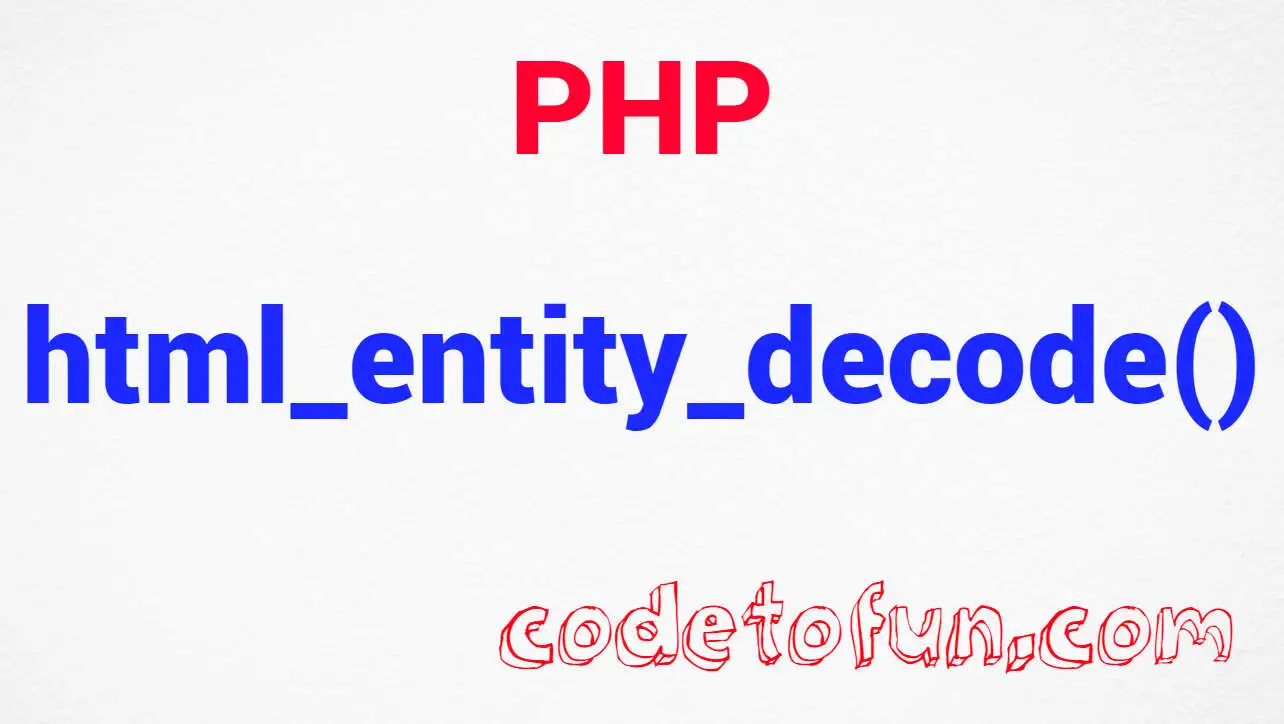
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Display Fibonacci Series
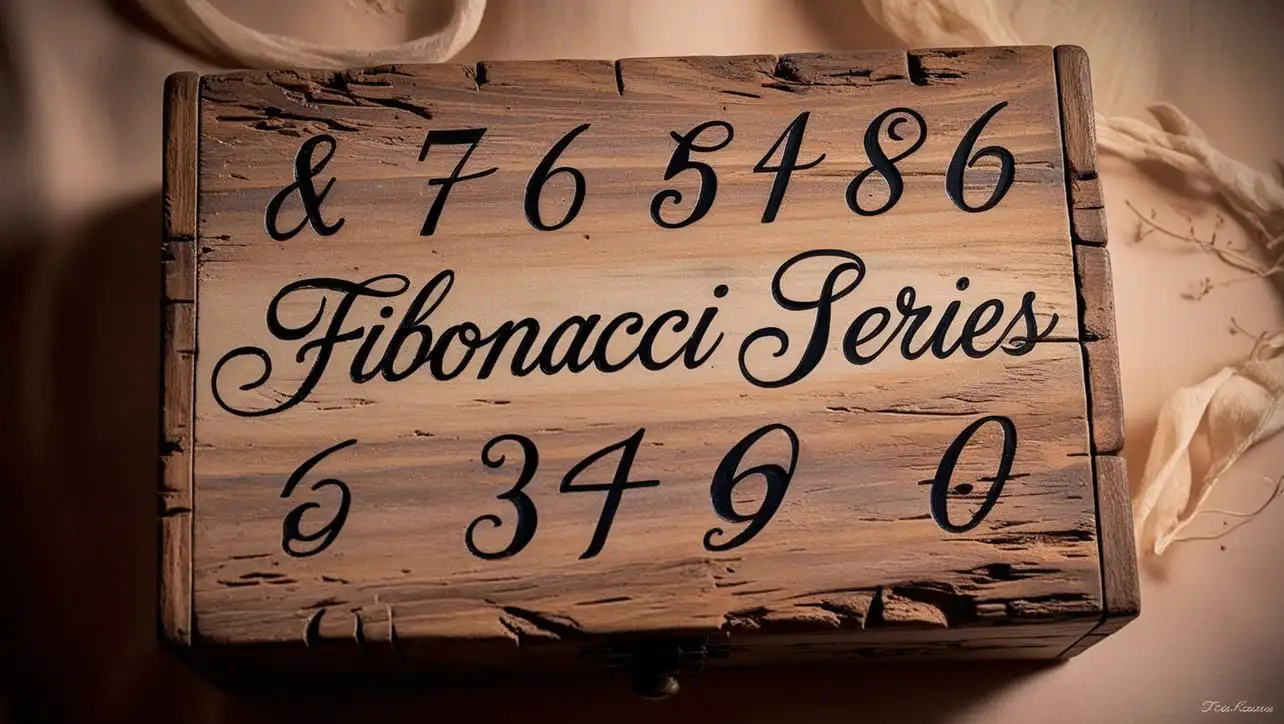
Photo Credit to CodeToFun
π Introduction
The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1.
This series has widespread applications in various fields, including mathematics and computer science.
In this tutorial, we'll explore a PHP program that generates and displays the Fibonacci series.
π Example
Let's dive into the PHP code that generates and displays the Fibonacci series.
<?php
// Function to display Fibonacci series up to n terms
function displayFibonacci($n)
{
$first = 0;
$second = 1;
echo "Fibonacci series up to $n terms: ";
for ($i = 0;$i < $n;++$i)
{
echo "$first, ";
$next = $first + $second;
$first = $second;
$second = $next;
}
echo "\n";
}
// Driver program
// Replace this value with the desired number of terms
$terms = 10;
// Call the function to display Fibonacci series
displayFibonacci($terms);
?>
π» Testing the Program
To test the program with a different number of terms, simply replace the value of the $terms variable.
Fibonacci series up to 10 terms: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Run the script to see the Fibonacci series up to the desired number of terms.
