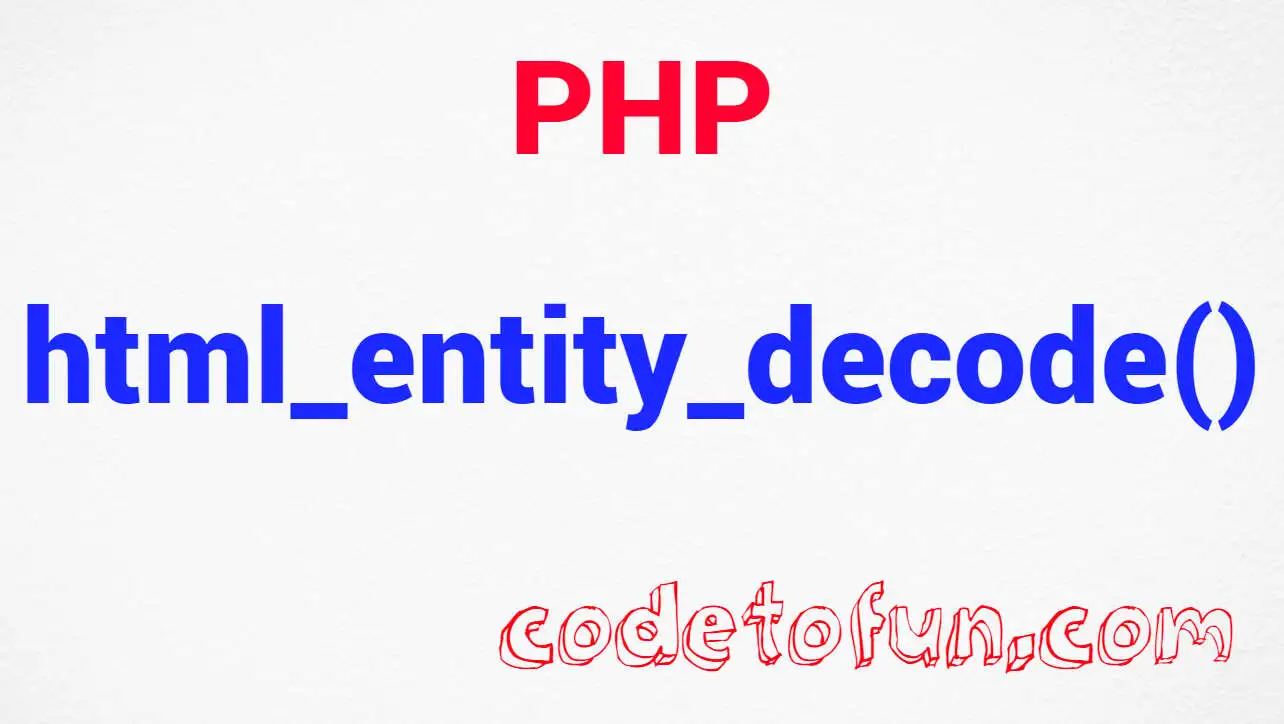
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Check Disarium Number
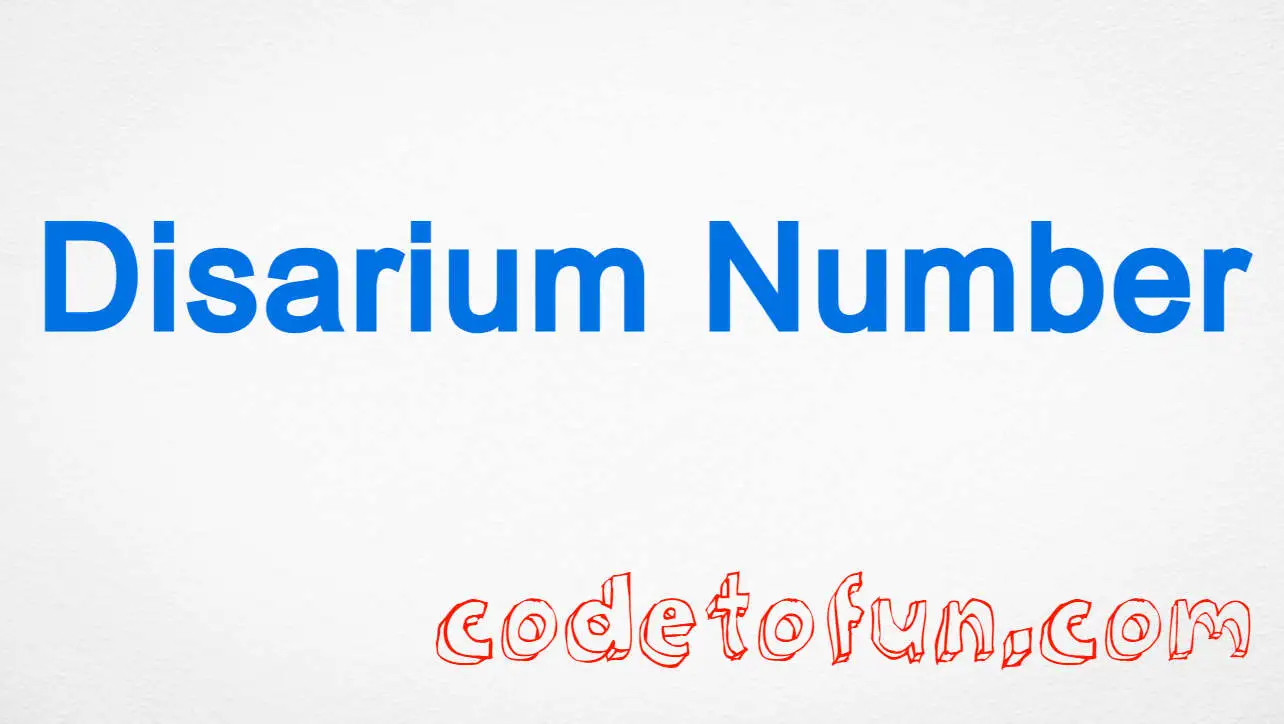
Photo Credit to CodeToFun
π Introduction
In the realm of programming, exploring mathematical patterns is a fascinating endeavor. One such numeric pattern is the Disarium number.
