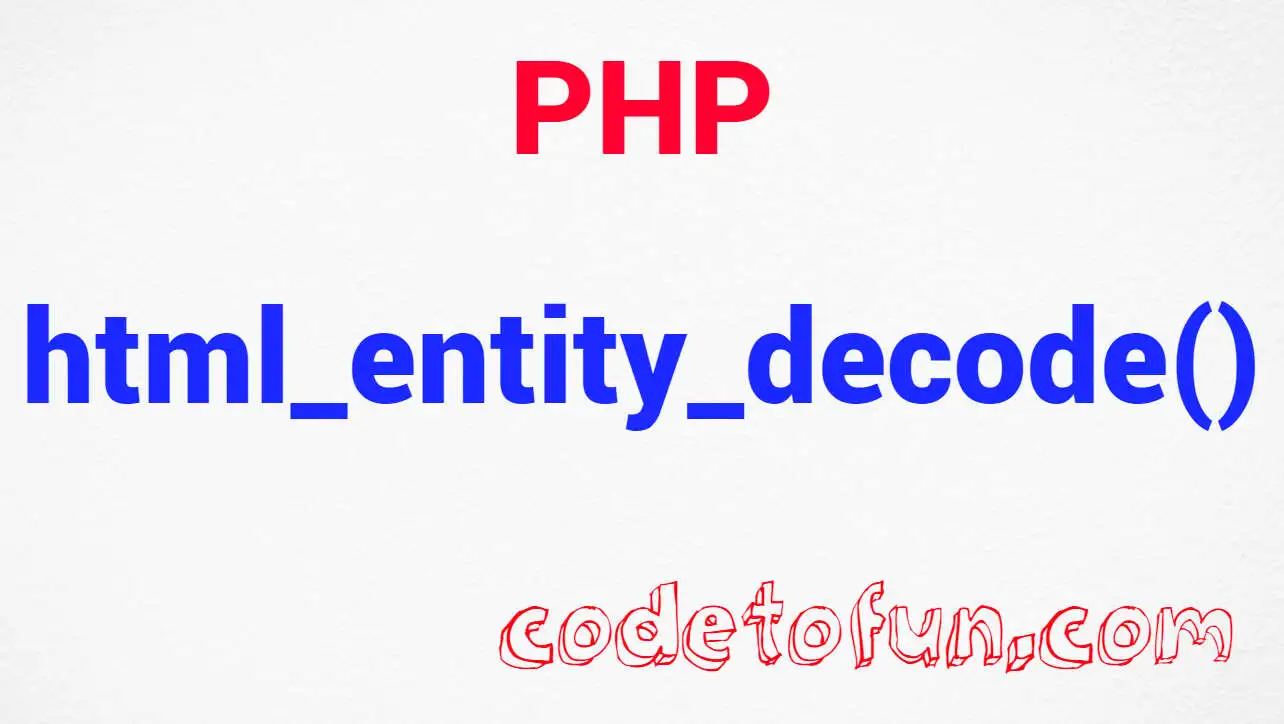
PHP Basic
PHP Interview Programs
- PHP Interview Programs
- PHP Abundant Number
- PHP Amicable Number
- PHP Armstrong Number
- PHP Average of N Numbers
- PHP Automorphic Number
- PHP Biggest of three numbers
- PHP Binary to Decimal
- PHP Common Divisors
- PHP Composite Number
- PHP Condense a Number
- PHP Cube Number
- PHP Decimal to Binary
- PHP Decimal to Octal
- PHP Disarium Number
- PHP Even Number
- PHP Evil Number
- PHP Factorial of a Number
- PHP Fibonacci Series
- PHP GCD
- PHP Happy Number
- PHP Harshad Number
- PHP LCM
- PHP Leap Year
- PHP Magic Number
- PHP Matrix Addition
- PHP Matrix Division
- PHP Matrix Multiplication
- PHP Matrix Subtraction
- PHP Matrix Transpose
