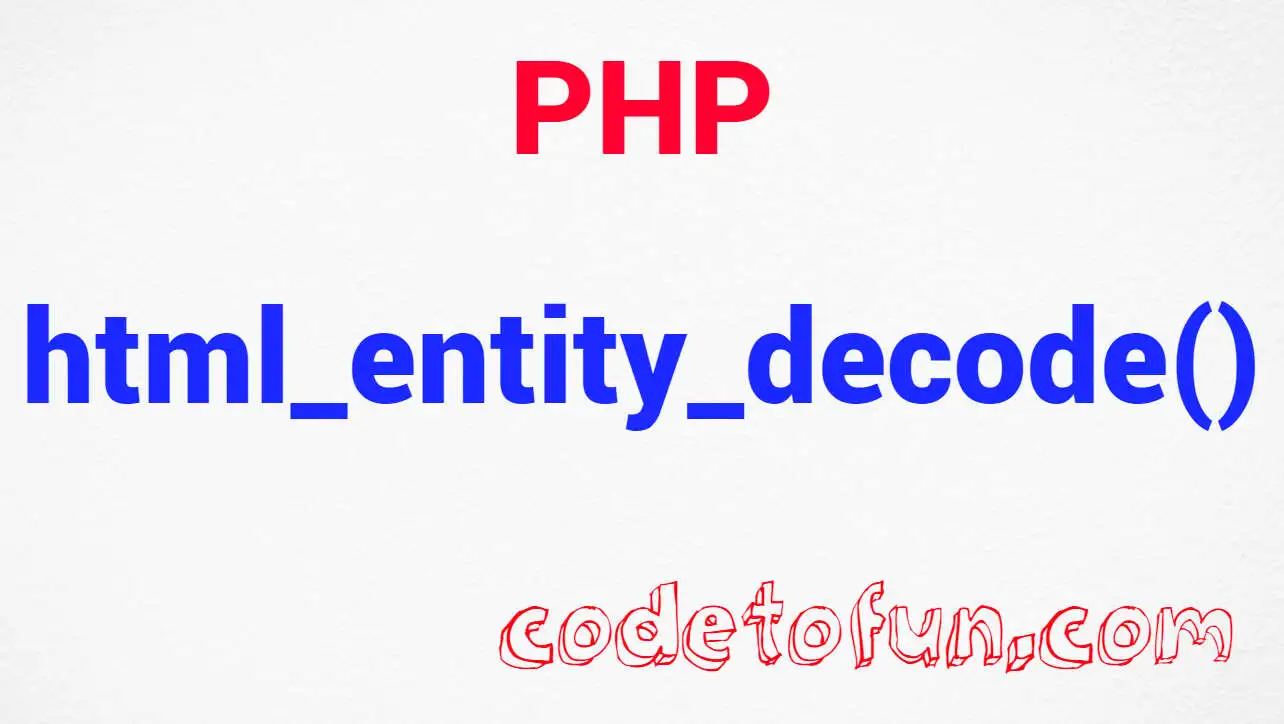
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Check Happy Number
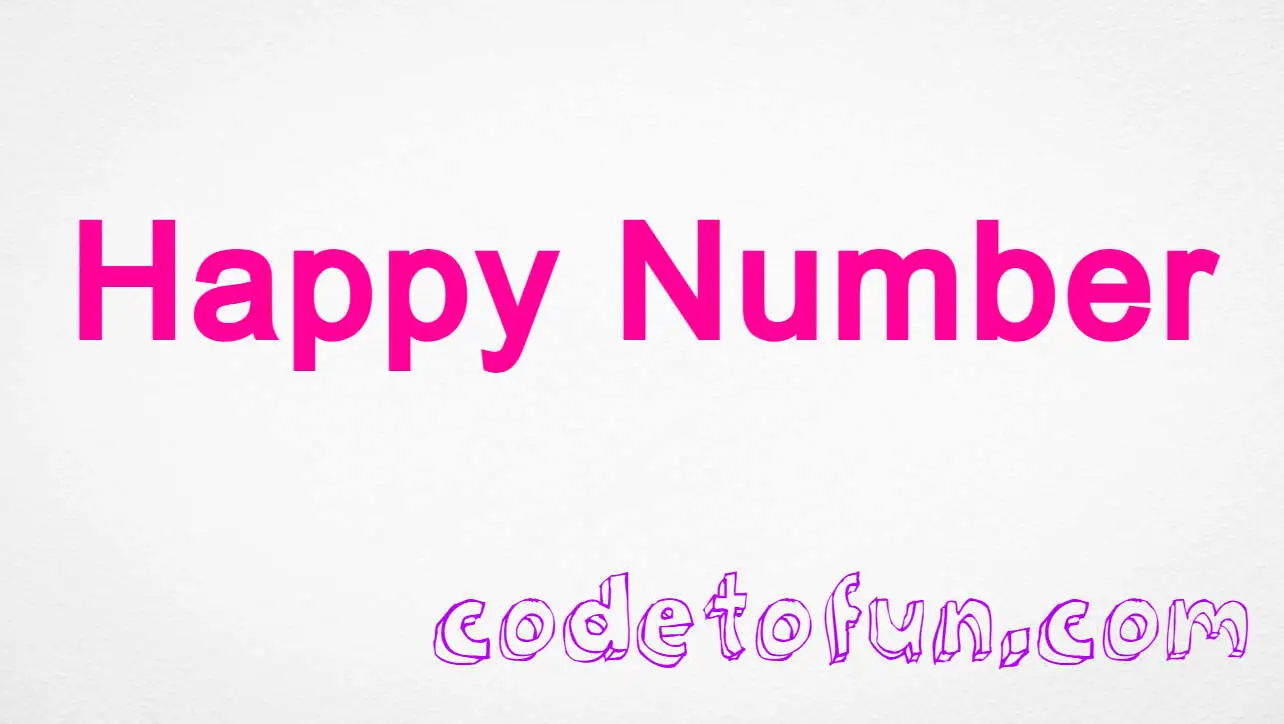
Photo Credit to CodeToFun
π Introduction
In the domain of programming, certain mathematical properties and patterns are often explored. One such interesting concept is the notion of "happy numbers."
