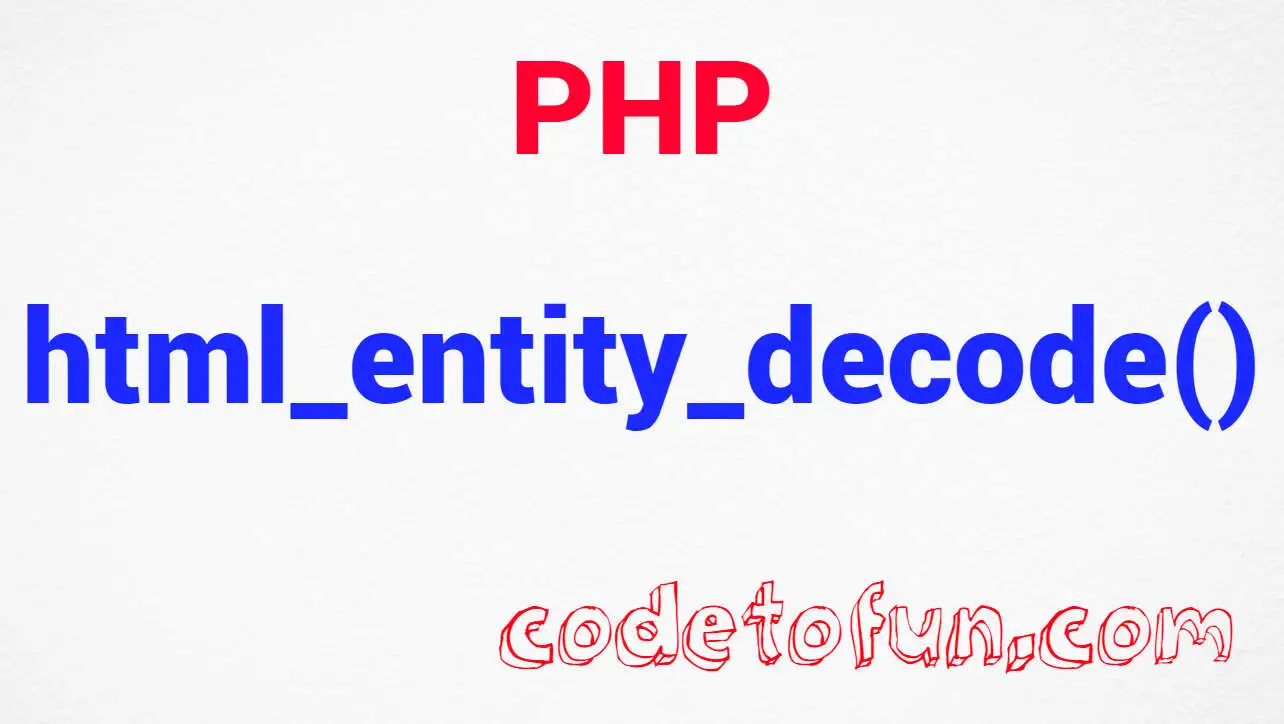
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Check for a Cube Number
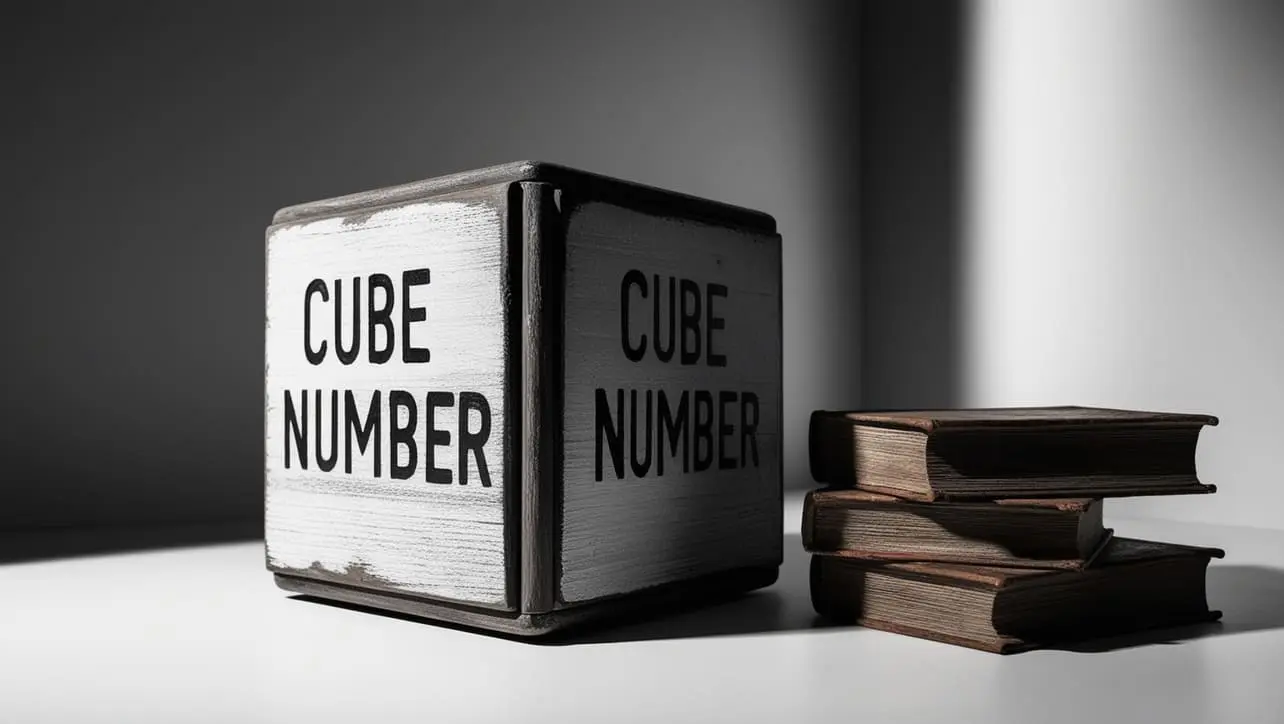
Photo Credit to CodeToFun
π Introduction
In the realm of programming, mathematical properties of numbers often lead to interesting and practical applications. One such property is being a cube number. A cube number is an integer that is the cube of an integer.
In this tutorial, we'll delve into a PHP program that efficiently checks whether a given number is a perfect cube or not.
π Example
Let's take a look at the PHP code that accomplishes this functionality.
<?php
// Function to check if a number is a perfect cube
function isPerfectCube($number)
{
// Calculate the cube root of the number
$cubeRoot = pow($number, 1 / 3);
// Check if the cube root is close to an integer
return abs($cubeRoot - round($cubeRoot)) < 1e-10;
}
// Driver program
// Replace this value with your desired number
$number = 27;
// Check if the number is a perfect cube
if (isPerfectCube($number))
{
echo $number . " is a perfect cube.\n";
}
else
{
echo $number . " is not a perfect cube.\n";
}
?>
π» Testing the Program
To test the program with different numbers, simply replace the value of the $number variable in the script.
27 is a cube number.
Run the script to check if the number is a perfect cube.
π§ How the Program Works
- The program defines a function isPerfectCube that takes a number as input and returns true if it is a perfect cube, and false otherwise.
- Inside the function, it calculates the cube root of the number using the pow function.
- It then checks if the cube root is close to an integer using a tolerance value (1e-10 in this case).