- PHP Maximum Value of an Array
- PHP Minimum Value of an Array
- PHP Multiplication Table
- PHP Natural Number
- PHP Number Combination
- PHP Odd Number
- PHP Palindrome Number
- PHP Pascalβs Triangle
- PHP Perfect Number
- PHP Perfect Square
- PHP Power of 2
- PHP Power of 3
- PHP Pronic Number
- PHP Prime Factor
- PHP Prime Number
- PHP Smith Number
- PHP Strong Number
- PHP Sum of Array
- PHP Sum of Digits
- PHP Swap Two Numbers
- PHP Triangular Number
PHP Program to Check Abundant Number
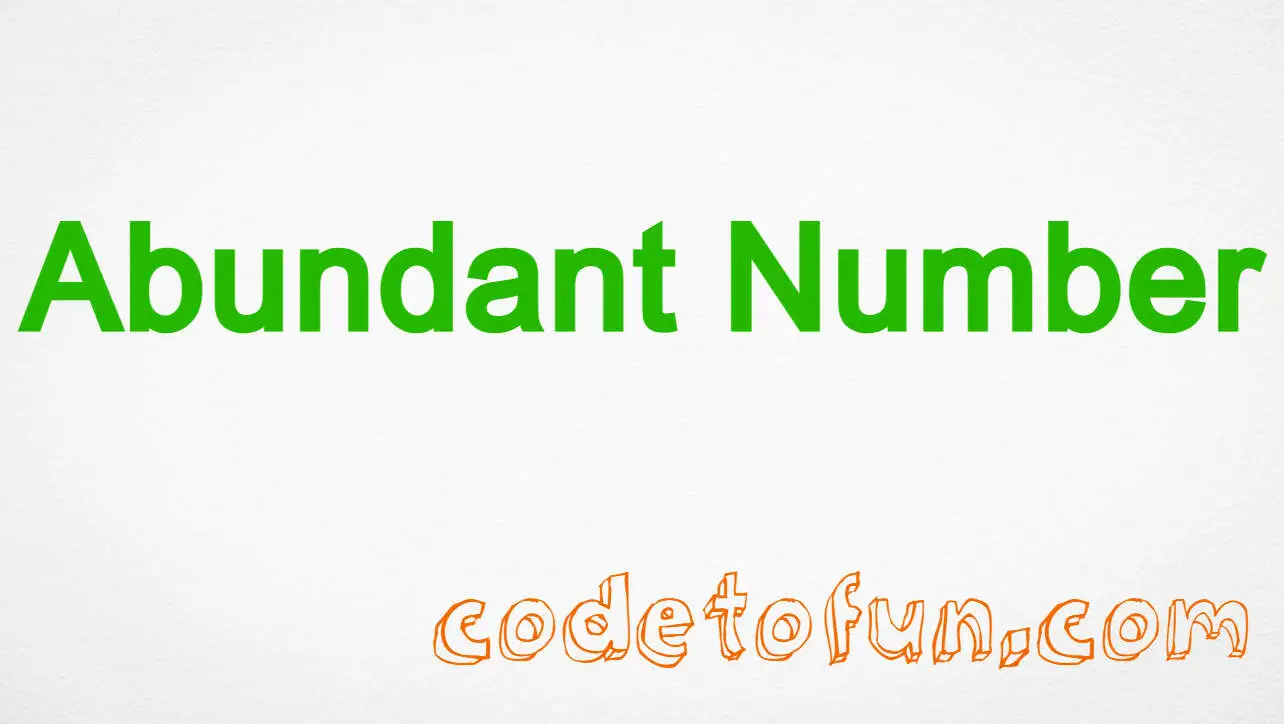
Photo Credit to CodeToFun
π Introduction
In the realm of programming, understanding and identifying special types of numbers is a fascinating exploration. One such category is abundant numbers.