In this tutorial, we'll delve into a PHP program designed to check whether a given number is a happy number.
π Example
Let's take a look at the PHP code that checks whether a given number is a happy number or not.
<?php
// Function to calculate the sum of squares of digits
function sumOfSquares($n)
{
$sum = 0;
while ($n > 0)
{
$digit = $n % 10;
$sum += $digit * $digit;
$n /= 10;
}
return $sum;
}
// Function to check if a number is a happy number
function isHappyNumber($n)
{
$slow = $n;
$fast = $n;
do
{
$slow = sumOfSquares($slow);
$fast = sumOfSquares(sumOfSquares($fast));
}
while ($slow != $fast);
return $slow == 1;
}
// Replace this value with your desired number
$number = 19;
// Check if the number is a happy number
if (isHappyNumber($number))
{
echo "$number is a Happy Number.\n";
}
else
{
echo "$number is not a Happy Number.\n";
}
?>
π» Testing the Program
To test the program with a different number, replace the value of $number in the code.
19 is a Happy Number.
Run the script to check if the number is a happy number.
π§ How the Program Works
- The program defines a function sumOfSquares to calculate the sum of the squares of digits of a given number.
- It then defines a function isHappyNumber that checks whether a given number is a happy number using Floyd's cycle detection algorithm.
- The script tests the program with a sample number (in this case, 19).
π Between the Given Range
Let's take a look at the php code that checks for Happy Numbers in the specified range.
<?php
// Function to check if a number is happy
function isHappy($num)
{
while ($num != 1 && $num != 4)
{
$sum = 0;
while ($num)
{
$sum += ($num % 10) * ($num % 10);
$num /= 10;
}
$num = $sum;
}
return $num == 1;
}
// Function to find and display happy numbers in a given range
function findHappyNumbers($start, $end)
{
echo "Happy numbers in the range $start to $end:\n";
for ($i = $start;$i <= $end;++$i)
{
if (isHappy($i))
{
echo "$i ";
}
}
echo "\n";
}
// Driver program
// Define the range
$start = 1;
$end = 50;
// Call the function to find and display happy numbers
findHappyNumbers($start, $end);
?>
π» Testing the Program
The provided script checks for happy numbers within the range of 1 to 50. To test with a different range, modify the values of $start and $end in the code.
Happy numbers in the range 1 to 50: 1 7 10 13 19 23 28 31 32 44 49
Run the script to see the happy numbers in the specified range.
π§ How the Program Works
- The program defines a function isHappy that checks if a number is a happy number using the iterative process described earlier.
- The function findHappyNumbers iterates through the specified range and prints the happy numbers.
π§ Understanding the Concept of Happy Number
A happy number is a positive integer defined by the following process: Starting with any positive integer, replace the number by the sum of the squares of its digits, and repeat the process until the number equals 1, or it loops endlessly in a cycle that does not include 1.
For example, let's take the number 19:
- 12+92 = 82
- 82+22 = 68
- 62+82 = 100
- 12+02+02 = 1
In this case, 19 is a happy number because the process ends with 1.
π’ Optimizing the Program
While the provided program is effective, there are various ways to optimize and enhance it further. Consider exploring different algorithms or incorporating additional features based on your specific requirements.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
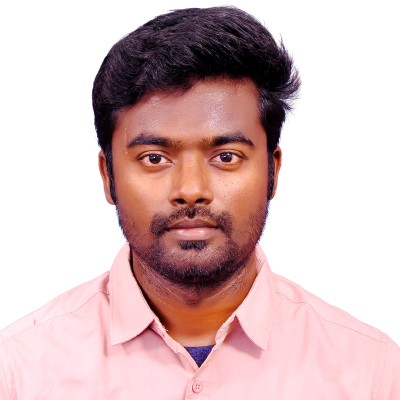
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Check Happy Number), please comment here. I will help you immediately.