π§ How the Program Works
- The program defines a function displayFibonacci that takes the number of terms ($n) as input and echoes the Fibonacci series up to $n terms.
- Inside the function, it initializes variables $first and $second with 0 and 1, respectively.
- It then uses a loop to calculate and echo the Fibonacci series up to the specified number of terms.
- The loop updates the values of $first and $second in each iteration to generate the next Fibonacci number.
π§ Understanding the Concept of Fibonacci Series
The Fibonacci series starts with 0 and 1. Each subsequent number in the series is the sum of the two preceding ones. The series begins as follows:
0, 1, 1, 2, 3, 5, 8, 13, 21, ...
π’ Optimizing the Program
While the provided program is straightforward, consider exploring and implementing optimizations for larger Fibonacci series, such as using memoization techniques to avoid redundant calculations.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
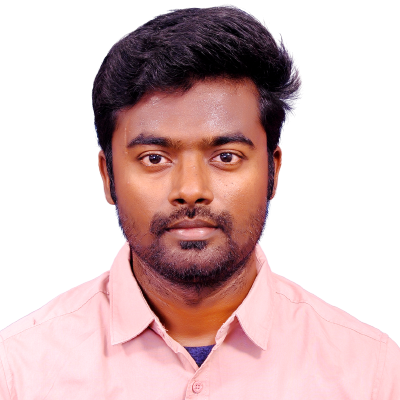
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Display Fibonacci Series), please comment here. I will help you immediately.