An abundant number, also known as an excessive number, is a positive integer that is smaller than the sum of its proper divisors (excluding itself).
In this tutorial, we will delve into a PHP program designed to check whether a given number is an abundant number or not.
The program will employ logic to calculate the sum of the proper divisors of a number and determine if it exceeds the number itself.
π Example
Let's take a look at the PHP code that achieves this functionality.
<?php
// Function to check if a number is abundant
function isAbundant($num)
{
$sum = 1; // Start with 1 as every number is divisible by 1
// Iterate from 2 to the square root of the number
for ($i = 2;$i * $i <= $num;++$i)
{
if ($num % $i == 0)
{
$sum += $i;
if ($i != $num / $i)
{
$sum += $num / $i;
}
}
}
// If the sum of divisors exceeds the number, it is abundant
return $sum > $num;
}
// Driver program
// Replace this value with your desired number
$number = 12;
// Check if the number is abundant
if (isAbundant($number))
{
echo "$number is an abundant number.\n";
}
else
{
echo "$number is not an abundant number.\n";
}
?>
π» Testing the Program
To test the program with different numbers, simply replace the value of $number in the code.
12 is an abundant number.
Run the script to check if the number is an abundant number.
π§ How the Program Works
- The program defines a function isAbundant that takes a number as input and returns true if the number is abundant and false otherwise.
- Inside the function, it iterates through numbers from 2 to the square root of the given number to find its divisors.
- For each divisor found, it adds it to the sum, including the corresponding divisor on the other side of the square root.
- Finally, it compares the sum of divisors with the original number to determine if it is abundant.
π Between the Given Range
Let's explore the PHP code that checks for abundant numbers within the given range.
<?php
// Function to check if a number is abundant
function isAbundant($number)
{
$sum = 0;
// Find divisors and calculate their sum
for ($i = 1;$i <= $number / 2;++$i)
{
if ($number % $i == 0)
{
$sum += $i;
}
}
return $sum > $number;
}
// Given range: 1 to 50
$lowerLimit = 1;
$upperLimit = 50;
echo "Abundant numbers between $lowerLimit and $upperLimit are: ";
// Check and display abundant numbers in the range
for ($i = $lowerLimit;$i <= $upperLimit;++$i)
{
if (isAbundant($i))
{
echo "$i ";
}
}
?>
π» Testing the Program
Abundant numbers between 1 and 50 are: 12 18 20 24 30 36 40 42 48
Run the script, and it will output the abundant numbers between 1 and 50.
π§ How the Program Works
- The program defines a function isAbundant that checks if a given number is abundant.
- Inside the function, it iterates through potential divisors and calculates their sum.
- The main section then specifies the range (1 to 50) and checks for and displays abundant numbers within that range.
π§ Understanding the Concept of Abundant Number
Before diving into the code, let's take a moment to understand abundant numbers. An abundant number is a positive integer for which the sum of its proper divisors (excluding itself) is greater than the number itself.
For example, the number 12 is abundant because its divisors (excluding 12) are 1, 2, 3, 4, 6, and the sum of these divisors is 16, which is greater than 12.
π’ Optimizing the Program
While the provided program is effective, there are opportunities for optimization, such as caching previously calculated divisor sums to avoid redundant computations.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
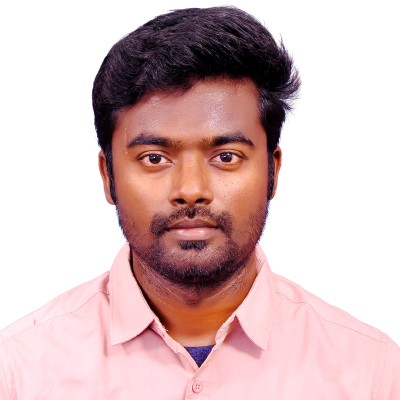
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP Program to Check Abundant Number), please comment here. I will help you immediately.