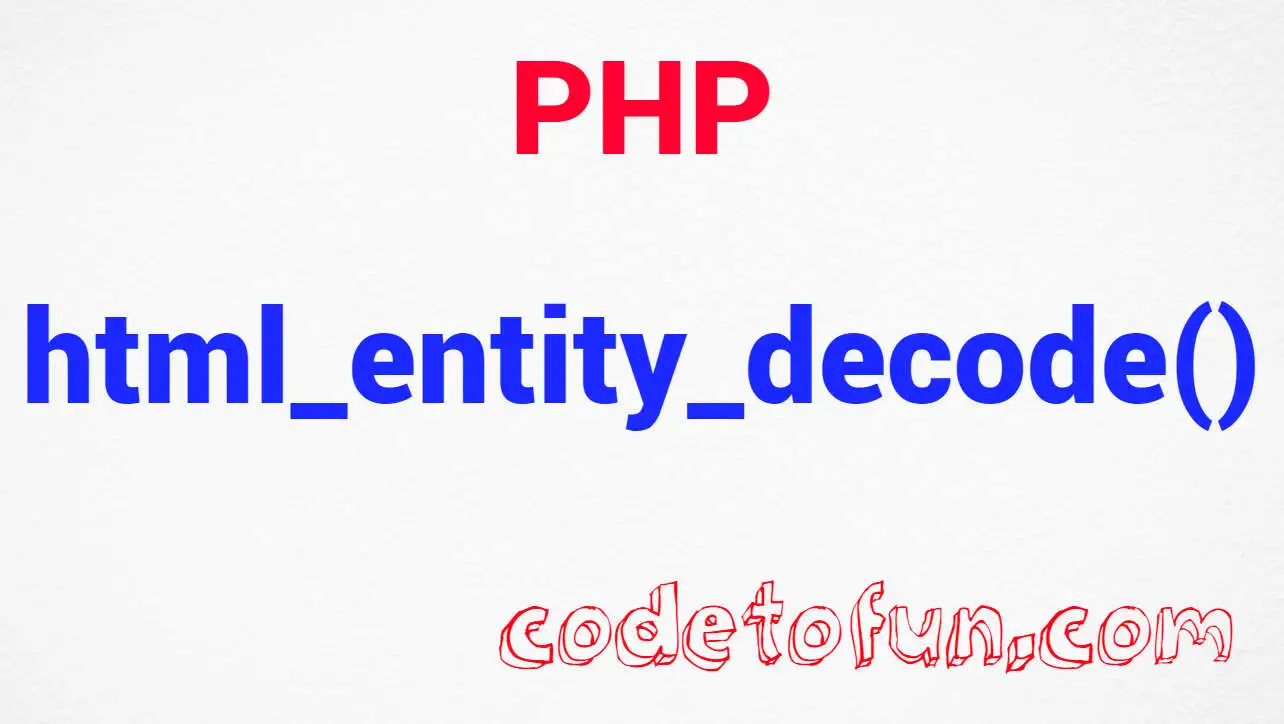
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to find Biggest of three numbers
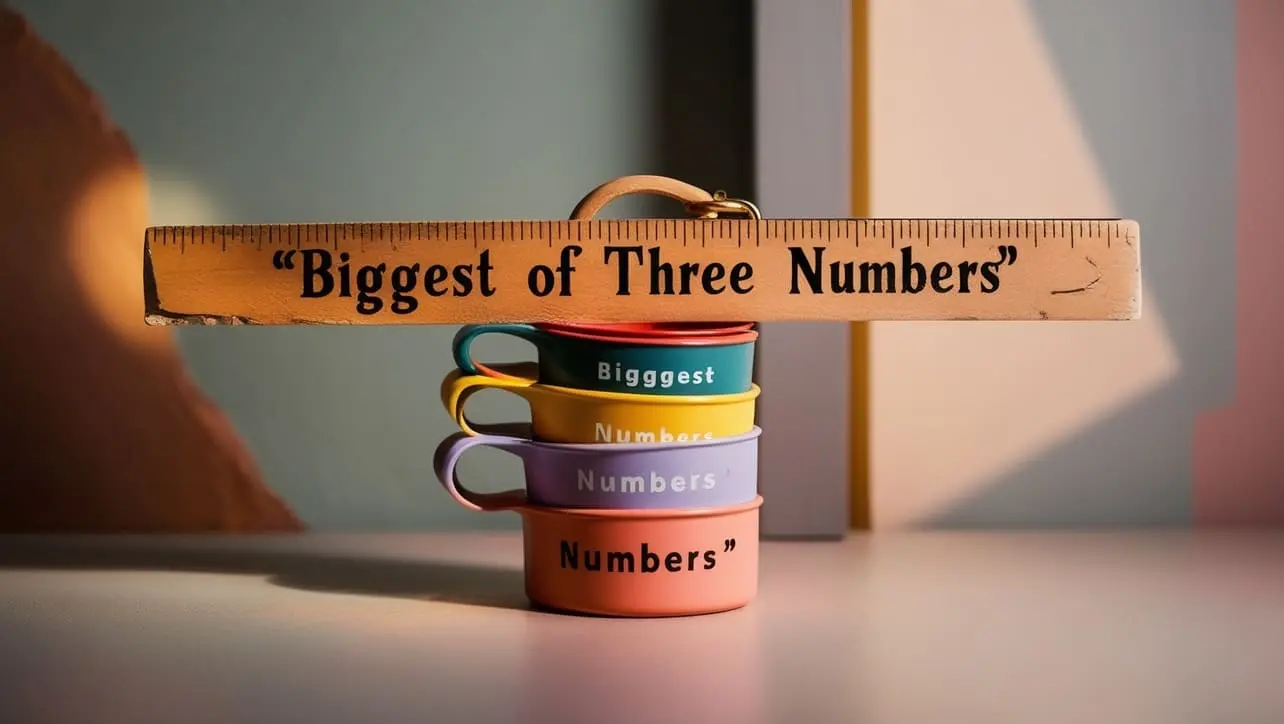
Photo Credit to CodeToFun
π Introduction
In the domain of programming, solving problems related to numerical comparisons is a common task. One such problem is determining the largest among three given numbers. This is a fundamental operation in many algorithms and applications.
In this tutorial, we will walk through a PHP program designed to find the biggest among three numbers. The program compares the values and identifies the maximum, showcasing a fundamental concept in programming logic.
π Example
Let's delve into the PHP code that accomplishes this task.
<?php
// Function to find the biggest of three numbers
function findBiggest($num1, $num2, $num3)
{
if ($num1 >= $num2 && $num1 >= $num3) {
return $num1;
} elseif ($num2 >= $num1 && $num2 >= $num3) {
return $num2;
} else {
return $num3;
}
}
// Driver program
// Replace these values with your desired numbers
$number1 = 14;
$number2 = 7;
$number3 = 22;
// Call the function to find the biggest number
$result = findBiggest($number1, $number2, $number3);
// Display the result
echo "The biggest number is: $result\n";
?>
π» Testing the Program