- The main part of the script initializes a variable with a number and calls the isPerfectCube function to determine if it's a perfect cube.
π Between the Given Range
Let's dive into the PHP code that identifies cube numbers in the specified range.
<?php
// Function to check if a number is a cube number
function isCubeNumber($number)
{
// Calculate the cube root
$cubeRoot = round(pow($number, 1 / 3));
// Check if the cube of the cube root is equal to the original number
return ($cubeRoot * $cubeRoot * $cubeRoot == $number);
}
// Display cube numbers in the range 1 to 50
echo "Cube numbers in the range 1 to 50:\n";
for ($i = 1;$i <= 50;++$i)
{
if (isCubeNumber($i))
{
echo "$i ";
}
}
?>
π» Testing the Program
Cube numbers in the range 1 to 50: 1 8 27
Run the script to see the cube numbers in the specified range.
π§ How the Program Works
- The program defines a function isCubeNumber that checks if a given number is a cube number.
- Inside the function, it calculates the cube root of the number and checks if the cube of the cube root is equal to the original number.
- The program then iterates through the numbers from 1 to 50, calling the function to identify and display cube numbers.
π§ Understanding the Concept of Cube Number
A cube number is the result of raising an integer to the power of 3. For example, 2 * 2 * 2 = 8, so 8 is a cube number.
π’ Optimizing the Program
The provided program is straightforward, but you might consider optimizing it further. For instance, you can explore alternative methods for checking if a number is a cube number without using the cbrt function.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
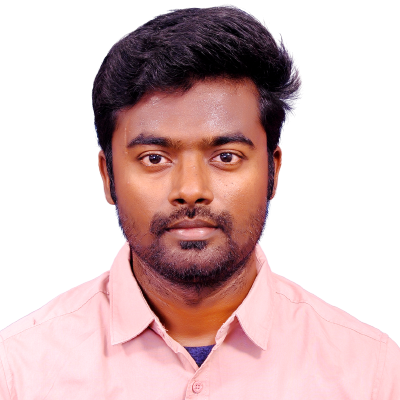
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Check for a Cube Number), please comment here. I will help you immediately.