A Disarium number is a number defined by the sum of its digits each raised to the power of its respective position.
In this tutorial, we'll delve into a PHP program designed to check whether a given number is a Disarium number.
π Example
Let's explore the PHP code that checks whether a given number is a Disarium number.
<?php
// Function to count the number of digits in a given number
function countDigits($number)
{
$count = 0;
while ($number != 0)
{
$count++;
$number = (int)($number / 10);
}
return $count;
}
// Function to check if a number is a Disarium number
function isDisarium($number)
{
$originalNumber = $number;
$digitCount = countDigits($number);
$sum = 0;
while ($number != 0)
{
$digit = $number % 10;
$sum += pow($digit, $digitCount);
$digitCount--;
$number = (int)($number / 10);
}
return ($sum == $originalNumber);
}
// Replace this value with your desired number
$inputNumber = 89;
// Call the function to check if the number is Disarium
if (isDisarium($inputNumber))
{
echo "$inputNumber is a Disarium number.\n";
}
else
{
echo "$inputNumber is not a Disarium number.\n";
}
?>
π» Testing the Program
To test the program with different numbers, replace the value of $inputNumber in the script.
89 is a Disarium number.
Run the script to check if the number is a Disarium number.
π§ How the Program Works
- The program defines a function countDigits to count the number of digits in a given number.
- The isDisarium function checks if a number is a Disarium number by summing the digits each raised to the power of its position.
- The main script tests the isDisarium function with a sample number (replace it with your desired number).
π Between the Given Range
Let's examine the PHP code that checks for Disarium numbers in the specified range.
<?php
// Function to check if a number is Disarium
function isDisarium($number)
{
$originalNumber = $number;
$sum = 0;
$length = strlen((string)$number);
while ($number > 0)
{
$digit = $number % 10;
$sum += pow($digit, $length);
$number = (int)($number / 10);
$length--;
}
return $sum === $originalNumber;
}
// Find Disarium numbers in the range 1 to 100
$disariumNumbers = [];
for ($i = 1;$i <= 100;$i++)
{
if (isDisarium($i))
{
$disariumNumbers[] = $i;
}
}
// Output format
echo "Disarium numbers in the range 1 to 100: \n" . implode(' ', $disariumNumbers);
?>
π» Testing the Program
Disarium numbers in the range 1 to 100: 1 2 3 4 5 6 7 8 9 89
Run the script to see the Disarium numbers in the range of 1 to 100.
π§ How the Program Works
- The program defines a function isDisarium that checks if a given number is a Disarium number.
- Inside the function, it calculates the sum of each digit raised to the power of its respective position.
- The program then iterates through numbers from 1 to 100, checking each for Disarium properties.
- Disarium numbers found are stored in an array, and the result is printed in the desired output format.
π§ Understanding the Concept of Disarium Numbers
Before we dive into the code, let's grasp the concept of Disarium numbers.
A Disarium number is a number such that the sum of its digits, each raised to the power of its position, equals the number itself.
For example, the number 89 is a Disarium number because 8^1 + 9^2 equals 89.
π Conclusion
Understanding and implementing programs to identify numeric patterns, such as Disarium numbers, is a valuable skill in the world of programming.
The provided php program offers a practical example of checking whether a given number follows the Disarium pattern.
Feel free to use and modify this code for your specific use cases. Happy coding!
π¨βπ» Join our Community:
Author
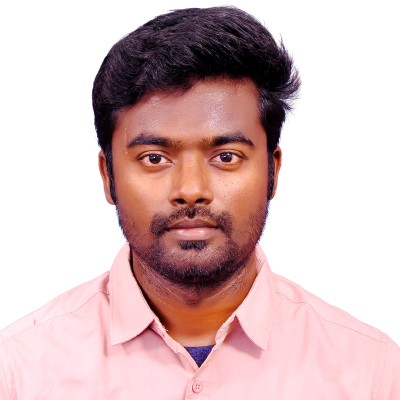
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Check Disarium Number), please comment here. I will help you immediately.