To test the program with different numbers, simply replace the values of number1, number2 and number3 in the main function.
The biggest number is: 22
π§ How the Program Works
- The program defines a function findBiggest that takes three numbers as input and returns the largest among them using conditional statements (if-elseif-else).
- Replace the values of $number1, $number2, and $number3 with the desired numbers.
- The program calls the findBiggest function, and the result is displayed.
π§ Understanding the Concept to find the Biggest of three Numbers
Before delving into the code, let's understand the concept behind finding the biggest of three numbers. The program uses conditional statements (if-else) to compare the values and determine which is the largest.
For example, consider the numbers 14, 7, and 22. The program evaluates and identifies 22 as the largest number.
π’ Optimizing the Program
While the provided program is straightforward, consider exploring and implementing alternative approaches or optimizations for determining the maximum of three numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
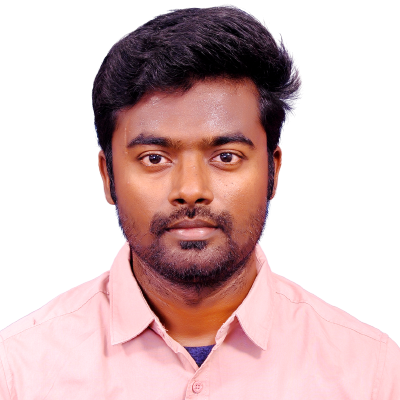
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to find Biggest of three numbers) please comment here. I will help you immediately.