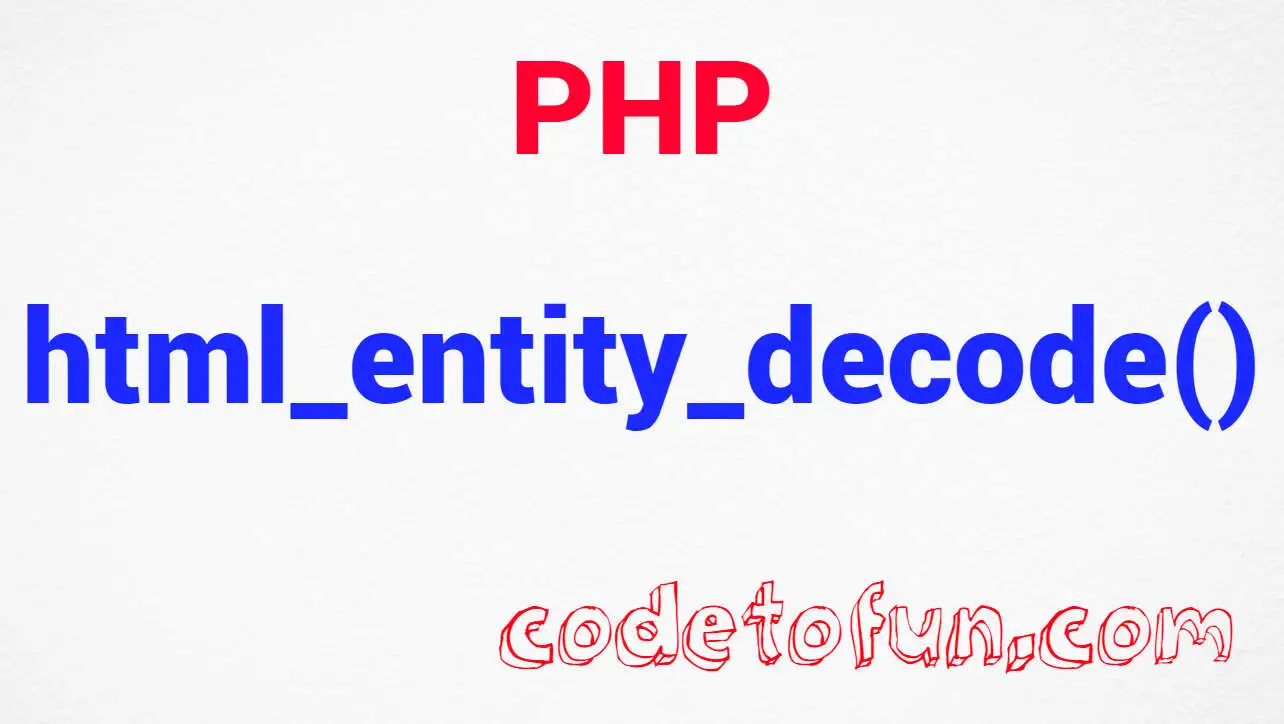
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to find Common Divisors
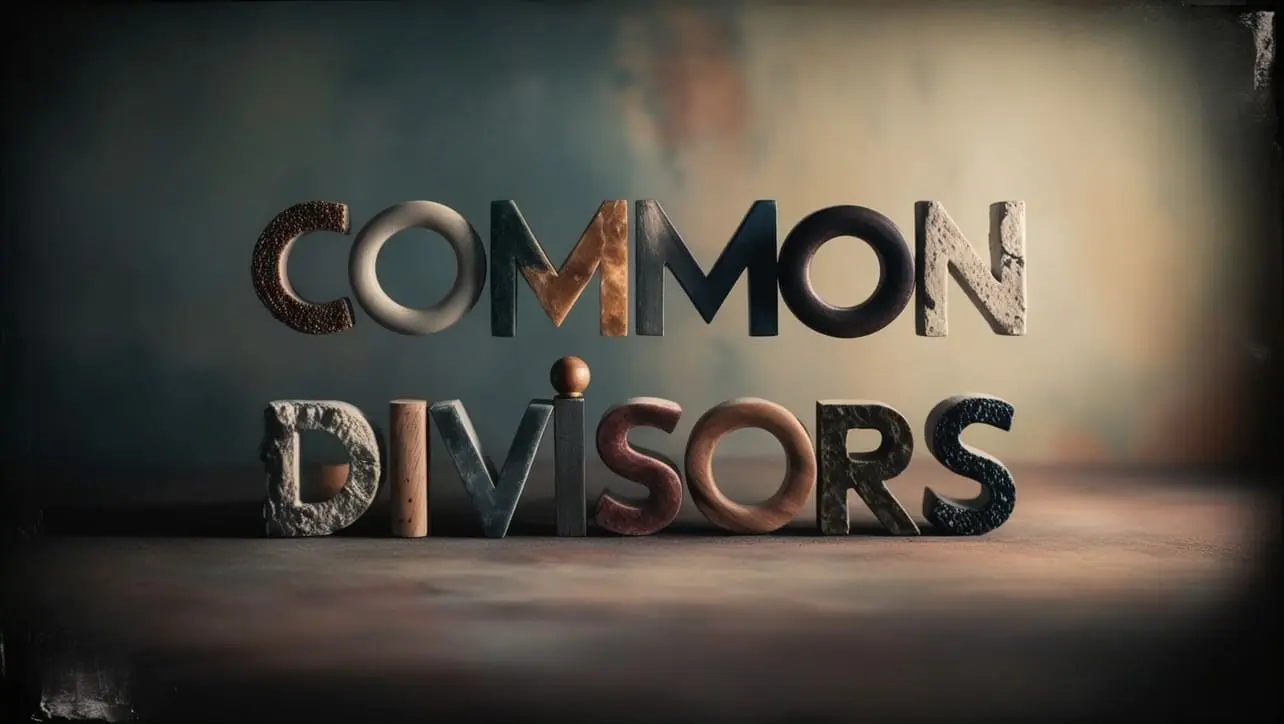
Photo Credit to CodeToFun
π Introduction
In the realm of programming, solving mathematical problems is a common and essential task. One such mathematical problem is finding the common divisors of two numbers. Divisors are numbers that divide another number without leaving a remainder. The common divisors of two numbers are the divisors that both numbers share.
In this tutorial, we will walk through a simple yet effective PHP program to find the common divisors of two numbers. The logic behind this program involves identifying the divisors of each number and then determining which of these divisors are common to both.
π Example
Here's a PHP program to find the common divisors of two numbers:
<?php
// Function to find common divisors
function findCommonDivisors($num1, $num2)
{
echo "Common divisors of $num1 and $num2 are: ";
// Iterate up to the smaller of the two numbers
$limit = min($num1, $num2);
for ($i = 1; $i <= $limit; ++$i) {
// Check if $i is a divisor of both numbers
if ($num1 % $i == 0 && $num2 % $i == 0) {
echo "$i ";
}
}
echo "\n";
}
// Driver program
// Replace these values with your desired numbers
$number1 = 24;
$number2 = 36;
// Call the function to find common divisors
findCommonDivisors($number1, $number2);
?>
π» Testing the Program
To test the program with different numbers, simply replace the values of number1 and number2 in the main function.
Common divisors of 24 and 36 are: 1 2 3 4 6 12
π§ How the Program Works
- The program defines a function findCommonDivisors that takes two numbers as input and prints their common divisors.
- Inside the function, it iterates through numbers from 1 to the smaller of the two input numbers.
- For each iteration, it checks if the current number is a divisor of both input numbers.
- If it is, the number is a common divisor, and it is printed.
π§ Understanding the Concept of Common Divisors
Before delving into the code, let's take a moment to understand the concept of common divisors. In mathematics, a divisor of a number is an integer that divides the number without leaving a remainder. Common divisors of two numbers are divisors that both numbers share.
For example, consider the numbers 12 and 18. The divisors of 12 are 1, 2, 3, 4, 6, and 12. The divisors of 18 are 1, 2, 3, 6, 9, and 18. The common divisors are 1, 2, 3, and 6.
π’ Optimizing the Program
While the provided program is effective, there are ways to optimize it for larger numbers. One optimization involves using the greatest common divisor (GCD) of the two numbers. The GCD is the largest positive integer that divides both numbers without leaving a remainder.
Consider exploring and implementing such optimizations to enhance the efficiency of your program.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
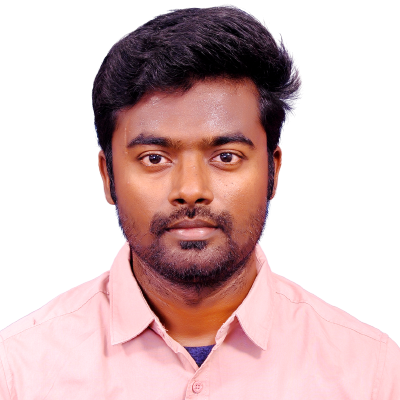
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to find Common Divisors) please comment here. I will help you immediately.